Learn C# Scripting for Unity in 15 Minutes (2024 Working)
Summary
TLDRIn this Unity tutorial by Raja from Charger Games, viewers are guided through basic C# scripting techniques in Unity within 10-15 minutes. The video covers essential topics such as object destruction, keyboard and mouse input handling, physics integration with rigidbodies, collision detection, and loading new scenes. Additionally, it demonstrates how to display text on screen and control game objects using arrow keys. The tutorial is fast-paced, offering practical, step-by-step instructions to help users quickly grasp Unity scripting fundamentals.
Takeaways
- 🚀 The video focuses on learning C# scripting in Unity within 10-15 minutes, using a 3D cube as the example.
- 📦 Start by creating a C# script, attaching it to the cube, and learning the basics of the Start and Update functions.
- 💥 The Destroy function can be used to remove the game object immediately or after a delay, such as 3 seconds.
- 🖱️ The OnMouseDown function allows you to destroy the object when clicked with the mouse.
- ⌨️ You can also use keyboard inputs, such as pressing the spacebar, to destroy the object using the Input.GetKeyDown function.
- 🏀 Physics in Unity is demonstrated by adding a Rigidbody component to the cube, allowing it to react to gravity.
- 💨 Rigidbody forces, like AddForce, can make the cube jump or move by pressing keys, adding velocity in specific directions.
- 🔄 Collisions with other objects can be detected using the OnCollisionEnter function, which checks the object's tag and triggers actions like destroying an object.
- 🎮 Scene management is introduced, where pressing a key like 'R' loads a new level or scene in Unity using SceneManager.
- 📝 Text elements, such as displaying 'You Win' on collision events, are also covered using Unity's UI system and GameObject.SetActive.
Q & A
What is the purpose of the 'Start' and 'Update' functions in Unity scripts?
-The 'Start' function is called at the start of the game when the game begins, while the 'Update' function is called repeatedly every single frame.
How can you destroy a game object in Unity using a script?
-You can destroy a game object by calling the 'Destroy' function with the game object as its argument, typically within the 'Start' or 'Update' function.
What does the 'OnMouseDown' function do in Unity scripts?
-The 'OnMouseDown' function is called when the object with the script attached is clicked with the mouse.
How can you make a game object respond to keyboard inputs in Unity?
-You can use the 'Input.GetKeyDown' method to check if a specific key is pressed and then perform an action, such as destroying the object, in response.
What is a Rigidbody component and how does it affect a game object in Unity?
-A Rigidbody component makes a game object part of Unity's physics engine, allowing it to have mass and be affected by gravity and other forces.
How can you add force to a game object in Unity to make it move?
-You can add force to a game object by accessing its Rigidbody component and calling 'RB.AddForce' with a Vector3 direction and a force amount.
What does the 'OnCollisionEnter' function do in Unity scripts?
-The 'OnCollisionEnter' function is called automatically when the game object collides with another game object, allowing you to perform actions based on the collision.
How can you detect if a game object has collided with a specific tag in Unity?
-You can check the tag of the colliding object within the 'OnCollisionEnter' function using 'collision.gameObject.tag' and compare it to the desired tag.
What is the process to load a new scene in Unity when a certain condition is met?
-To load a new scene, you can use the 'SceneManager.LoadScene' function with the scene name as its argument after ensuring the scene is added to the build settings.
How can you display text on the screen in Unity using UI?
-You can create a UI Text element, position it, set the text properties, and then activate or deactivate it using script by setting the 'SetActive' property to true or false.
What is the role of the horizontal and vertical axis in controlling game object movement in Unity?
-The horizontal axis typically corresponds to left and right arrow keys, while the vertical axis corresponds to up and down arrow keys. You can use these axis values to control the movement of a game object.
Outlines
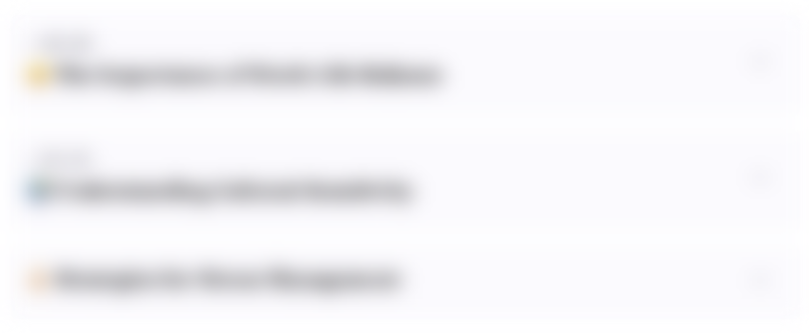
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
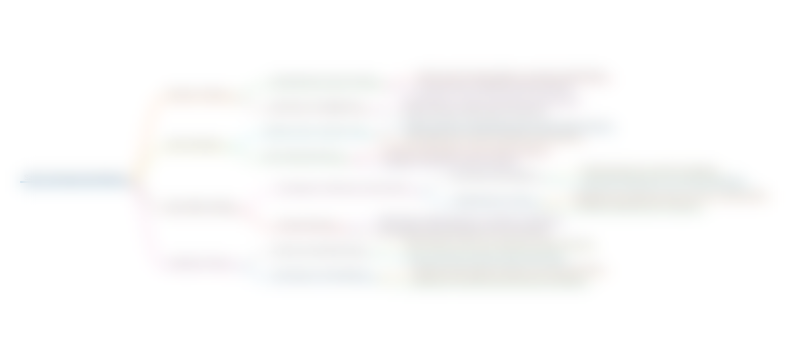
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
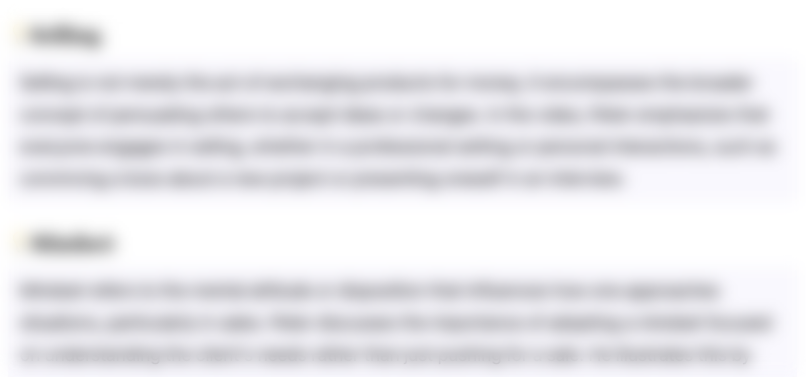
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
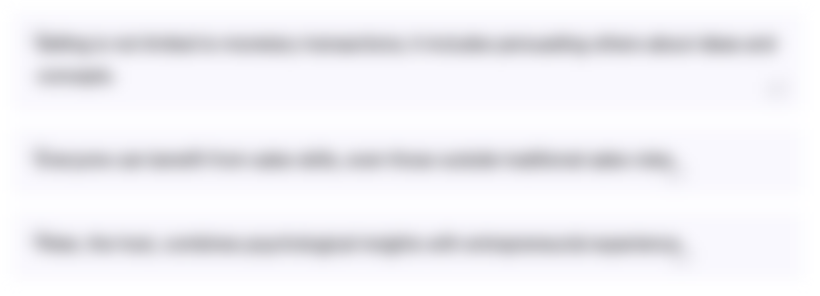
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
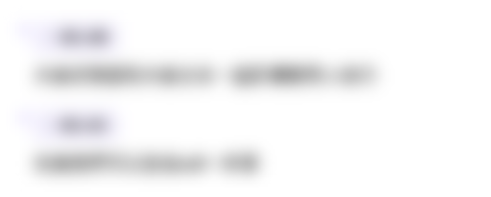
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
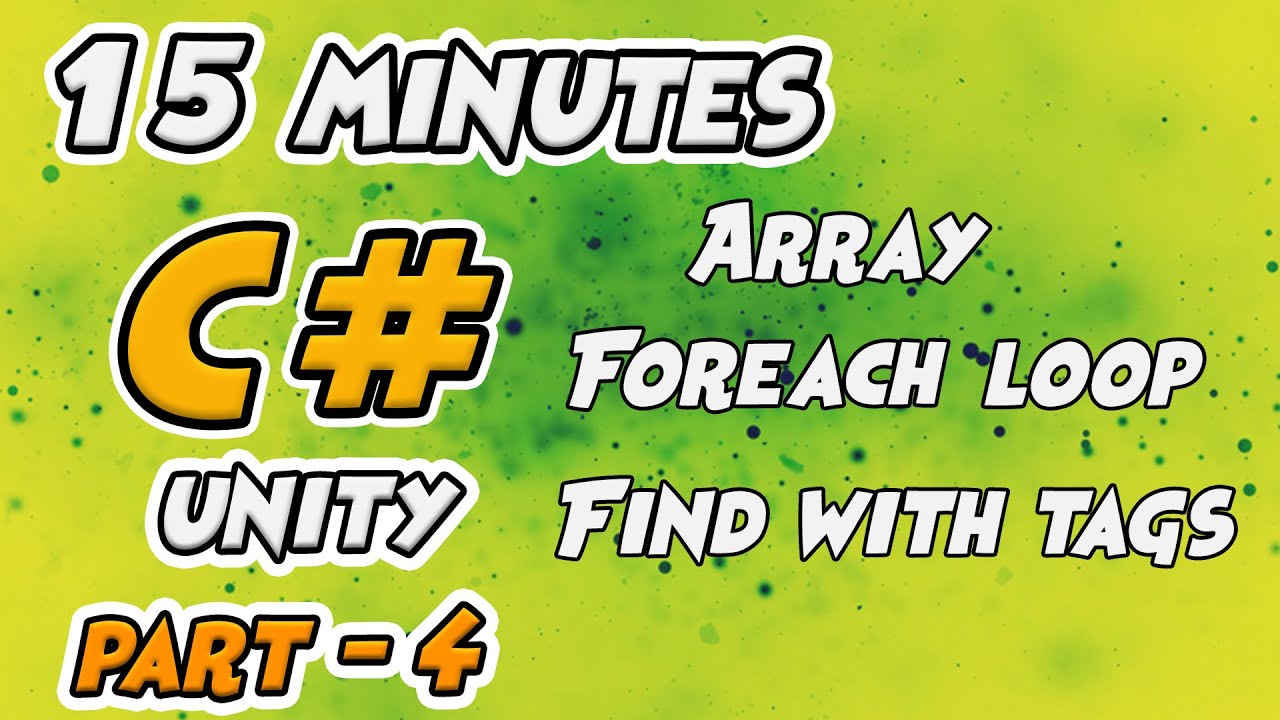
Learn C# Scripting for Unity 15 Minutes - Part 4 - Foreach Loop, Array, Find Tags
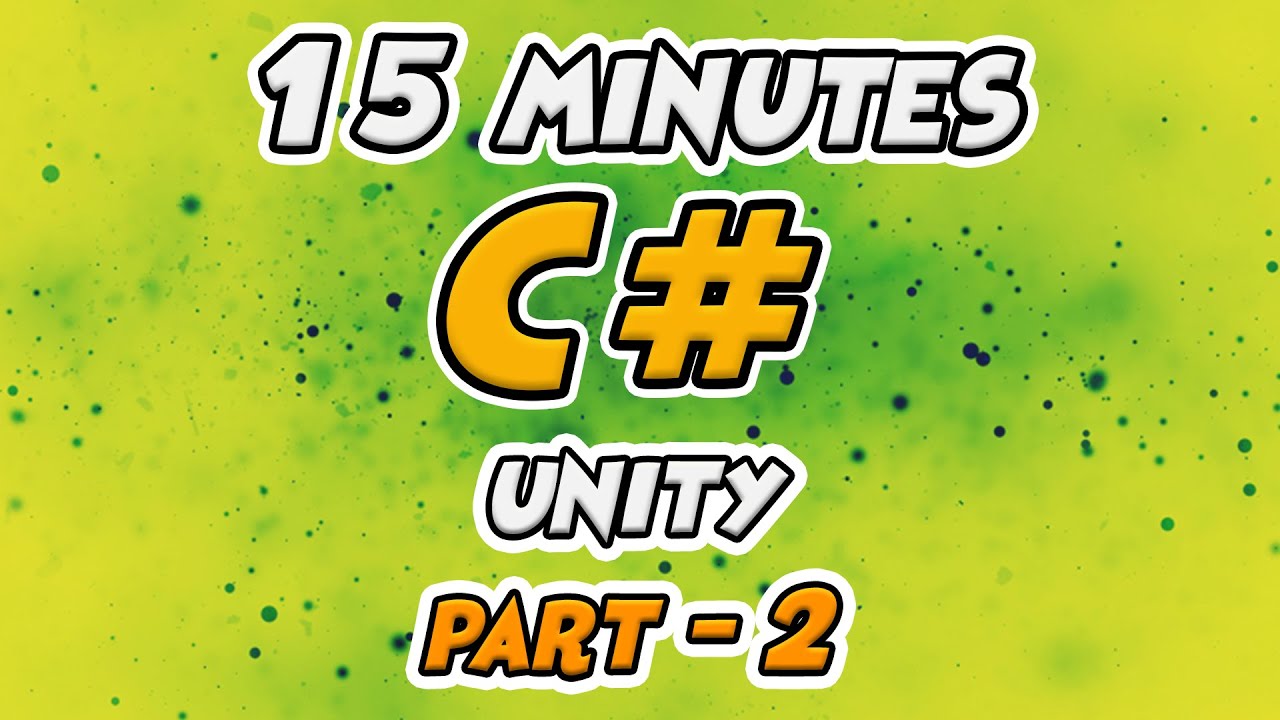
Learn C# Scripting For Unity in 15 Minutes - Part 2
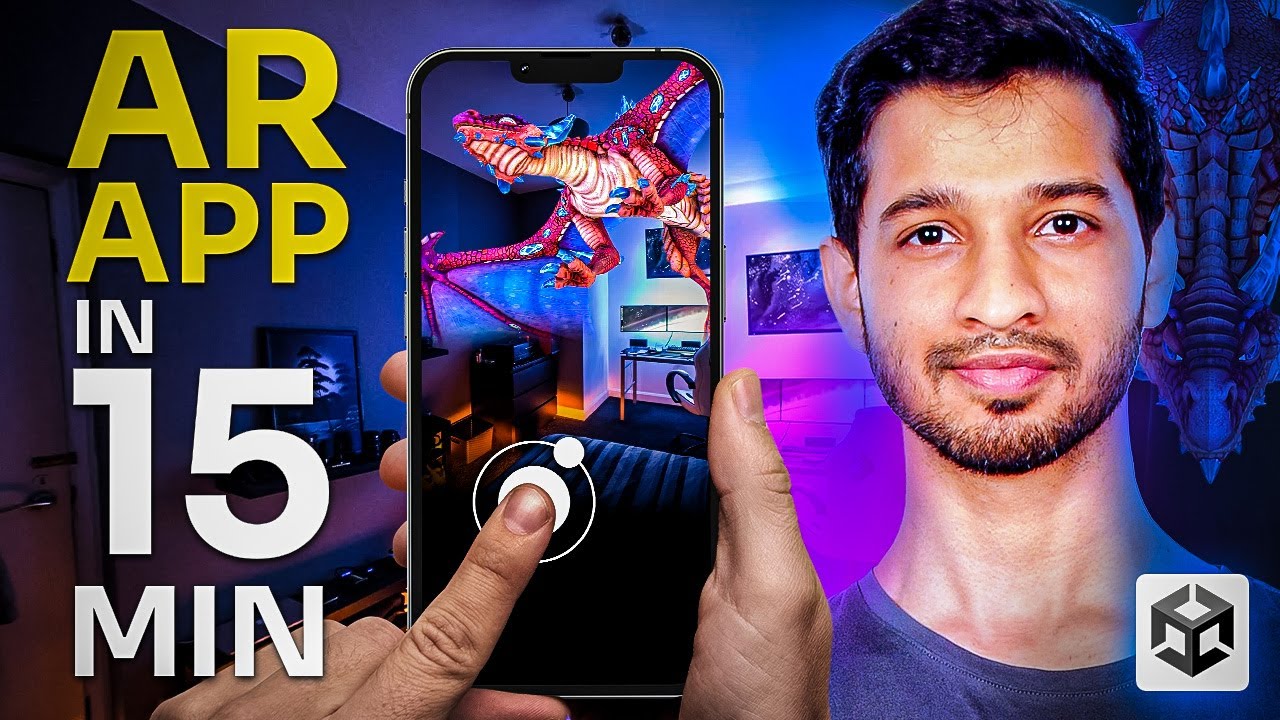
Let’s Make an AR App in 15 MINUTES!! (Beginner Friendly)
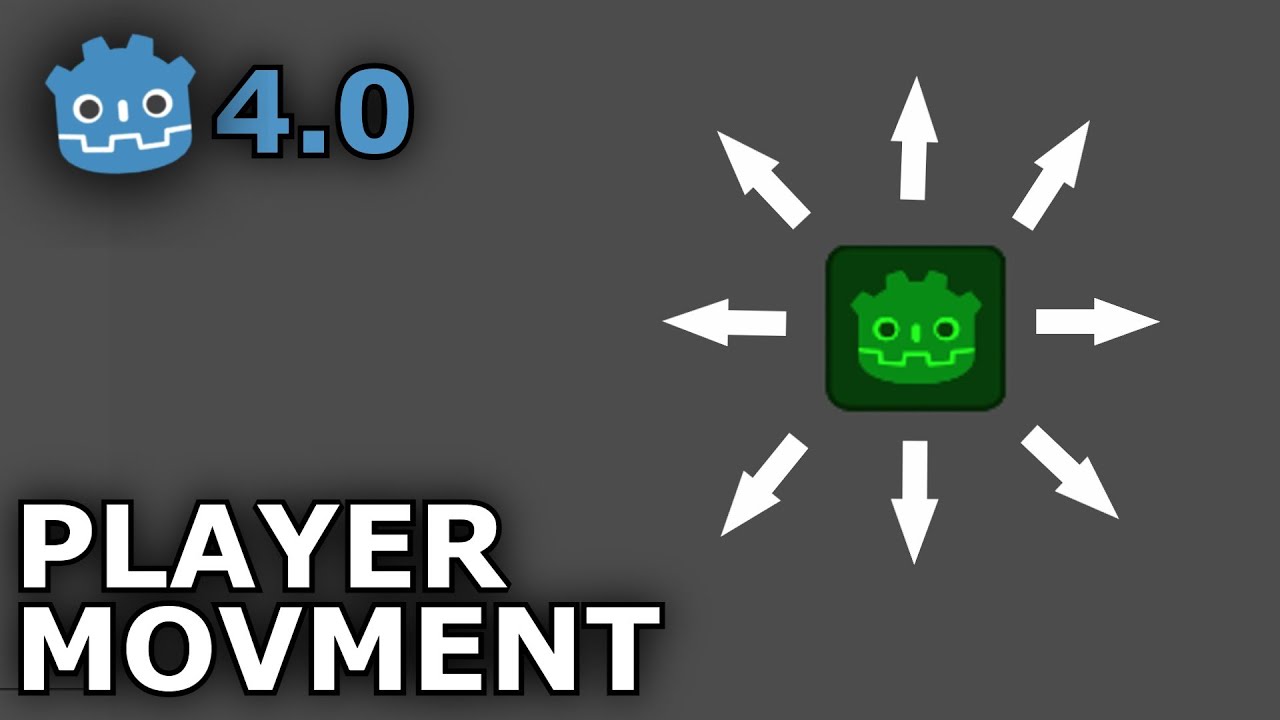
How to Create SMOOTH Player Movement in Godot 4.0
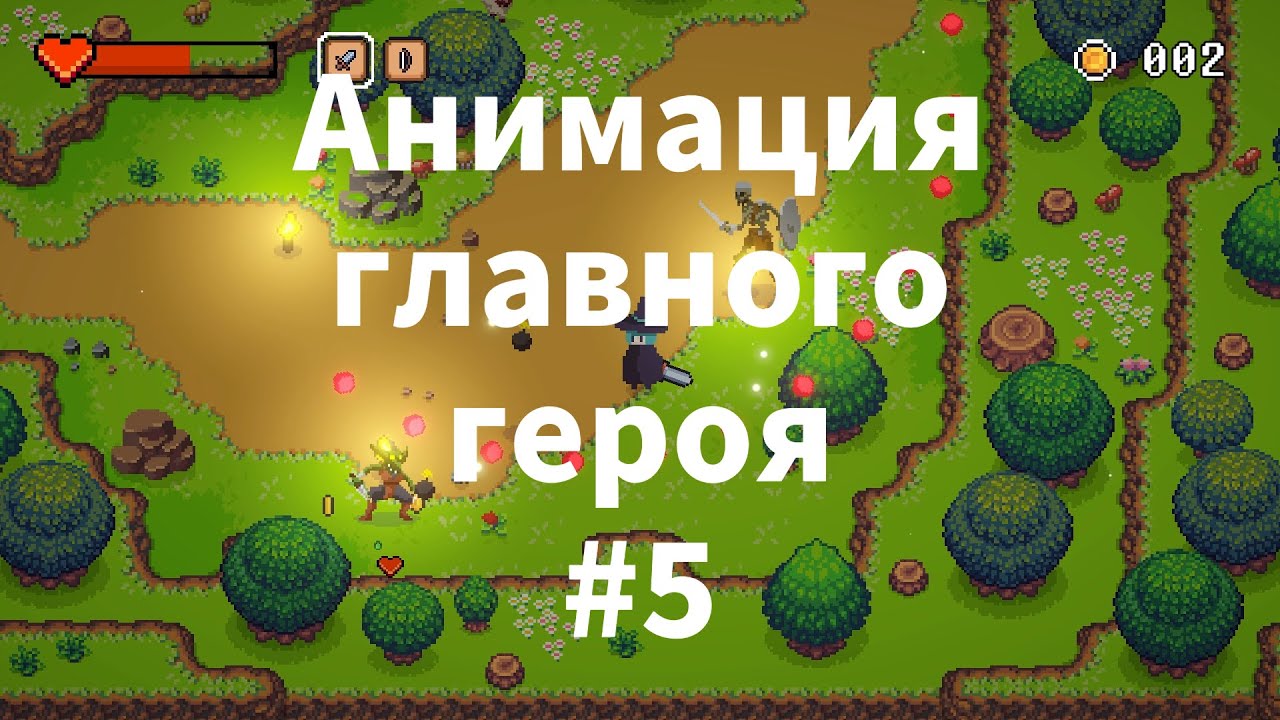
2D Top Down игра на Unity с нуля #5 | Анимация главного героя
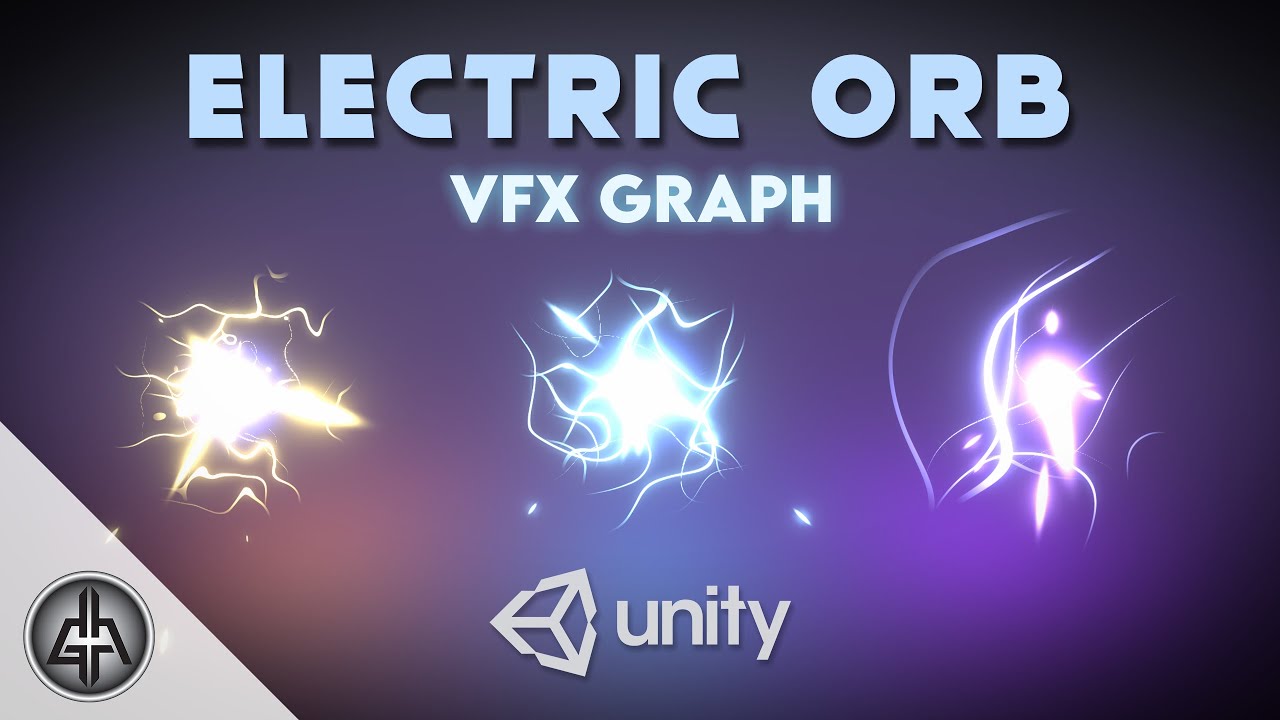
Unity VFX Graph - Electricity Tutorial (Procedural Shader)
5.0 / 5 (0 votes)