Redux - Complete Tutorial (with Redux Toolkit)
Summary
TLDRIn this comprehensive tutorial, the presenter guides viewers through the essentials of Redux and Redux Toolkit, focusing on the core concepts of the state management library. Starting with an overview of Redux's role in managing global state for React applications, the tutorial delves into the three main components: the store, actions, and reducers. The presenter then demonstrates how to build a simple application using Redux Toolkit, showcasing the ease of creating and connecting state slices, actions, and reducers. The video also covers advanced topics, including asynchronous actions with 'createAsyncThunk' and the use of Redux DevTools for debugging. By the end, viewers are equipped with a solid understanding of Redux and its practical implementation in modern web development.
Takeaways
- 📚 Redux is a state management library for maintaining global state in React applications.
- 🏗️ The three main concepts of Redux are the store, actions, and reducers.
- 🛠️ The store holds the global state and is accessible from any component in the React app.
- 🔄 Actions describe changes to the state and have a type and an optional payload.
- 🎯 Reducers are pure functions that determine how the state should be updated based on actions.
- 📂 Redux Toolkit simplifies the setup and usage of Redux by providing utilities like `createSlice` and `createAsyncThunk`.
- 🔄 Immutability is a core principle in Redux, meaning the state should never be directly mutated but copied and replaced with updates.
- 🔗️ React Redux's `Provider` component is used to connect the Redux store to the React app, making the state accessible to all components.
- 🔑 The `useSelector` and `useDispatch` hooks allow React components to access the state and dispatch actions, respectively.
- 🛠️ Asynchronous actions can be handled with `createAsyncThunk`, which enables fetching data or performing other async tasks within Redux.
- 🐛 The Redux DevTools extension is a valuable tool for debugging and visualizing the state changes and actions in a Redux application.
Q & A
What is Redux and what does it do?
-Redux is a state management library that allows you to manage global state in the context of React applications. It enables state to be accessible from any component, regardless of its position in the component tree, making it easier to handle and update application state across different parts of the app.
What are the three main concepts of Redux?
-The three main concepts of Redux are the store, actions, and reducers. The store holds the global state, actions describe changes to the state, and reducers are functions that perform the state changes based on the action type and payload.
How does Redux ensure immutability of state?
-Redux ensures immutability by not allowing direct mutations to the state. Instead, reducers create a copy of the state, make changes to that copy, and then replace the entire state with the updated copy. This prevents unintended side effects and maintains the integrity of the state across the application.
What is a Redux toolkit and how does it simplify Redux setup?
-Redux toolkit is a collection of utilities and conventions that simplify the setup and usage of Redux. It provides functions like 'configureStore' for creating the store, 'createSlice' for generating reducers and actions, and 'createAsyncThunk' for handling asynchronous actions. These utilities reduce boilerplate code and streamline the process of building Redux applications.
How do you create a Redux store using Redux toolkit?
-To create a Redux store with Redux toolkit, you import 'configureStore' from '@reduxjs/toolkit'. You then use 'configureStore' to create your store, passing in an object with a 'reducer' property. Initially, this can be an empty object, and later you can add your reducers from different slices of state.
What is the purpose of the 'Provider' component in React Redux?
-The 'Provider' component in React Redux is used to make the Redux store available to all components in the application. It is a higher-order component that wraps your application, allowing components to access the store and use hooks like 'useSelector' and 'useDispatch' to interact with the Redux state and dispatch actions.
How do you create a slice of state in Redux using Redux toolkit?
-To create a slice of state in Redux with Redux toolkit, you use the 'createSlice' function. This function takes an object with properties like 'name', 'initialState', and 'reducers'. The 'name' is a string that identifies the slice, 'initialState' is the starting state, and 'reducers' is an object where each key is a reducer function that handles specific actions.
What is an action in Redux and how is it structured?
-An action in Redux is an object that describes a change to the state. It has a 'type' property, which is a string that identifies the action, and may have a 'payload' property, which carries additional data needed for the action. Actions are used to trigger updates to the state by being dispatched to the store.
How do you handle asynchronous actions in Redux with Redux toolkit?
-Asynchronous actions in Redux with Redux toolkit are handled using 'createAsyncThunk'. This function creates an action creator that returns a function, which can be an asynchronous operation like an API call. The async thunk action returns a promise, and you can handle the pending, fulfilled, and rejected states in the reducers to update the state accordingly.
What is the Redux DevTools and how does it help with debugging?
-Redux DevTools is a browser extension that provides a visual interface for monitoring and debugging Redux applications. It allows you to track the state changes, inspect actions, and even jump back and forth in time to see the state at different points. This tool is invaluable for understanding the flow of state updates and diagnosing issues in the application.
How does the order of defining synchronous and asynchronous actions differ in Redux toolkit?
-For synchronous actions in Redux toolkit, you first define the reducer and then create the action using 'createSlice'. However, for asynchronous actions, you define the action creator first using 'createAsyncThunk', and then you handle it in the reducers using 'extraReducers' builder, which allows you to handle the pending, fulfilled, and rejected states.
Outlines
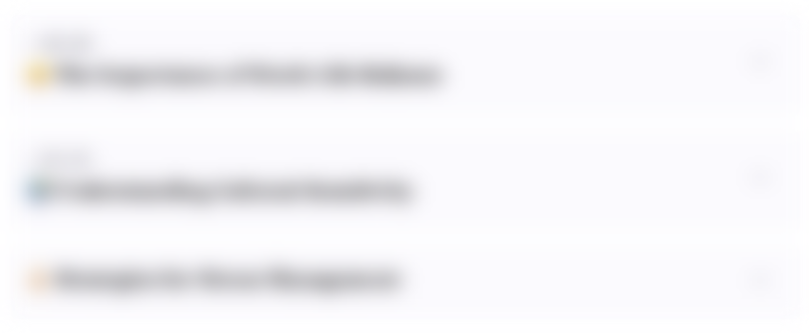
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
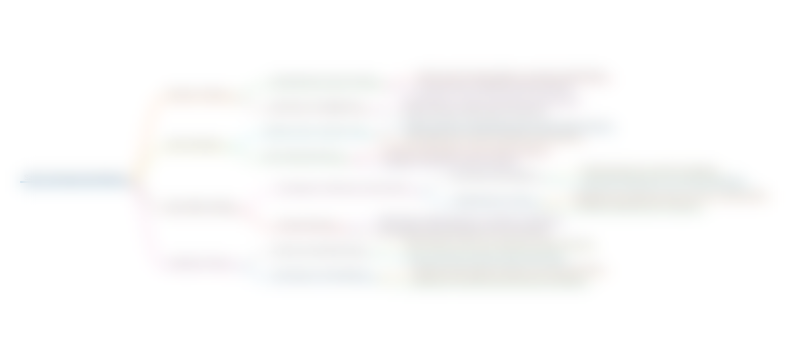
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
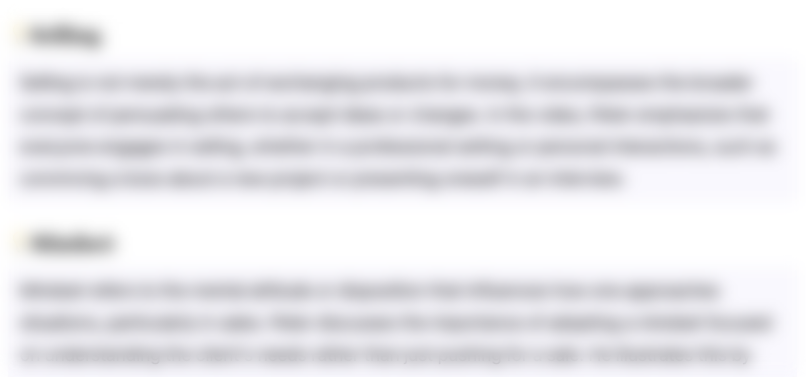
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
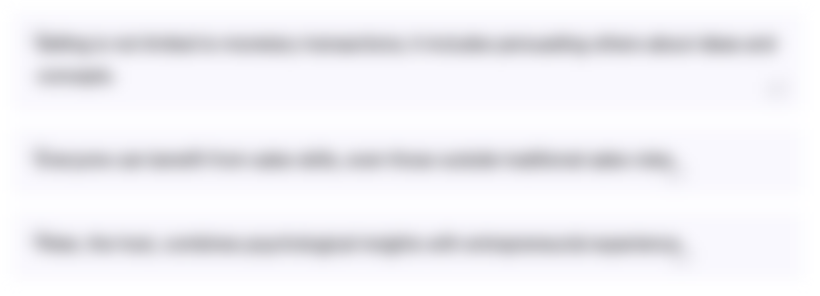
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
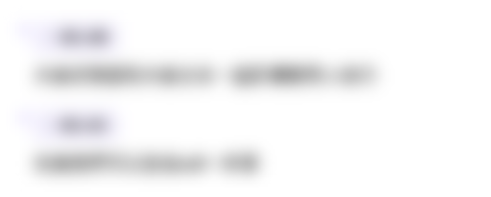
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード5.0 / 5 (0 votes)