std::move and the Move Assignment Operator in C++
Summary
TLDRIn this C++ tutorial video, Deshawn continues the series on move semantics, focusing on two crucial aspects: `std::move` and the move assignment operator. He explains `std::move` as a function that converts an object into an r-value, enabling move operations. Deshawn then demonstrates how to implement the move assignment operator for efficient resource transfer between objects. The video also highlights the difference between move constructors and assignment operators, emphasizing the importance of these concepts in modern C++ programming. Viewers are encouraged to explore the topic further through the series and take advantage of a special Skillshare offer for learning new skills.
Takeaways
- 📚 The video is part of a C++ series focusing on move semantics, continuing the journey from previous episodes.
- 🔗 The host encourages viewers to watch prior videos on move semantics and L values for better understanding.
- 🎯 The main topics for this video are `std::move`, the move assignment operator, and their applications in C++ programming.
- 🛠️ The `std::move` function is introduced as a utility to efficiently move resources from one object to another without copying.
- 🔄 `std::move` is used to cast an object to an r-value reference, enabling the use of move constructors or move assignment operators.
- 📝 The move assignment operator is explained as a special assignment operator used to move resources into an existing object.
- 💡 The video demonstrates how to implement the move assignment operator in a custom class, emphasizing the importance of handling self-assignment.
- ⚠️ A key point is made about the difference between using `std::move` for an existing variable versus a temporary, and its implications on resource management.
- 🔑 The concept of the 'rule of fifths' or 'rule of thirds' is mentioned, indicating a set of best practices including move constructors and move assignment operators.
- 📈 The video includes a practical example of implementing and testing a move assignment operator in a custom string class.
- 🎁 The host promotes Skillshare as an online learning platform offering a wide range of creative classes, with a special offer for the viewers.
- 👋 The video concludes with a summary of the move assignment operator and `std::move`, and an invitation for viewers to engage with the content and ask questions.
Q & A
What is the main topic of Deshawn's C++ series video?
-The main topic of the video is move semantics in C++, specifically focusing on std::move, the move assignment operator, and how to implement them in custom classes.
Why is understanding move semantics important for C++ programmers?
-Understanding move semantics is important because it allows for efficient transfer of resources between objects without unnecessary copying, which can improve performance and resource management in C++ programs.
What is the purpose of std::move in C++?
-The purpose of std::move is to efficiently transfer the contents of an object to another, effectively 'moving' the resources and leaving the original object in an unspecified but valid state.
What is the difference between a move constructor and a move assignment operator?
-A move constructor is used to initialize a new object with the resources of a temporary object, while a move assignment operator is used to transfer the resources from one existing object to another, modifying the existing object in the process.
Why is it necessary to check if the move assignment is happening to the same object in the move assignment operator?
-It is necessary to check because moving an object into itself would lead to self-assignment, which can cause issues like memory leaks if not handled properly by first nullifying the current object's resources.
What does the rule of five in C++ include, and why is it important for classes that manage resources?
-The rule of five includes the destructor, copy constructor, copy assignment operator, move constructor, and move assignment operator. It is important for classes that manage resources to ensure proper handling of object lifetimes and resource management.
How does the move assignment operator differ from the copy assignment operator in C++?
-The move assignment operator transfers ownership of resources from one object to another, potentially leaving the source object in a valid but unspecified state, while the copy assignment operator creates a copy of the resource, which is generally more expensive in terms of performance.
What is the role of 'noexcept' in the move assignment operator?
-The 'noexcept' specifier in the move assignment operator indicates that the function is not expected to throw exceptions. This can help with optimization and exception safety in C++ programs.
Why is Skillshare mentioned in the video, and what does it offer to its users?
-Skillshare is mentioned as a sponsor of the video. It offers an online learning community with thousands of classes on various creative topics, allowing users to learn new skills at their own pace with bite-sized video lessons.
What is the significance of the 'rule of thirds' and 'rule of fifths' in C++ programming?
-The 'rule of thirds' refers to the three special member functions (constructor, copy assignment operator, and destructor) that need to be properly defined for classes managing resources. The 'rule of fifths' extends this to include move constructors and move assignment operators, which are important for efficient resource management in modern C++.
Outlines
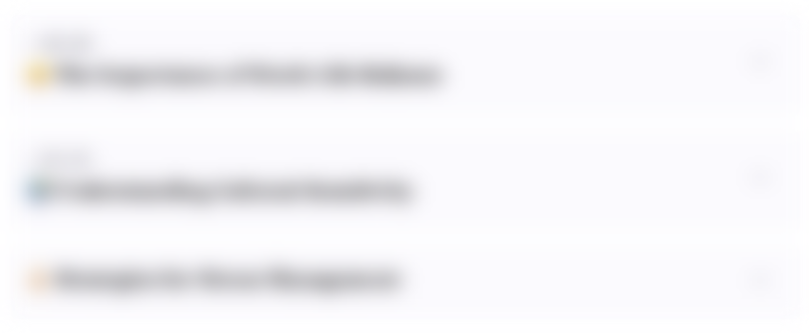
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
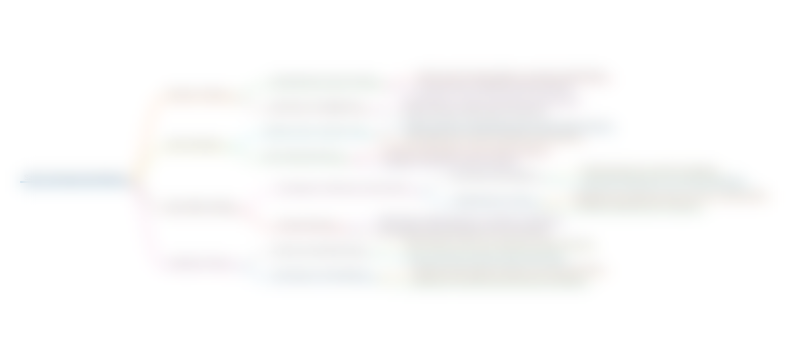
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
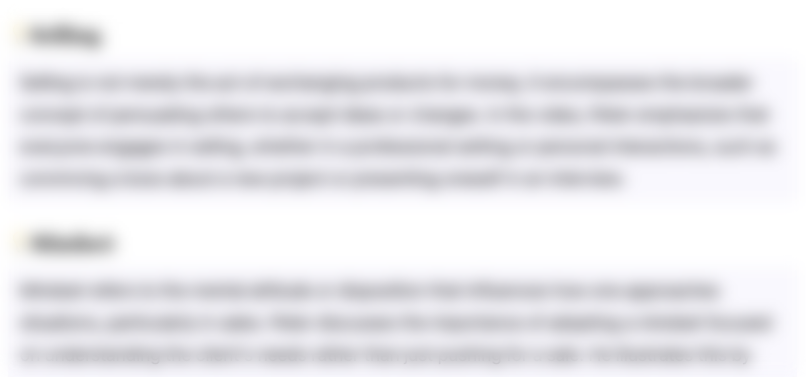
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
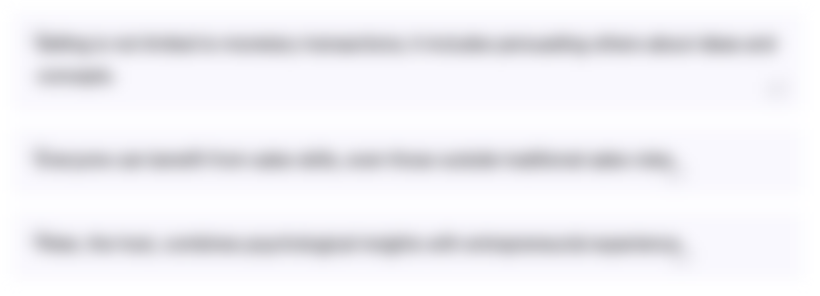
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
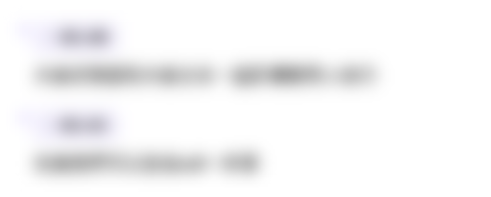
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示

Cara Menampilkan Karakter dengan Bahasa Assembly Menggunakan EMU8086
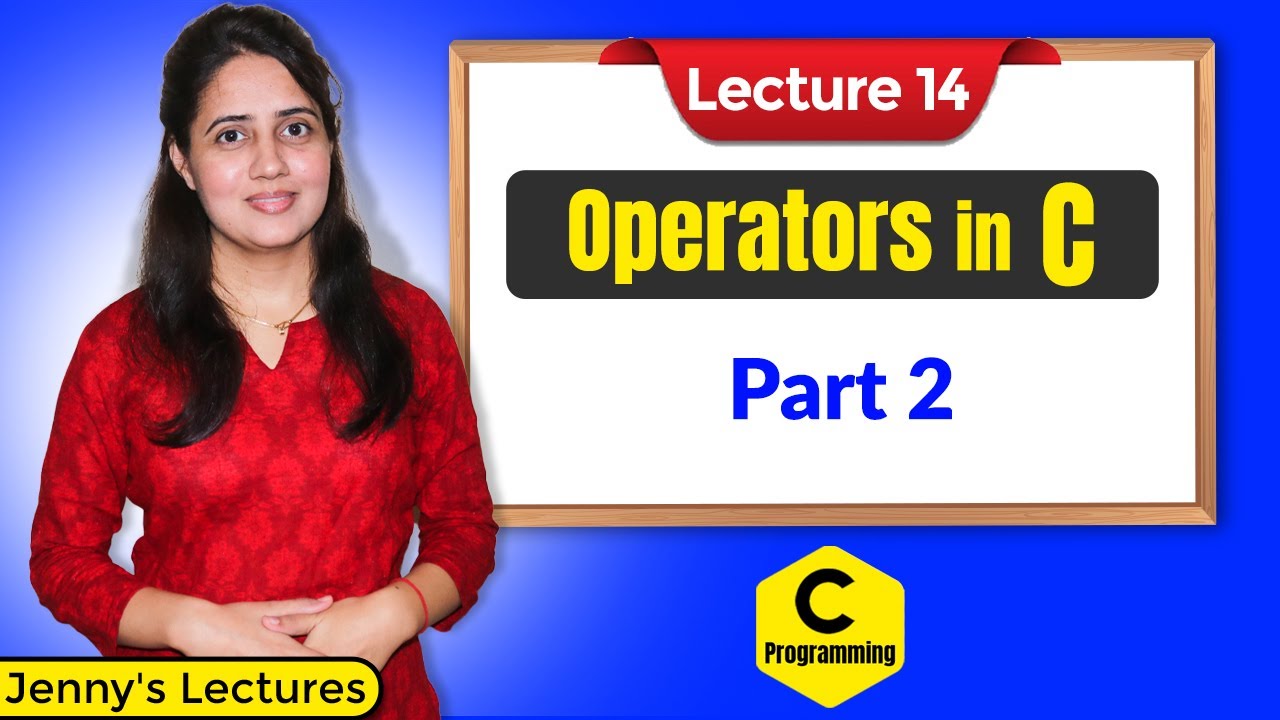
C_14 Operators in C - Part 2 | Arithmetic & Assignment Operators | C Programming Tutorials
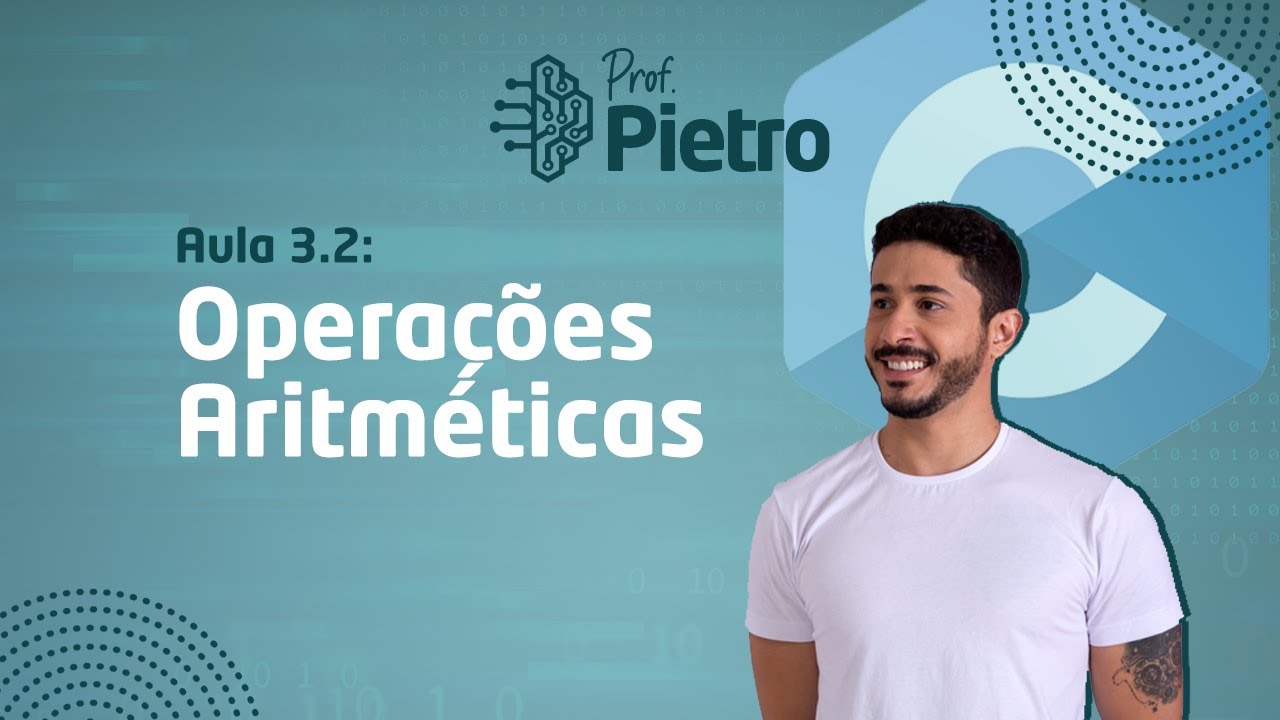
Linguagem C - Aula 3.2 - Aprenda a realizar cálculos em C (2022)
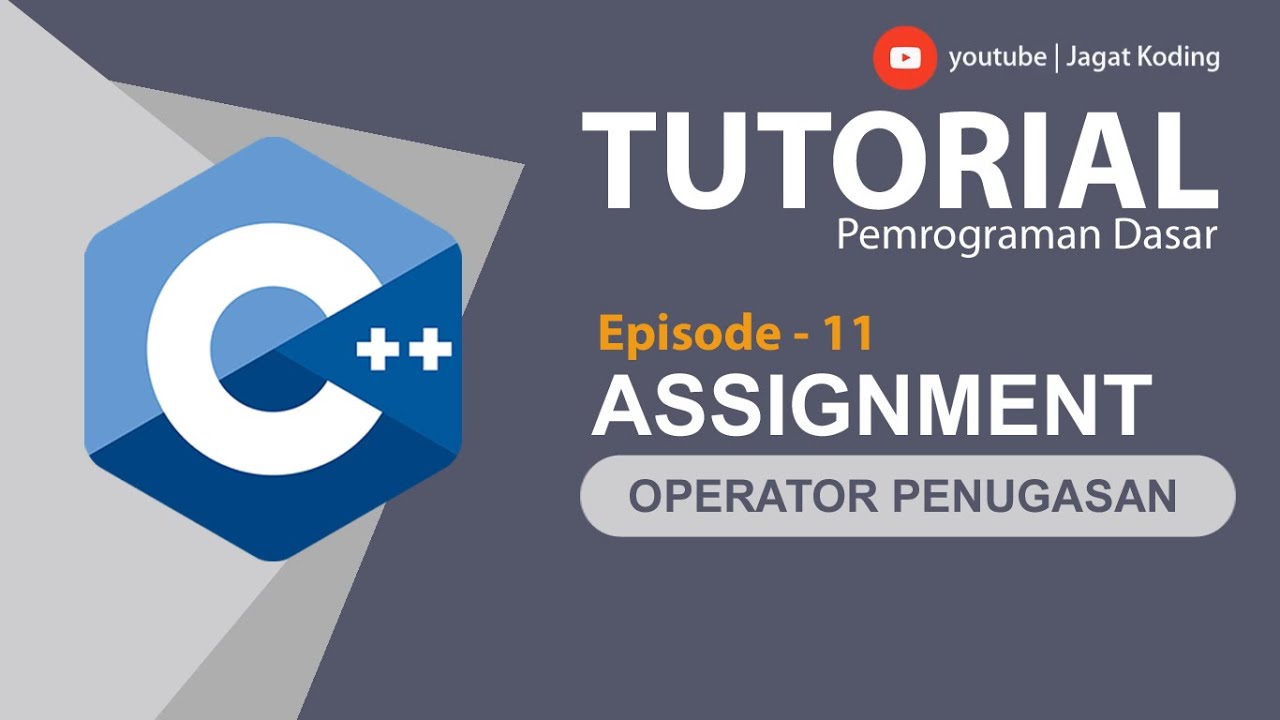
C++ 11 | Operator Assignment | Tutorial Pemrograman C++
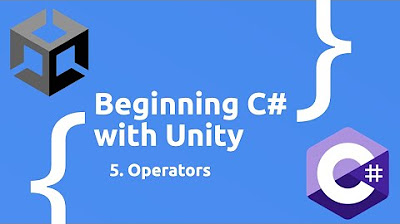
Beginning C# with Unity (2023 Edition) - Operators
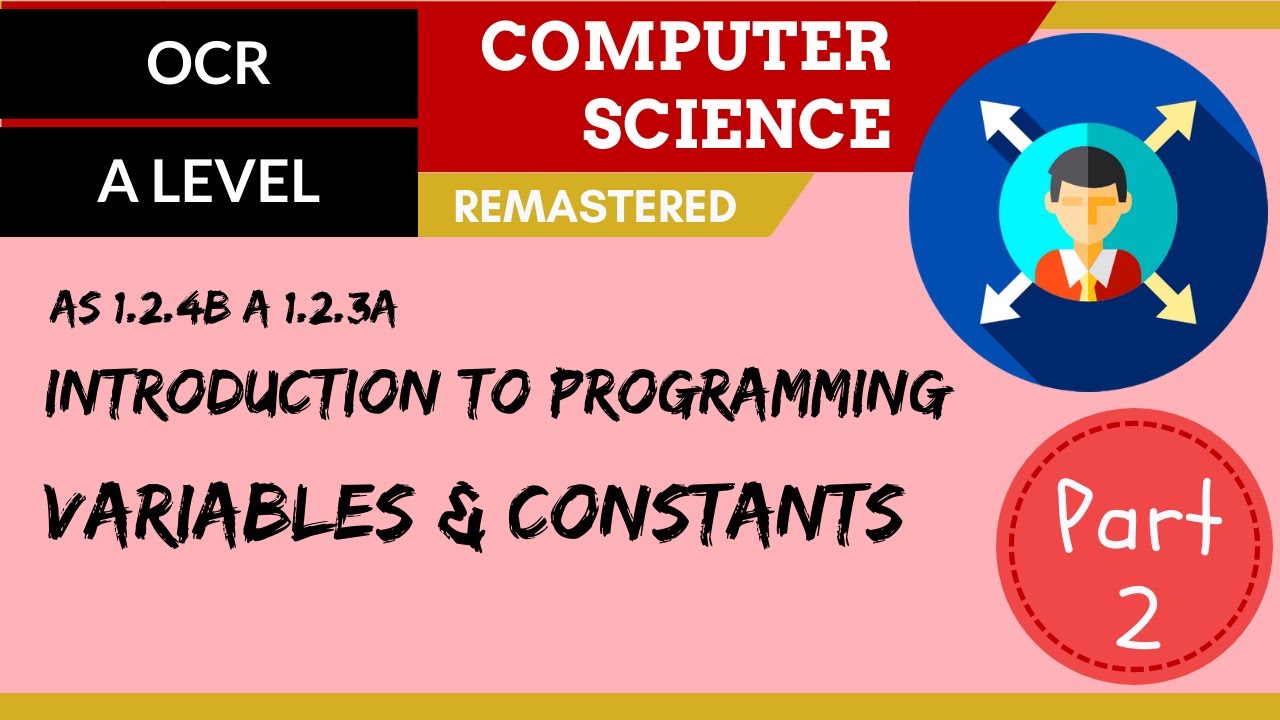
41. OCR A Level (H046-H446) SLR8 - 1.2 Introduction to programming part 2 variables & constants
5.0 / 5 (0 votes)