Math in Python is easy + exercises 📐
Summary
TLDRIn this Python tutorial, the basics of arithmetic operators and math functions are covered, including addition, subtraction, multiplication, division, exponents, and modulus. The video explains how to use built-in functions like `round()`, `abs()`, `pow()`, and how to access constants like `math.pi` and `math.e` from the `math` module. Exercises include calculating the circumference and area of a circle, as well as finding the hypotenuse of a right triangle. The lesson is perfect for beginners looking to strengthen their understanding of math operations in Python.
Takeaways
- 😀 Basic arithmetic operators in Python include addition (+), subtraction (-), multiplication (*), division (/), exponentiation (**), and modulus (%).
- 😀 The augmented assignment operators like +=, -=, *=, /=, **=, and %= are shortcuts for modifying variables more concisely.
- 😀 The modulus operator (%) is often used to check whether a number is even or odd by dividing the number by 2.
- 😀 The round() function rounds a floating point number to the nearest integer or specified decimal places.
- 😀 The abs() function returns the absolute value of a number, which is its distance from zero without regard to sign.
- 😀 The pow() function raises a number (base) to a specified power (exponent).
- 😀 The max() and min() functions allow you to find the maximum and minimum values from a set of numbers.
- 😀 The math module in Python provides constants like math.pi (for π) and math.e (for the exponential constant) as well as useful functions like math.sqrt() for square roots.
- 😀 The math module includes math.ceil() to round up a number and math.floor() to round down a number.
- 😀 Practical exercises demonstrated include calculating the circumference and area of a circle, as well as the hypotenuse of a right triangle, using relevant formulas and math functions.
Q & A
What is the purpose of using augmented assignment operators in Python?
-Augmented assignment operators, like '+=', '-=' or '*=', are used to modify the value of a variable in a concise manner. They save space and improve readability by combining an operation with an assignment, reducing the need for longer expressions.
What does the modulus operator (%) do in Python?
-The modulus operator (%) returns the remainder of a division. For example, if you divide 10 by 3, the remainder is 1. It’s commonly used to determine whether a number is even or odd by checking the remainder when divided by 2.
How does the round function in Python work?
-The 'round' function in Python rounds a floating-point number to the nearest integer. You can also specify the number of decimal places you want to round to, which is useful for limiting the precision of results in calculations.
What is the difference between 'math.ceil()' and 'math.floor()' functions?
-The 'math.ceil()' function rounds a number up to the nearest integer, while 'math.floor()' rounds a number down to the nearest integer. For example, 'math.ceil(9.1)' would return 10, while 'math.floor(9.9)' would return 9.
What is the 'abs()' function used for in Python?
-The 'abs()' function returns the absolute value of a number, which is its distance from zero without considering its sign. For instance, 'abs(-4)' will return 4, and 'abs(4)' will return 4.
What is the importance of the 'math' module in Python?
-The 'math' module provides access to mathematical functions like square roots, trigonometric functions, and constants like 'pi' and 'e'. It's essential for performing advanced mathematical operations that aren't available with basic Python syntax.
How do you calculate the circumference of a circle in Python?
-To calculate the circumference of a circle, you can use the formula: circumference = 2 * pi * radius. You would import the 'math' module to access 'math.pi' and ask for user input for the radius, then apply the formula.
What is the formula for calculating the area of a circle?
-The formula for calculating the area of a circle is: area = pi * radius^2. In Python, you can use 'math.pi' for the value of pi and 'pow(radius, 2)' or 'radius ** 2' to square the radius.
How can you calculate the hypotenuse of a right triangle in Python?
-To calculate the hypotenuse of a right triangle, you use the Pythagorean theorem: C = sqrt(A^2 + B^2). In Python, you would square both sides (A and B), sum them, and then apply 'math.sqrt()' to find the hypotenuse.
What happens if you divide a number by zero in Python?
-Dividing a number by zero in Python raises a 'ZeroDivisionError'. To prevent this, it's important to check for division by zero before performing a division operation.
Outlines
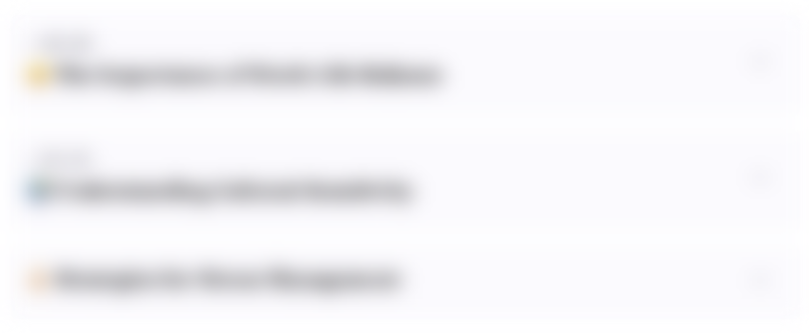
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
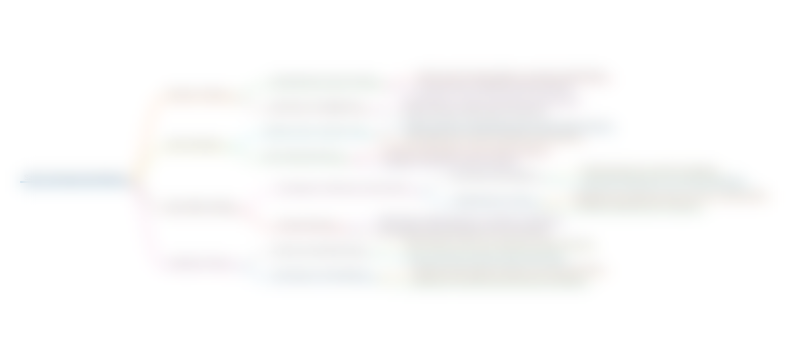
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
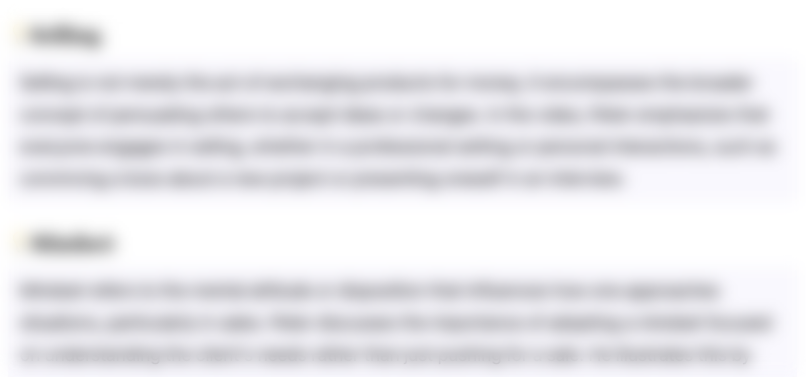
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
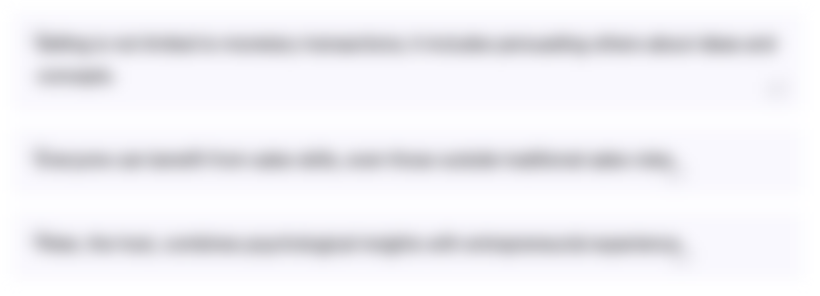
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
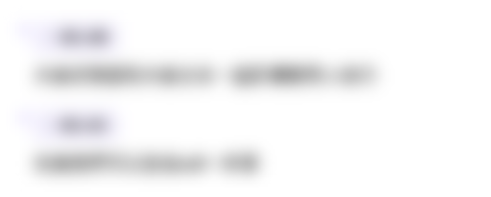
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
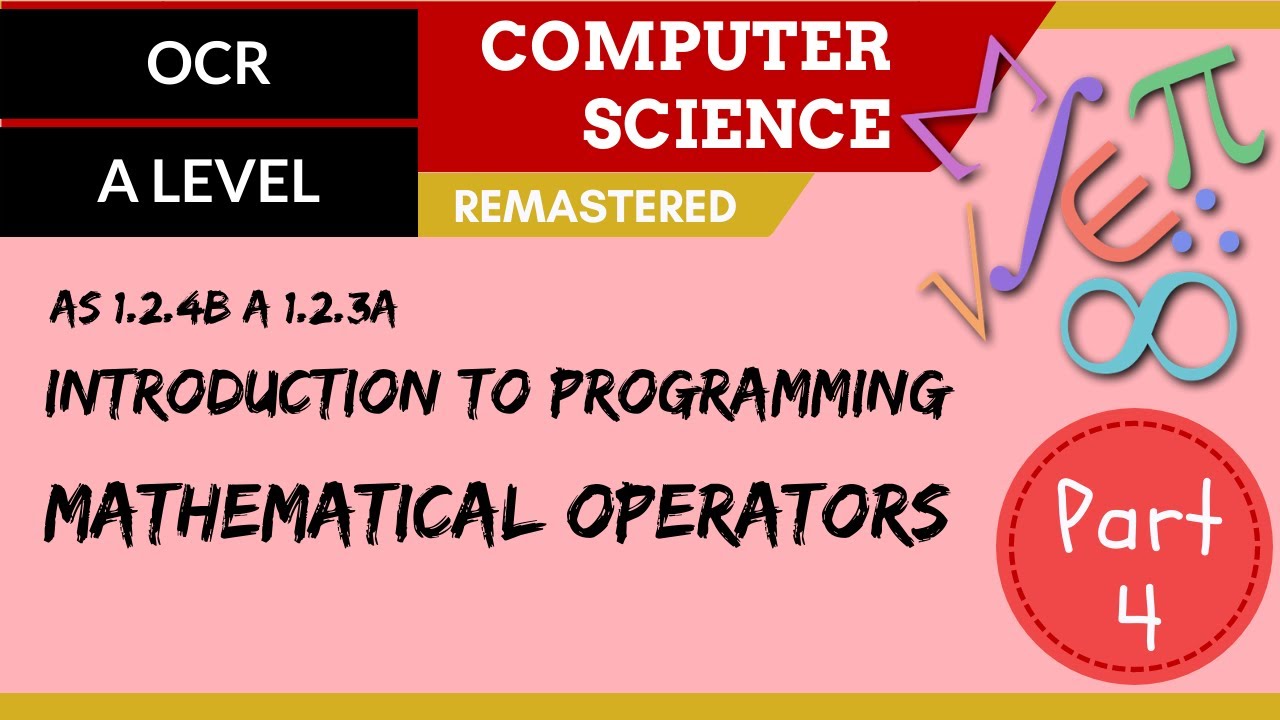
43. OCR A Level (H046-H446) SLR8 - 1.2 Introduction to programming part 4 mathematical operators
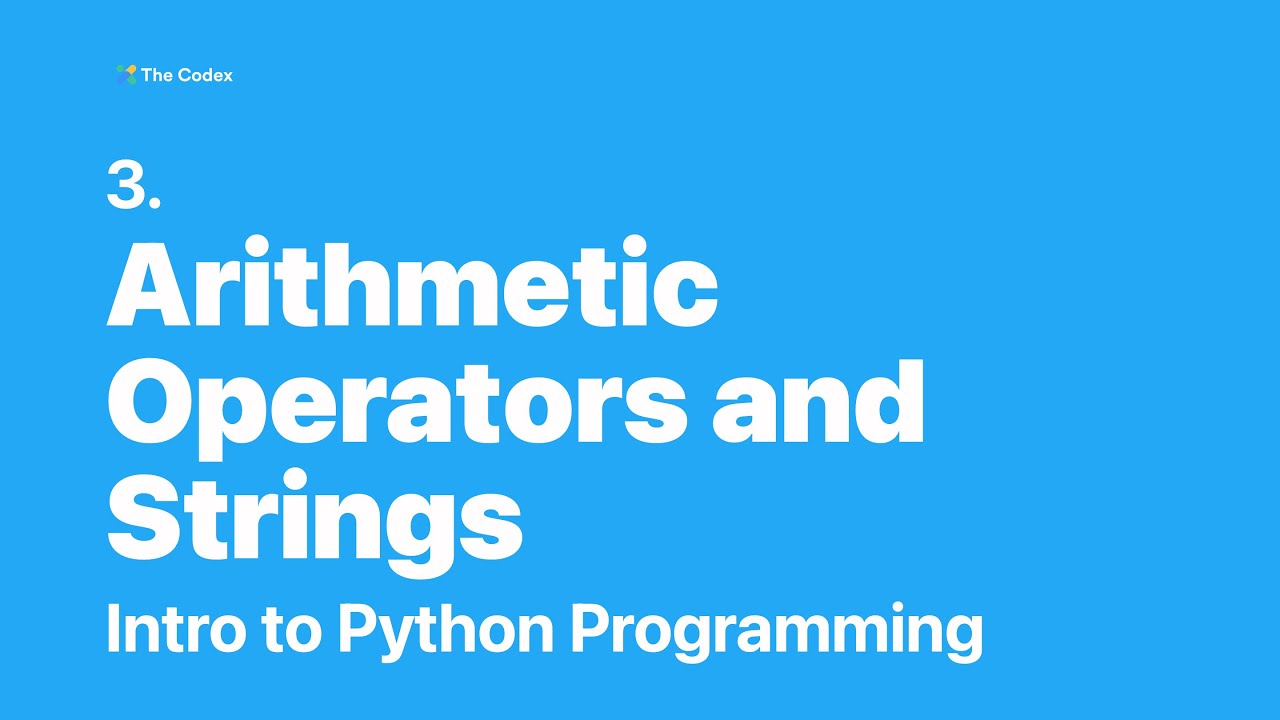
Python Programming #3 - Arithmetic Operators and Strings
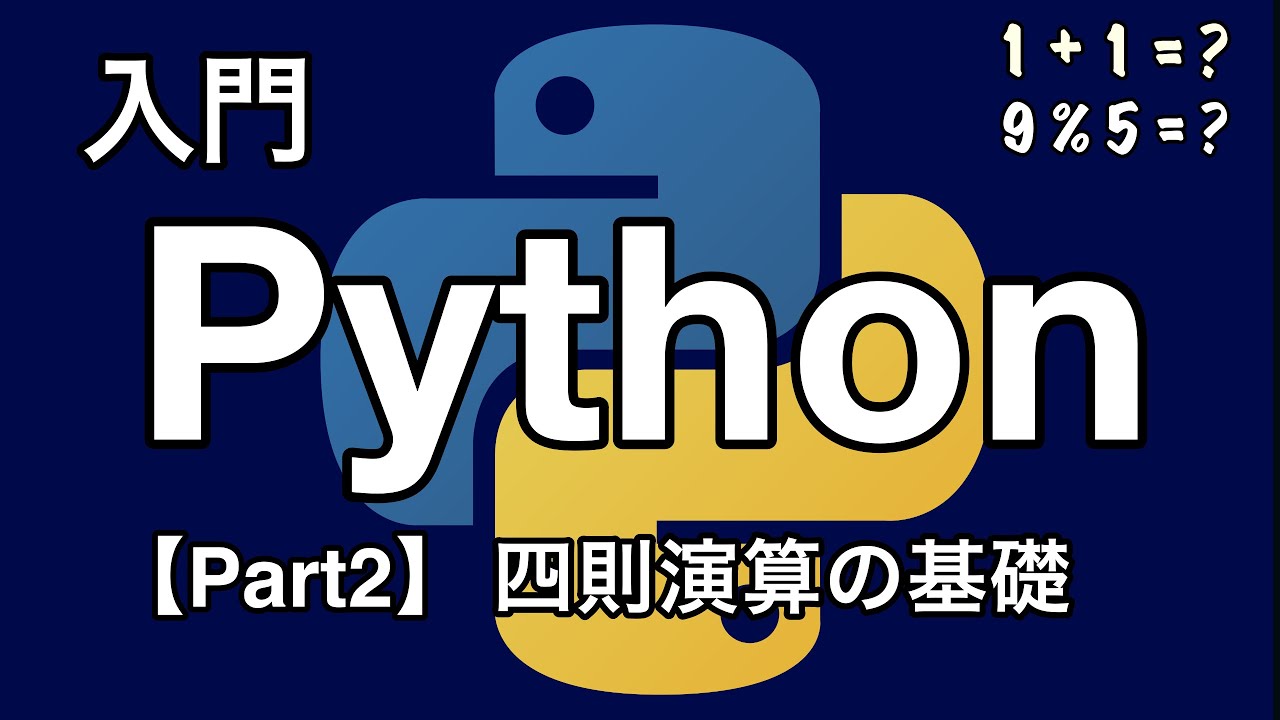
【Python入門編】四則演算の基礎 【Part2】
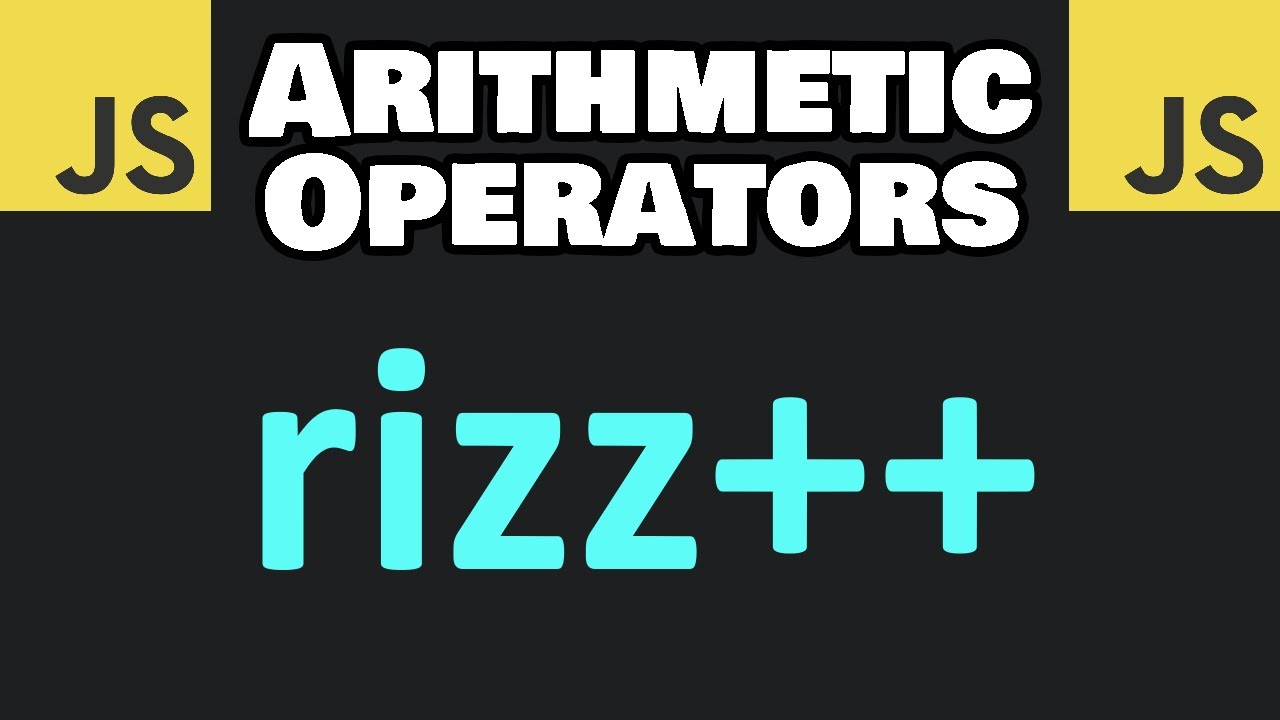
JavaScript ARITHMETIC OPERATORS in 8 minutes! ➕
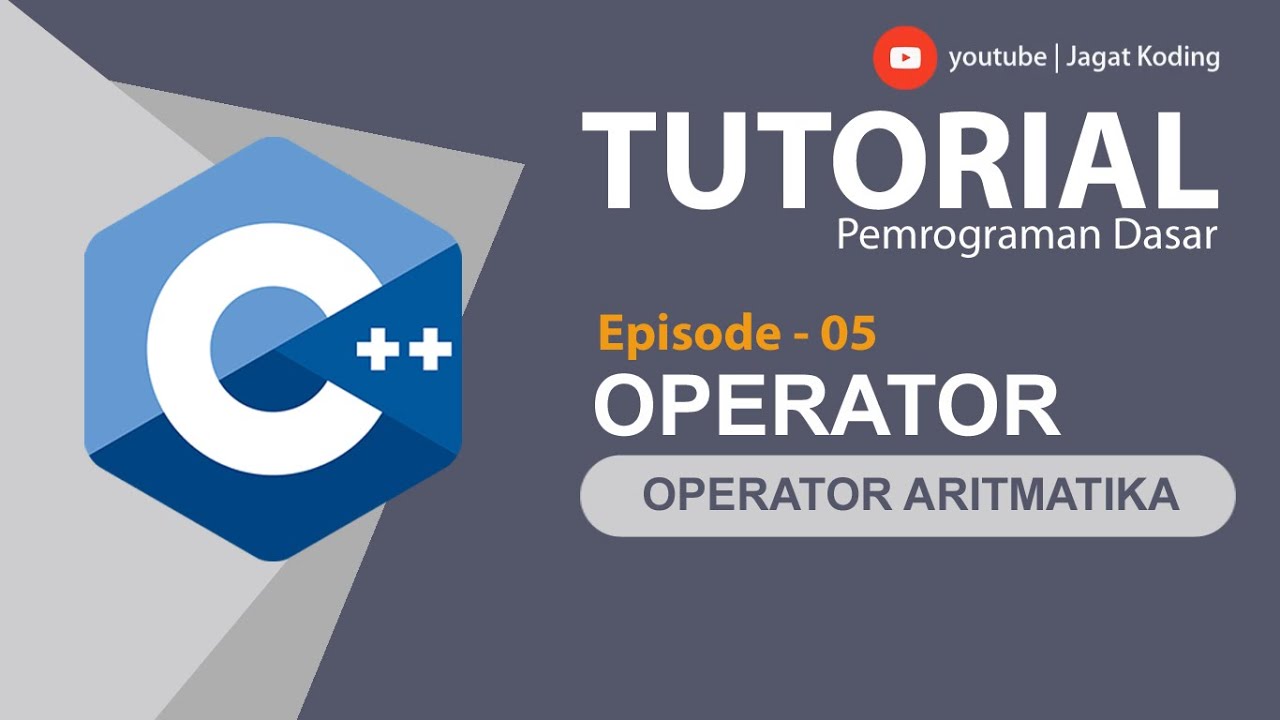
C++ 05 | Operator Aritmatika | Tutorial Dev C++ Indonesia
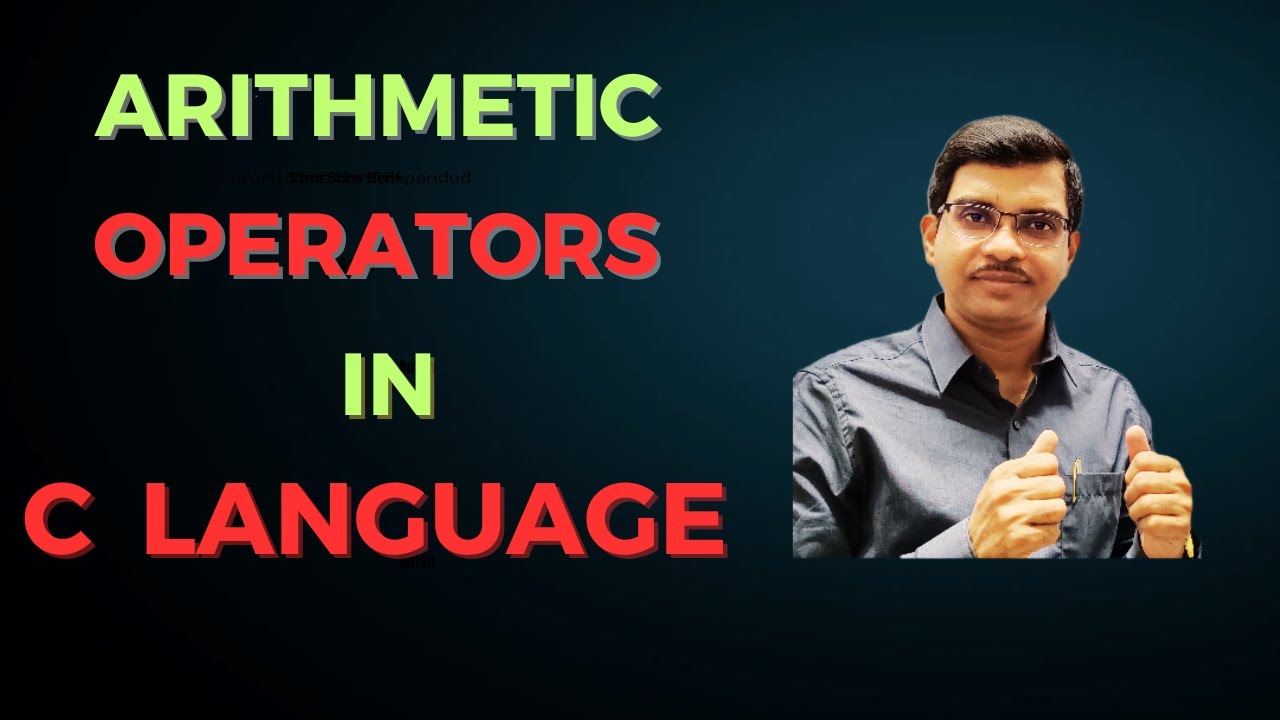
C_12 Arithmetic Operators in C Language | C Programming Tutorials
5.0 / 5 (0 votes)