JavaScript Arrays
Summary
TLDRThis JavaScript tutorial introduces arrays, a fundamental data structure used to store lists of items such as products or selected colors. It demonstrates how to declare, initialize, and manipulate arrays in JavaScript, showcasing their dynamic nature in terms of length and element types. The tutorial also reveals that arrays are objects with inherited properties, including a 'length' property. The instructor encourages viewers to enroll in a comprehensive JavaScript course for further learning and practice, especially for those aiming for a career in web or mobile development.
Takeaways
- 📝 In JavaScript, arrays are used to store lists of items like products in a shopping cart or selected colors.
- 🔑 An array is declared and initialized with meaningful names, such as 'selectedColors', and can start as an empty array using array literals.
- 📚 You can add items to an array, like 'red' and 'blue', and view them in the console to see the array with its elements.
- 🔢 Arrays in JavaScript have dynamic lengths, allowing for expansion as new elements are added, such as 'green'.
- 🎯 Each element in an array has an index, starting from zero for the first element, which is used to access specific elements.
- 🌐 JavaScript is a dynamic language, meaning the types of variables, including the contents and lengths of arrays, can change at runtime.
- 🔄 The types of objects within an array can also be dynamic, allowing for a mix of different data types, such as strings and numbers.
- 📊 Arrays in JavaScript are objects and inherit properties from other objects, which can be accessed using dot notation.
- 📏 The 'length' property of an array returns the number of elements within it, demonstrating the dynamic nature of array size.
- 🛠️ The script suggests that arrays will be further explored in a comprehensive section of the course, covering various operations that can be performed on them.
- 📚 The video is part of a JavaScript course aimed at teaching essential features for web and mobile application developers, including exercises that are relevant to technical programming interviews.
Q & A
What is the purpose of using an array in JavaScript?
-An array in JavaScript is used to store a list of items, such as a list of products in a shopping cart or a list of colors selected by a user.
How do you declare and initialize an array in JavaScript?
-You declare an array by using a variable name followed by square brackets to denote an array literal. For example, `let selectedColors = [];` initializes an empty array.
How can you add elements to an array in JavaScript?
-You can add elements to an array by assigning values to the array using indices. For instance, `selectedColors[0] = 'red'; selectedColors[1] = 'blue';` adds 'red' and 'blue' to the array.
How do you display the contents of an array in the console?
-You can display the contents of an array in the console by using `console.log(selectedColors);`, where `selectedColors` is the name of the array.
What is the significance of array indices in JavaScript?
-Array indices in JavaScript determine the position of an element within the array. The index of the first element is 0, the second is 1, and so on.
How can you access a specific element in an array?
-To access a specific element in an array, you use its index in square brackets. For example, `console.log(selectedColors[0]);` will display the first element of the array.
Is the length of an array in JavaScript fixed or dynamic?
-The length of an array in JavaScript is dynamic and can change at runtime. You can add or remove elements to expand or shrink the array.
Can an array in JavaScript contain elements of different types?
-Yes, in JavaScript, an array can contain elements of different types. It's possible to have strings, numbers, and other data types in the same array.
What does it mean to say that an array is an object in JavaScript?
-In JavaScript, an array is technically an object and has properties that can be accessed using dot notation. This means it inherits properties from the object prototype.
What is the 'length' property of an array used for?
-The 'length' property of an array returns the number of elements in the array, providing a way to determine its size.
What is the tutorial offering for those interested in learning JavaScript?
-The tutorial is part of a JavaScript course designed for beginners and experienced developers alike, covering essential JavaScript features with exercises and interview questions to help master the language.
Outlines
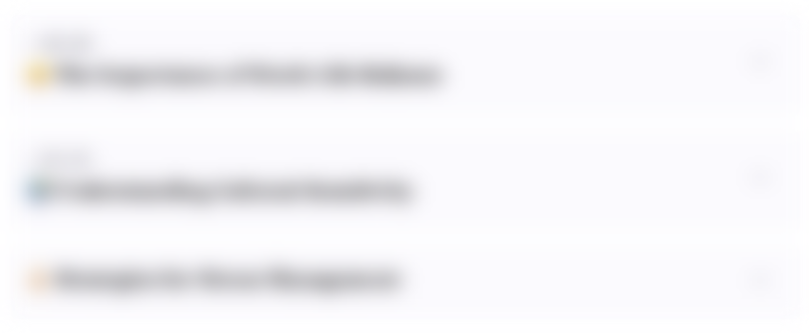
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
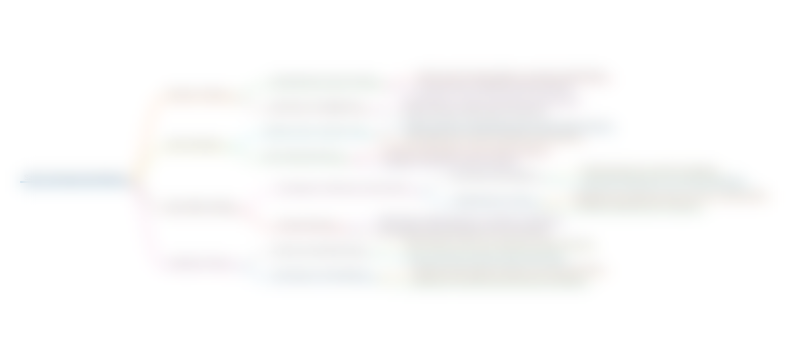
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
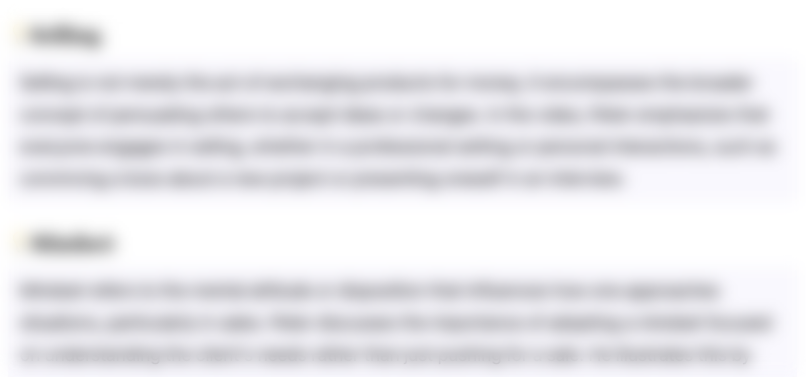
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
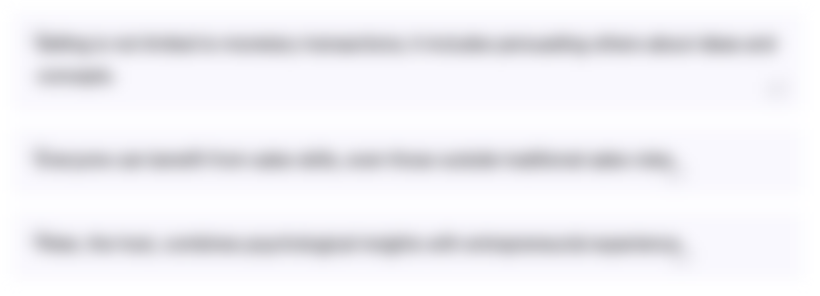
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
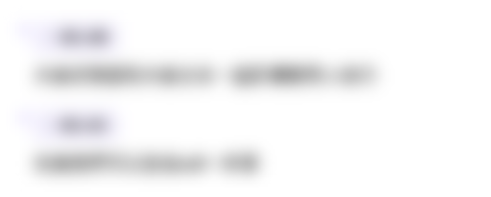
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
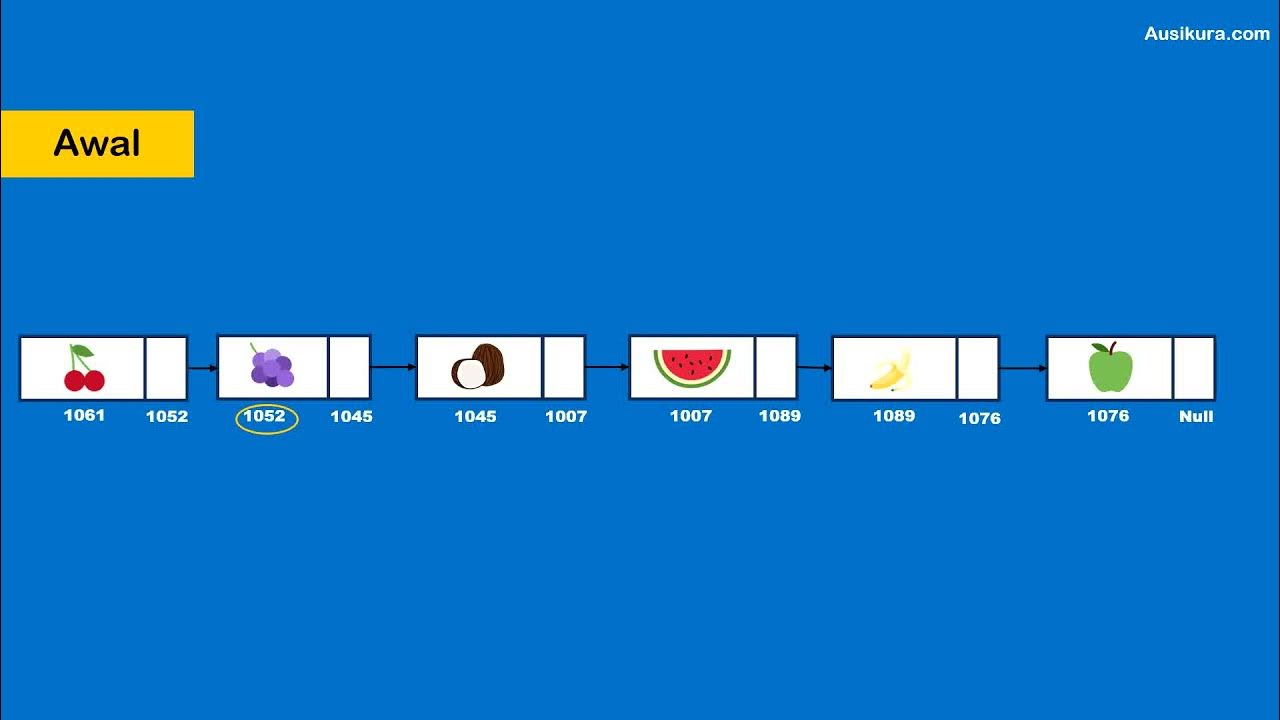
Pengenalan Linked List - struktur Data (Animasi)

Data Structures & Algorithms #1 - What Are Data Structures?
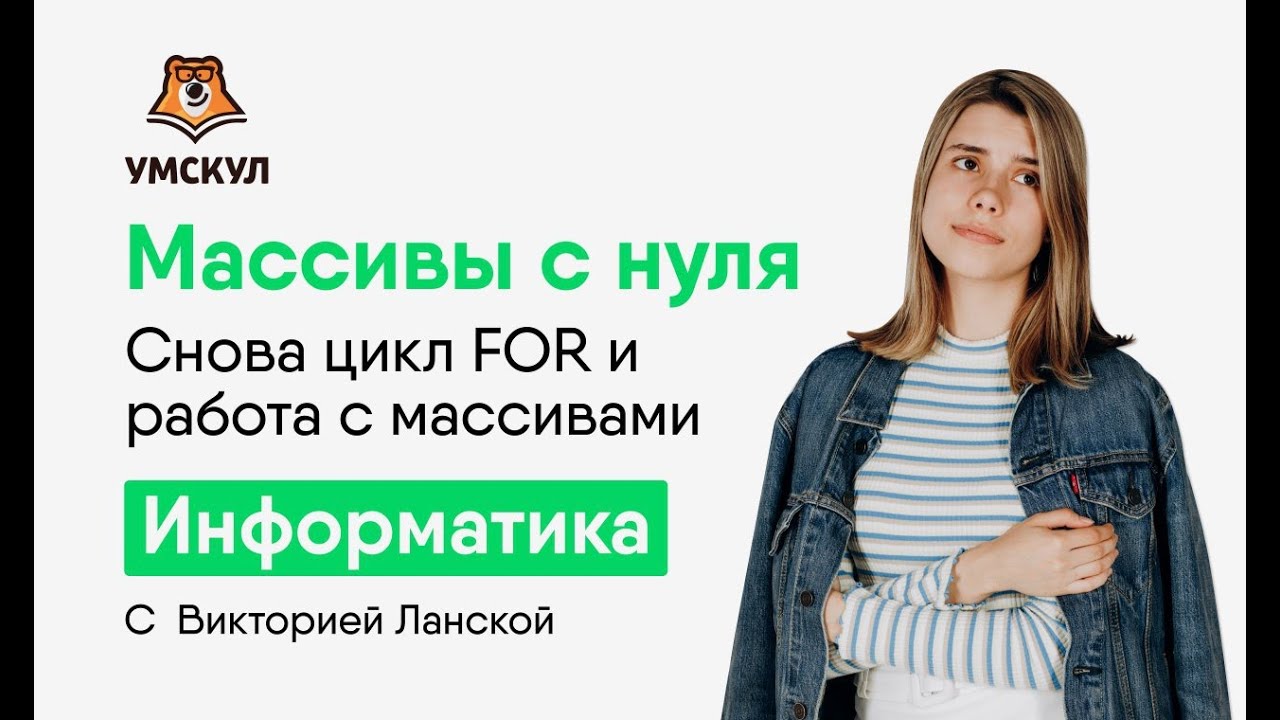
Python для ЕГЭ. Массивы с нуля. Снова цикл for и работа с массивами.

GCSE Python Programming 5 - Lists/Arrays

Memahami Fungsi Array || Algoritma dan Struktur Data

Linked List Data Structure | Illustrated Data Structures
5.0 / 5 (0 votes)