L3. Longest Substring Without Repeating Characters | 2 Pointers and Sliding Window Playlist
Summary
TLDRIn this educational video, the presenter dives into the problem of finding the longest substring without repeating characters using the sliding window and two-pointer technique. They start with a brute force approach, explaining its limitations and time complexity, then transition to a more efficient solution involving a hash array to track character indices. The presenter guides viewers through the optimized algorithm step by step, highlighting its linear time complexity and constant space complexity due to the fixed-size hash array, wrapping up with a pseudo code for clarity.
Takeaways
- 📘 The video is a continuation of an A to Z DSA course focusing on a problem involving the concept of sliding window and two-pointer techniques.
- 🔍 The problem presented is to find the longest substring without repeating characters in a given string that can contain any character from the 256 possible characters.
- 📌 The definition of a substring is explained as any consecutive portion of the string, and the task is to find the longest such substring without repeating characters.
- 🤔 A brute force approach is initially discussed, which involves generating all possible substrings and checking for repeating characters, but this method is inefficient.
- 🚫 The script emphasizes that if a repeating character is found, there's no need to continue checking further substrings as they will also contain repetitions.
- 🔑 The use of a hash array of size 256 is introduced to keep track of characters that have been seen and their last seen index to optimize the search for the longest substring.
- 🔄 The sliding window technique is introduced as an optimized approach, which involves maintaining a window of non-repeating characters and adjusting it based on the presence of repeating characters.
- 📈 The time complexity of the brute force approach is analyzed to be O(n^2), which is not efficient, and the need for optimization to around O(n) is discussed.
- 📝 Pseudo code is provided to demonstrate the sliding window algorithm, which includes initializing a hash array, maintaining left and right pointers, and updating the maximum length found.
- 🕊️ The optimized sliding window approach has a time complexity of O(n) and a space complexity of O(1), making it a more efficient solution to the problem.
- 👍 The video concludes with an invitation for viewers to like and subscribe if they found the content helpful and understood the material.
Q & A
What is the main problem discussed in the video script?
-The main problem discussed in the video script is finding the longest substring without repeating characters in a given string.
What is a substring as defined in the script?
-A substring is any portion of the string that is consecutive in nature. For example, 'ca' is a substring of 'cadebz', but 'c' and 'C' are not consecutive and thus not a substring.
What is the brute force approach to solving the problem mentioned in the script?
-The brute force approach involves generating all possible substrings starting from each character and checking for repeating characters. If a repeat is found, the process stops for that particular substring.
How does the script suggest optimizing the brute force solution?
-The script suggests using a two-pointer and sliding window approach to optimize the solution, which is more efficient than the brute force method.
What is the role of the hash array in the optimized solution?
-The hash array is used to remember the last seen index of each character. It helps in efficiently checking if a character has already appeared in the current window of the substring being considered.
What is the time complexity of the brute force approach mentioned in the script?
-The time complexity of the brute force approach is O(n^2), where n is the length of the string, due to the nested loops required to generate all substrings.
What is the space complexity of the brute force approach?
-The space complexity of the brute force approach is O(1) since no additional space is used that scales with the input size, aside from the temporary variables.
How does the two-pointer and sliding window approach improve the time complexity?
-The two-pointer and sliding window approach improves the time complexity to O(n), as it only requires a single pass through the string with constant time operations for each character.
What is the space complexity of the optimized solution using the hash array?
-The space complexity of the optimized solution is O(256), which corresponds to the size of the hash array needed to store the indices of the last seen characters for all possible ASCII values.
How does the script describe the process of updating the left and right pointers in the sliding window approach?
-The script describes updating the right pointer to expand the window and checking the hash array for repeating characters. If a repeat is found, the left pointer is moved to the right of the last occurrence of the repeating character to maintain the no-repeat condition, and the window is then expanded again.
Outlines
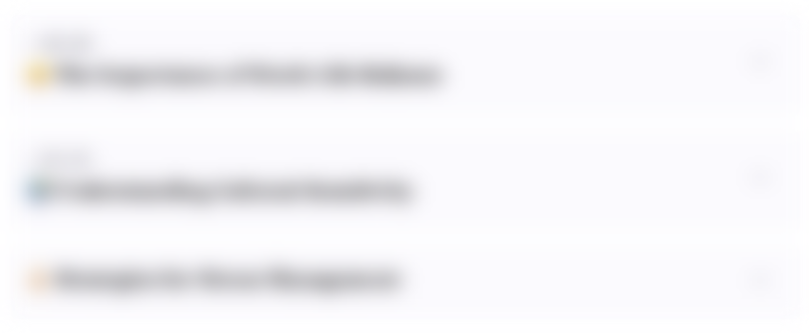
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
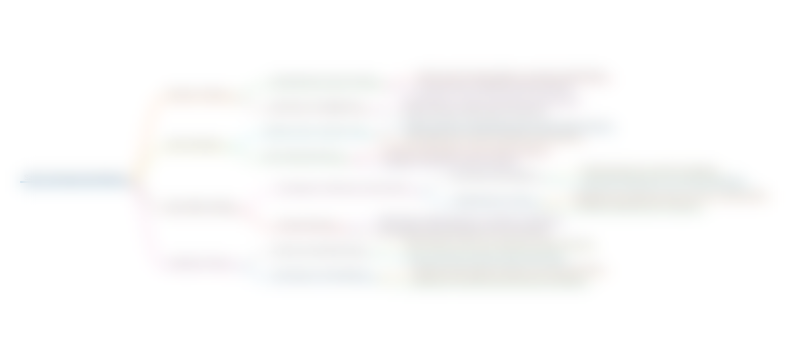
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
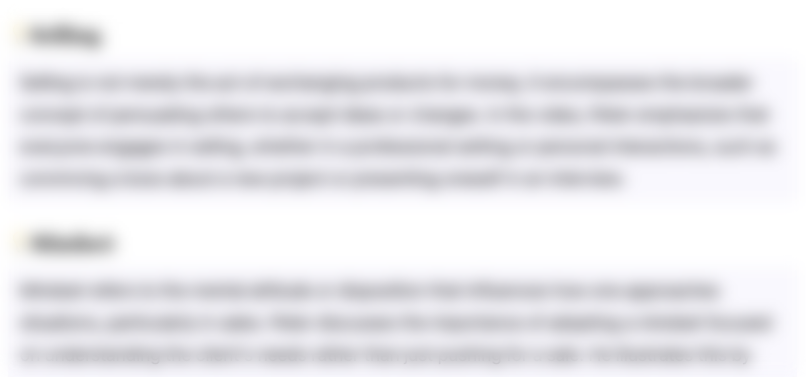
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
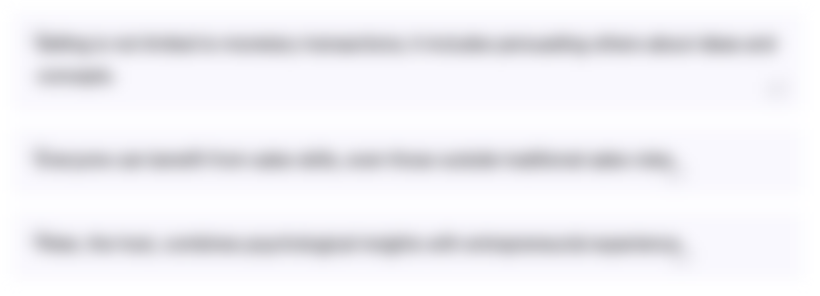
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
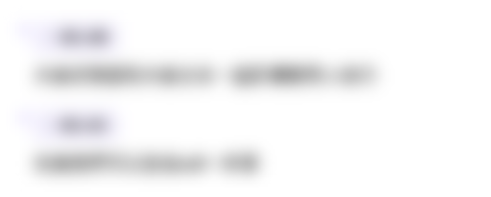
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示

Longest Substring Without Repeating Characters - Leetcode 3 - Sliding Window (Python)
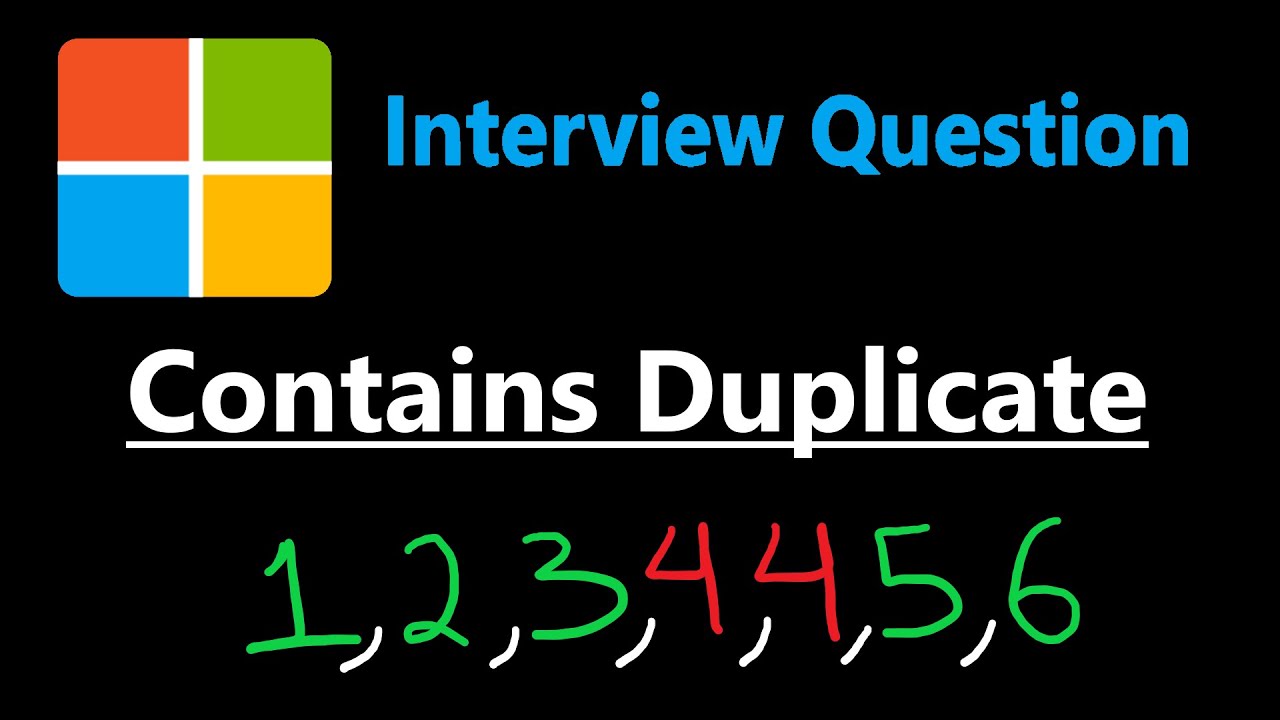
Contains Duplicate - Leetcode 217 - Python

Two Sum - Leetcode 1 - HashMap - Python

Majority Element I | Brute-Better-Optimal | Moore's Voting Algorithm | Intuition 🔥|Brute to Optimal
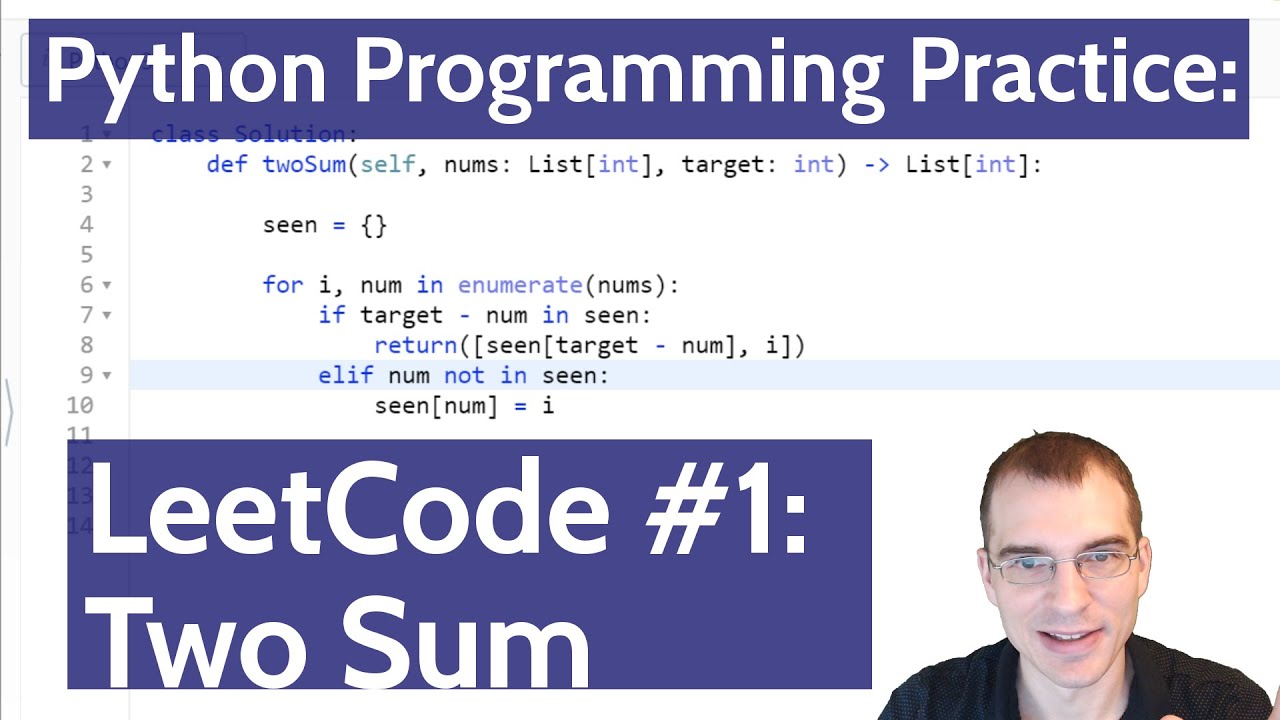
Python Programming Practice: LeetCode #1 -- Two Sum
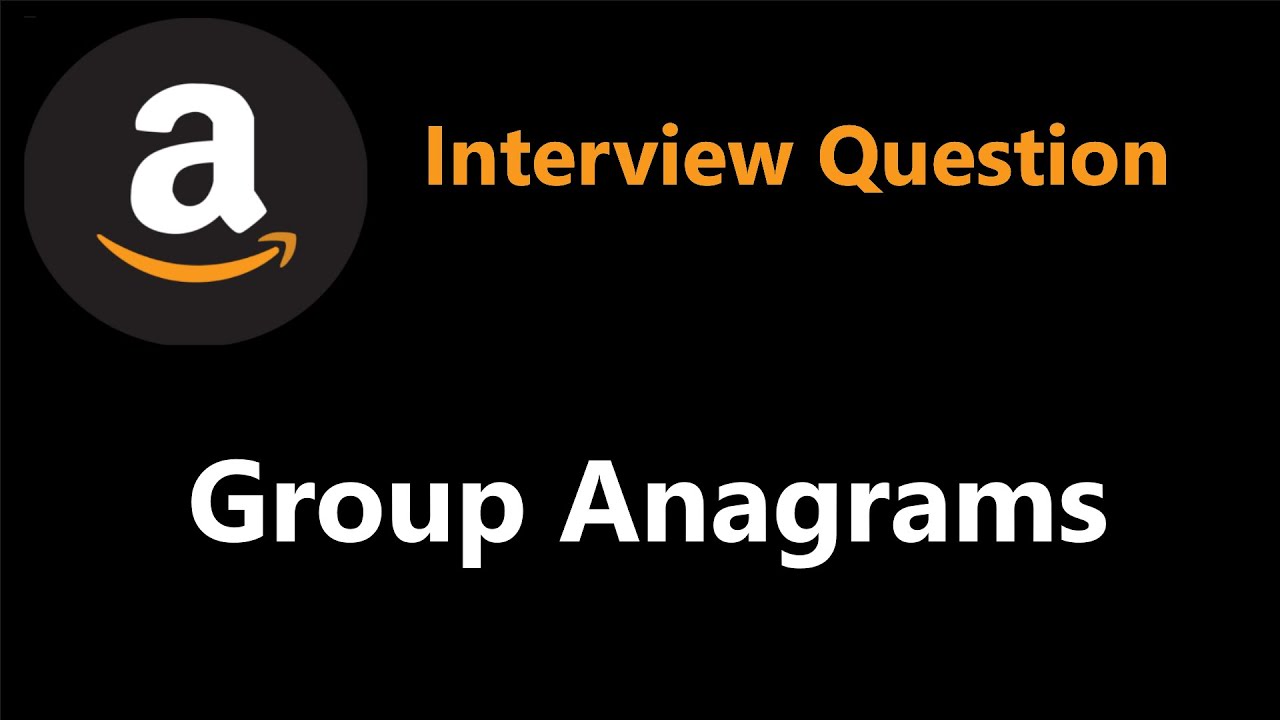
Group Anagrams - Categorize Strings by Count - Leetcode 49
5.0 / 5 (0 votes)