Monotonic Stack Data Structure Explained
Summary
TLDRThis video introduces the monotonic stack, a powerful tool for problem-solving in coding interviews. It's a stack that maintains order, either increasing or decreasing, and is used to find the next largest height in a list. The script explains how a brute force approach would be inefficient, and instead, a monotonically decreasing stack is used to solve the problem in O(n) time. The video walks through an example with five people's heights, demonstrating how the stack works and why it's more efficient than checking every element. It concludes by explaining the code implementation and the stack's ability to store both position and magnitude of values, which is key to its efficiency.
Takeaways
- 📚 The monotonic stack is a data structure used for problem-solving in coding interviews, particularly useful for maintaining order in a stack with greedy logic.
- 🔄 It can be either monotonically increasing, where elements stay the same or increase from left to right, or monotonically decreasing, where elements stay the same or decrease.
- 👥 An example problem discussed is finding the height value of the next tallest person given an array of heights, with a brute force solution having a time complexity of O(n^2).
- 🔄 The monotonic stack approach iterates from right to left, maintaining a monotonically decreasing order to efficiently find the next greater element.
- 🔍 If the stack is empty when a new element is encountered, it means there is no greater element on the right, so negative one is assigned as the next greater height.
- 🧩 The stack invariant is maintained by popping elements that are less than or equal to the current element being considered, ensuring the stack remains monotonically decreasing.
- 💡 The top element of the stack represents the next greatest height greater than the current element, providing the solution for the current position.
- 💻 The code implementation involves looping from right to left, using a stack to keep track of the next greater elements, and pushing the current element onto the stack after processing.
- 🚀 The monotonic stack achieves a time complexity of O(n) by storing both the position and magnitude of values, which is a significant improvement over the brute force method.
- 🎯 The final implementation results in an efficient algorithm for the next greater element problem, demonstrating the power of the monotonic stack in solving such interview questions.
Q & A
What is a monotonic stack?
-A monotonic stack is a data structure that maintains a specific order of elements, either monotonically increasing or decreasing, and uses greedy logic to keep elements orderly as new elements are added.
How does the monotonic stack differ from a regular stack?
-A monotonic stack differs from a regular stack in that it enforces a specific order (increasing or decreasing) among its elements, whereas a regular stack follows a last-in, first-out (LIFO) principle without any order requirement.
What is the purpose of using a monotonic stack in coding interviews?
-In coding interviews, a monotonic stack is used to solve problems efficiently by leveraging its ordered structure to quickly find next greater elements, which can lead to solutions with better time complexity compared to brute force methods.
What is the time complexity of the brute force solution for finding the next greater element in an array?
-The time complexity of the brute force solution for finding the next greater element in an array is O(n^2), where n is the number of elements in the array.
How does the monotonic stack help in reducing the time complexity for finding the next greater element?
-The monotonic stack helps in reducing the time complexity by maintaining a decreasing order of elements, allowing for the next greater element to be found in constant time, thus achieving a linear time complexity of O(n).
What is the strategy for maintaining a monotonically decreasing stack in the given example?
-In the given example, the strategy for maintaining a monotonically decreasing stack is to pop elements from the stack if they are less than or equal to the current element being considered, ensuring that the stack always contains elements greater than the current element.
How does the monotonic stack handle the case when there is no greater element to the right?
-When there is no greater element to the right, the stack is empty, and the value assigned is negative one, indicating that there is no greater height on the right side of the current element.
What is the significance of the position and magnitude in the context of the monotonic stack?
-In the context of the monotonic stack, the position is significant as it determines the order of elements, and the magnitude is significant as it is used to determine the next greater element by popping elements from the stack.
Can you provide a step-by-step explanation of how the monotonic stack processes the example array of heights?
-The monotonic stack processes the example array of heights by iterating from right to left, maintaining a decreasing order. For each height, it pops elements from the stack that are less than or equal to the current height. If the stack is empty, it assigns negative one. Otherwise, the top element of the stack is the next greater height. Each height is then pushed onto the stack to maintain the decreasing order.
What is the final output of the monotonic stack after processing the example array of heights?
-The final output of the monotonic stack after processing the example array of heights is an array where each element represents the next greater height to the right or negative one if there is no greater height.
Outlines
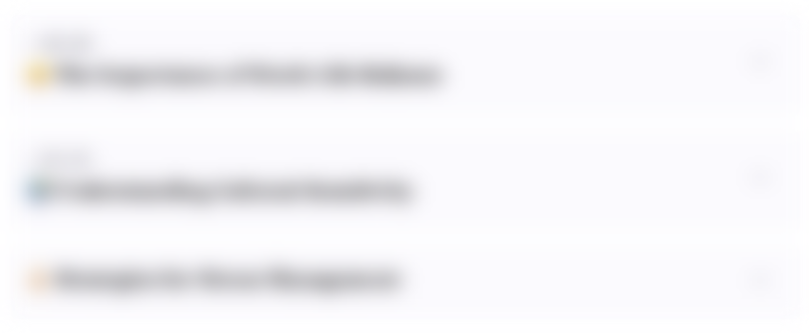
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
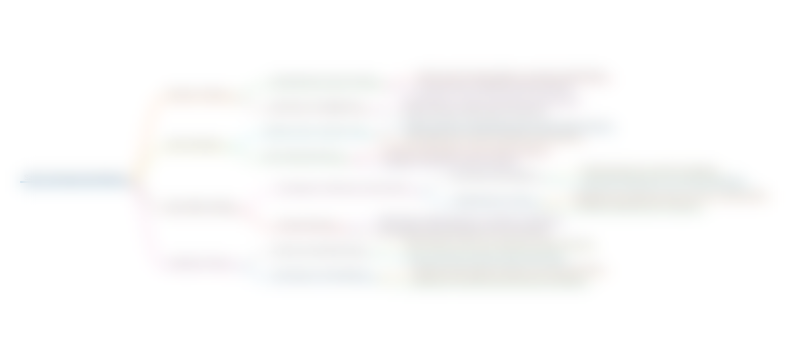
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
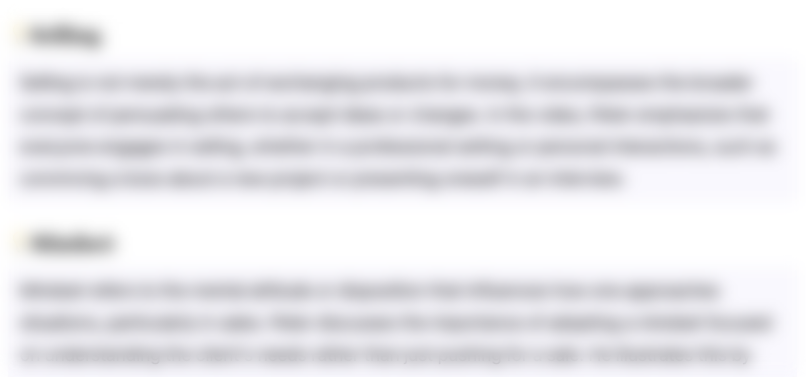
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
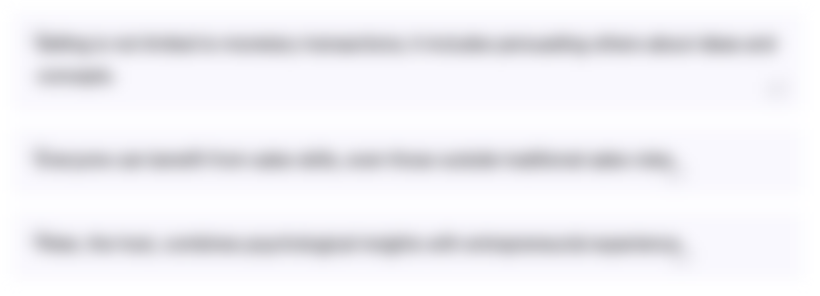
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
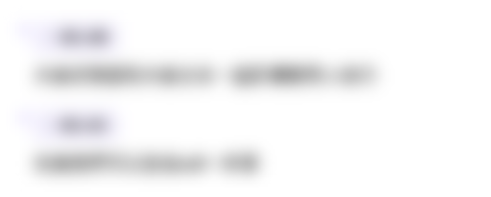
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тариф5.0 / 5 (0 votes)