C# IEnumerable (Generator) Explained
Summary
TLDRThis video script offers a comprehensive tutorial on the concept of IEnumerable in programming. It begins with a basic explanation of IEnumerable and its function as a generator in other languages. The script then dives into a practical demonstration of creating an IEnumerable to generate numbers, explaining the use of 'yield return' over 'return' for continuous function execution. It further illustrates the execution order with steps and emphasizes the importance of not enumerating the list more than once for memory efficiency. The script concludes with a custom implementation of IEnumerable, comparing it to the generator approach and highlighting the benefits of using generators for efficient memory usage.
Takeaways
- 🔢 An `IEnumerable` can be created by using a method that yields a sequence of numbers.
- 🔄 In other languages, `IEnumerable` is known as a generator, used to produce a sequence of values.
- 📚 The `yield return` statement is used to yield a value and then continue the function for the next iteration.
- 🔁 When you use `IEnumerable`, it doesn't exit the function after yielding a value; it continues to the next iteration.
- 📈 The order of execution in an `IEnumerable` is predictable and can be demonstrated by stepping through the code.
- 🗂️ You can enumerate an `IEnumerable` to list its values, but doing so multiple times can be inefficient as it requires re-execution of the generator.
- 🚫 A common mistake is reusing an `IEnumerable` after it has been enumerated, as this can lead to unexpected results.
- 💾 `IEnumerable` is memory efficient because it generates values one at a time, rather than all at once.
- 🔄 The concept of a generator is to produce values on-demand, which is useful for processing large datasets without high memory usage.
- 🛠️ Custom implementation of `IEnumerable` involves creating a class that implements the `IEnumerable` interface and the `IEnumerator` interface, including methods like `MoveNext` and `Current`.
Q & A
What is an IEnumerable and how does it work?
-IEnumerable is an interface used to create a collection of values that can be iterated over. It works by yielding values one by one instead of creating a complete collection in memory, thus helping with memory efficiency.
What is the difference between 'return' and 'yield return' in the context of IEnumerable?
-'Return' exits the function after yielding a value, whereas 'yield return' yields a value and allows the function to continue executing the next time it's called.
Why is IEnumerable referred to as a generator in some programming languages?
-IEnumerable is referred to as a generator because it generates values on-the-fly, one at a time, rather than creating a static collection of values.
How does the process of enumeration work with IEnumerable?
-Enumeration with IEnumerable involves triggering the generator to produce values one by one. This is done by calling methods like 'ToList()', 'First()', or 'Count()' which initiate the iteration process.
What is the significance of the 'GetEnumerator' method in the context of IEnumerable?
-The 'GetEnumerator' method is crucial as it returns the enumerator that is used to iterate through the collection created by the IEnumerable.
Why should you be cautious when using 'Count' on an IEnumerable?
-Using 'Count' on an IEnumerable triggers enumeration, which means it goes through all the values to count them. If you need to use the values again, it should be avoided as it would enumerate them a second time.
What is the purpose of the 'Current' property in an IEnumerator?
-The 'Current' property in an IEnumerator holds the current value of the enumeration, which is the value that has been yielded by the generator at the current position of iteration.
How does the 'MoveNext' function relate to the iteration process in IEnumerable?
-The 'MoveNext' function is part of the IEnumerator interface and is used to advance the enumerator to the next element in the collection. It returns true if the enumerator was successfully advanced, false if the end is reached.
What is the role of the 'Reset' method in IEnumerator?
-The 'Reset' method is used to reset the enumerator to its initial position, which is before the first element in the collection. This allows the iteration to start over from the beginning.
Can you provide an example of a realistic mistake when working with IEnumerable?
-A common mistake is reusing an IEnumerable after it has been enumerated, which results in an empty sequence because the generator has already produced all its values.
What is the benefit of using IEnumerable over creating a full list of items?
-IEnumerable is beneficial for memory efficiency as it generates values one at a time rather than allocating memory for the entire list, which is particularly useful when dealing with large datasets.
Outlines
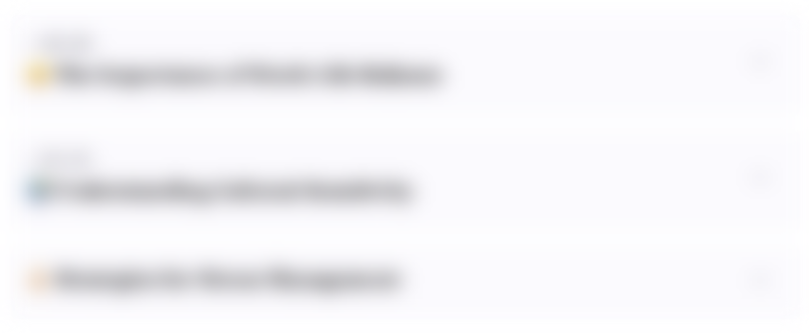
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
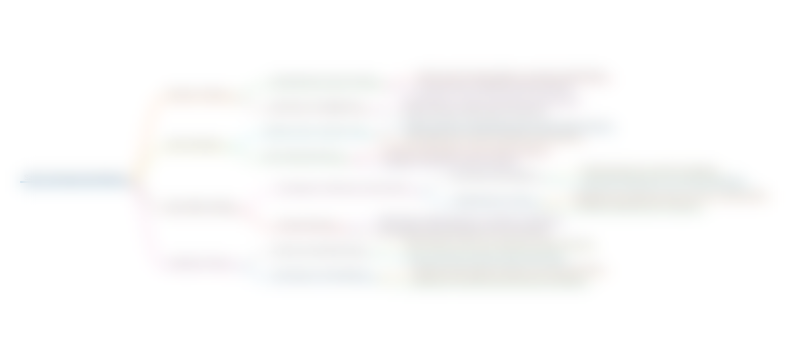
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
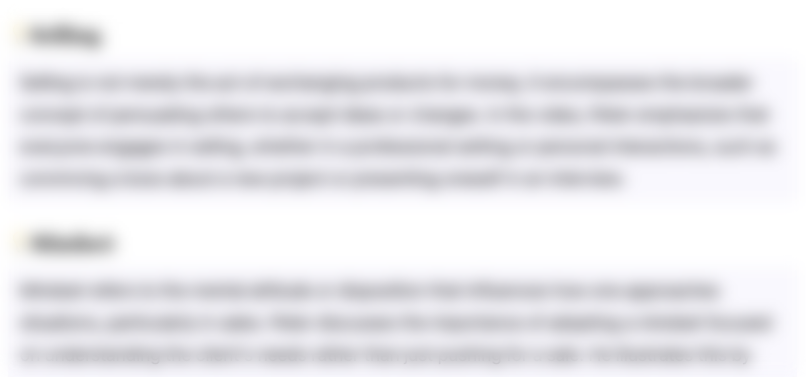
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
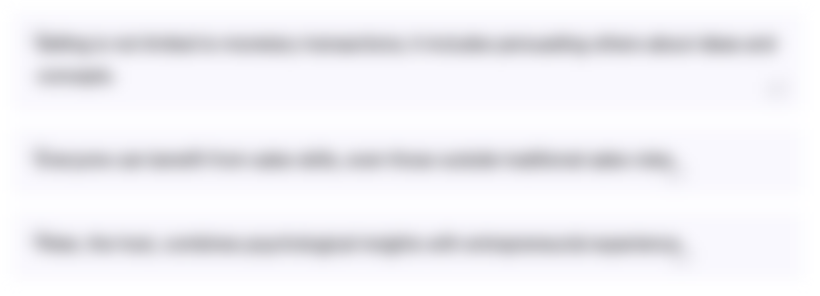
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
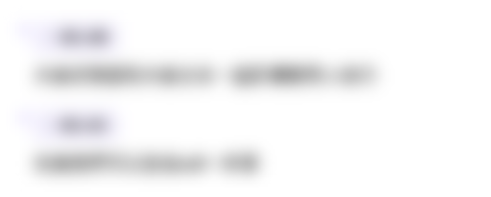
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード5.0 / 5 (0 votes)