Studi Kasus C++ 11 - Bubble Sort - Sorting pada C++
Summary
TLDRThis video explains the concept and implementation of the Bubble Sort algorithm in programming, focusing on optimizing the sorting process. The speaker demonstrates how to perform Bubble Sort using a for-loop and swap adjacent elements to arrange them in order. The video then introduces a significant optimization, where the sorting process halts early if no swaps are needed in an iteration, reducing unnecessary steps. The speaker encourages viewers to experiment with the code and modify it for their needs, providing a useful learning resource for those wanting to understand and improve sorting algorithms.
Takeaways
- 😀 The speaker demonstrates the basic working of Bubble Sort, where adjacent elements are compared and swapped if necessary to sort a list.
- 😀 A key part of the Bubble Sort process is to repeatedly pass through the list, ensuring that larger elements 'bubble up' to the end.
- 😀 The process uses a variable 'Y' and a for loop to iterate through the elements of the list, with the goal of sorting them in ascending order.
- 😀 After sorting, the program outputs the result in a flat (horizontal) format, showcasing the sorted list.
- 😀 The speaker explains how the sorting works through four main steps and how the results are displayed after completion.
- 😀 To optimize the sorting process, a status variable (acak) is introduced to track whether any swaps have been made during an iteration.
- 😀 The optimization prevents unnecessary iterations once the list is sorted, improving the efficiency of the Bubble Sort algorithm.
- 😀 The variable 'acak' helps determine if any elements were out of order during the iteration. If no swaps were made, it signals that the sorting process is complete.
- 😀 If the list is already sorted, the program breaks out of the loop early, reducing the number of sorting steps to just the necessary ones.
- 😀 This optimization allows the algorithm to complete sorting faster by avoiding further iterations when the data is already in order.
- 😀 The speaker encourages viewers to experiment with the sorting values and to explore ways of improving the algorithm further.
Q & A
What is Bubble Sort?
-Bubble Sort is a simple sorting algorithm where each pair of adjacent elements is compared and swapped if they are in the wrong order. This process is repeated until the entire list is sorted.
What are the typical stages in Bubble Sort?
-In the typical Bubble Sort, the algorithm works through several stages. Each stage involves comparing and potentially swapping adjacent elements, and this process is repeated until the list is fully sorted.
How does the speaker improve the Bubble Sort algorithm?
-The speaker optimizes Bubble Sort by introducing a flag (`acak`) that tracks whether any swaps were made during a cycle. If no swaps are made, it indicates that the list is already sorted, and the algorithm breaks early, avoiding unnecessary iterations.
What is the role of the `acak` variable in the optimized Bubble Sort?
-The `acak` variable tracks whether any swaps have been made during a pass through the list. If no swaps are made, it means the list is sorted, and the algorithm breaks early, improving efficiency.
What happens when no swaps are made during a pass through the list?
-When no swaps are made, it indicates that the list is already sorted. The algorithm then stops further iterations and outputs the sorted list.
What does the speaker mean by 'stopping the algorithm early'?
-Stopping the algorithm early refers to breaking out of the sorting loop as soon as the list is determined to be sorted, thereby saving unnecessary iterations.
Why is it important to optimize the Bubble Sort algorithm?
-Optimizing Bubble Sort is important because it reduces the number of passes through the list. In the worst-case scenario, the traditional Bubble Sort can take many iterations, but the optimization stops it early if the list is already sorted.
How does the algorithm detect that the list is sorted?
-The algorithm detects that the list is sorted by checking the `acak` variable after each pass. If no swaps were made during the pass (meaning `acak` is zero), the algorithm knows the list is sorted and breaks out of the loop.
What happens if the `acak` variable is greater than zero?
-If the `acak` variable is greater than zero, it indicates that a swap was made during the pass, so the algorithm continues to the next cycle to further sort the list.
Can this optimized Bubble Sort be applied to any type of data structure?
-This optimized Bubble Sort is typically applied to linear data structures like arrays or lists where elements can be compared and swapped easily. It may not be as effective for non-linear structures like trees or graphs.
Outlines
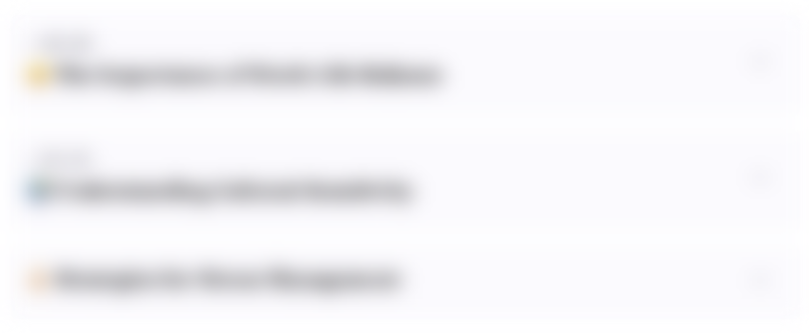
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
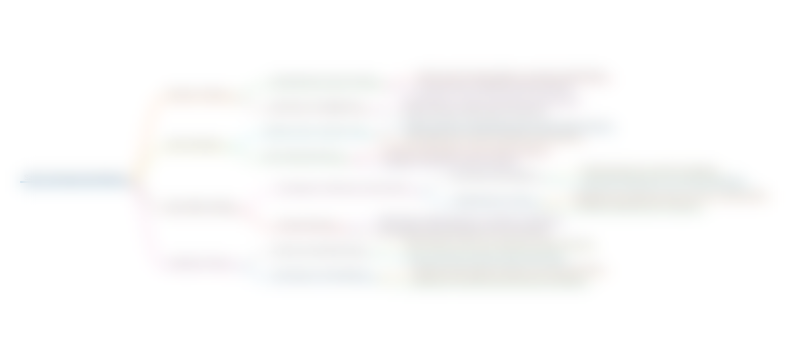
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
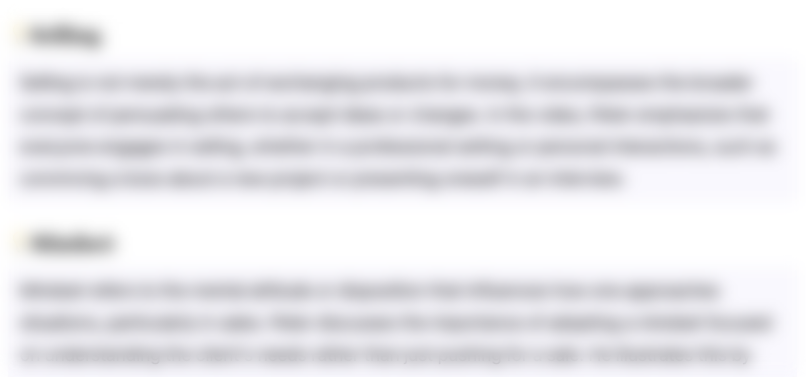
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
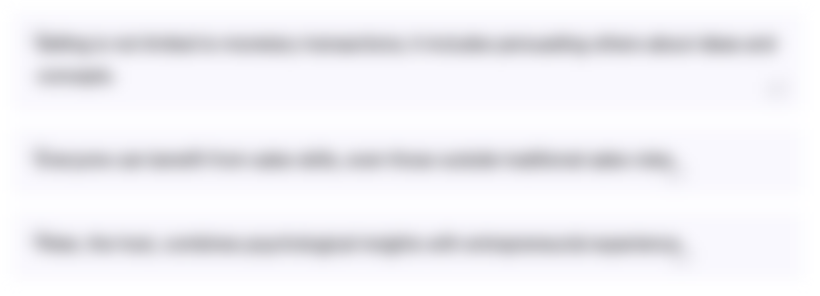
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
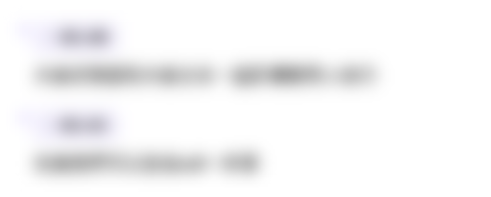
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
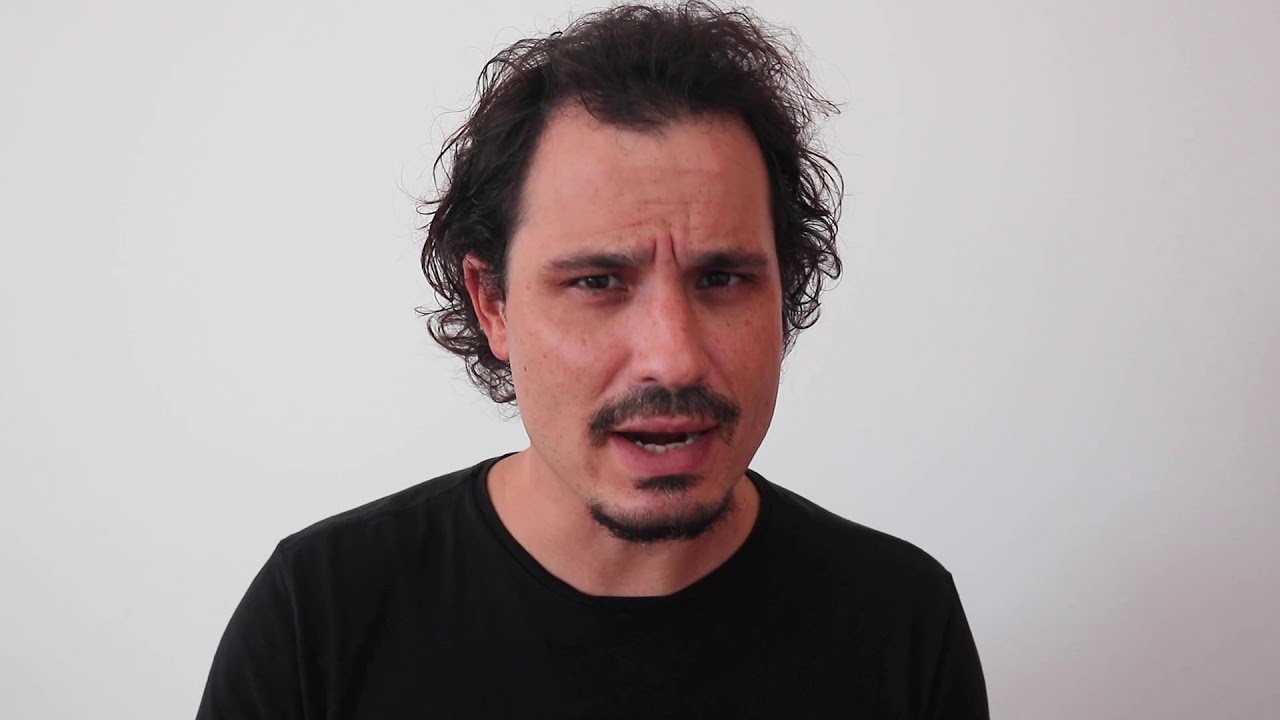
EDB1 IMD UFRN (2020.6): Ordenação por Bolha
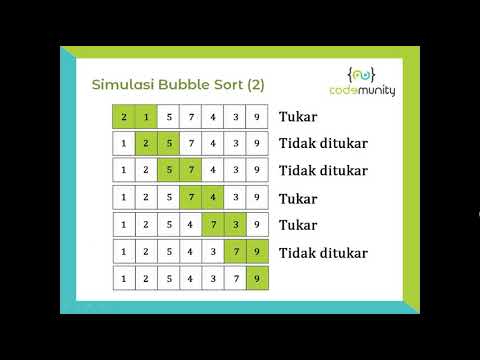
Penjelasan Bubble Sort (Bahasa Indonesia)
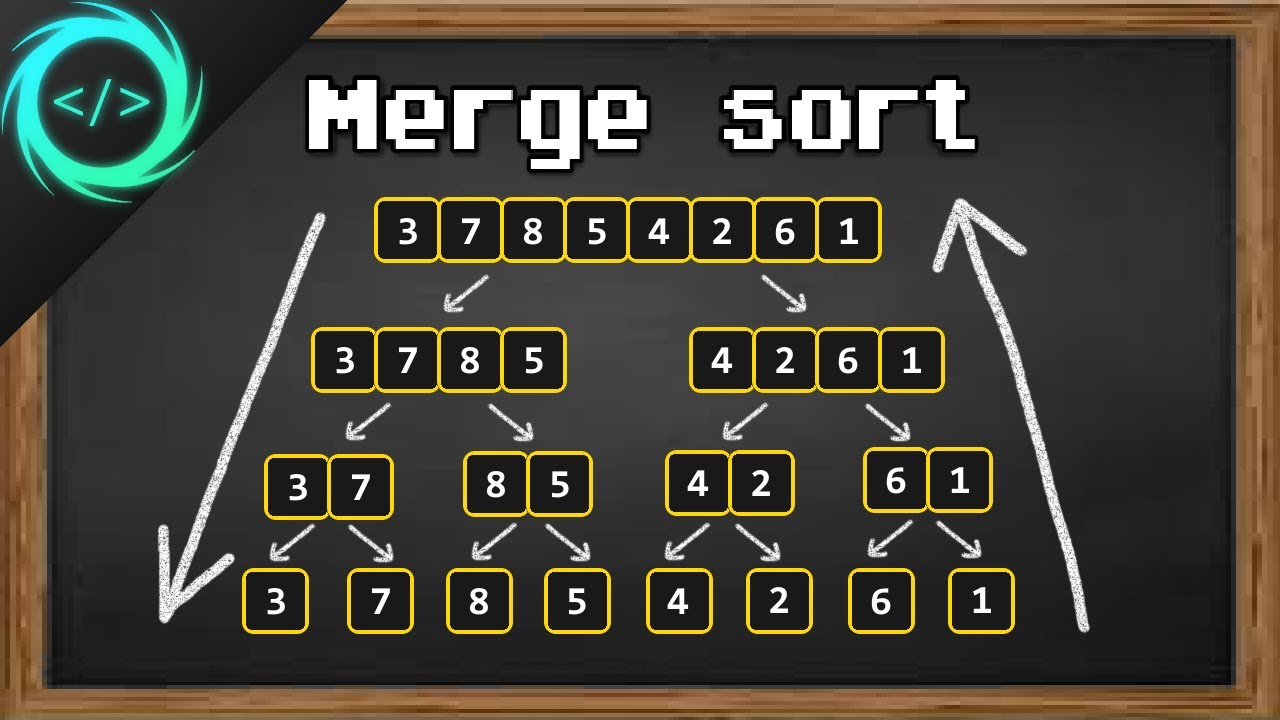
Learn Merge Sort in 13 minutes 🔪

Sorting - Part 1 | Selection Sort, Bubble Sort, Insertion Sort | Strivers A2Z DSA Course
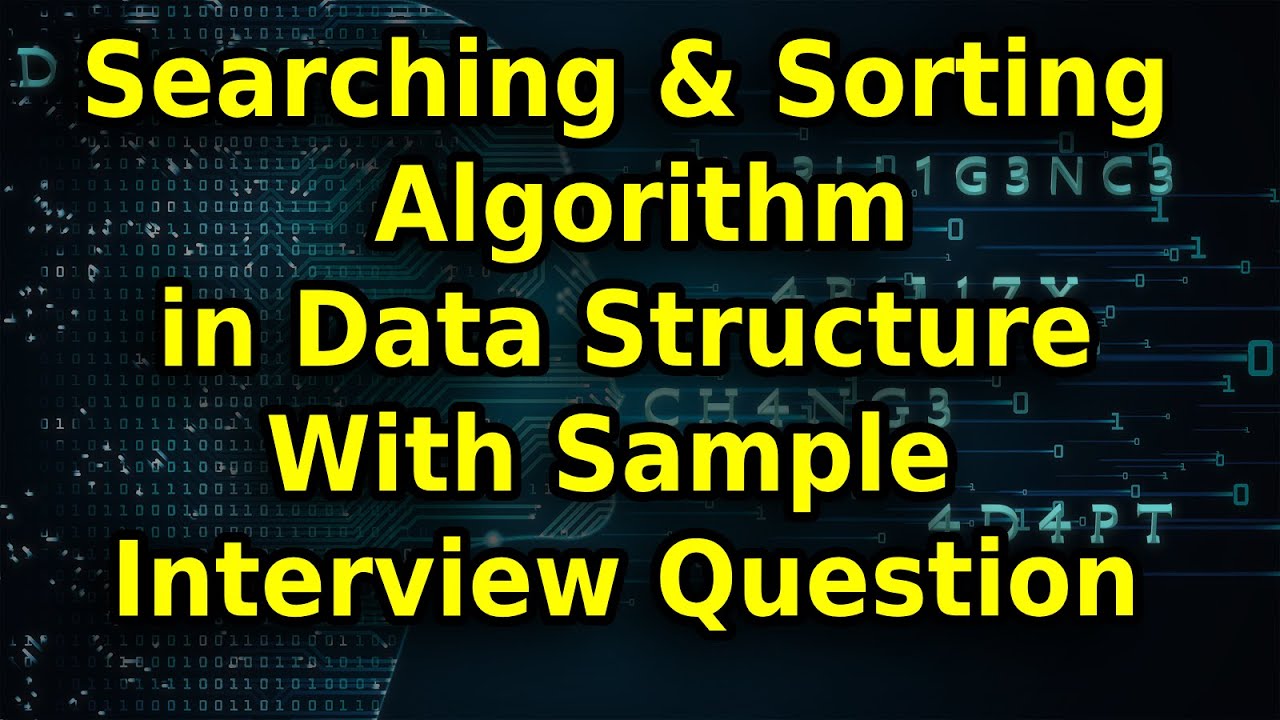
Learn Searching and Sorting Algorithm in Data Structure With Sample Interview Question
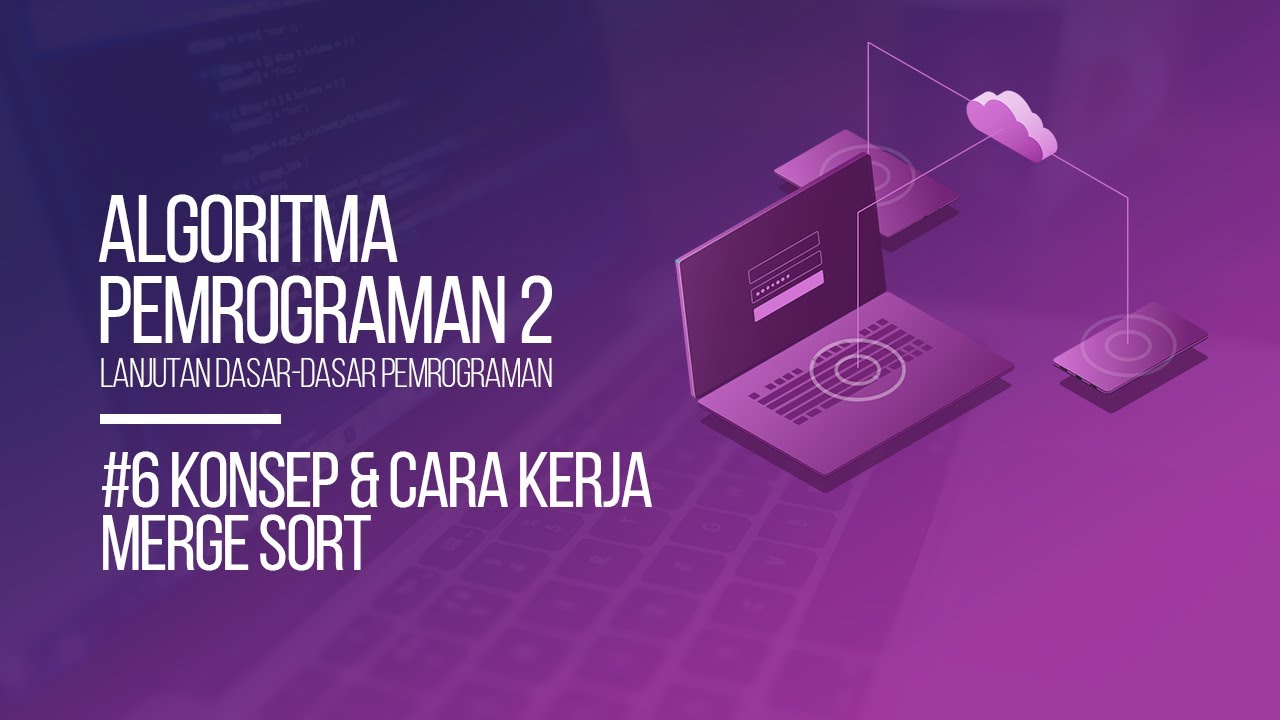
#6 KONSEP LOGIKA DAN CARA KERJA MERGE SORT | ALGORITMA PEMROGRAMAN 2
5.0 / 5 (0 votes)