Algorithms: Binary Search
Summary
TLDRIn this educational video, Gayle Laakmann McDowell, author of 'Cracking the Coding Interview,' introduces binary search, an efficient algorithm for finding an item in a sorted array. She uses a classroom example with 300 papers to illustrate the concept, highlighting how it quickly narrows down the search space. McDowell explains that binary search operates by repeatedly dividing the array in half, comparing the midpoint with the target, and focusing on the relevant half. The video covers both recursive and iterative implementations, emphasizing the importance of working with sorted data. She challenges viewers to apply binary search to new data types, like strings, after understanding its basic principles.
Takeaways
- 📚 Binary search is an intuitive algorithm used for finding an item in a sorted list by repeatedly dividing the search interval in half.
- 👨🏫 The concept is explained with an analogy of a teacher looking for a student's paper in an alphabetized stack.
- 🔍 The algorithm starts by comparing the target value to the middle element of the array.
- ✅ If the value matches, the search is successful. If not, the search continues in the half where the value could be, based on whether it's less than or greater than the midpoint.
- 📉 The search space is reduced by half with each comparison, making binary search very efficient.
- 🕒 The worst-case time complexity of binary search is O(log n), where 'n' is the number of elements in the array.
- 💻 Binary search can be implemented both recursively and iteratively.
- 🛠️ A common pitfall is integer overflow when calculating the midpoint; this can be avoided by using the formula `(left + right) / 2` instead of `(left + (right - left) / 2)`.
- 🔄 The iterative implementation involves a while loop that continues until the target is found or the search space is exhausted.
- 🔢 Binary search is applicable to any sorted data type, including integers, strings, or other ordered collections.
- 🚀 The script challenges viewers to try implementing binary search on a new data type, such as a string, to reinforce the concept.
Q & A
What is the main concept of binary search as described in the transcript?
-Binary search is an algorithm for finding an item from a sorted list of items. It works by repeatedly dividing in half the portion of the list that could contain the item, until you've narrowed down the possible locations to just one.
How does the teacher-student example illustrate the binary search concept?
-The teacher-student example illustrates binary search by showing how a teacher would efficiently find a student's paper from an alphabetized stack by dividing the stack in half each time and comparing the midpoint with the student's name until the paper is found.
Why is it important for the array to be sorted before performing a binary search?
-The array must be sorted because binary search relies on the order of elements to determine whether to search the left or right half of the array. Without a sorted array, the algorithm would not function correctly.
What is the time complexity of binary search?
-The time complexity of binary search is O(log n), where n is the number of elements in the array. This is because with each comparison, the search space is reduced by half.
How does the recursive implementation of binary search work?
-In the recursive implementation, the binary search function calls itself with adjusted left and right boundaries until it either finds the target value or determines that the value is not in the array.
What is the potential pitfall mentioned in the script regarding the recursive implementation?
-The potential pitfall is integer overflow when calculating the midpoint by adding left and right together. To avoid this, the midpoint can be calculated as (left + (right - left) / 2) instead.
How is the iterative implementation of binary search different from the recursive one?
-The iterative implementation uses a while loop to repeatedly adjust the left and right boundaries without the need for recursive function calls, making it more memory efficient.
What is the condition to exit the while loop in the iterative binary search?
-The while loop in the iterative binary search exits when the left boundary is greater than the right, indicating that the target value is not in the array or the search space has been exhausted.
Can binary search be applied to data types other than integers?
-Yes, binary search can be applied to other data types such as strings, as long as the data is sorted and comparable.
What is the challenge given to the viewer at the end of the transcript?
-The challenge given to the viewer is to try implementing binary search on a new data type like a string.
Outlines
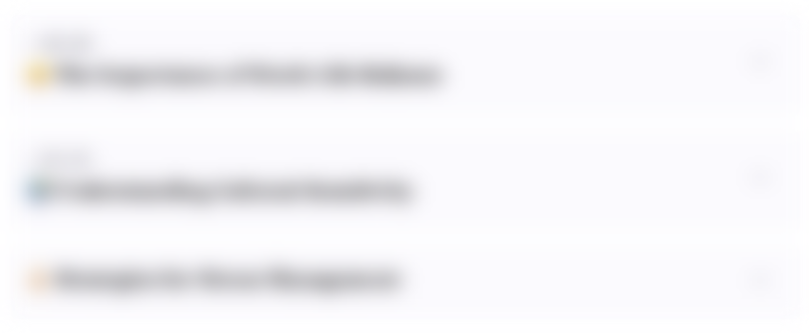
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
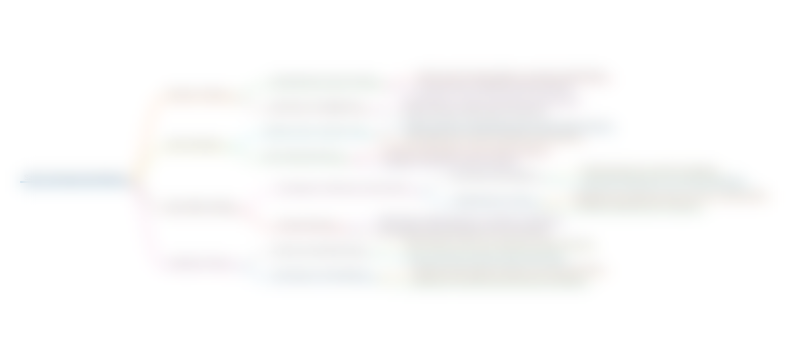
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
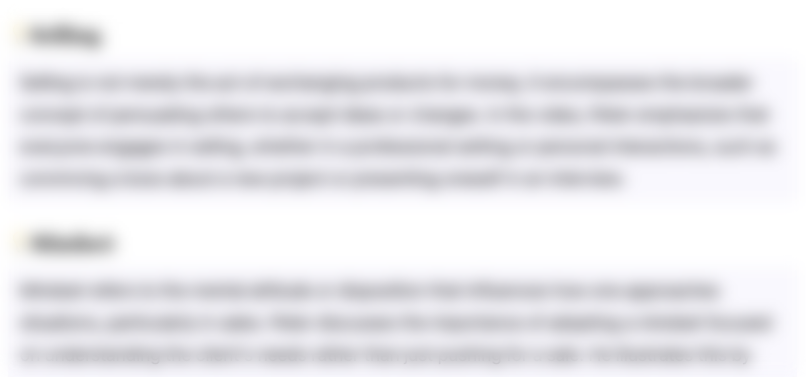
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
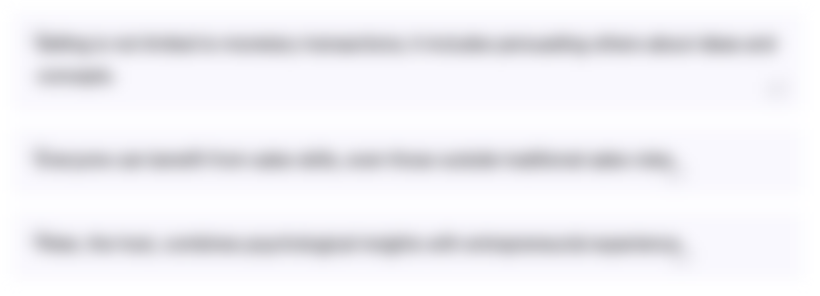
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
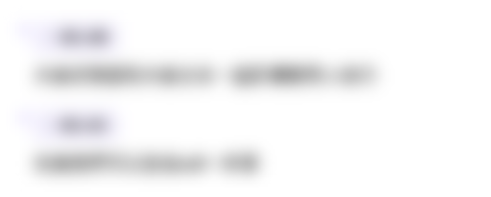
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
5.0 / 5 (0 votes)