PYTHON While Loop with Sentinel Value
Summary
TLDRThe video explains how loops work in programming, specifically focusing on while loops and sentinel values. It highlights the importance of having a condition, like pressing 'q', to exit a loop. Without such a condition, the loop would run infinitely. The example provided shows a program that prints a face using characters, allowing the user to choose the character for the eyes and mouth. The loop continues until the user presses 'q', which stops the program and prints 'Goodbye'. Sentinel values, such as 'q', help control loop execution and prevent infinite loops.
Takeaways
- 🔄 Loops are used to repeat the same task multiple times in a program.
- ⚠️ An infinite loop occurs if there is no condition to exit the loop.
- 🛑 Pressing 'q' will allow the user to exit the loop in the program example.
- 📊 The loop can be used for tasks like summing quantities in an inventory.
- 💡 A sentinel value, such as 'q', is a predefined value used to exit a loop.
- 🧠 Another way to exit a loop is using a condition like entering a negative number.
- 👀 The program example allows the user to change the character for the face, except for the nose, which is always a '0'.
- 🔠 The eyes and mouth start as default dashes but can be changed by the user.
- 🔁 As long as the user doesn't press 'q', the loop will continue asking for a new character.
- 👋 When the user presses 'q', the loop exits and the program says 'Goodbye'.
Q & A
What is the purpose of using loops in programming?
-Loops are used to repeat the same task multiple times in a program, allowing for efficient execution of repetitive actions without duplicating code.
What can happen if a loop doesn't have an exit condition?
-If a loop lacks an exit condition, it can result in an infinite loop, which continues indefinitely and prevents the program from progressing.
How can a user exit the loop in the example provided?
-In the example, the user can exit the loop by pressing the letter 'q', which serves as the sentinel value to terminate the loop.
What is a sentinel value in programming?
-A sentinel value is a specific value used to indicate the end of a loop. In this case, 'q' is the sentinel value that tells the program to stop the loop.
What does the program in the example do?
-The program allows the user to print a face made of characters, where they can choose the character for the eyes and mouth. The nose is always set to zero. The loop continues until the user presses 'q' to quit.
How does the program handle input from the user?
-The program takes user input to determine which character to use for the face. If the user enters 'q', the loop ends, and the program prints 'Goodbye'. Otherwise, it continues to ask for more input.
What is the default face printed when the program starts?
-Initially, the face is printed with dashes for the eyes and mouth, and the nose is always a zero.
What happens when the user enters a different character (e.g., 'x' or '@')?
-When the user enters a different character, such as 'x' or '@', the program updates the face using those characters for the eyes and mouth, while the nose remains as a zero.
Why does the nose always remain a zero in the program?
-The nose is hardcoded to always be a zero, regardless of what characters the user enters for the eyes and mouth.
How does the loop know when to stop in the example?
-The loop checks if the user's input is equal to 'q'. If it is, the loop terminates and the program prints 'Goodbye'. If it's not, the loop continues to ask for new input.
Outlines
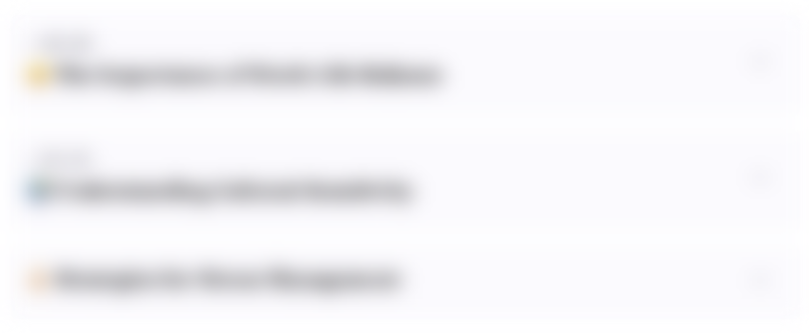
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
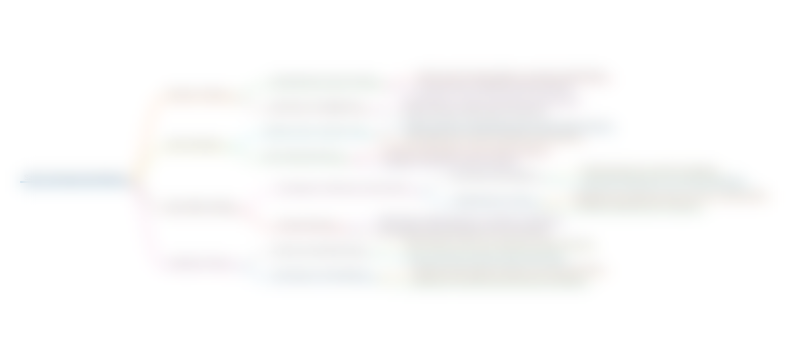
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
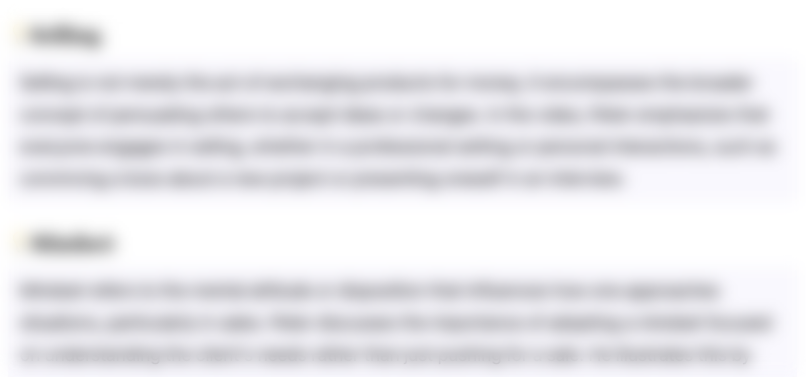
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
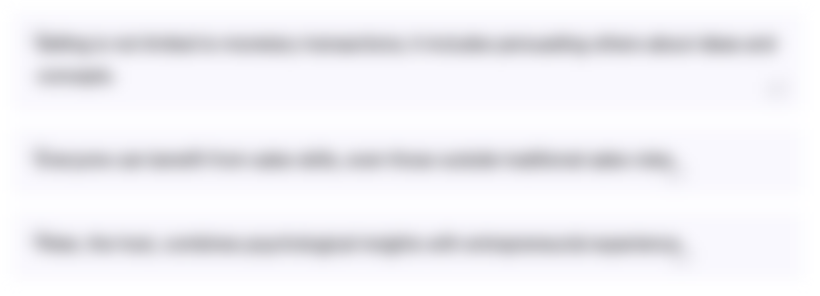
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
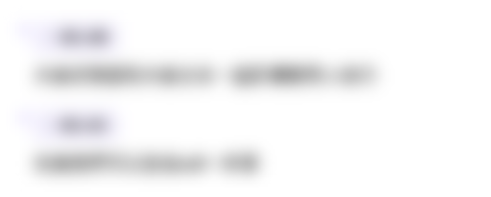
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
5.0 / 5 (0 votes)