#15 String Interpolation | Angular Components & Directives | A Complete Angular Course
Summary
TLDRThis lecture delves into the concept of data binding in Angular, specifically focusing on one-way data binding and string interpolation. The instructor guides through creating a 'product list' component, demonstrating how to dynamically display product details using properties within the component class. The video showcases transitioning from static HTML to dynamic content by binding properties like product name, price, and color. It further illustrates the use of string interpolation for complex expressions, method calls, and ternary operations to render conditional messages, offering a comprehensive understanding of Angular's data binding capabilities.
Takeaways
- 📚 The lecture provided an overview of data binding, explaining the concept of one-way data binding and its implementation methods.
- 🔍 Two methods for achieving one-way data binding were discussed: string interpolation and property binding.
- 💻 A new Angular component named 'product list' was created using the Angular CLI with the 'ng generate component' command.
- 📝 Initially, the 'product list' component displayed hard-coded values for product details, demonstrating static HTML content.
- 🔑 Dynamic content generation was introduced by creating properties within the 'product list' component class, such as 'name', 'price', and 'color'.
- 🦄 String interpolation was utilized to display the values of these properties in the component's view template using double curly braces.
- 🔢 The script showed how to perform calculations and use TypeScript expressions within the double curly braces for dynamic content.
- 🛍 An example of creating a 'product' object with properties and using it in the view template with string interpolation was given.
- 💡 The use of methods, such as 'get discounted price', within string interpolation to perform calculations and return values was demonstrated.
- 📈 The ability to use TypeScript's built-in methods like 'toFixed' to format numbers within string interpolation was highlighted.
- 📉 The use of the ternary operator within string interpolation to conditionally display messages based on the 'in stock' status of a product was explained.
Q & A
What is the main topic of the lecture?
-The main topic of the lecture is string interpolation in Angular for data binding from the component class to the view template.
What are the two ways to achieve one-way data binding in Angular mentioned in the script?
-The two ways to achieve one-way data binding in Angular are using string interpolation and property binding.
How is a new component created in Angular using the CLI?
-A new component is created in Angular using the Angular CLI with the 'ng generate component' command followed by the component name.
What is the purpose of deleting the spec.ts file in the new component?
-The spec.ts file is often deleted as it contains test code, and the focus of the lecture is on component functionality rather than testing.
What is the selector used for the product list component in the script?
-The selector used for the product list component is 'app-product-list'.
How are static values in the product list component's HTML template replaced with dynamic values?
-Static values are replaced with dynamic values by using properties from the component class within the HTML template through string interpolation.
What is the syntax used for string interpolation in Angular templates?
-The syntax used for string interpolation in Angular templates is '{{ expression }}', where 'expression' can be a property name or a TypeScript expression.
How can an object's properties be accessed within the view template using string interpolation?
-An object's properties can be accessed using the syntax 'object.property' within the double curly braces of string interpolation.
What is the purpose of the 'toFixed' method in the context of the script?
-The 'toFixed' method is used to format numbers to a specified number of decimal places, in this case, to limit the display of the discounted price to two decimal points.
How can a ternary operator be used within string interpolation syntax?
-A ternary operator can be used within string interpolation to conditionally display different messages based on the evaluation of an expression, such as showing 'only X items left' or 'not in stock'.
What does the lecture demonstrate about using methods within string interpolation?
-The lecture demonstrates that you can call a component method within string interpolation to perform calculations or operations, such as getting a discounted price.
Outlines
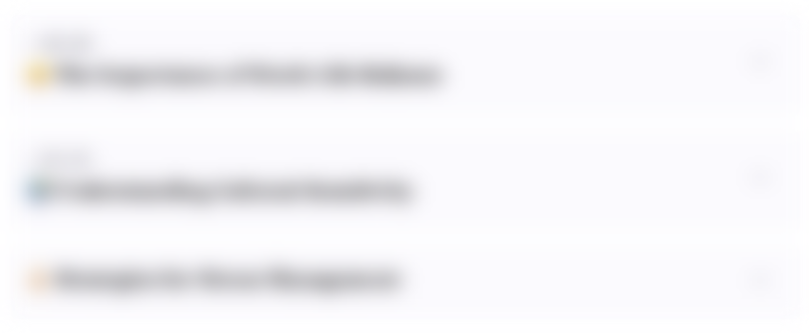
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
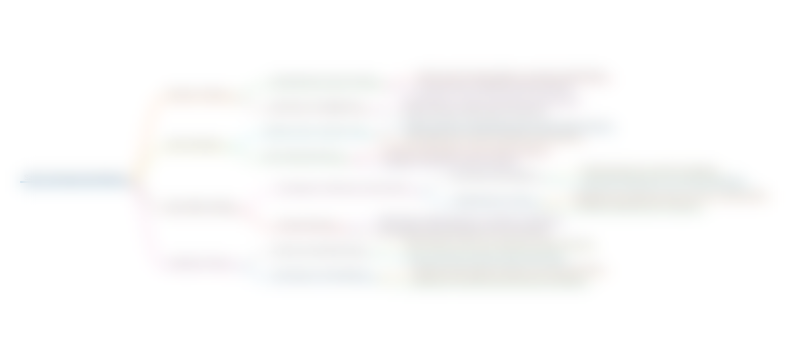
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
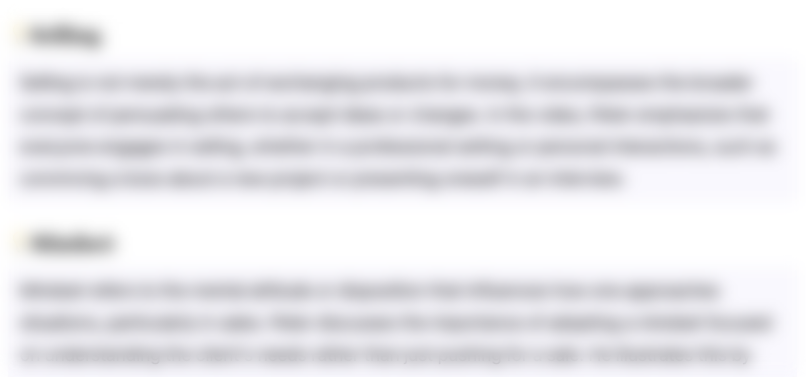
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
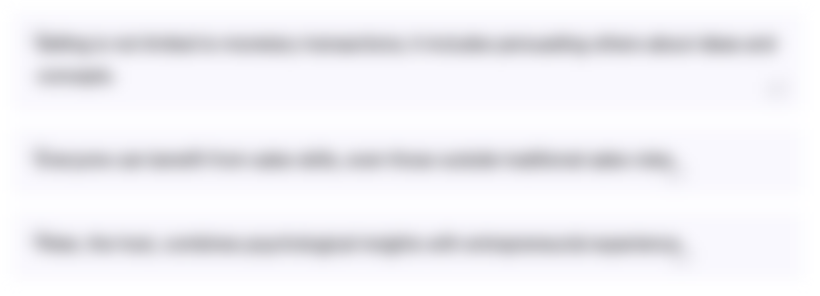
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
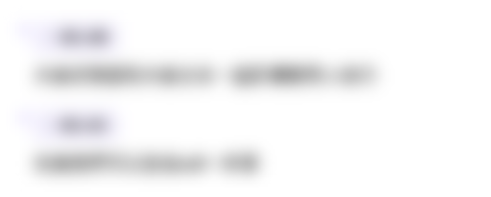
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
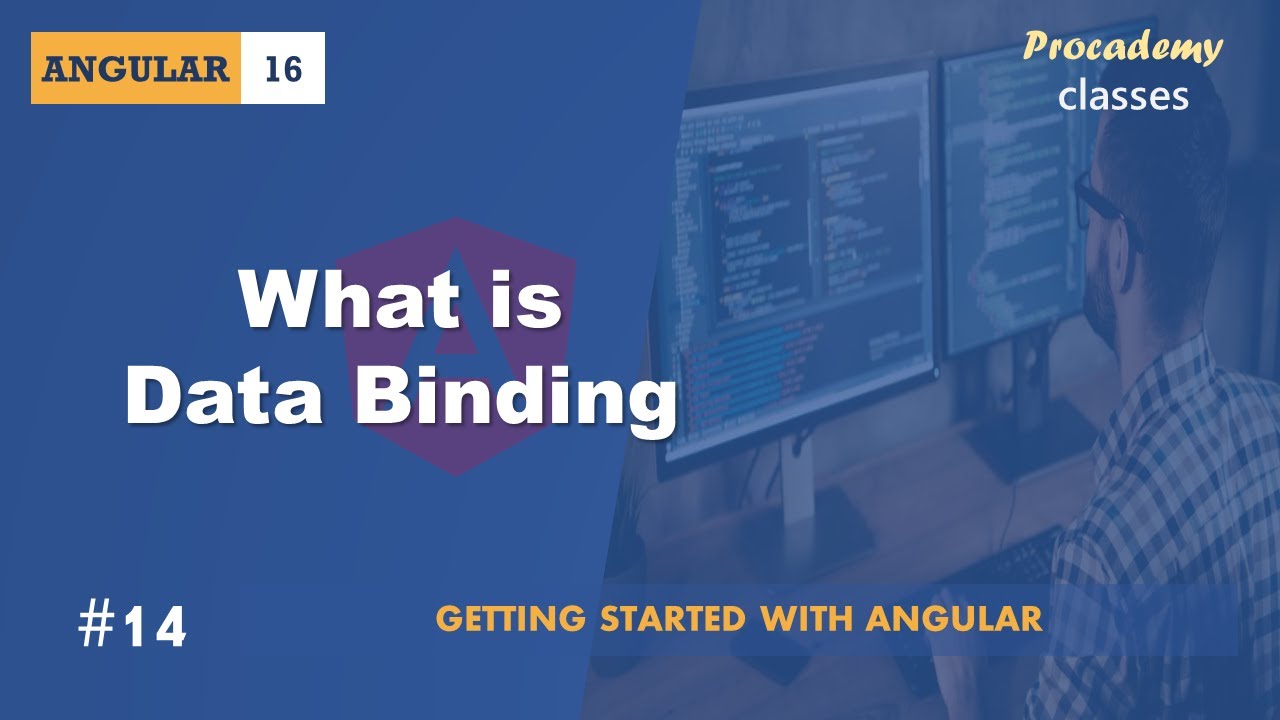
#14 What is Data Binding | Angular Components & Directives | A Complete Angular Course
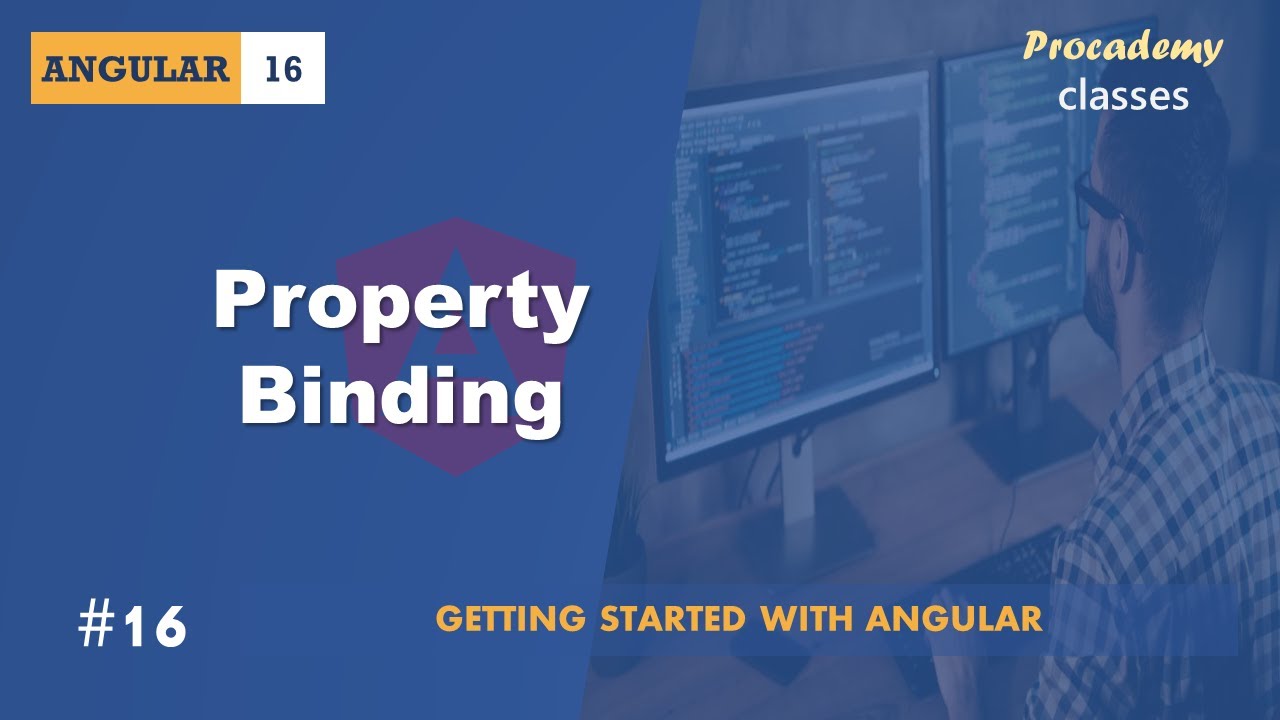
#16 Property Binding | Angular Components & Directives | A Complete Angular Course

#17 Event Binding | Angular Components & Directives | A Complete Angular Course

#18 Two way Data Binding | Angular Components & Directives | A Complete Angular Course
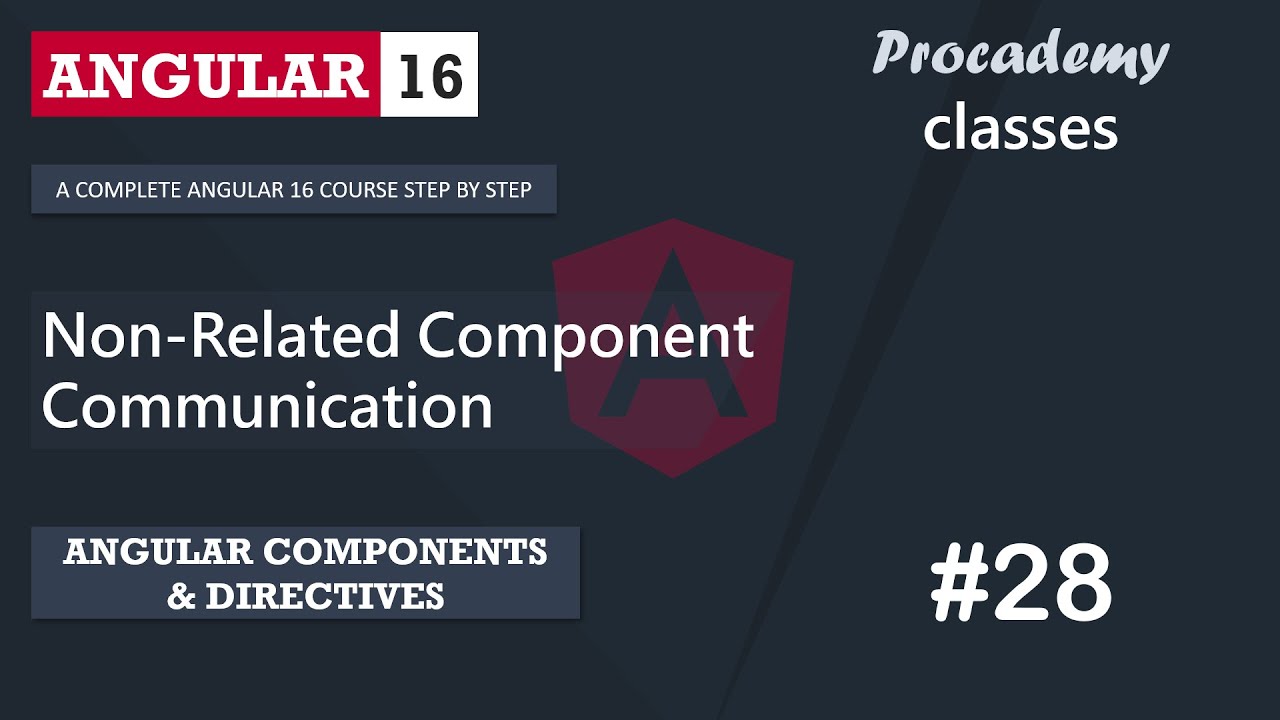
#28 Non-Related Component Communication | Angular Component & Directives | A Complete Angular Course

#03 Editing the First Angular Project | Getting Started with Angular | A Complete Angular Course
5.0 / 5 (0 votes)