59. OCR GCSE (J277) 2.1 Bubble sort
Summary
TLDRIn this educational video, Craig introduces the bubble sort algorithm, a simple yet inefficient method for sorting data. He demonstrates the process using a list of breakfast cereals, explaining how items are compared and swapped to achieve alphabetical order. The video highlights the algorithm's key features, its implementation, and its limitations, making it suitable for small data sets. Aimed at GCSE students, Craig emphasizes understanding the algorithm's steps and prerequisites without memorizing the code. The video also promotes a book, 'Essential Algorithms for A Level Computer Science,' which covers GCSE algorithms in detail, providing a comprehensive guide for students.
Takeaways
- 🔍 The video explains the bubble sort algorithm, which is used to sort an unordered list of items.
- 🔄 The algorithm works by comparing each item with the next one and swapping them if they are out of order.
- 🔝 It 'bubbles up' the largest or smallest item to the end of the list through successive passes.
- ⏱ Despite being the most inefficient sorting algorithm, bubble sort is simple to implement and popular for small data sets.
- 🌰 The video uses a data set of breakfast cereals to demonstrate the sorting process in alphabetical order.
- 🔑 The key to bubble sort is to ensure that after each pass, the last item is in its correct position.
- 🔁 The sorting process involves multiple passes through the list, with each pass reducing the number of comparisons needed.
- 📉 The algorithm is demonstrated step by step, showing how the list progresses towards being fully sorted.
- 📚 The video references a book, 'Essential Algorithms for A Level Computer Science', which covers algorithms including bubble sort.
- 💻 The book provides high-level introductions, structured English descriptions, diagrams, examples, pseudocode, and actual code in Python and Visual Basic.
Q & A
What is the bubble sort algorithm?
-The bubble sort algorithm is a simple sorting algorithm that repeatedly steps through the list to be sorted, compares each pair of adjacent items, and swaps them if they are in the wrong order. The pass through the list is repeated until no swaps are needed, which indicates that the list is sorted.
How does the bubble sort algorithm work?
-It works by making multiple passes through the list. During each pass, it compares adjacent elements and swaps them if they are in the wrong order. The largest or smallest element 'bubbles up' to its correct position at the end of each pass. This process continues until the entire list is sorted.
Why is bubble sort considered inefficient?
-Bubble sort is considered inefficient because it has a high computational complexity, especially for large datasets. It has an average and worst-case time complexity of O(n^2), where n is the number of items being sorted. This makes it impractical for large lists compared to more advanced algorithms like quicksort or mergesort.
What is the advantage of bubble sort despite its inefficiency?
-The advantage of bubble sort is its simplicity and ease of implementation. It is easy to understand and code, making it a good choice for educational purposes or for sorting very small datasets where its inefficiency is not a significant drawback.
How does the bubble sort algorithm ensure that a particular item is in its correct position?
-After each pass through the list, the algorithm can be sure that the last item it checked is in its correct position because it has 'bubbled up' to the end of the list. This item is then ignored in subsequent passes, reducing the number of comparisons needed in each pass.
What is the purpose of the 'swapped' flag in the bubble sort algorithm?
-The 'swapped' flag is used to track whether any swaps have been made during a pass. If no swaps are made during a pass, it means the list is already sorted, and the algorithm can terminate early, improving efficiency slightly.
Can you explain the process of a single pass in bubble sort using the example of sorting breakfast cereals?
-In the example, the first pass starts by comparing Cornflakes and Crunchy Nut Clusters, swapping them because they are out of order. Then, it compares Weetabix and Sugar Puffs, but they are in order, so no swap occurs. The next comparison is between Fruit 'n' Fibre and Weetabix, which are out of order, so they are swapped. Finally, Cornflakes and Weetabix are compared and swapped. After this pass, Weetabix is in its correct position at the top of the list.
How many passes are typically required to sort a list with bubble sort?
-The number of passes required to sort a list with bubble sort depends on the initial order of the list. In the worst case, where the list is sorted in reverse order, it requires n-1 passes, where n is the number of items in the list.
What is the significance of the zero-indexing in the bubble sort algorithm?
-Zero-indexing is significant in bubble sort because it affects the range of the loop used for comparisons. Since array indices start at 0, the algorithm adjusts the range of the loop to ensure that it compares all adjacent elements in the list without going out of bounds.
What is the role of pseudocode in teaching the bubble sort algorithm?
-Pseudocode is an informal high-level description of the operating principle of a program or other algorithm. It plays a crucial role in teaching the bubble sort algorithm by providing a structured yet easily understandable representation of the algorithm's logic, which helps in grasping the concept without getting bogged down by specific programming language syntax.
How does the book 'Essential Algorithms for A Level Computer Science' help in understanding algorithms like bubble sort?
-The book provides a comprehensive approach to understanding algorithms by introducing them from a high-level perspective, providing simple-structured English descriptions, diagrams, and examples. It also includes pseudocode and actual code in multiple programming languages, which helps readers to not only understand the algorithms but also to implement them.
Outlines
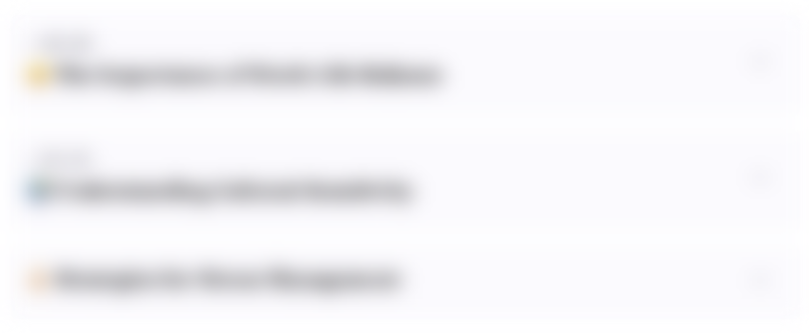
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
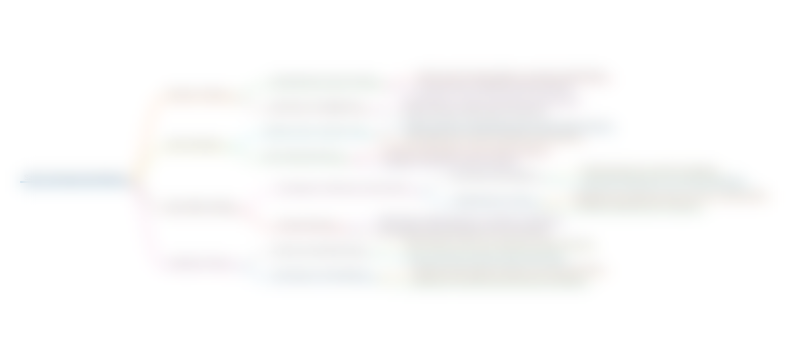
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
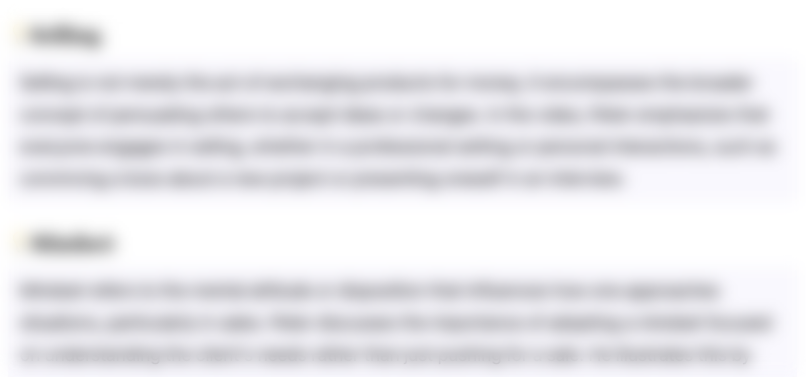
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
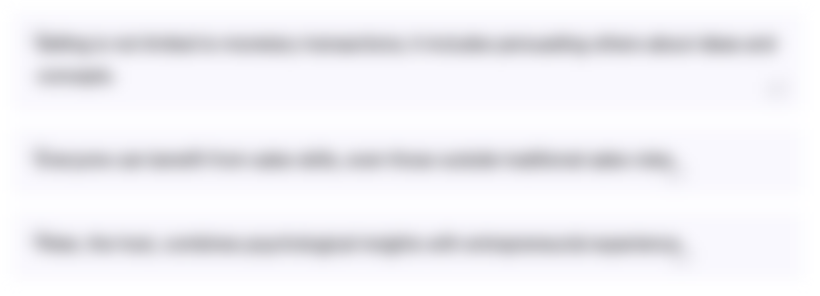
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
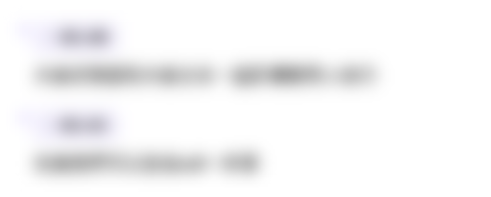
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тариф5.0 / 5 (0 votes)