L-5.5: Sum of Subsets Problem | Dynamic Programming
Summary
TLDRIn this video, the Sum of Subset problem is explained in detail, focusing on the task of finding a subset of a set whose sum equals a given value. The speaker covers both brute force and dynamic programming approaches to solving the problem, emphasizing the differences in time complexity. The brute force method involves checking all subsets, while the dynamic programming approach optimizes the solution by storing results of subproblems, reducing the time complexity from exponential to polynomial. The video also includes an explanation of the recursive equation and the concept of overlapping subproblems, which leads to a more efficient solution.
Takeaways
- 😀 The 'Sum of Subset' problem involves finding if there is a subset of numbers from a given set that adds up to a target sum.
- 😀 The main goal is to determine whether such a subset exists, answering 'Yes' or 'No', without needing to find the actual subset.
- 😀 A brute force approach involves checking all possible subsets, which has an exponential time complexity of O(2^n), where n is the number of elements.
- 😀 The problem can be solved recursively by either including or excluding each element of the set and checking the remaining sum.
- 😀 A recursive equation is used in dynamic programming to optimize the solution, with base cases such as returning 'True' if the sum is zero and 'False' if no elements remain.
- 😀 Dynamic programming optimizes the recursive approach by storing intermediate results, thus avoiding redundant calculations and reducing time complexity.
- 😀 The time complexity of the dynamic programming approach is O(n * m), where n is the number of elements in the set and m is the target sum.
- 😀 Dynamic programming is more efficient than brute force when the target sum (m) is not excessively large, making the solution feasible for smaller sums.
- 😀 If the target sum is much larger than the number of elements in the set, the time complexity of dynamic programming might not be significantly better than brute force.
- 😀 The video also explains the recursive tree structure, where each node represents a choice (include or exclude an element), and the total depth corresponds to the number of elements in the set.
- 😀 The solution can be optimized by using dynamic programming to solve overlapping subproblems, reducing the number of unique subproblems to O(n * m).
Q & A
What is the main goal of the Sum of Subset problem?
-The goal is to determine if there exists a subset of a given set whose sum is equal to a specified target sum. The answer should be either 'Yes' or 'No'.
How does the brute force method approach the Sum of Subset problem?
-The brute force method involves generating all possible subsets of the set, calculating their sums, and checking if any subset's sum equals the target value. This results in an exponential time complexity of O(2^n).
What is the time complexity of the brute force method?
-The time complexity of the brute force method is O(2^n), where `n` is the number of elements in the set.
What is the recursive approach used to solve the Sum of Subset problem?
-In the recursive approach, for each element, you either include it in the subset or exclude it. The sum is adjusted accordingly, and the problem is solved recursively by considering both cases until a base case is met.
What are the base cases for the recursive equation?
-The base cases are: 1) If there are no elements left and the sum is 0, return 'Yes'. 2) If there are no elements left and the sum is not 0, return 'No'. 3) If the sum is 0 and there are elements left, return 'Yes'. 4) If an element's value exceeds the target sum, exclude it.
How does dynamic programming improve the brute force method?
-Dynamic programming improves the brute force method by storing the results of subproblems in a table to avoid redundant calculations. This reduces the time complexity from exponential to polynomial.
What is the time complexity of the dynamic programming approach?
-The time complexity of the dynamic programming approach is O(n * m), where `n` is the number of elements and `m` is the target sum.
When is the dynamic programming approach more efficient than the brute force method?
-The dynamic programming approach is more efficient when the target sum is relatively small compared to the number of elements, as it reduces the time complexity from O(2^n) to O(n * m).
What happens when the target sum is greater than 2^n?
-If the target sum is very large, greater than 2^n, the dynamic programming approach will not provide significant benefits, and the time complexity will behave similarly to the brute force method, which is exponential.
Why is the time complexity of dynamic programming O(n * m)?
-The time complexity is O(n * m) because we are solving a subproblem for each combination of elements (`n`) and each possible sum (`m`), storing the results to avoid redundant computations.
Outlines
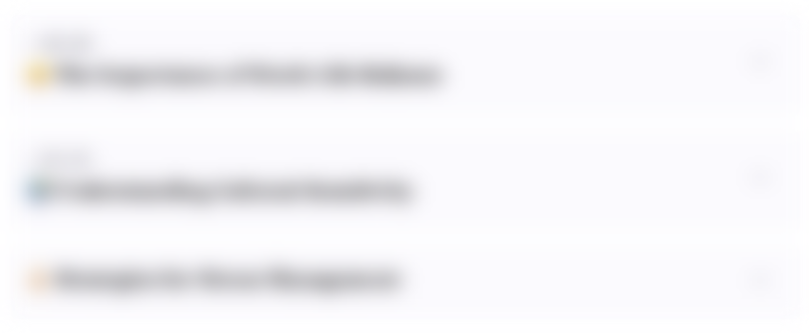
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
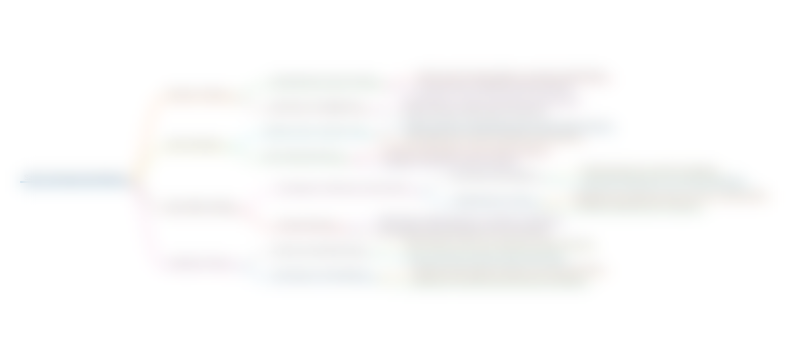
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
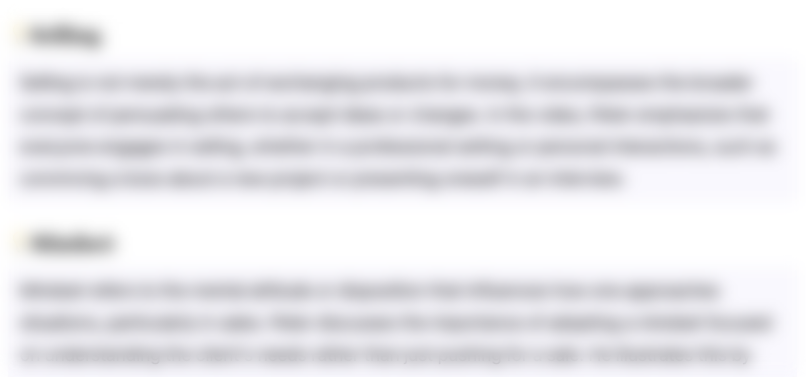
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
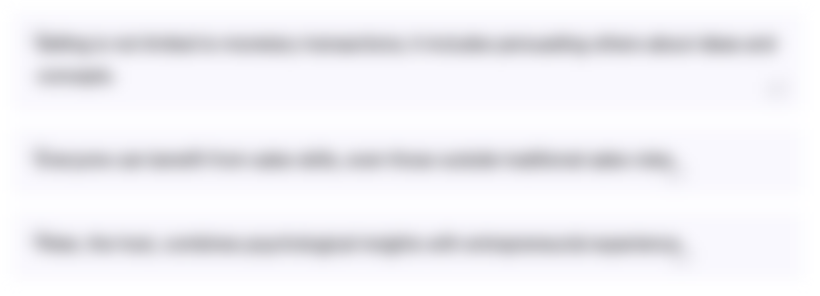
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
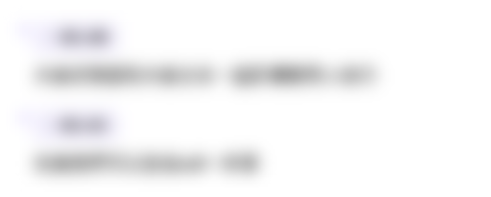
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
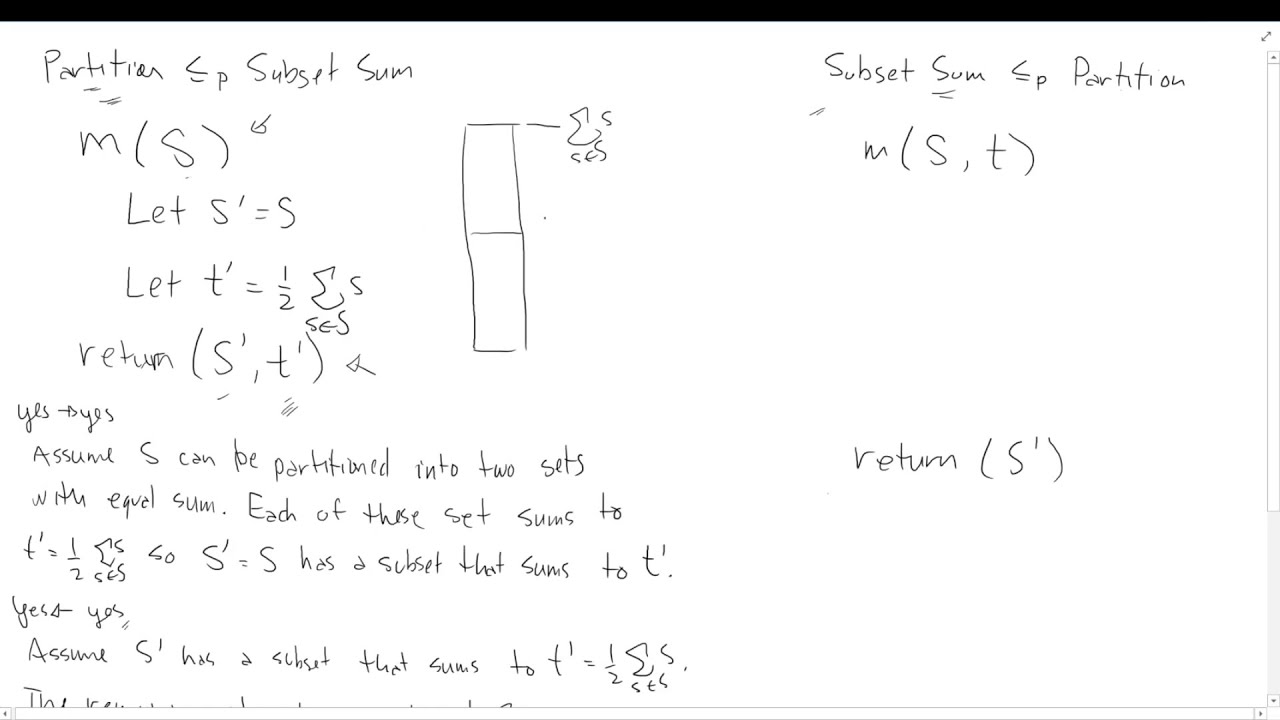
Subset Sum and Partition are NP-complete - Complexity Theory - Design and Analysis of Algorithms
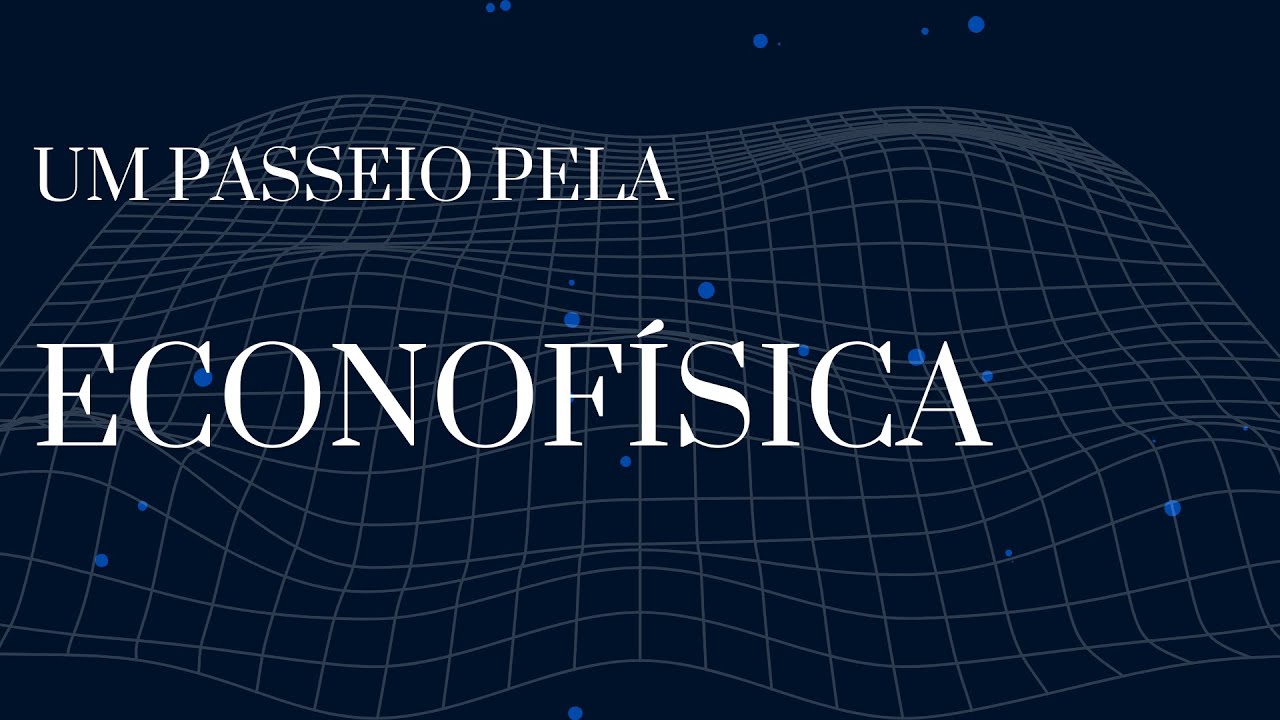
Econofísica - 5 Momentos Estatísticos
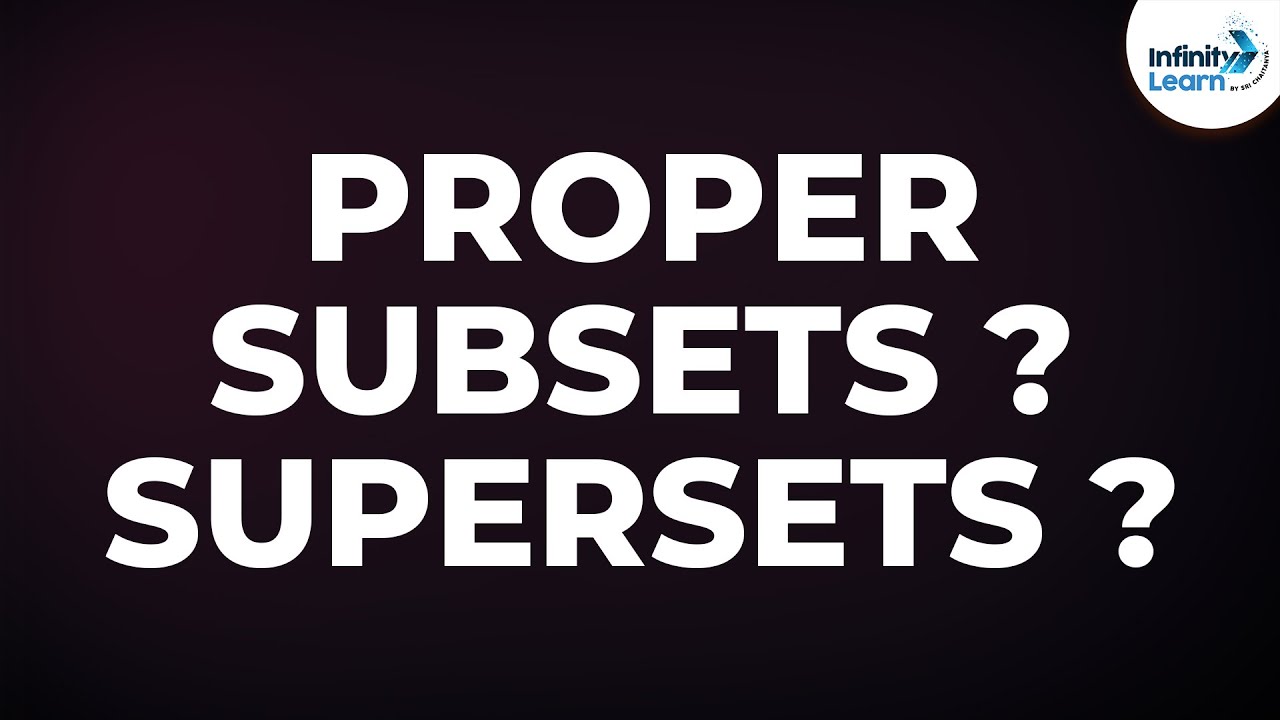
Subsets, Proper Subsets and Supersets | Don't Memorise

Solving Problems Involving Quadratic Equations and Rational Algebraic Equations (Part 1)
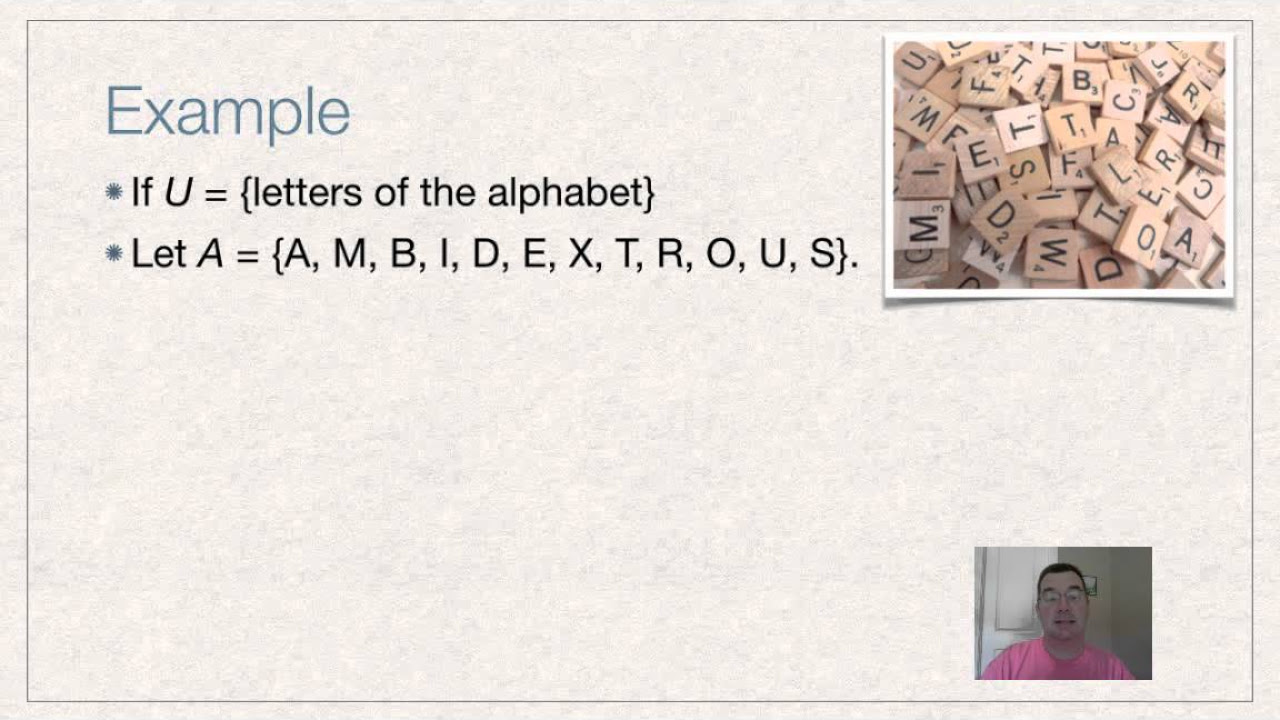
Complements of Sets

Types of sets
5.0 / 5 (0 votes)