Assembly Programming with RISC-V: Part 1
Summary
TLDRThis four-part series introduces the fundamentals of writing assembly for the RISC-V instruction set architecture (ISA). It covers essential topics like registers, memory organization, and the execution flow of programs from compilation to loading. Viewers will learn about calling conventions for procedure calls, memory operations using load and store instructions, and practical examples, including a function for swapping values. This comprehensive guide aims to provide a solid foundation for understanding RISC-V assembly programming and its applications in systems programming.
Takeaways
- 😀 The RISC-V instruction set architecture (ISA) acts as an interface between software and hardware, defining how to program the machine with instructions.
- 😀 Understanding the stateful elements, like the program counter and register file, is crucial for grasping how programs execute on RISC-V.
- 😀 RISC-V features 32 general-purpose registers (x0 to x31), with the x0 register hardwired to zero, meaning it always reads as zero and ignores writes.
- 😀 Memory is organized as an array of bytes divided into segments, such as text, data, stack, and heap, with little-endian byte order in RISC-V.
- 😀 Assembly instructions correspond directly to machine instructions, with a one-to-one mapping for readability and debugging purposes.
- 😀 Calling conventions dictate how arguments are passed and return values are managed, ensuring binary compatibility across different systems.
- 😀 Up to seven integer or pointer arguments can be passed through registers a0 to a7, while larger arguments are passed via the stack.
- 😀 Common data movement instructions include `lw` (load word) and `sw` (store word), which facilitate the transfer of data between registers and memory.
- 😀 Disassemblers, such as the GNU `objdump`, are essential tools for examining the machine code and understanding program structure.
- 😀 The assembly code can have multiple valid implementations for a given function, and understanding processor behavior can help optimize these implementations.
Q & A
What is the primary focus of the four-part series on RISC-V assembly?
-The series focuses on writing simple assembly for the RISC-V instruction set architecture (ISA), covering data movement, arithmetic and logic instructions, control flow, and procedures.
What foundational knowledge is assumed for understanding RISC-V assembly?
-A basic knowledge of number representation in binary and hexadecimal is assumed.
What are the key stateful elements a program interacts with in the RISC-V architecture?
-The key elements include the program counter, the register file (which contains general-purpose registers), and memory.
How does RISC-V handle endianness?
-RISC-V uses a little-endian byte order, meaning the least significant byte is stored at the lowest memory address.
What is the purpose of calling conventions in RISC-V?
-Calling conventions define how registers are used for procedure calls and returns, ensuring binary compatibility across different applications that follow the same Application Binary Interface (ABI).
How are integer or pointer arguments passed in RISC-V?
-Up to seven integer or pointer arguments are passed using registers a0 through a7; larger arguments are passed on the stack.
What tools can be used to analyze machine instructions in RISC-V?
-Tools like `objdump` from the GNU Compiler Collection can be used to disassemble machine instructions and display them in a readable format.
What are the mnemonics for loading and storing data in RISC-V?
-The mnemonic `lw` stands for load word, and `sw` stands for store word, used to transfer data between memory and registers.
What registers are specifically highlighted in the RISC-V architecture?
-The 32 general-purpose registers, labeled x0 through x31, are highlighted, with x0 being hardwired to zero.
What is the significance of the example function that swaps memory contents?
-The swap function demonstrates how to use assembly to manipulate memory contents through registers and highlights the impact of calling conventions on register usage.
Outlines
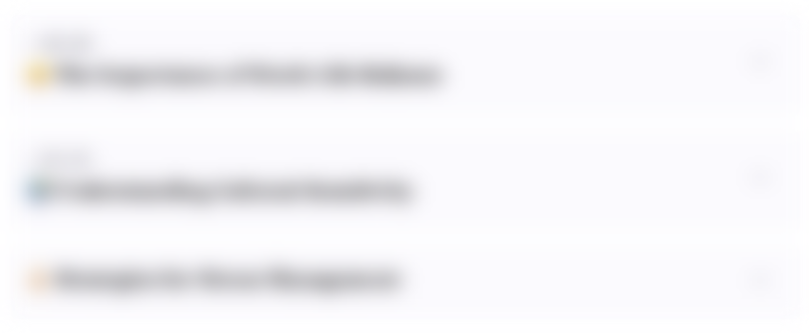
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
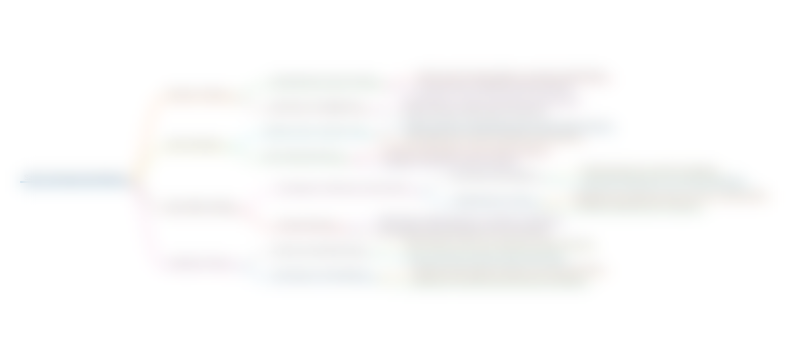
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
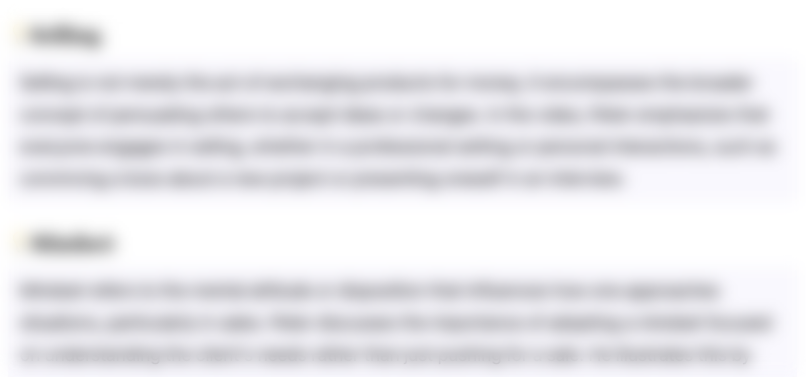
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
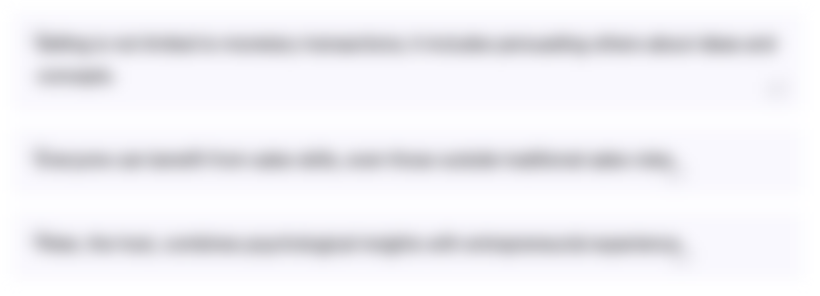
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
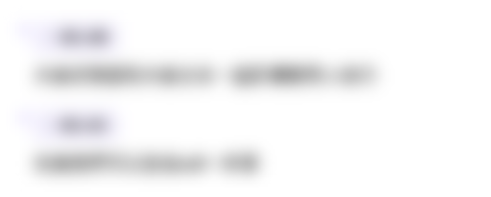
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示

[CS61C FA20] Lecture 07.3 - RISC-V Intro: RISC-V add/sub Instructions
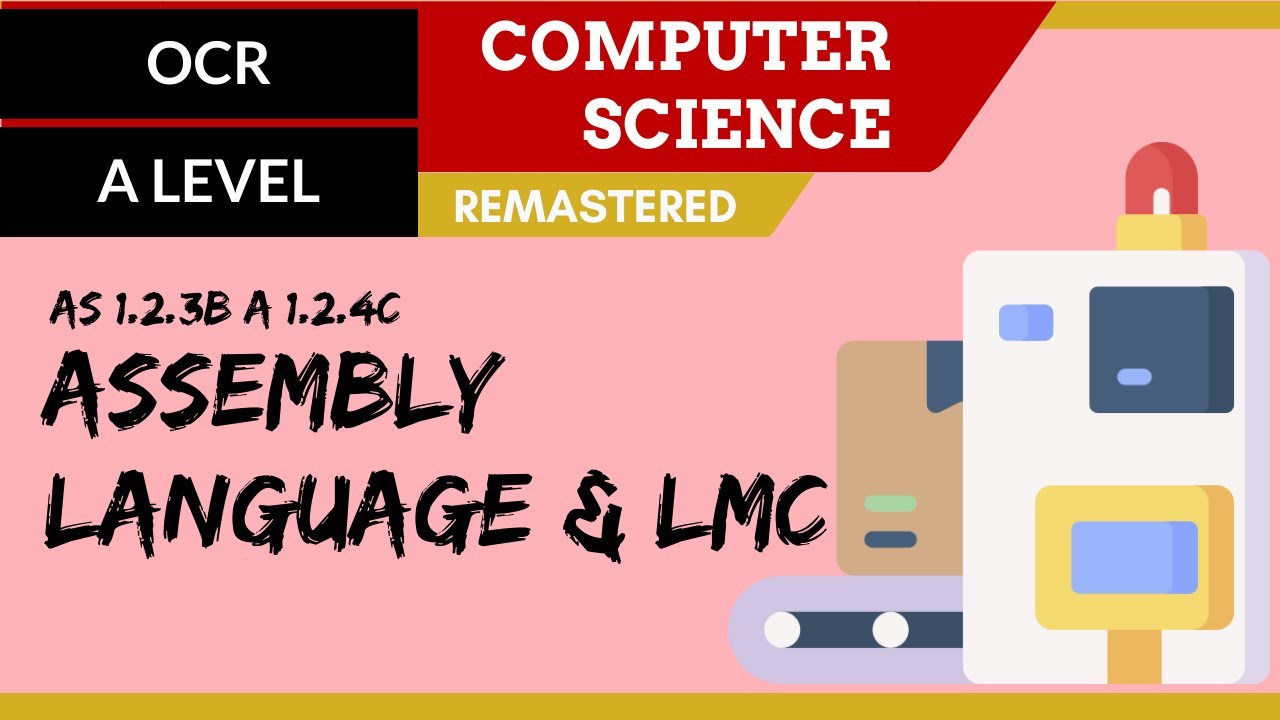
34. OCR A Level (H046-H446) SLR7 - 1.2 Assembly language and LMC language
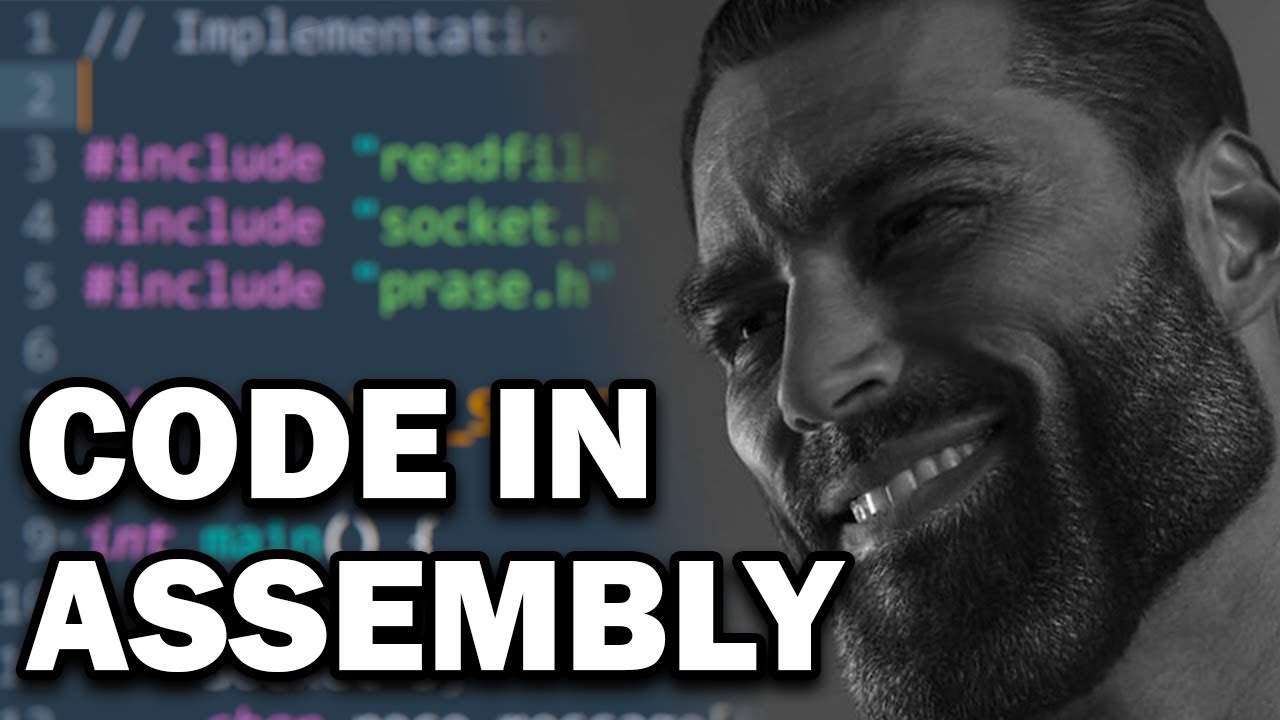
you can become a GIGACHAD assembly programmer in 10 minutes (try it RIGHT NOW)
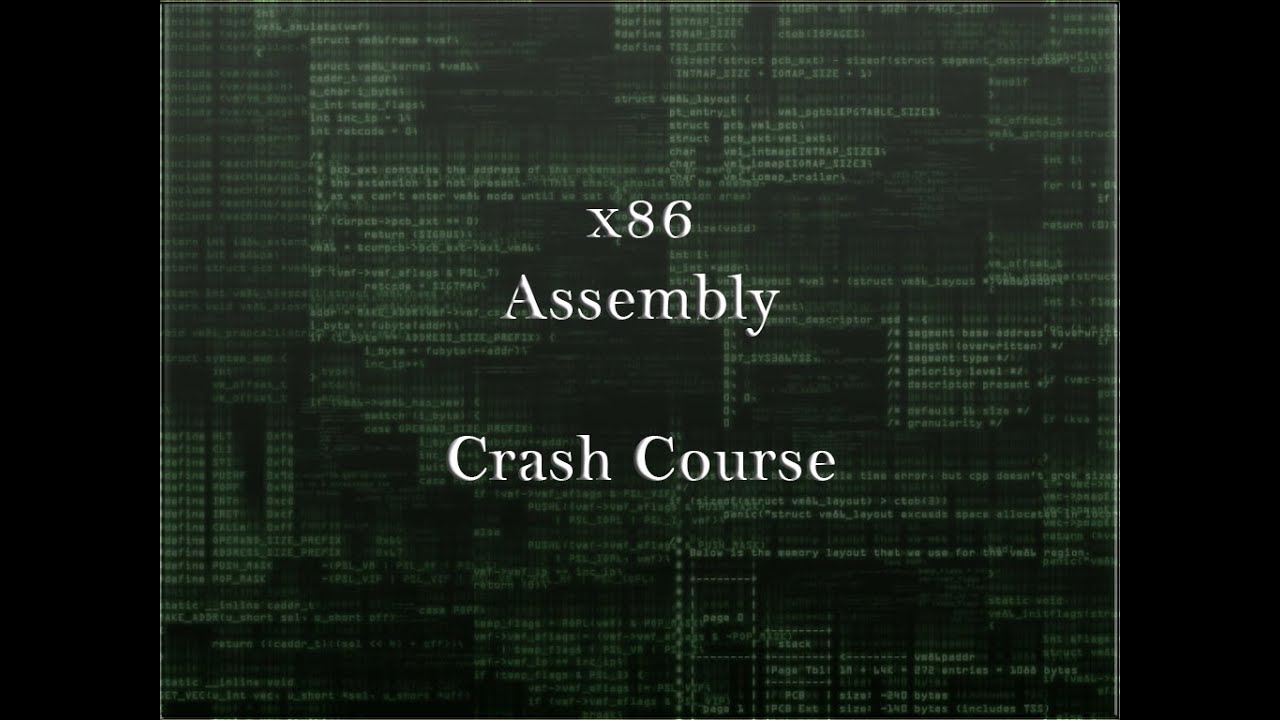
x86 Assembly Crash Course
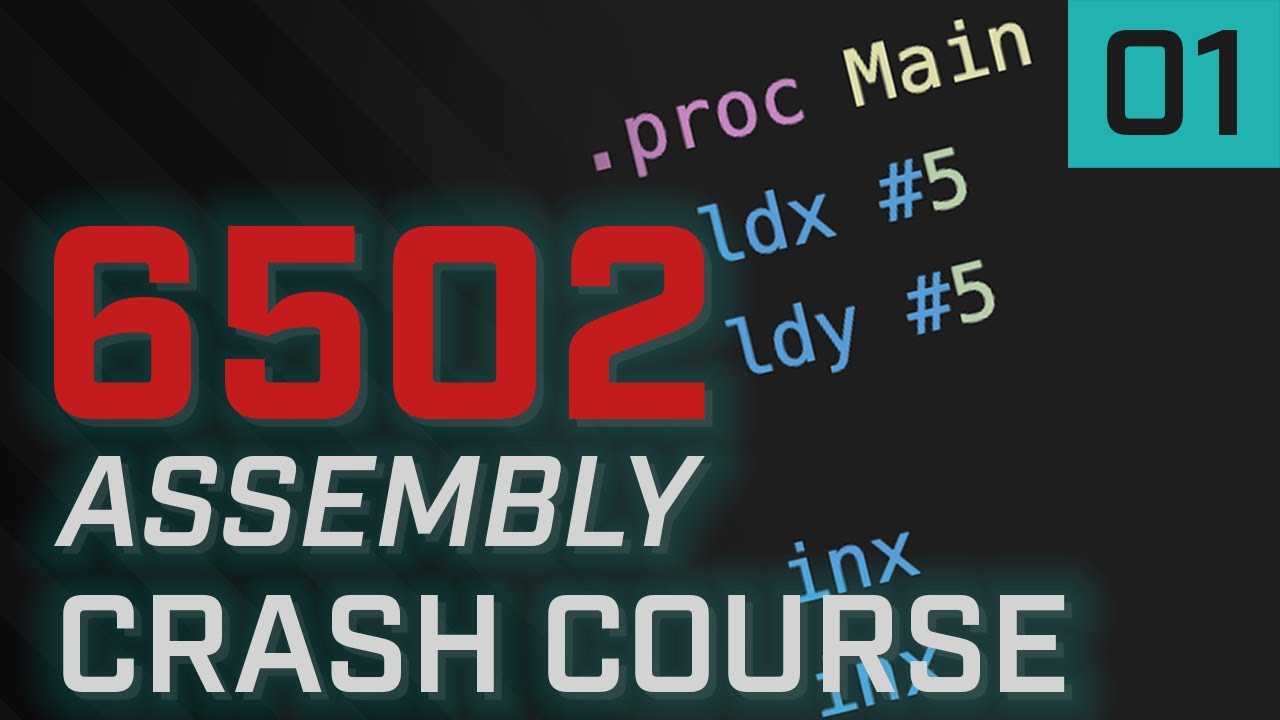
Basics - 6502 Assembly Crash Course 01

RISC vs CISC | RISC | Reduced Instruction Set Computer | CISC | Complex Instruction Set Computer
5.0 / 5 (0 votes)