C# Async/Await/Task Explained (Deep Dive)
Summary
TLDRThis video script offers an in-depth exploration of asynchronous programming in C#, focusing on the concepts behind async and await rather than just their implementation. The presenter uses the analogy of making tea to explain how async operations work, highlighting the difference between synchronous and asynchronous tasks. The script delves into the technical aspects, such as the role of the task, the state machine created by async, and the checkpoints introduced by await. It aims to clarify the underlying mechanisms that allow for non-blocking code execution, making it easier for viewers to understand and utilize async/await effectively in their C# applications.
Takeaways
- 😀 The presenter aims to clarify the concepts behind async/await in C# rather than just showing implementation.
- 🔍 The video uses the analogy of making tea to explain asynchronous programming, emphasizing non-blocking operations.
- 💡 It's highlighted that async/await allows for multitasking by freeing up the thread to do other work while waiting for external tasks to complete.
- 🛠️ The script explains that once a task hits an await, it yields control back to the thread pool, allowing other tasks to run.
- 🔄 The concept of a 'task' is introduced as a bridge between the state machine and the await checkpoints.
- 📚 The async keyword is described as spawning a state machine, which is a key component of asynchronous programming.
- 🔑 The await keyword is likened to checkpoints within the state machine, controlling the flow of execution.
- 🖥️ The script provides insight into how the .NET runtime manages threads, emphasizing the role of the thread pool.
- 🌐 It discusses how network operations are handled asynchronously, involving the operating system and network drivers.
- 🔍 The presenter uses ILSpy to demonstrate how async methods are translated into a state machine by the compiler.
Q & A
What is the main focus of the video?
-The main focus of the video is to explain the underlying concepts behind async and await in C#, rather than just the implementation.
Why does the presenter believe that understanding async/await can help with sleepless nights?
-Understanding async/await can fill gaps in knowledge that might cause confusion or keep one awake at night, implying that it's a concept that can be puzzling if not fully grasped.
What analogy does the presenter use to explain async/await?
-The presenter uses the analogy of making a cup of tea to explain async/await, where boiling water is a synchronous task and preparing the tea while the water is boiling is an asynchronous task.
How does the presenter describe the difference between synchronous and asynchronous operations?
-The presenter describes synchronous operations as tasks that are completed by the same thread without waiting for external processes, while asynchronous operations allow the thread to be free to do other tasks while waiting for an external process to complete.
What is a 'task' in the context of the video?
-In the context of the video, a 'task' is an object that represents an asynchronous operation and is the bridge between the state machine created by async and the await keyword.
What is a 'state machine' as explained in the video?
-A 'state machine' in the video refers to the object that is created when a method is made asynchronous. It holds the state of the method across await calls.
What is the role of the 'await' keyword in asynchronous programming?
-The 'await' keyword is used to pause the execution of the current method until the awaited task is completed, allowing other operations to run in the meantime.
How does the presenter demonstrate that a task can be completed by a different thread?
-The presenter demonstrates that a task can be completed by a different thread by creating a long loop that runs in parallel while an asynchronous task is awaited, showing that the task can finish on a different thread while the loop is still running.
What is the purpose of the thread pool as explained in the video?
-The thread pool manages the execution of tasks by distributing them across available threads, allowing for efficient use of system resources and preventing thread overload.
How does the presenter explain the concept of a state machine checkpoint?
-The presenter explains that a state machine checkpoint is created by the await keyword, which divides the asynchronous method into parts that can be executed sequentially as different states of the state machine.
What does the presenter suggest is the key to understanding asynchronous programming?
-The presenter suggests that understanding the task as a bridge between the state machine and the code, the async keyword as what spawns the state machine, and the await keyword as defining the checkpoints within the state machine is key to understanding asynchronous programming.
Outlines
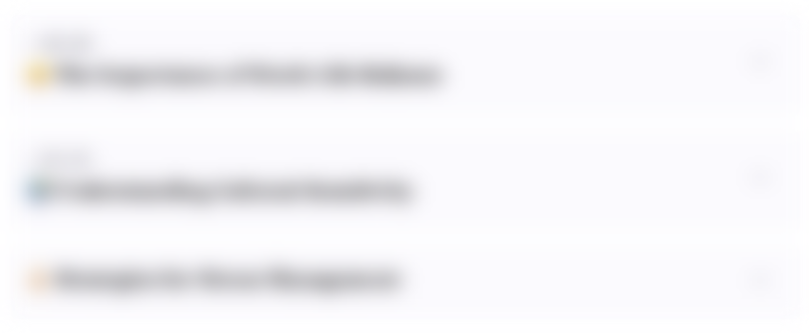
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
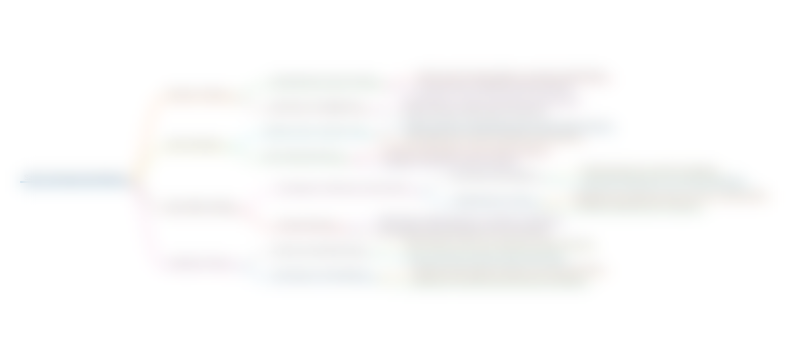
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
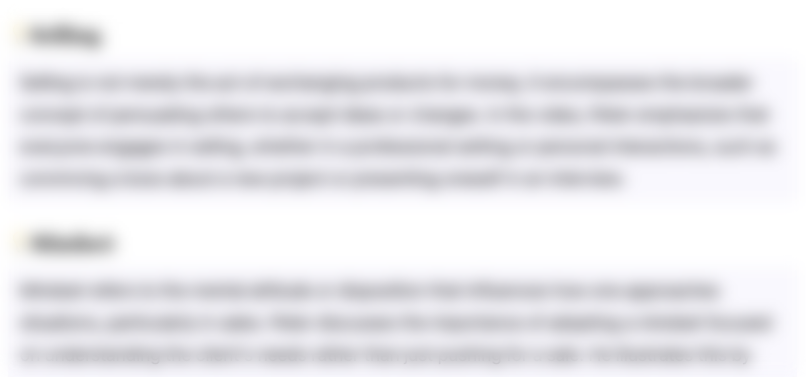
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
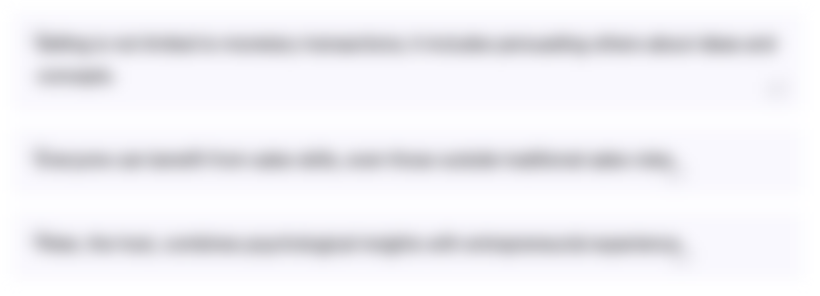
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
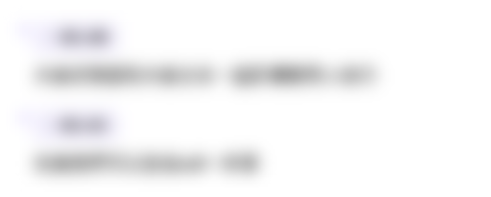
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
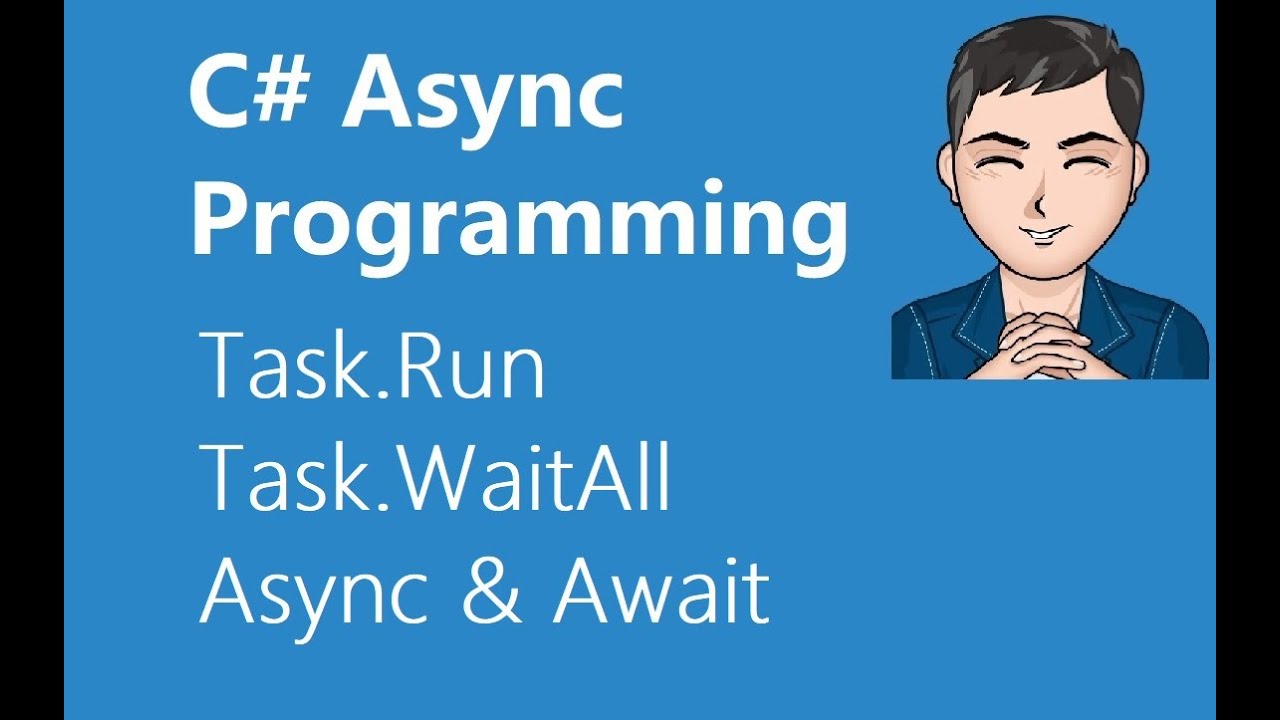
Asynchronous Programming in C# Explained (Task.Run, Task.WaitAll, Async and Await)
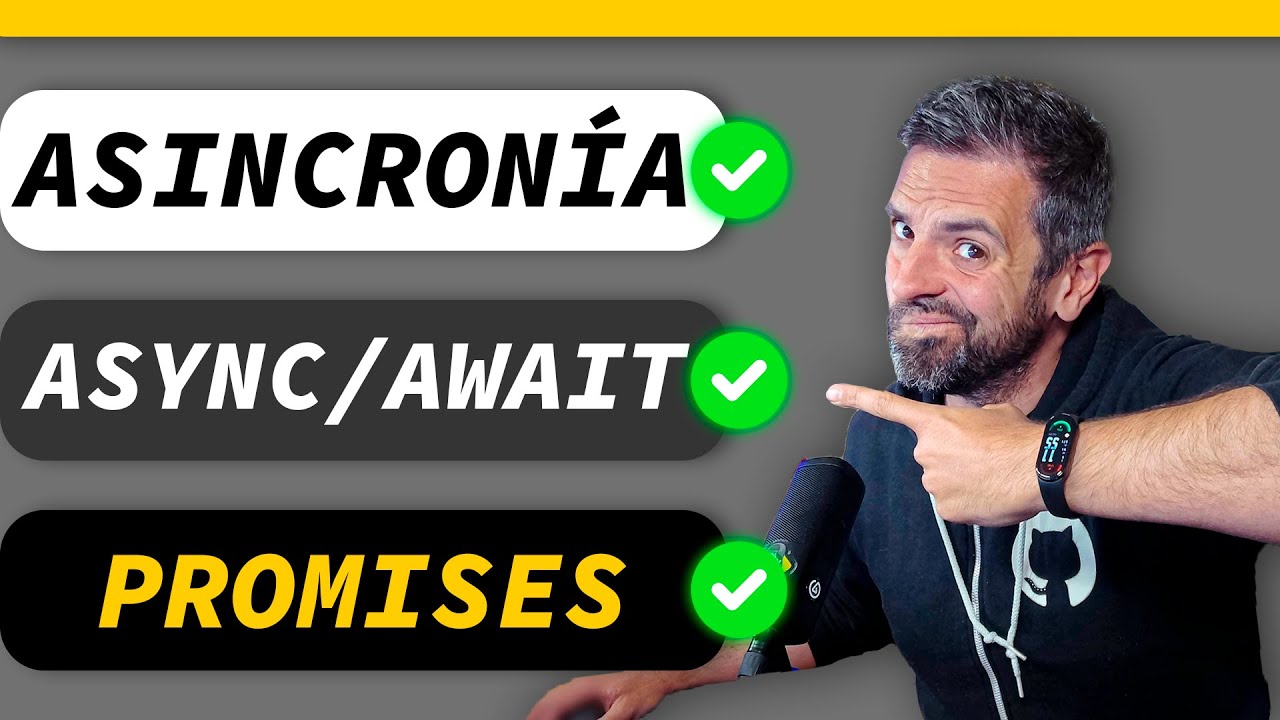
Asincronía en JavaScript: Todo lo que necesitas saber
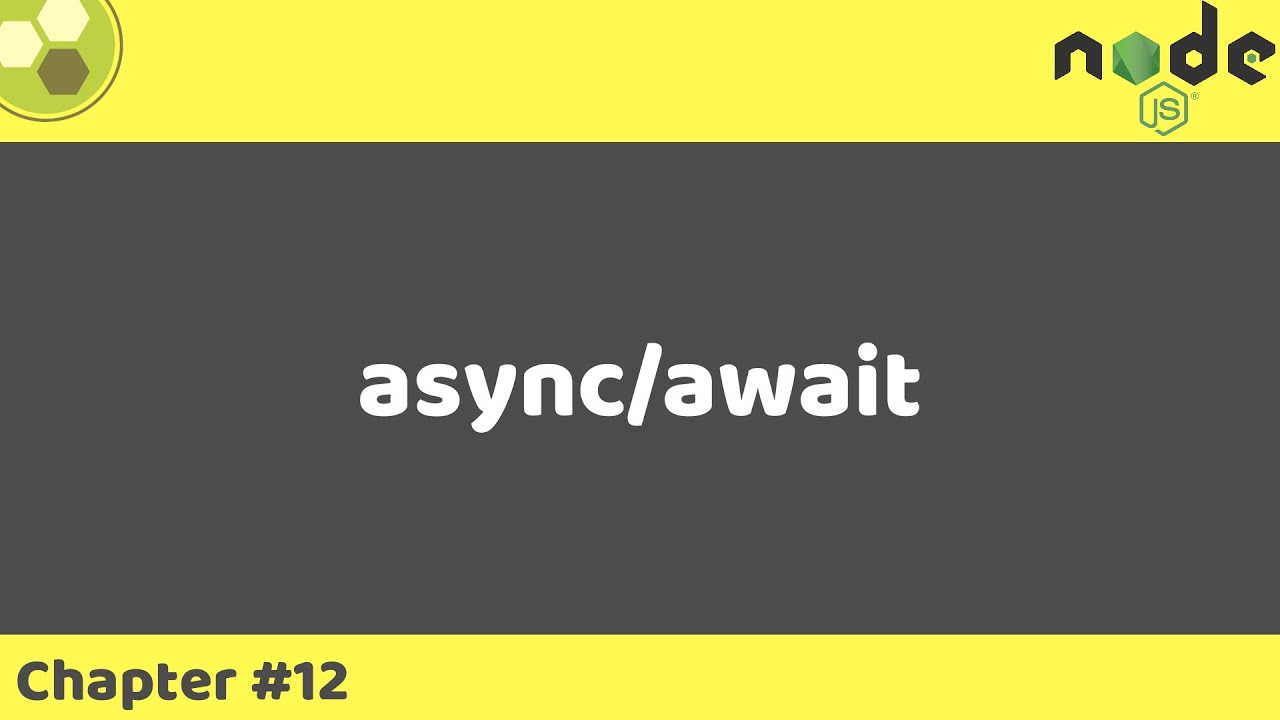
Node.js Tutorial #12 | async/await
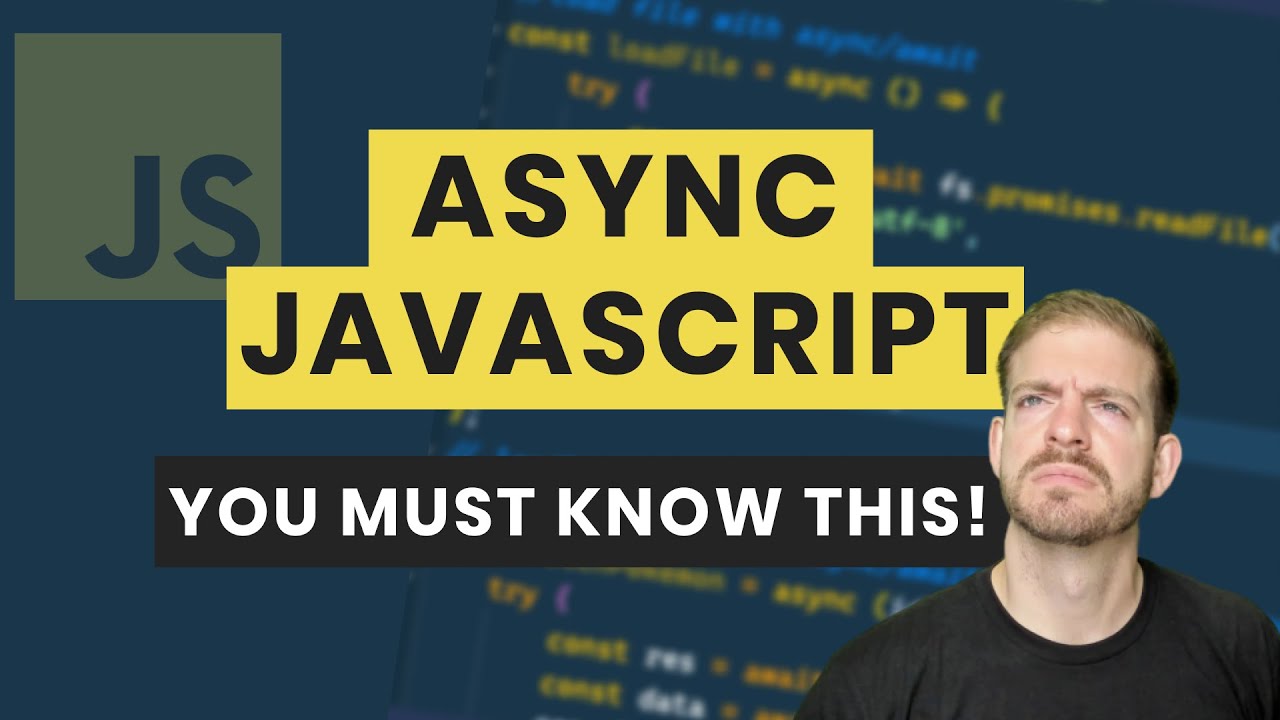
Asynchronous JavaScript in ~10 Minutes - Callbacks, Promises, and Async/Await
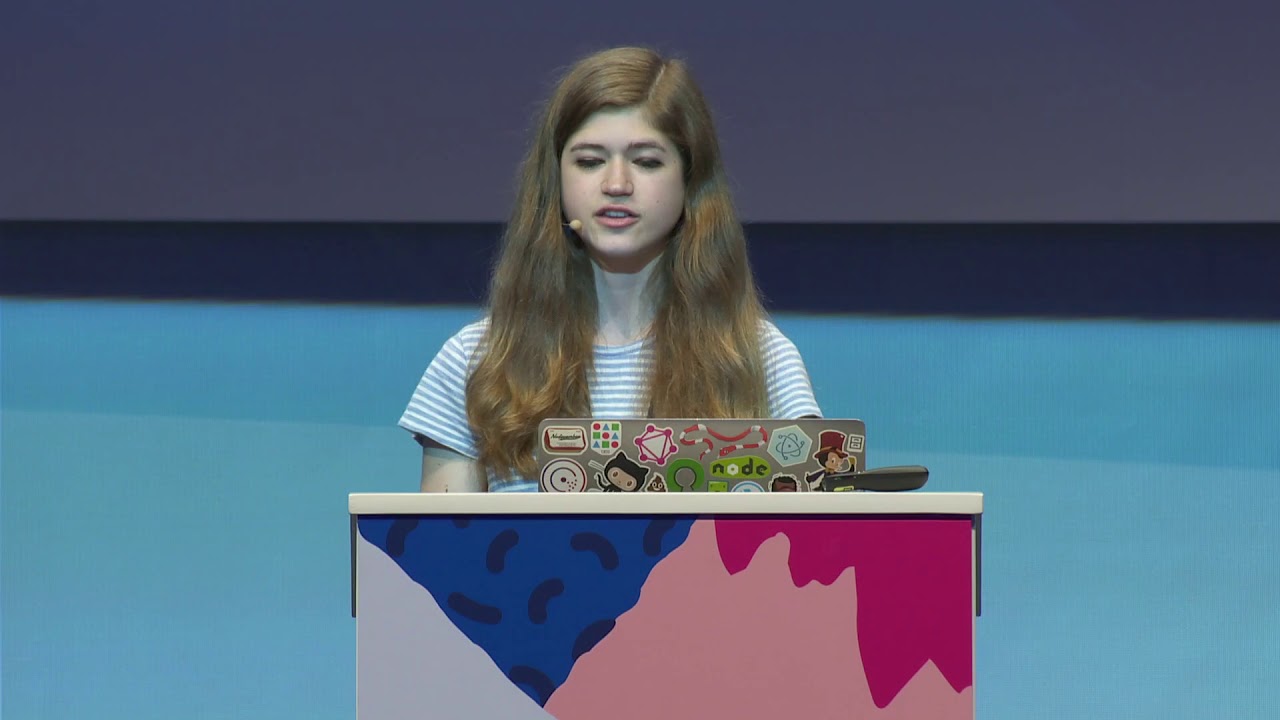
Asynchrony: Under the Hood - Shelley Vohr - JSConf EU
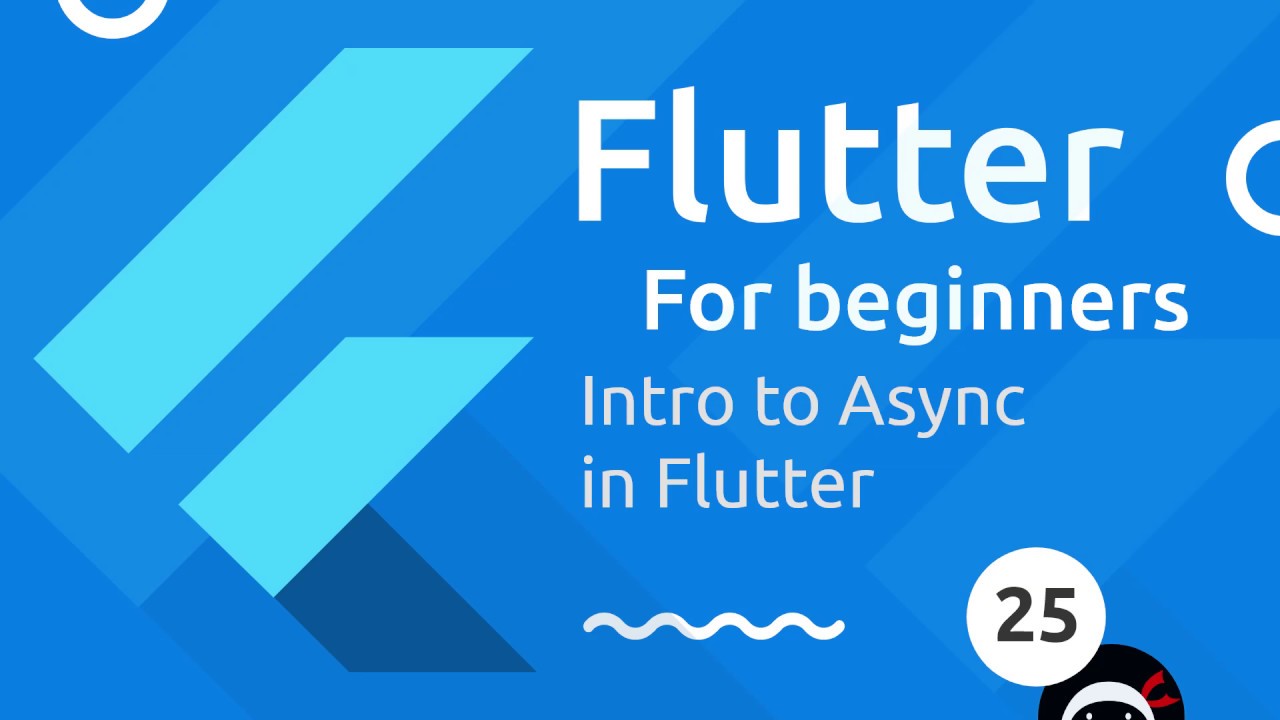
Flutter Tutorial for Beginners #25 - Asynchronous Code
5.0 / 5 (0 votes)