Java programming - Introduction of Array Concept and Algorithm
Summary
TLDRThis video introduces Java arrays, explaining their declaration, initialization, and element access. It covers how to loop through arrays using both traditional and enhanced `for` loops, and demonstrates operations such as summing, averaging, and searching for elements. The video also discusses copying arrays and using linear search techniques. Key concepts like array length, constant array sizes, and displaying arrays vertically are explored. By the end, viewers will have a solid understanding of how to work with arrays in Java, from basic operations to more advanced techniques.
Takeaways
- π Arrays are sequences of values with the same type, and they can be declared in different ways depending on the programming language (e.g., Java, C#, Python).
- π In Java, arrays are declared with a type followed by square brackets (e.g., `int[] numbers`).
- π To initialize an array in Java, you need to use the `new` keyword along with the type and size (e.g., `new int[10]` for an integer array of size 10).
- π Array indices start at 0, meaning the first element is accessed with index 0, the second with index 1, and so on.
- π You can initialize an array with specific values using curly braces, such as `new int[] {10, 20, 30, 40, 50}`.
- π The `for` loop is the most common way to iterate over an array and retrieve or manipulate its elements. It is used to access each index from 0 to the arrayβs length.
- π To display all elements in an array, you can use a `for` loop with the array length, and print each element one by one.
- π Java also supports enhanced `for` loops (also called 'foreach'), which provide a simpler and more readable way to iterate through an array's elements.
- π Array operations often include summing values, calculating averages, and finding the maximum or minimum values. A typical approach is to use a loop for these calculations.
- π When performing searches in arrays, a linear search (using a `while` loop) can be used to find if a specific value exists. If found, a boolean variable (e.g., `found`) is set to true.
- π Copying an array requires a loop, as direct assignment (e.g., `array1 = array2`) only copies references, not the values themselves.
Q & A
What is an array, and how is it different from a regular variable in Java?
-An array is a collection of elements of the same type stored in contiguous memory locations. It allows you to store multiple values in a single variable, whereas a regular variable holds only one value of a specified type.
How do you declare and initialize an array in Java?
-In Java, you declare an array by specifying the type followed by square brackets (e.g., `int[] numbers`). To initialize an array, you can either use the `new` keyword with a specified size (e.g., `new int[10]`) or directly assign values in curly braces (e.g., `{10, 20, 30, 40}` for an array of integers).
What does the 'new' keyword do when initializing an array in Java?
-'New' is used to create an instance of an array in Java. When you use the `new` keyword, you are allocating memory for the array, and it also requires you to specify the array's size.
What does array indexing in Java mean, and how does it work?
-Array indexing in Java means accessing elements of an array using their position or index. Java arrays are zero-indexed, meaning the first element is at index 0, the second at index 1, and so on.
How do you loop through an array in Java and print all its elements?
-You can loop through an array using a `for` loop by iterating from `0` to `array.length - 1`. Inside the loop, you access each element using the array's index (e.g., `numbers[i]`). An enhanced `for` loop can also be used to iterate directly over the elements.
How can you calculate the sum of all elements in an array?
-To calculate the sum of all elements in an array, you can iterate through the array using a loop and accumulate the values in a variable. For example, using a `for` loop: `total += numbers[i];`.
What is a linear search, and how do you implement it to find an element in an array?
-A linear search is a method of finding a specific element in an array by checking each element sequentially from the beginning until the element is found. You can implement it by using a loop that compares each array element to the target value.
What is the difference between a regular `for` loop and an enhanced `for` loop when working with arrays?
-The regular `for` loop requires you to manually manage the loop counter and index to access array elements, whereas the enhanced `for` loop automatically iterates over the array's elements, simplifying the syntax.
How can you copy the contents of one array into another in Java?
-You can copy the contents of one array into another by using a loop to assign each element individually, or you can use the `System.arraycopy()` method to copy the entire array efficiently.
How can you modify the display of array elements, such as adding separators or formatting output?
-You can modify the display of array elements by adding conditional checks inside the loop. For example, you can print a separator (like a vertical bar) after each element except the last one by checking the loop index (`if (i < array.length - 1)`), and then print a space or bar accordingly.
Outlines
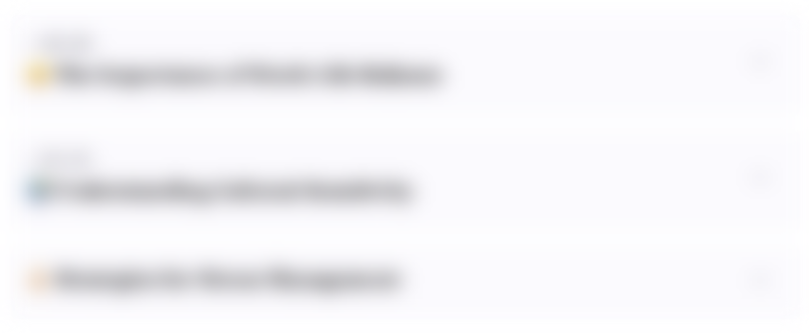
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
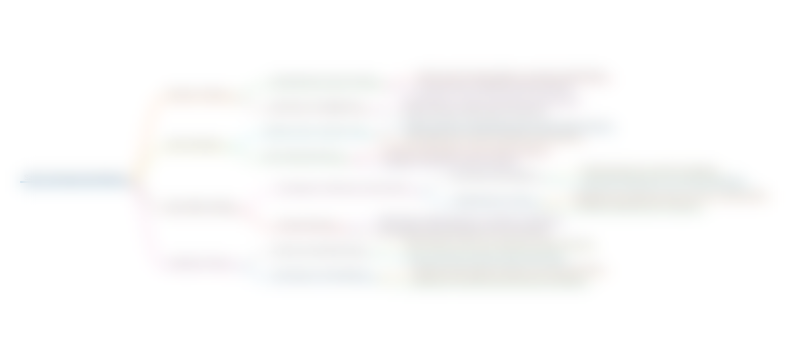
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
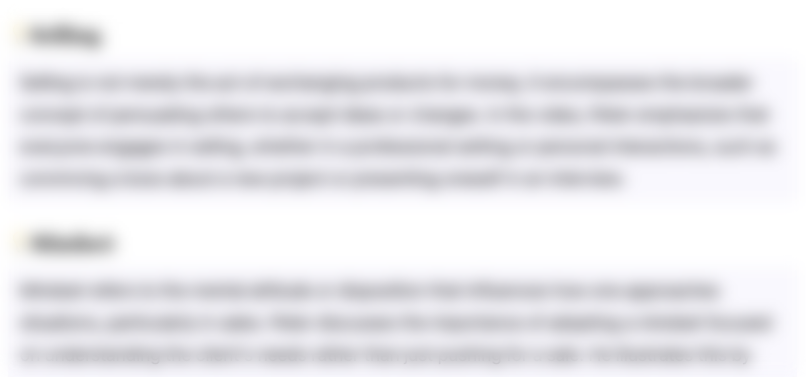
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
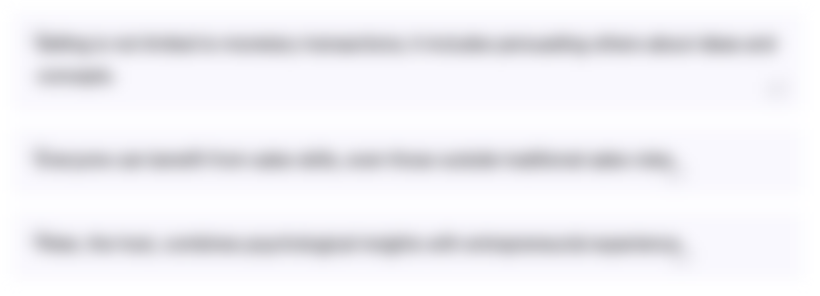
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
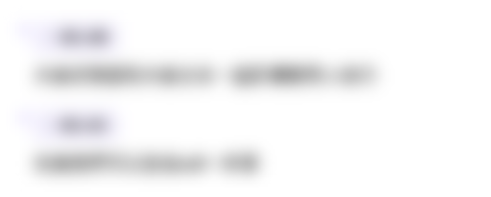
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)