2 Seeding1
Summary
TLDRThis video tutorial introduces the concept of database seeding in Laravel, explaining how to populate a database with predefined data. It covers creating a seeder using the `php artisan make:seeder` command, defining the data to be inserted using Laravel's `DB` facade, and running the seeder with the `php artisan db:seed` command. The tutorial also includes a practical example of inserting records into the `students` table. By the end, viewers will understand how to seed their Laravel database effectively for development purposes.
Takeaways
- 😀 Database seeding in Laravel is the process of populating your database with predefined data, often for testing purposes.
- 😀 To create a seeder in Laravel, use the Artisan command: `php artisan make:seeder SeederName`.
- 😀 The seeder file is created in the `database/seeders` directory, where you define the data to be inserted into the database.
- 😀 Laravel’s DB class is used to interact with the database in the seeder file, particularly with the `table()` and `insert()` methods.
- 😀 The example provided demonstrates how to insert data into the `students` table using an array of records.
- 😀 The `insert()` method allows you to pass multiple records in an array format, each record representing a row in the table.
- 😀 After defining your seeder, you can execute it using the command: `php artisan db:seed --class=SeederName`.
- 😀 The seeder class should be referenced in the `--class` option when running the Artisan command to specify which seeder to run.
- 😀 After running the seeder, you can check the database to verify the records were inserted successfully using tools like phpMyAdmin or Laravel's built-in database tools.
- 😀 Database seeders are helpful during development to quickly populate your database with sample data.
- 😀 The script highlights that Laravel's seeding process is a simple and effective way to automate data insertion into tables without manually entering it.
Q & A
What is database seeding in Laravel?
-Database seeding in Laravel is the process of populating the database with predefined data. This is often used to initialize the database with test data or default records during development.
How do you create a seeder in Laravel?
-You can create a seeder in Laravel using the Artisan command: `php artisan make:seeder SeederName`. The seeder name should be singular and represent the table name (e.g., `StudentSeeder` for the `students` table).
What does the `run()` method in a Laravel seeder do?
-The `run()` method in a Laravel seeder is where you define the actions to populate the database. Typically, you use the `DB::table()->insert()` method to insert data into the specified table.
What is the purpose of the `DB` facade in Laravel?
-The `DB` facade in Laravel provides a simple interface to interact with the database, including running queries and inserting data into tables.
What is the correct format for inserting data in a Laravel seeder?
-Data is inserted in a Laravel seeder using an associative array format. For example, `DB::table('students')->insert([['id' => 1, 'name' => 'John', 'score' => 80], ...]);`.
How do you run a specific seeder in Laravel?
-To run a specific seeder in Laravel, use the command `php artisan db:seed --class=SeederName`, replacing `SeederName` with the name of the seeder class, e.g., `StudentSeeder`.
What is the difference between `php artisan db:seed` and `php artisan db:seed --class=SeederName`?
-The command `php artisan db:seed` runs all seeders defined in the `DatabaseSeeder` class, while `php artisan db:seed --class=SeederName` runs a specific seeder class.
How can you verify if the data has been successfully seeded into the database?
-To verify if the data has been successfully seeded, you can check the corresponding table in your database, either using a database management tool or by querying the table directly.
What does the command `php artisan make:seeder StudentSeeder` generate?
-The command `php artisan make:seeder StudentSeeder` generates a seeder file named `StudentSeeder.php` in the `database/seeders` directory, containing a `run()` method where you can define the data insertion logic.
Can you insert multiple records in a single seeder in Laravel?
-Yes, you can insert multiple records in a single seeder by passing an array of associative arrays to the `insert()` method, with each array representing a record to be inserted.
Outlines
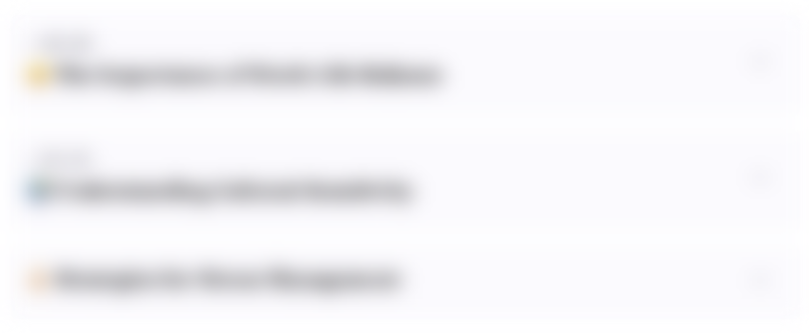
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
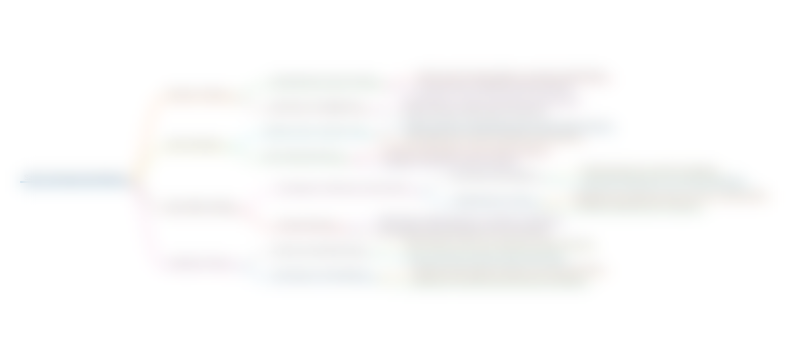
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
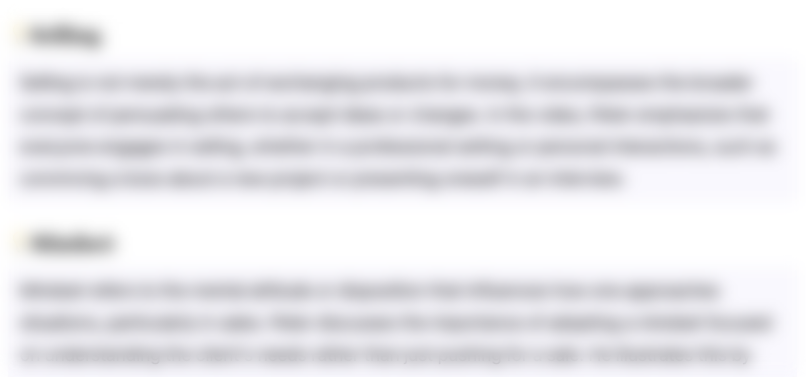
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
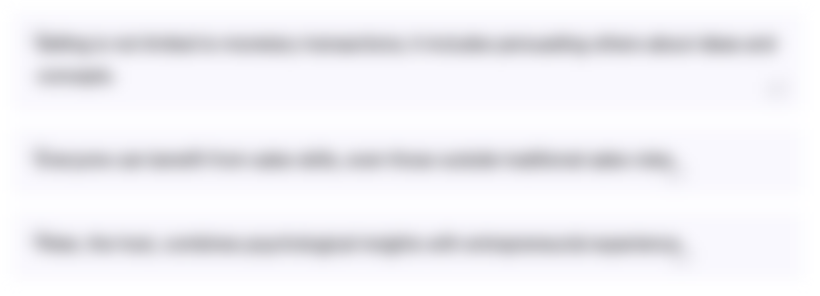
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
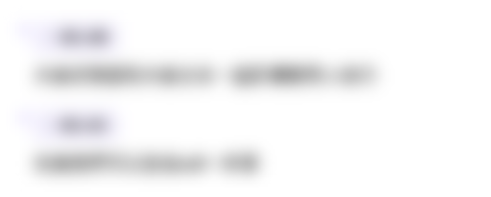
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
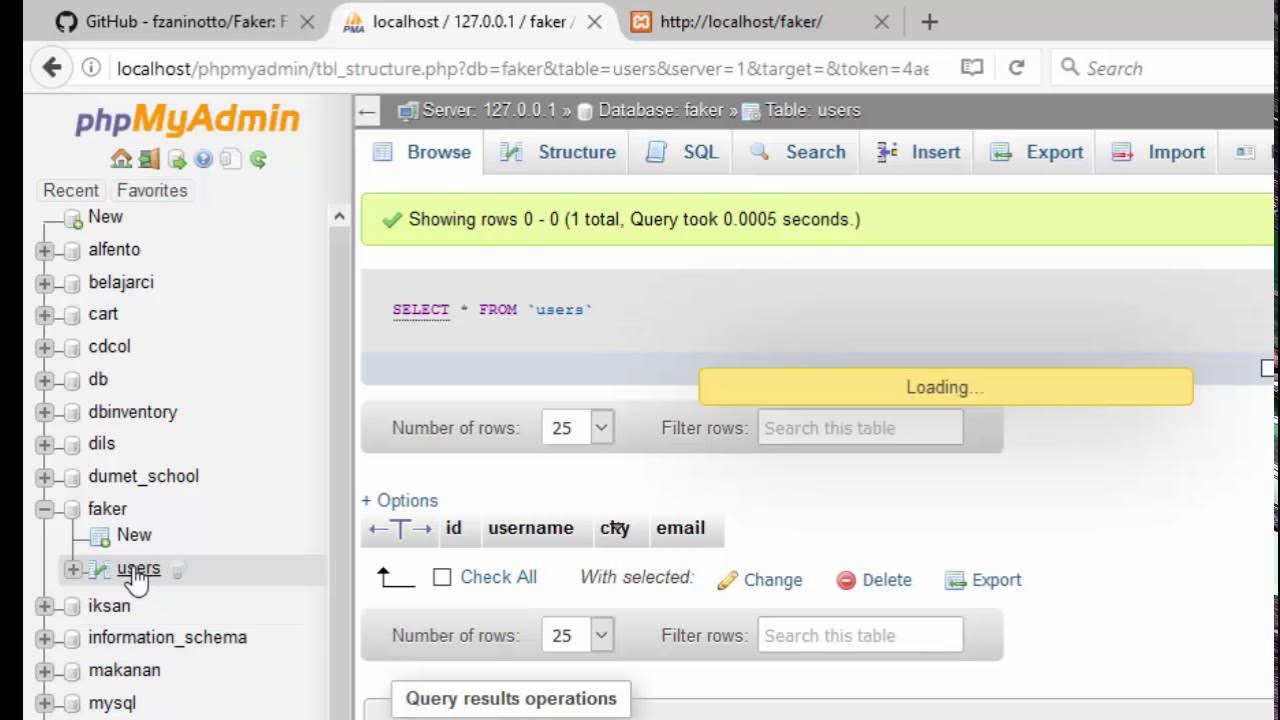
Cara Mengisi Databases Menggunakan Data Faker

[BGD01B-ID] Introduction to Databases
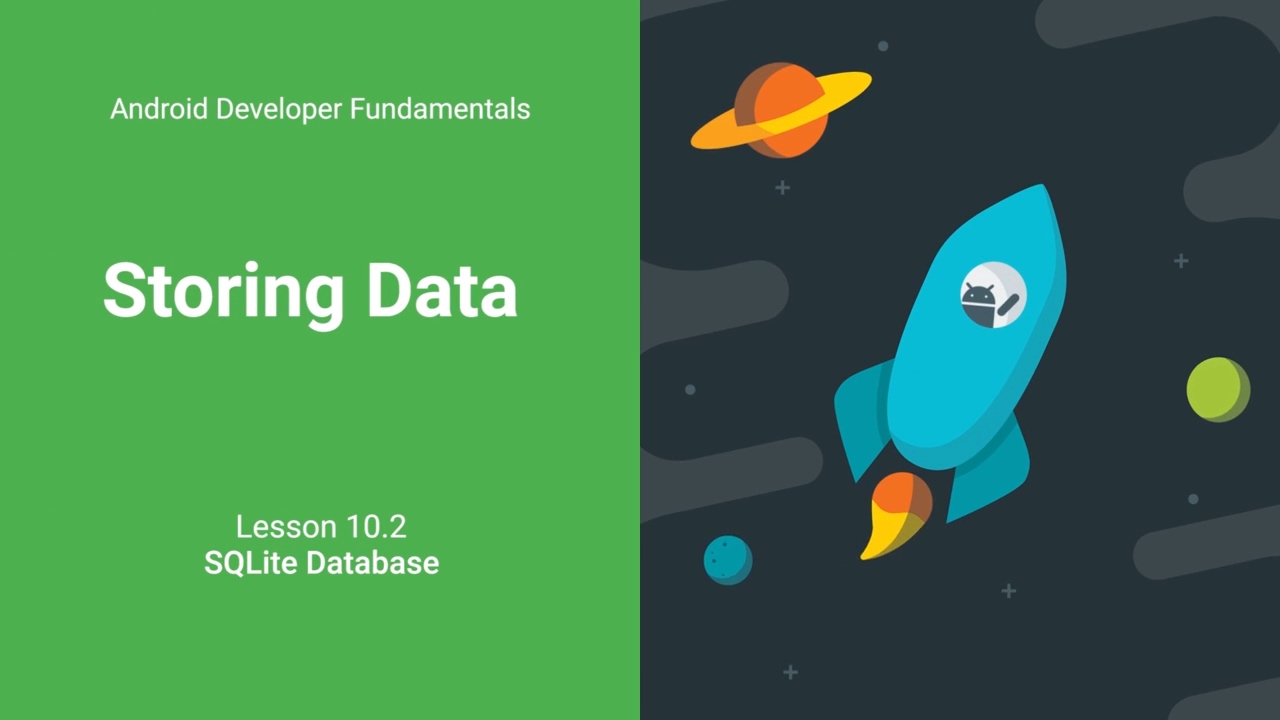
Store Data Using SQLite Database (Android Development Fundamentals, Unit 4: Lesson 10.2)
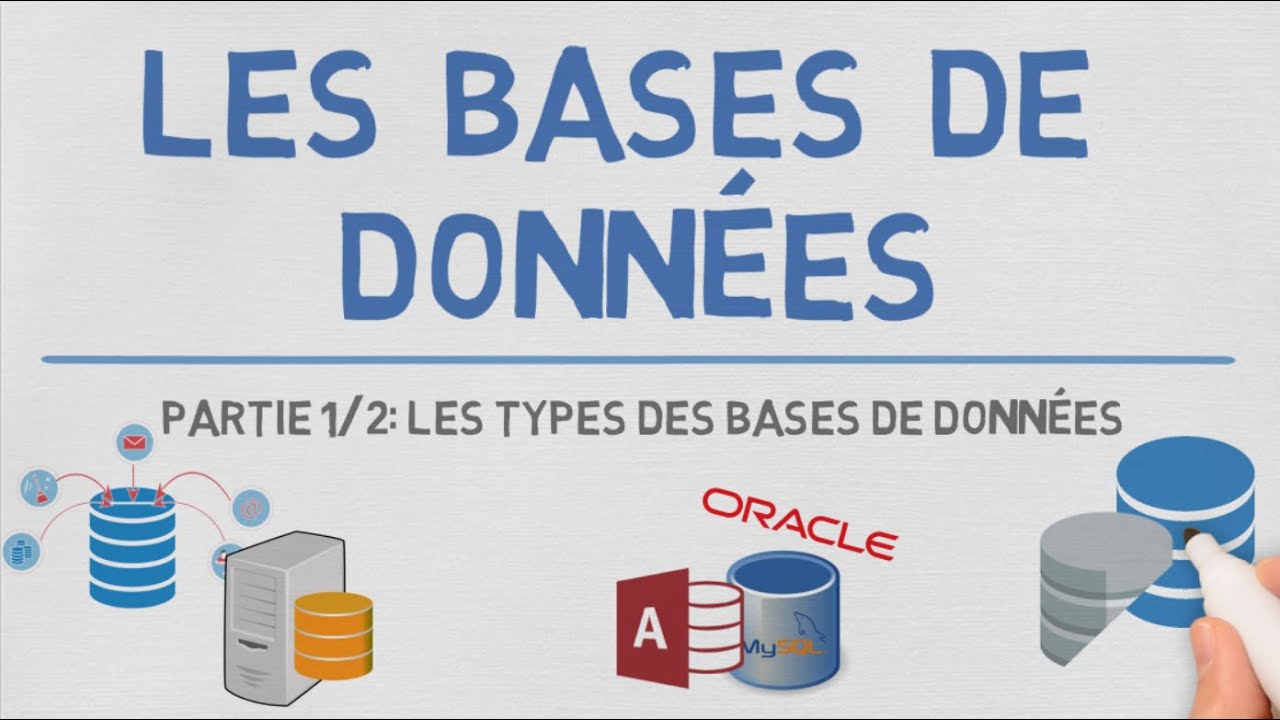
Les Bases de Données (1/2) - Les Bases de données Relationnelles

Database Programming Using Python
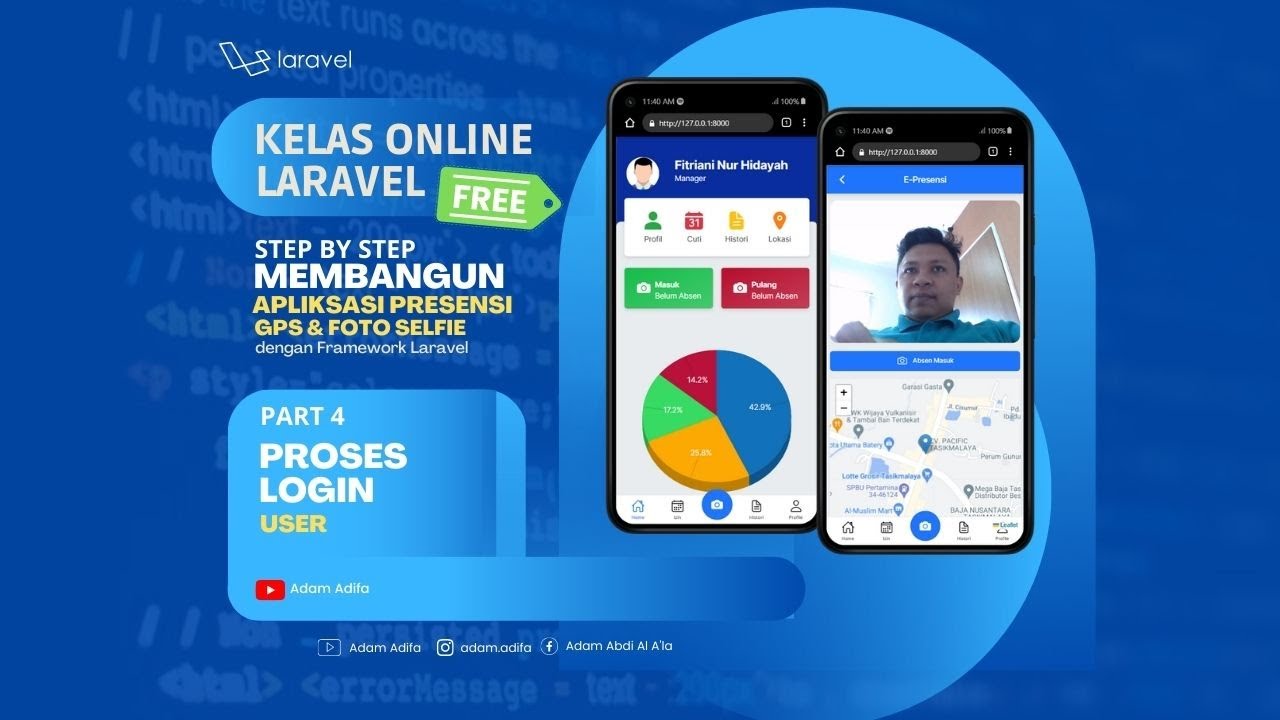
04 - Membuat Proses Login Untuk User
5.0 / 5 (0 votes)