Express JS #4 - Query Parameters
Summary
TLDRThis video script explains the use of query strings and parameters in backend development. It covers how they appear in a URL, their role in sending data across pages, and manipulating server-side data retrieval. The tutorial demonstrates filtering and sorting user data based on query parameters, using JavaScript's array filter method. It also shows how to handle cases where query parameters are incomplete.
Takeaways
- 🔍 Query strings are used to send data at the end of a URL, following a '?' symbol.
- 🔑 Query parameters are key-value pairs that can be added after the '?' in a URL, separated by '&'.
- 🌐 They are commonly used in web development to pass data between pages or to add additional data to HTTP requests.
- 📄 The query string is parsed into a JSON object by Express, making it easy to access the values.
- 🔄 Query parameters can be used to sort or filter data on the server side before sending it back to the client.
- 📝 In a GET request, query parameters are used to specify how data should be manipulated or filtered without altering the data itself on the server.
- 🛠️ Developers can use query parameters to implement features like searching or filtering within arrays of data, such as user objects.
- 📊 Filtering can be done based on substrings within specific fields of an object, like 'username' or 'display name'.
- 📈 The script demonstrates how to handle query parameters in a Node.js/Express application, including checking for their existence and applying filters.
- 💻 It's important to ensure that both the filter type and value are provided in the query parameters for the filtering to work correctly.
Q & A
What is a query string?
-A query string is a part of a URL that appears after a question mark (?) and contains key-value pairs that are used to send data to a web server.
How are query parameters used in backend development?
-Query parameters are used in backend development to send data from the client to the server, filter and sort data, and manipulate data retrieval from databases based on specific criteria.
What is the purpose of the equals sign '=' in a query string?
-The equals sign '=' in a query string is used to assign a value to a key, similar to assigning a value to a variable, but in the context of a URL.
How can you pass multiple key-value pairs in a query string?
-Multiple key-value pairs in a query string can be passed by separating each pair with an ampersand (&) symbol.
How does a query string differ from a request body?
-A query string is part of the URL and is used to send data to the server without changing the state of the server, while a request body is used in POST requests to send data to the server that may change its state.
What is the role of the request.query object in Express.js?
-The request.query object in Express.js is used to access the query parameters sent in the query string of the URL.
Can you provide an example of how to filter an array based on a query parameter in Express.js?
-Yes, you can filter an array based on a query parameter by accessing the request.query object, destructuring the specific query parameter, and using it as a condition in the array's filter method.
What is the significance of the includes method in filtering query parameters?
-The includes method is used to check if a string contains a specific substring, which is useful for filtering data based on partial matches of strings in query parameters.
How do you handle cases where only one of the query parameters (filter or value) is provided?
-You should handle cases where only one query parameter is provided by checking if both parameters exist and, if not, returning the original data without applying any filters.
What is the purpose of the filter function in JavaScript when dealing with query parameters?
-The filter function in JavaScript is used to create a new array with all elements that pass the test implemented by the provided function, which can be used to filter data based on query parameters.
How can query parameters be used to sort data retrieved from a server?
-Query parameters can be used to specify sorting criteria such as sorting by a specific field or in a specific order, which the server can then use to sort the data before sending it back to the client.
Outlines
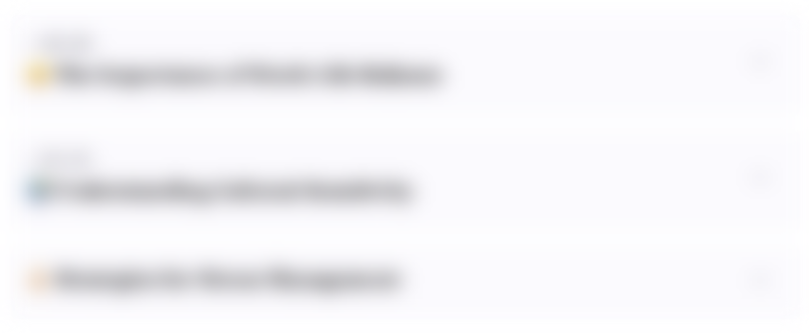
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
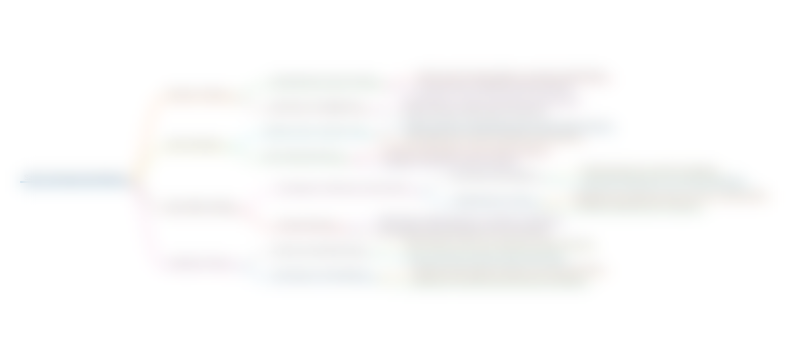
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
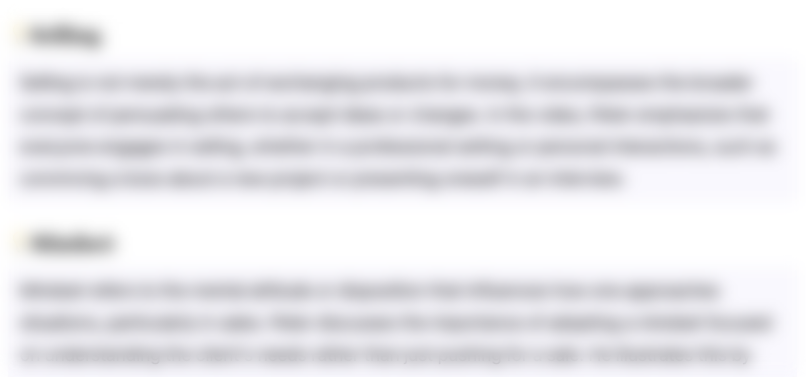
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
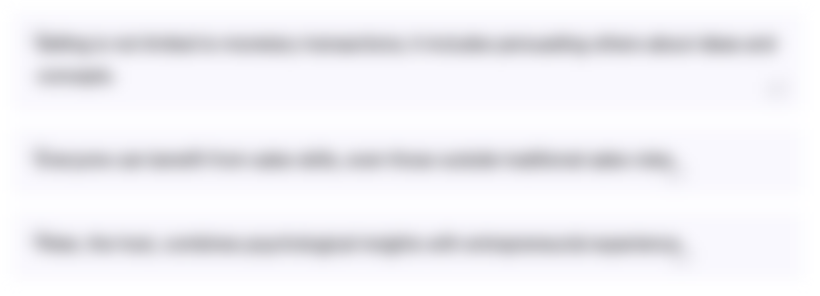
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
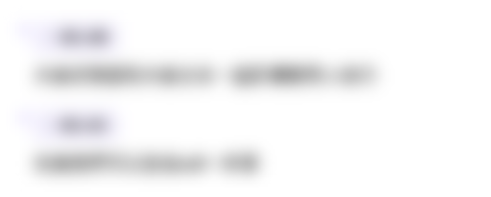
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
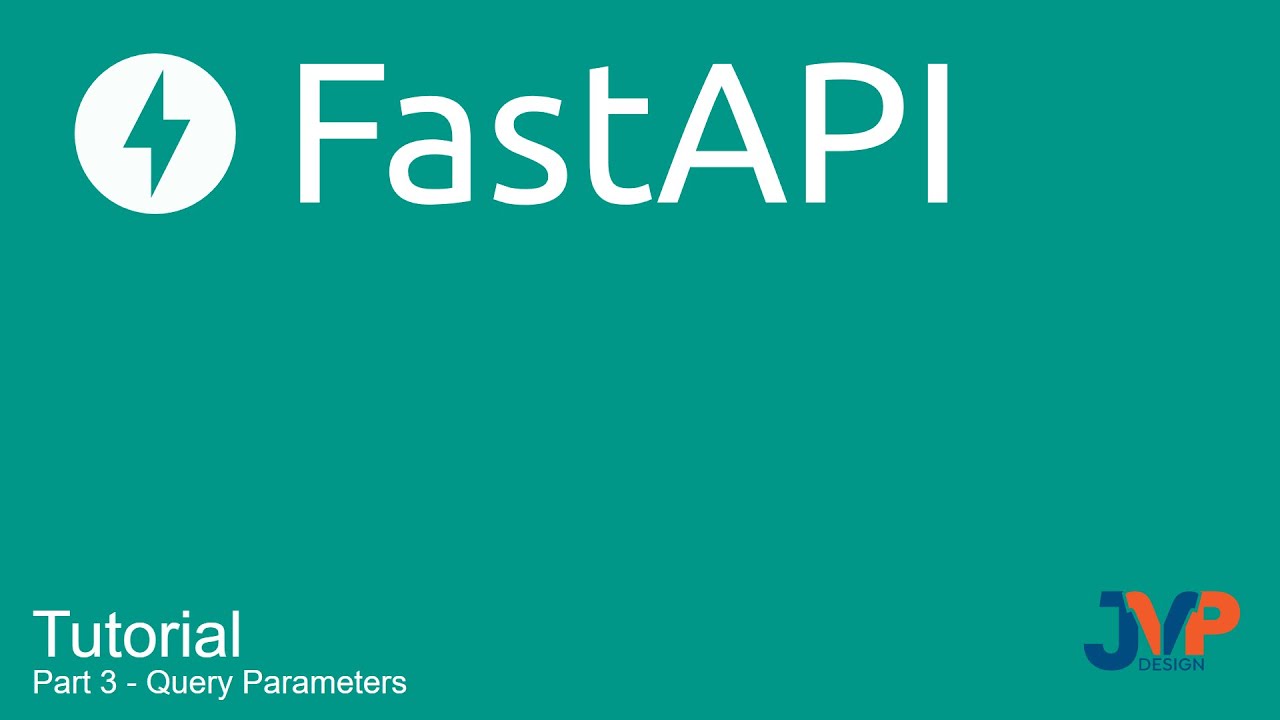
Fast API Tutorial, Part 3: Query Parameters
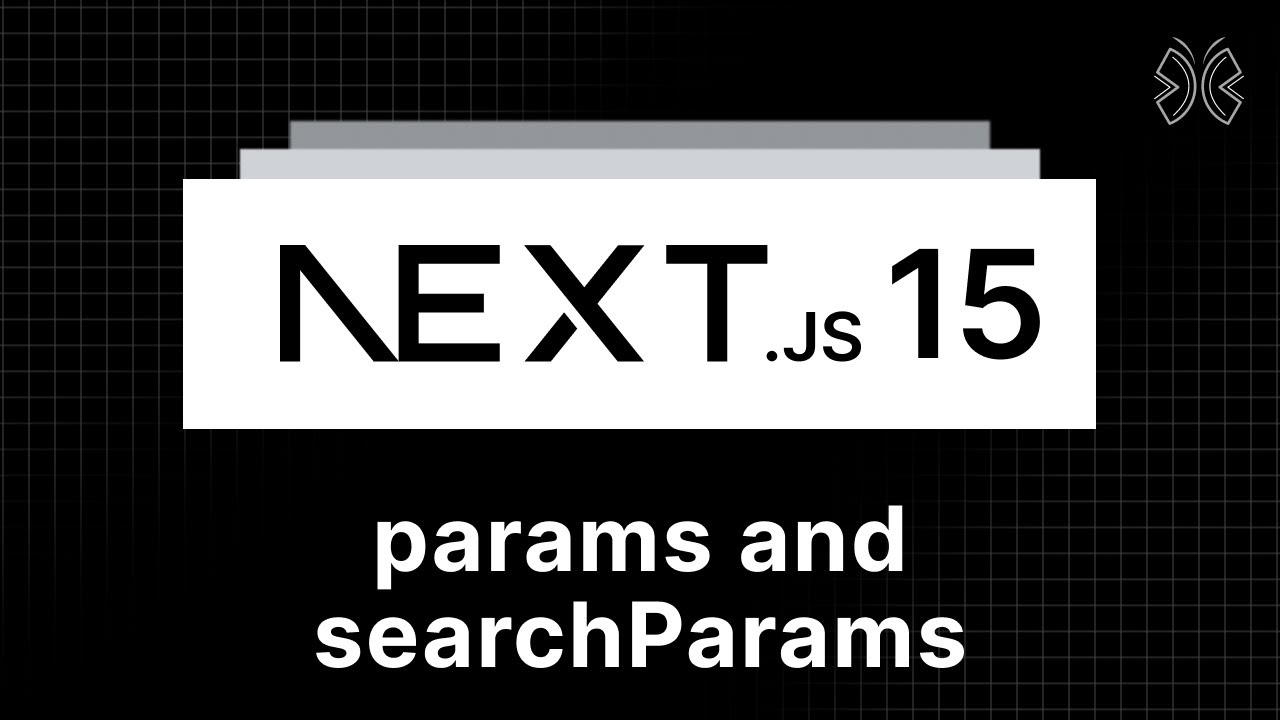
Next.js 15 Tutorial - 21 - params and searchParams

C++ Strings | What is String? full Explanation

Why I don't use React-Query and tRPC anymore
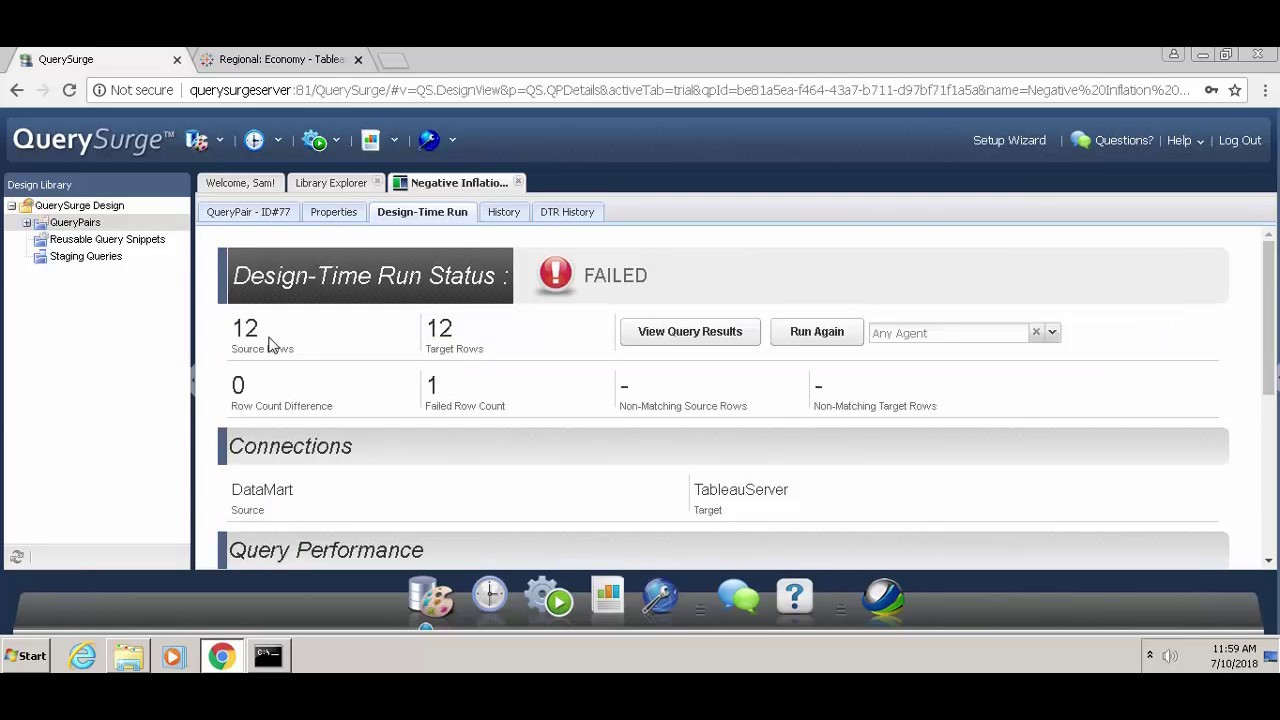
Comparing a Tableau report to a database using QuerySurge

#11 What is Routing | Fundamentals of NODE JS | A Complete NODE JS Course
5.0 / 5 (0 votes)