React Axios | Tutorial for Axios with ReactJS for a REST API
Summary
TLDRThis tutorial demonstrates how to integrate Axios with a React application to manage HTTP requests. The video covers setting up Axios, making GET, POST, DELETE, and PATCH requests, and dynamically updating the UI based on API responses. It also discusses error handling with try-catch blocks, passing parameters in requests, and adding authentication using tokens. By the end of the video, you'll understand how to interact with a REST API in a React app, including creating, updating, and deleting data, all while keeping the UI synchronized with the server.
Takeaways
- 😀 Axios is a popular promise-based HTTP request client used in React applications for making API calls.
- 😀 To use Axios in a React project, you need to install it via npm and import it into your component.
- 😀 You can configure Axios by creating an instance with a base URL and use it to perform GET requests to fetch data.
- 😀 In React, the fetched data can be stored in state and rendered dynamically using the `map` function.
- 😀 You can use Axios to send POST requests to create new data, such as adding a new course to the database.
- 😀 To update the data in the API, you can use a PATCH request with Axios to modify existing records.
- 😀 Axios can also be used for DELETE requests to remove data from the database and update the UI accordingly.
- 😀 Using async/await helps simplify handling asynchronous requests and ensures clean and readable code.
- 😀 Axios supports error handling using try/catch blocks to manage failed API calls and prevent application crashes.
- 😀 Axios can be used for adding query parameters to GET requests, enabling features like pagination or filtering.
- 😀 Axios can handle authentication by passing headers (e.g., API tokens) in the request, ensuring secure access to endpoints.
Q & A
What is Axios and how is it used in a React application?
-Axios is a popular promise-based HTTP client used for making HTTP requests, such as GET, POST, DELETE, and PATCH. In a React application, Axios can be used to interact with APIs to fetch or send data, and it works well with React's state management to update UI based on API responses.
How do you install Axios in a React project?
-To install Axios in a React project, you need to run `npm install axios` in your project folder. This will add Axios to the project's dependencies listed in `package.json`.
How do you set up Axios with a base URL in React?
-You can set up Axios with a base URL by creating an Axios instance using `const api = axios.create({ baseURL: 'your-api-url' })`. This instance can then be used to make requests to your API.
How does React fetch data from an API using Axios?
-To fetch data, you can use Axios inside a React component's `componentDidMount()` lifecycle method or a `useEffect()` hook in functional components. You perform a `GET` request and update the component's state with the data once it is received.
What are some examples of HTTP requests you can make with Axios?
-With Axios, you can make various types of HTTP requests such as: `GET` for fetching data, `POST` for creating new data, `DELETE` for removing data, and `PATCH` for updating existing data.
How can you use the `POST` method in Axios to send data to an API?
-To send data using the `POST` method, you can use `axios.post(url, data)`, where `url` is the API endpoint, and `data` is the object containing the information you want to send to the server. This can be useful for creating new resources.
What is the role of `this.setState()` in React when working with Axios?
-`this.setState()` is used to update the component's state with the data fetched from an API. After a successful Axios request, you can call `this.setState({ courses: data })` to store the data and trigger a re-render to display it in the UI.
Why would you use `async/await` with Axios in React?
-Using `async/await` allows you to handle asynchronous operations in a cleaner way by avoiding nested `.then()` callbacks. It simplifies the code and makes it easier to handle errors and API responses.
How can you handle errors in Axios requests?
-You can handle errors using `try-catch` blocks in `async` functions or by using `.catch()` with Axios promises. This ensures that if an error occurs (e.g., the API is unreachable), it is logged or handled gracefully without crashing the application.
How can Axios help with paginating API responses?
-Axios allows you to pass query parameters in the request, such as `limit` and `start`, to paginate API responses. For example, you can limit the number of items per request and specify where to start fetching, which can be useful for implementing infinite scroll or pagination in your app.
Outlines
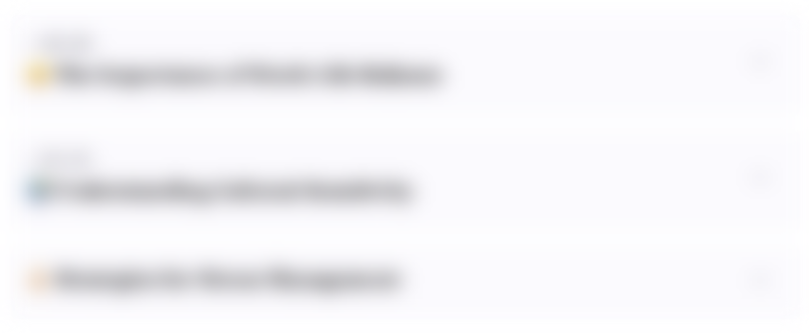
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
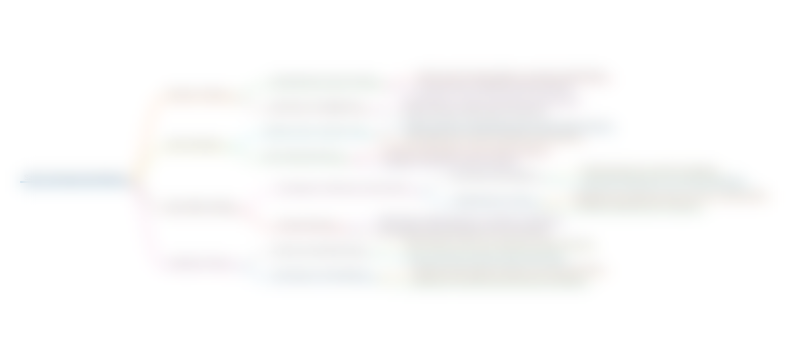
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
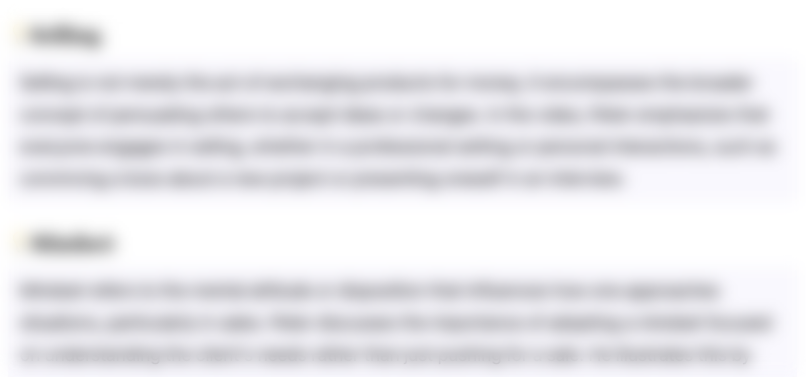
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
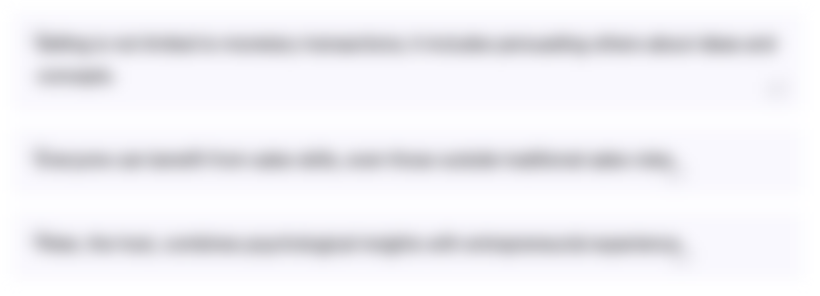
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
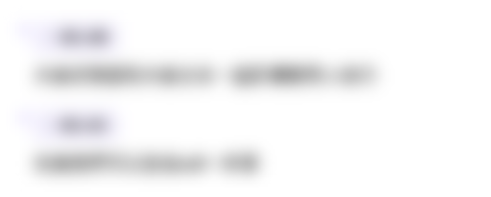
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
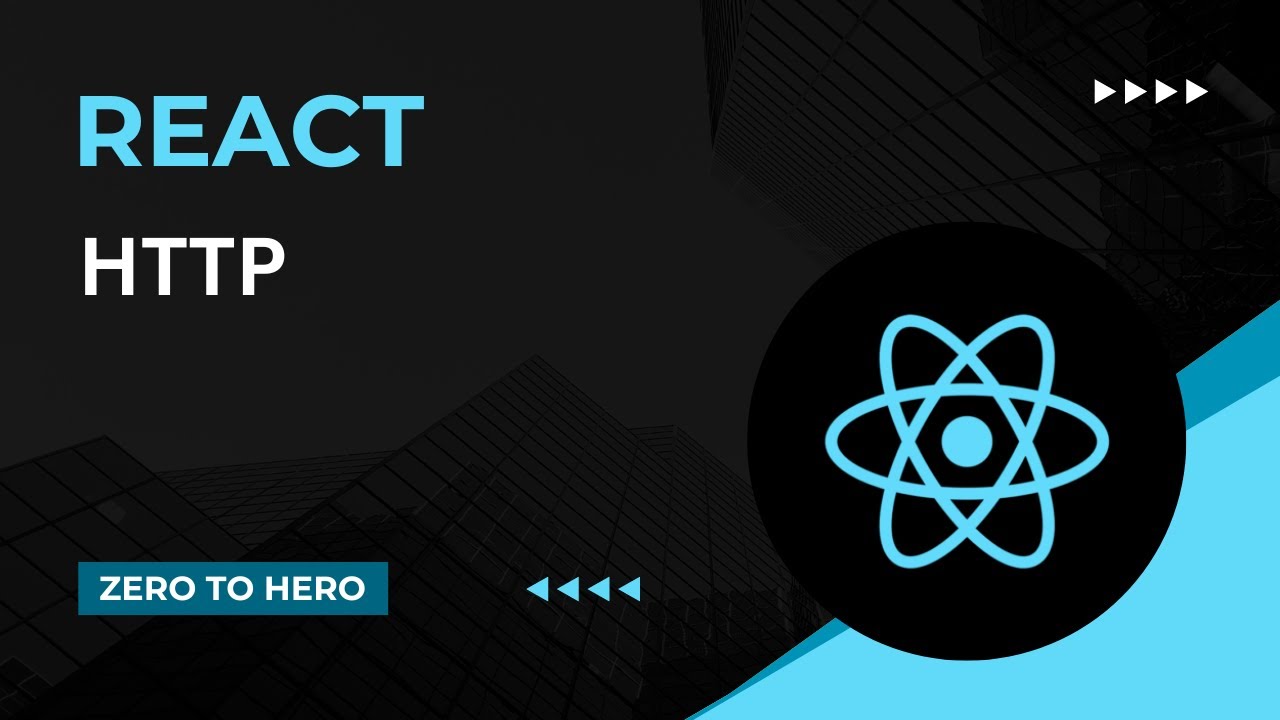
HTTP | Mastering React: An In-Depth Zero to Hero Video Series
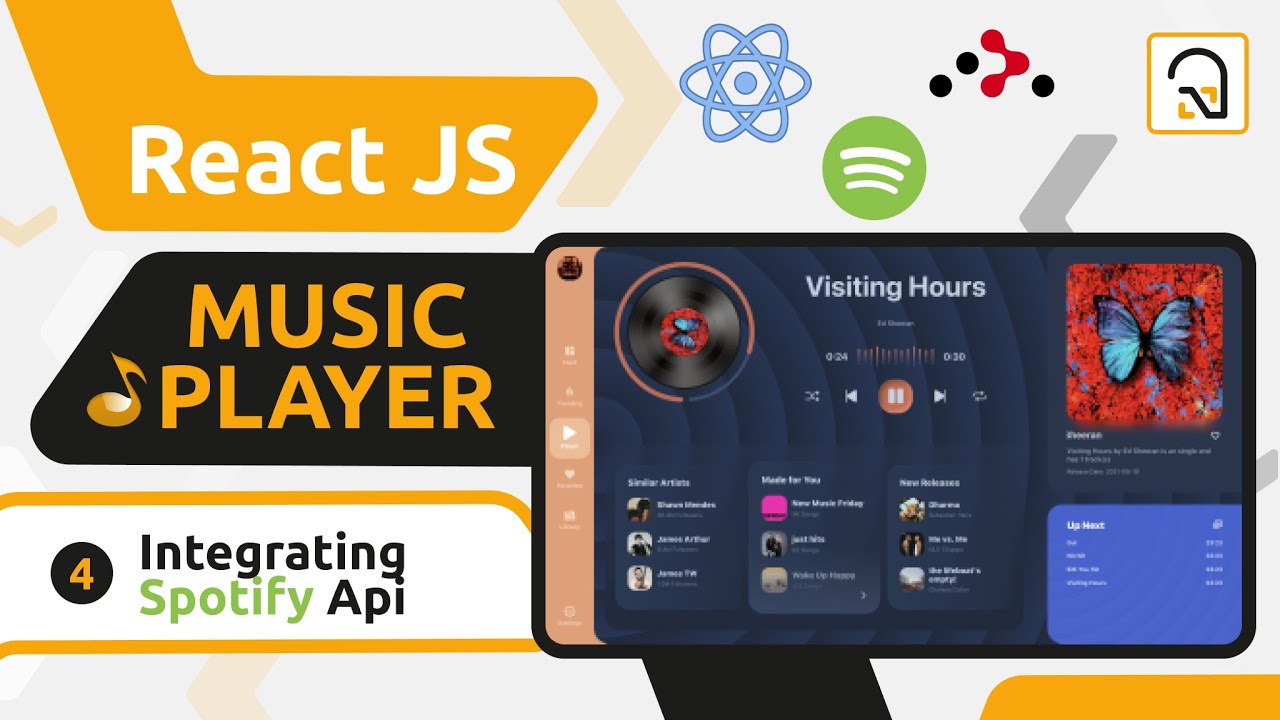
ReactJS Music Player #4: Integrating the Spotify Api in our React App

MERN Stack Tutorial #2 - Express App Setup

#08 HTTP Request & Response | HTTP Request & Response | ASP.NET Core MVC Course
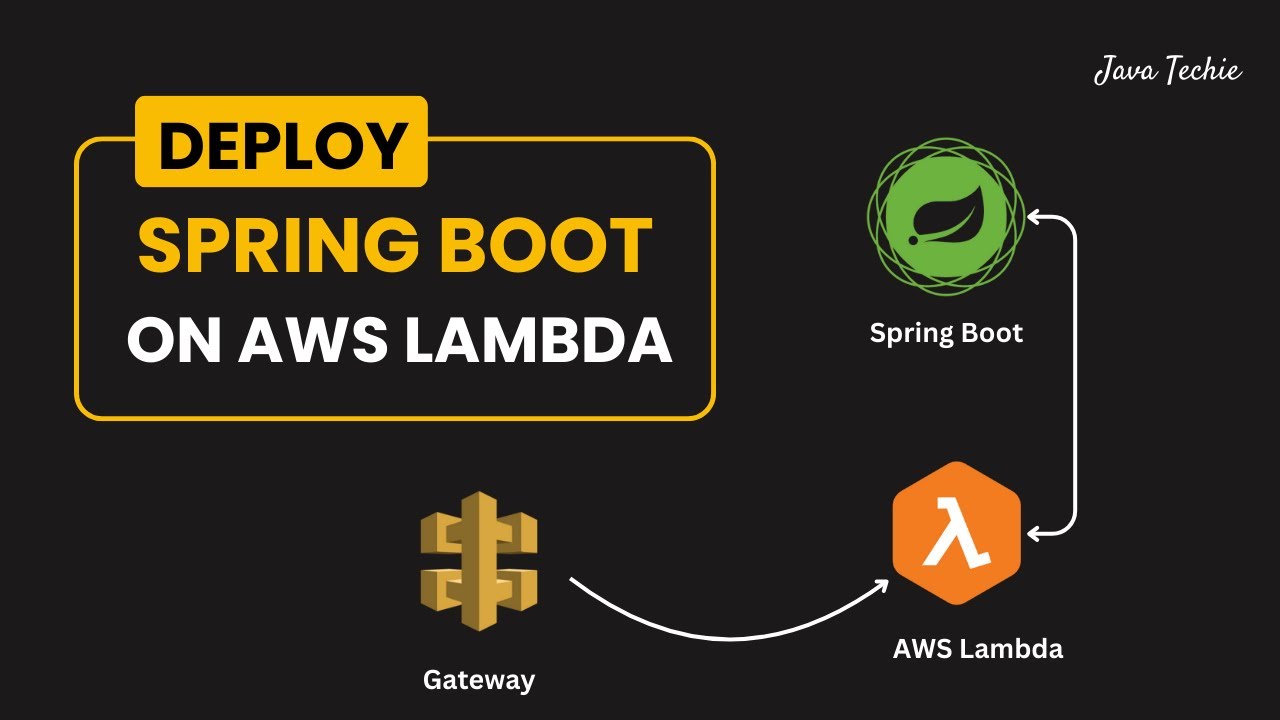
Deploy Spring Boot Serverless CRUD API to AWS Lambda 🔥 | API Gateway | @Javatechie
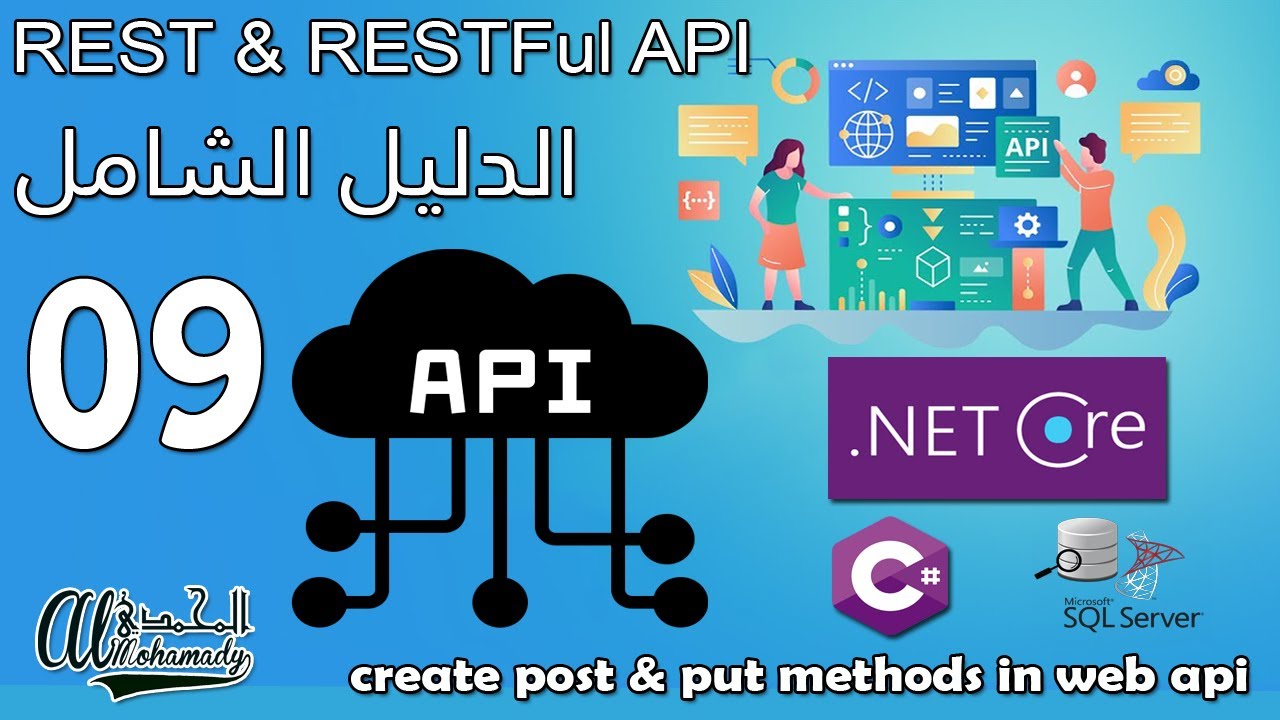
09 إنشاء دوال الحفظ و التعديل create post & put methods in web api
5.0 / 5 (0 votes)