#03 Editing the First Angular Project | Getting Started with Angular | A Complete Angular Course
Summary
TLDRIn this lecture, the instructor demonstrates how to create, run, and edit a basic Angular project. The process starts by running the Angular application on localhost (port 4200) using the 'ng serve' command. They then explain how to navigate through the project folder, open it in VS Code, and identify the source of the application's HTML rendering. The lecture covers the role of Angular components, data binding, and introduces dynamic content generation using Angular’s features. This foundational setup prepares for deeper exploration in upcoming lectures.
Takeaways
- 👨💻 The Angular project is set up and running on localhost port 4200 after using the 'ng serve' command.
- 🛑 Pressing Ctrl + C will terminate the Angular process, making the app unavailable at localhost 4200 until 'ng serve' is run again.
- 🗂 VS Code is recommended as the code editor for the course, though other editors like Atom or Sublime can also be used.
- 📂 To edit the project in VS Code, navigate to the project folder and open it through the editor.
- 💻 The default UI is loaded from the 'index.html' file when running the Angular app.
- 📜 When 'ng serve' is executed, it injects five scripts (runtime.js, polyfills.js, styles.js, vendor.js, and main.js) into 'index.html'.
- 📝 The 'approot' is a custom Angular component tag that loads the 'app.component.html' template when used in the HTML.
- 🎨 The 'app.component.ts' file is the core file where the class and logic of the app component are defined, which ties the HTML and TypeScript together.
- 🔗 Dynamic content in Angular can be generated using data binding with double curly braces, allowing for dynamic updates in the UI.
- 🔄 Angular enables single-page applications, meaning only one 'index.html' file is loaded, and the content changes dynamically without reloading the entire page.
Q & A
What is the purpose of the NG serve command in an Angular project?
-The NG serve command compiles the Angular project, creates necessary bundles, and starts a development server. This allows the project to be accessible at a URL, typically localhost:4200, while the process is running.
What happens when the process started by NG serve is killed?
-When the NG serve process is killed, the Angular application can no longer be accessed at localhost:4200 because the development server stops running. The project must be restarted with NG serve to make it accessible again.
How do you open an Angular project in Visual Studio Code?
-To open an Angular project in Visual Studio Code, go to 'File' > 'Open Folder,' navigate to the project folder, and select it. The project files will then be available for editing within VS Code.
Which file in an Angular project defines the main HTML structure that gets rendered initially?
-The `index.html` file in the `src` folder defines the main HTML structure that gets rendered when an Angular project is loaded in the browser.
What is the role of the app-root element in an Angular application?
-The `app-root` element serves as a selector for the root component in the Angular application, typically rendering the content defined in the `app.component.html` file.
What is the purpose of the app.component.ts file in an Angular project?
-The `app.component.ts` file contains the logic for the root component of the Angular application. It includes a class (e.g., AppComponent) that is decorated with the `@Component` decorator, defining the selector and the template for the component.
How does Angular generate dynamic content in the HTML?
-Angular generates dynamic content using data binding. For example, by using double curly braces (`{{}}`), you can bind a component's property (like `title`) to the HTML, dynamically rendering the property's value in the UI.
What is the difference between static and dynamic content in Angular?
-Static content is hardcoded in the HTML and does not change, whereas dynamic content is generated based on component properties and can change depending on user interaction or data updates.
Why does Angular use TypeScript instead of JavaScript, and how is it executed in the browser?
-Angular uses TypeScript because it provides better type checking and object-oriented programming features. However, TypeScript is compiled into JavaScript, which is then executed by the browser.
What is the importance of the scripts injected into the index.html file in an Angular application?
-The scripts injected into the `index.html` file (like `runtime.js`, `polyfills.js`, `vendor.js`, etc.) are essential for running the Angular application. They include necessary bundles and dependencies compiled by the NG serve command.
Outlines
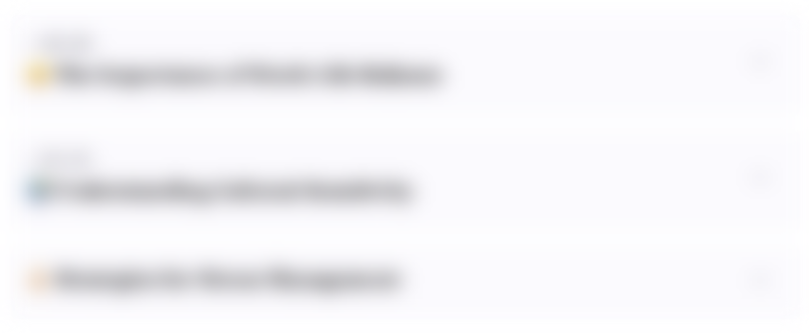
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
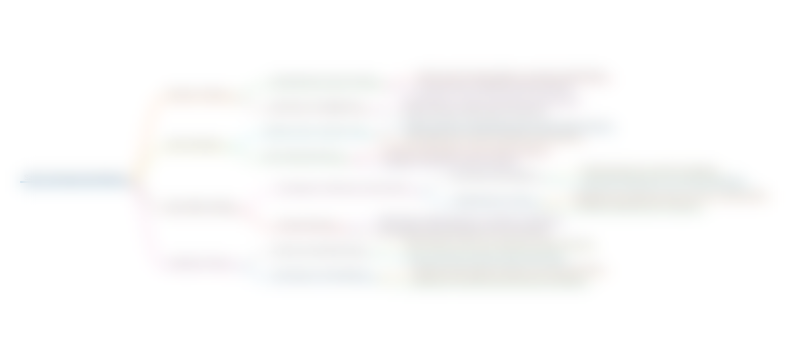
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
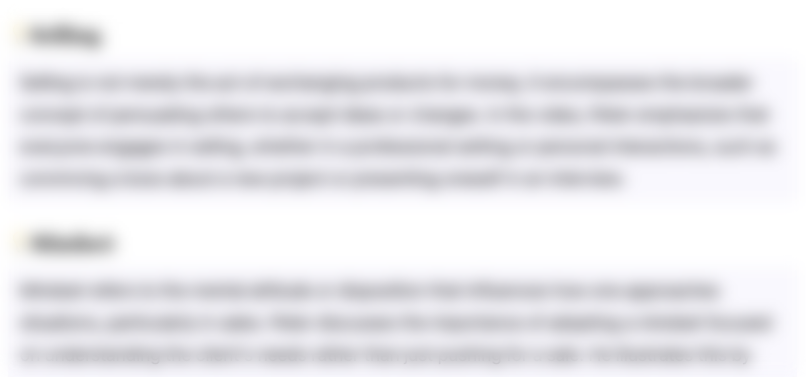
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
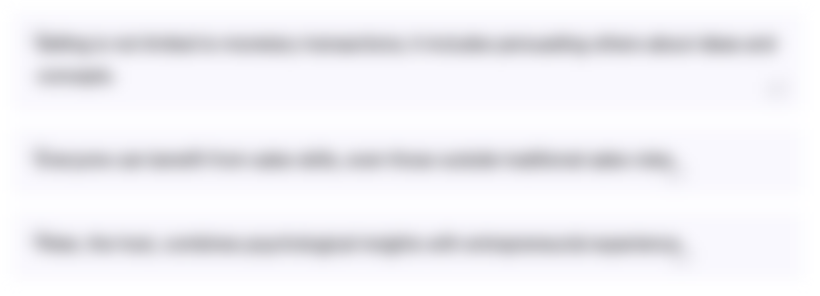
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
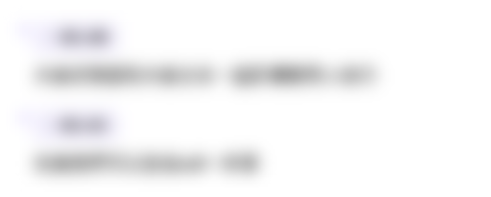
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
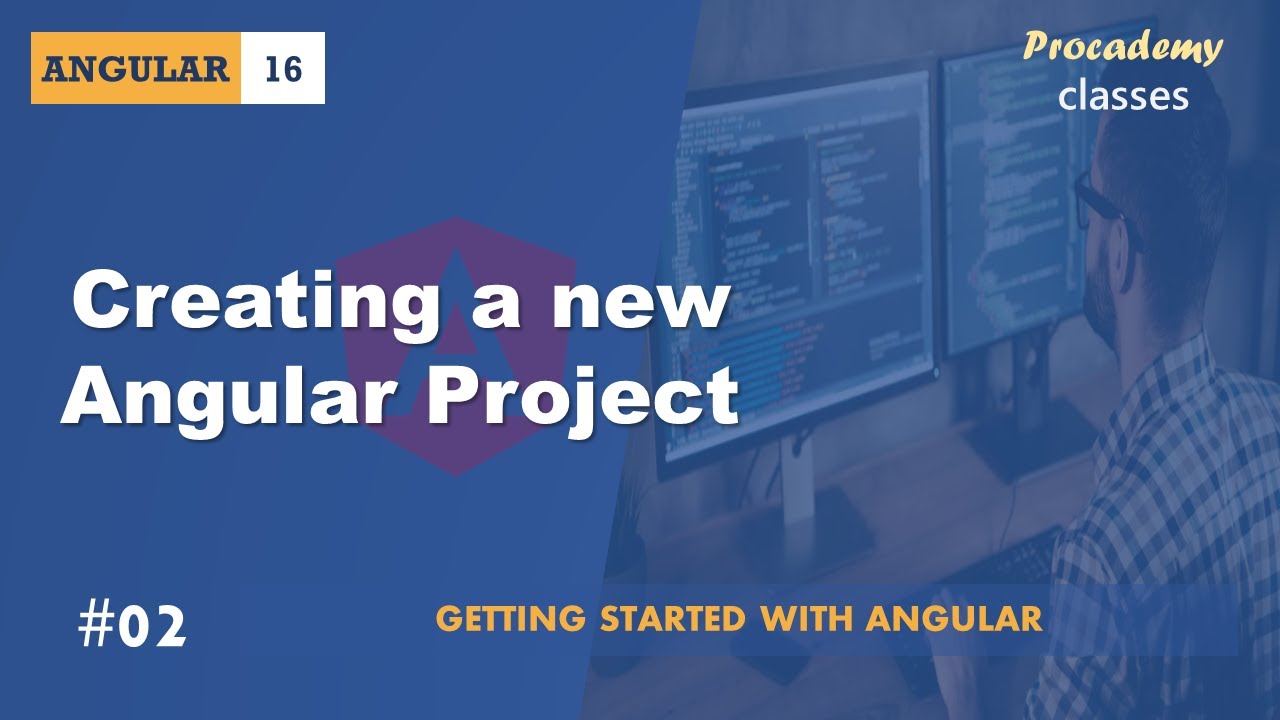
#02 Creating a new Angular Project | Getting Started with Angular | A Complete Angular Course
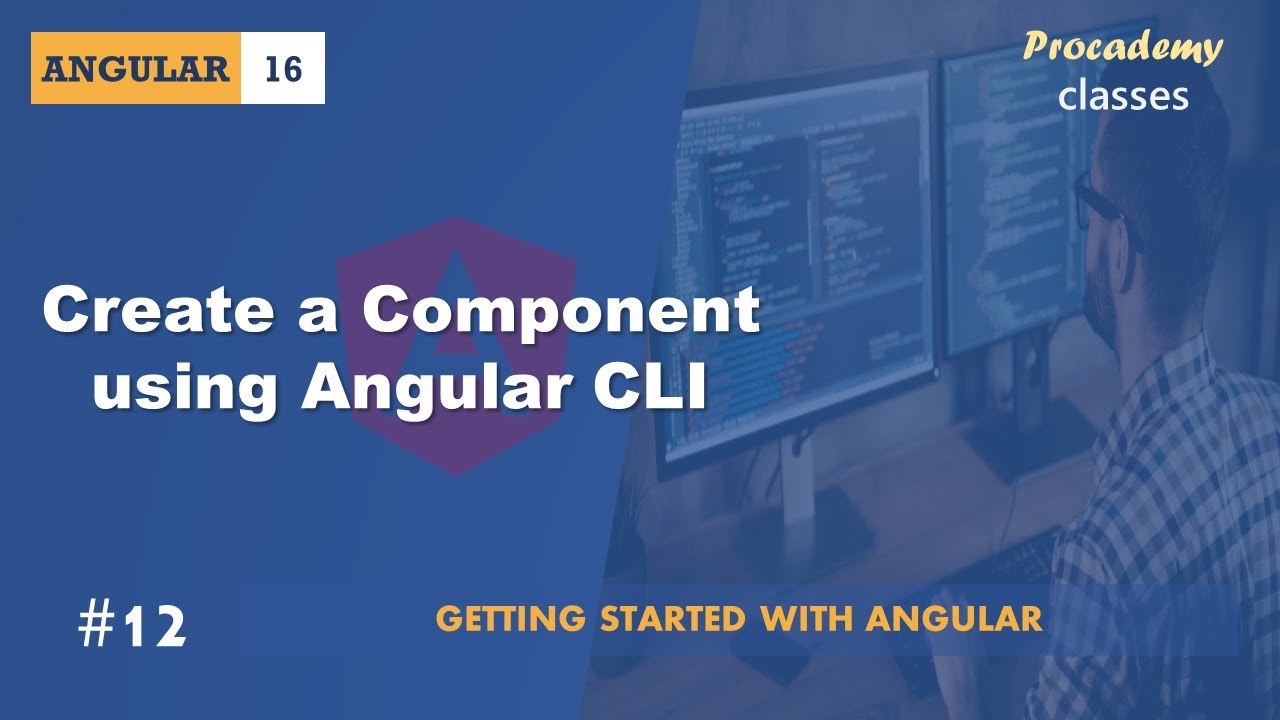
#12 Create Component using Angular CLI | Angular Components & Directives | A Complete Angular Course
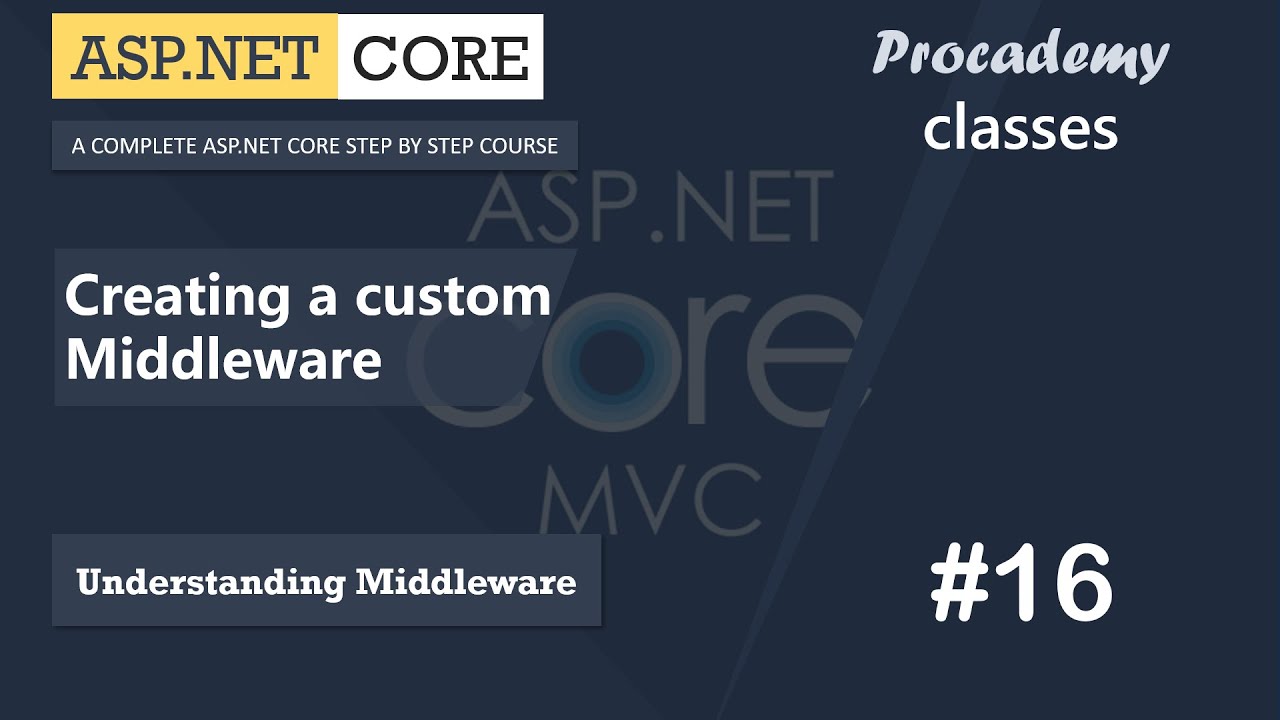
#16 Creating a Custom Middleware | Understanding Middleware | ASP.NET Core MVC Course
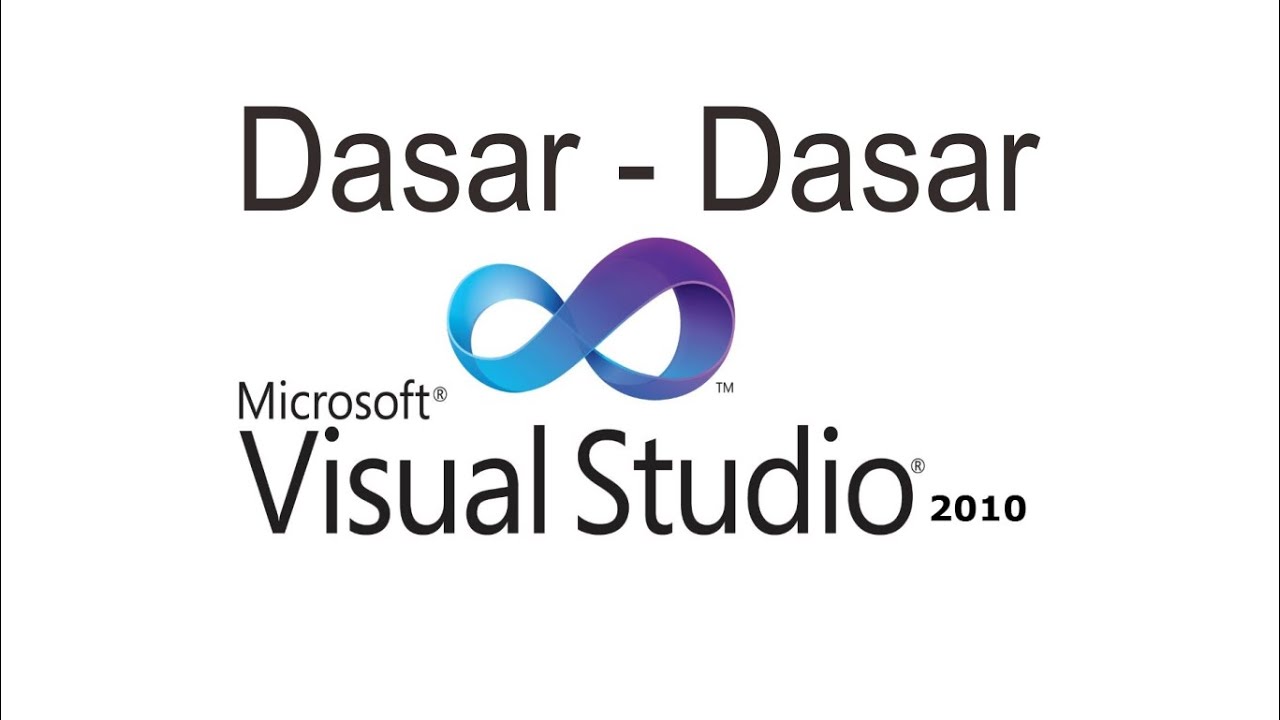
Belajar VB.NET - Dasar Visual Studio 2010
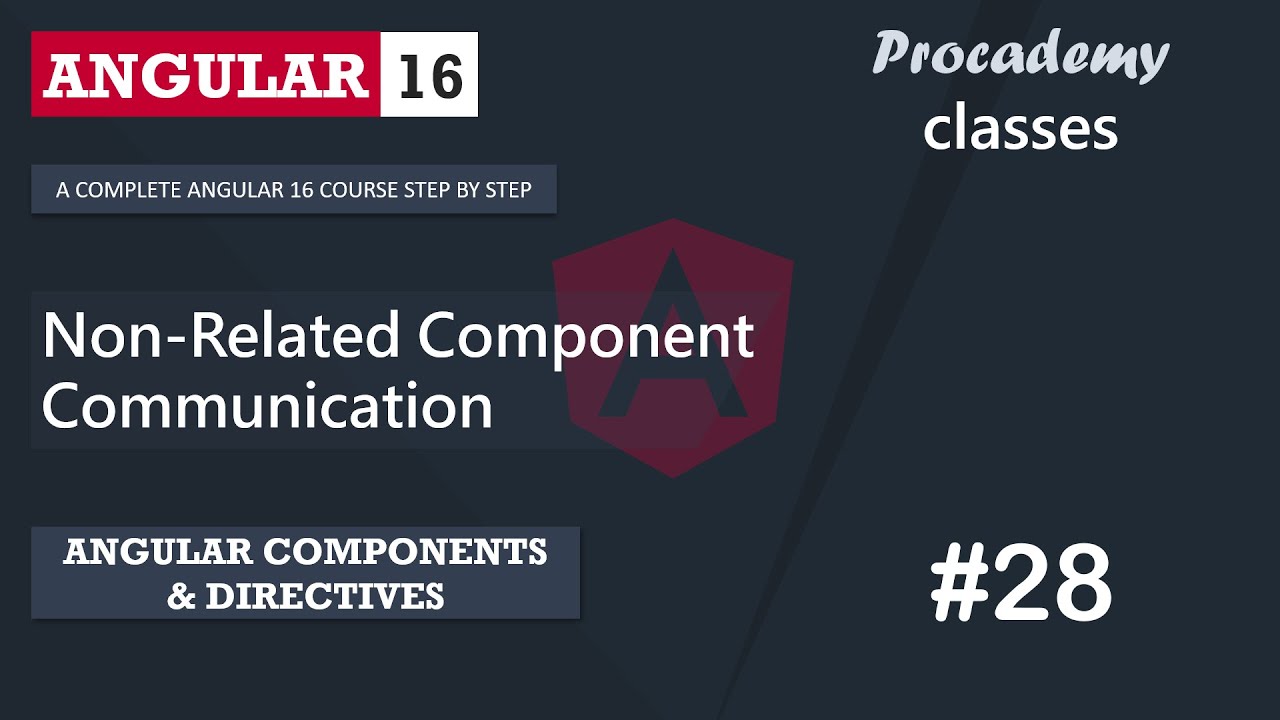
#28 Non-Related Component Communication | Angular Component & Directives | A Complete Angular Course
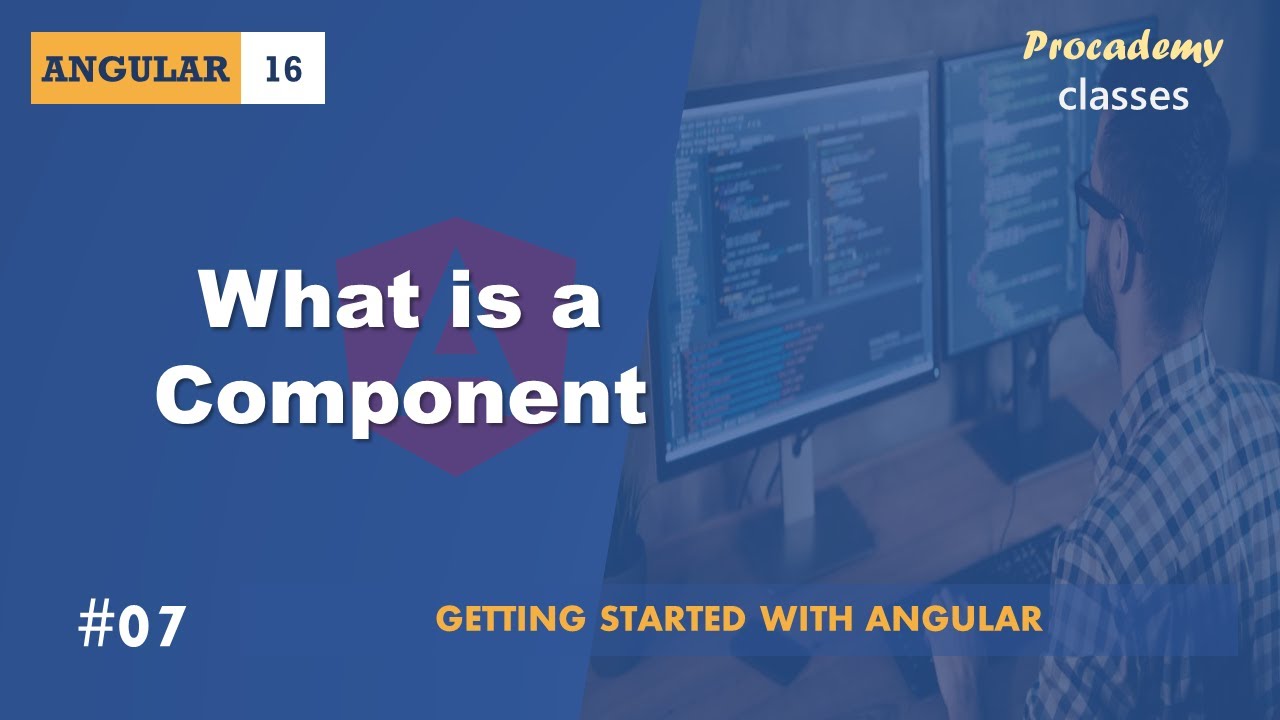
#07 What is a Component | Angular Components & Directives| A Complete Angular Course
5.0 / 5 (0 votes)