Dealing cards with functions | Intro to CS - Python | Khan Academy
Summary
TLDRThe script outlines the design of a Python program for playing various card games. The program includes functions to draw random cards and hands, with cards represented as strings like 'Queen of Hearts.' The author introduces modular functions such as drawing suits, ranks, and handling hands of different sizes. There is also flexibility for games to enable or disable face cards. The code uses randomness for shuffling, but doesn’t model a strict deck, which could be a limitation depending on the game's rules, especially in cases where duplicate cards aren’t allowed.
Takeaways
- 🃏 The program focuses on building car games where multiple games share functionality for dealing a deck of cards.
- 🎴 A `draw_card` function is needed to return a random playing card, which is represented as a string (e.g., 'Queen of Hearts').
- 🖐️ The `draw_hand` function is designed to draw a variable number of cards, and formats the result with a newline for better readability.
- 🎲 The `draw_card` function is broken into two subtasks: generating a random suit and a random rank.
- ♥️ The `get_suit` function randomly selects one of the four suits (Hearts, Diamonds, Spades, Clubs).
- 🔢 The `get_rank` function randomly selects from 13 possible ranks (Ace, 2–10, Jack, Queen, King), with some ranks returned as strings and numbers cast to strings.
- ⚠️ Consistency is important: ensure that `get_rank` always returns a string to avoid type errors when combining strings and integers.
- 🃑 A Boolean parameter is added to `get_rank` to optionally exclude face cards (Ace, Jack, Queen, King) when needed.
- 🎮 This Boolean parameter is passed through all relevant functions (`draw_card`, `draw_hand`) to control whether face cards are included in the game.
- ♠️ The limitation is that the deck isn't exhaustive—cards can repeat, which is a feature for some games but a limitation for others that require strict deck rules.
Q & A
What is the main goal of the program described in the script?
-The main goal of the program is to allow users to play various car games that share a common functionality for drawing and dealing cards.
How are playing cards represented in this program?
-Playing cards are represented as strings, with a format like 'Queen of Hearts' where the card's rank and suit are combined.
What is the purpose of the 'draw_card' function?
-The 'draw_card' function's purpose is to randomly select a card from a deck by choosing both a random suit and a random rank.
How does the 'draw_hand' function work?
-The 'draw_hand' function takes the number of cards as a parameter, draws that many cards, and returns them as a formatted string where each card is placed on a separate line.
What functions are used within 'draw_card' to get the suit and rank of a card?
-Two helper functions, 'get_suit' and 'get_rank', are used within 'draw_card' to randomly generate the card’s suit and rank respectively.
How does the 'get_suit' function decide which suit to return?
-The 'get_suit' function generates a random number between 1 and 4, and based on that number, it returns one of the four suits: Hearts, Diamonds, Spades, or Clubs.
How does the 'get_rank' function generate the rank of a card?
-The 'get_rank' function generates a number between 1 and 13, where 1 corresponds to Ace, 11 to Jack, 12 to Queen, and 13 to King. For numbers between 2 and 10, the function returns the number as a string.
What problem arises if 'get_rank' returns a number instead of a string?
-If 'get_rank' returns a number instead of a string, it causes a type error because the program tries to concatenate an integer and a string when constructing the card representation.
How does the program handle games that don't use face cards?
-The program adds a Boolean parameter to 'get_rank' that, when set to false, ensures only numbers between 2 and 10 are returned, excluding face cards (Ace, Jack, Queen, King).
What limitation is acknowledged regarding the deck in this program?
-The limitation is that the deck is not unique, meaning it’s possible to draw the same card multiple times, like two Ace of Spades. This is acceptable for games with multiple decks but may be a bug for games that require only one of each card.
Outlines
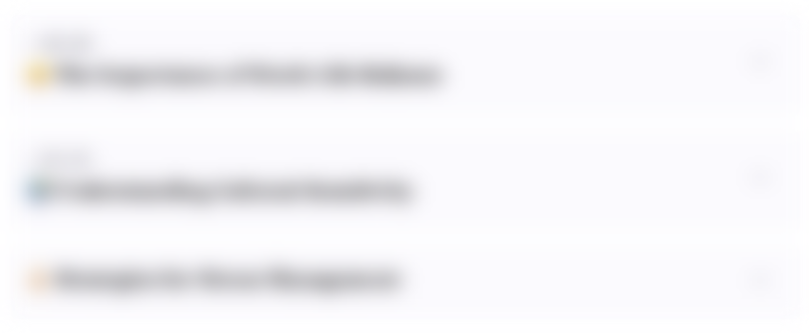
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
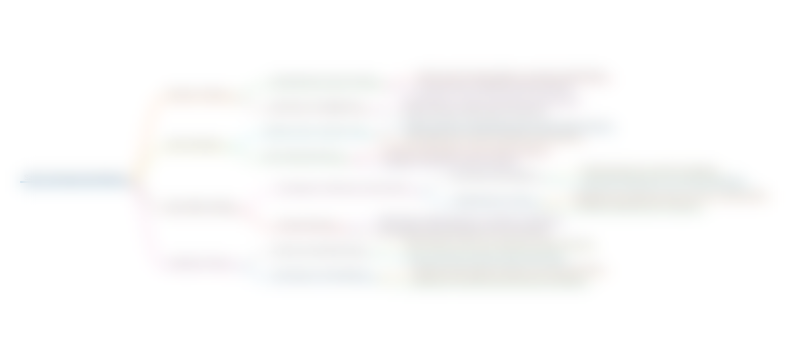
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
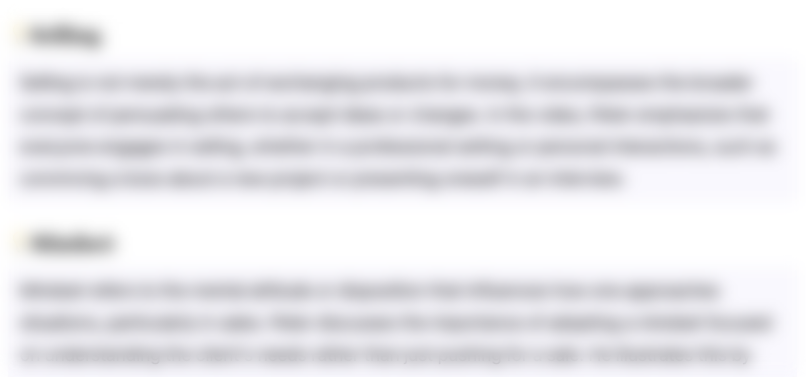
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
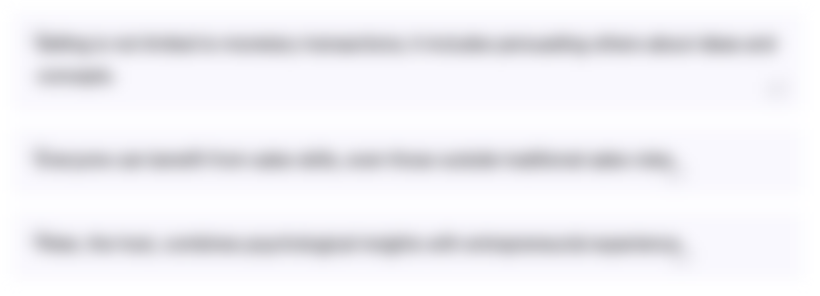
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
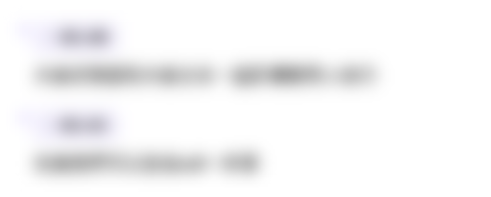
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
5.0 / 5 (0 votes)