Coding Exercise for Beginners in Python with solution | Exercise 23 | Python for Beginners #lec68
Summary
TLDRIn this Python programming tutorial, the focus is on converting student marks into grades using dictionaries. The video demonstrates how to create a dictionary with students' names as keys and their marks as values, then transform these marks into grades according to a specified grading system. The instructor guides viewers through writing a program that loops through the dictionary, applies conditional statements to assign grades, and stores these in a new dictionary. The video concludes with a practical coding exercise that reinforces the concepts discussed.
Takeaways
- 💻 The video is part of a series teaching Python programming language, specifically focusing on dictionaries.
- 📚 The previous video discussed dictionaries in Python, and this video builds on that knowledge with a coding exercise.
- 📝 The exercise involves converting student marks stored in a dictionary into grades using another dictionary.
- 🔑 The original dictionary contains student names as keys and their marks as values.
- 📊 The grading criteria are provided, with different ranges of marks corresponding to different grades (A+ for 91-100, A for 81-90, etc.).
- 📋 The video suggests creating an empty dictionary called 'student_grade' to store the converted grades.
- 🔍 The video demonstrates how to loop through the 'student_marks' dictionary to access each student's marks.
- 💡 It's explained that conditional statements (if-elif-else) are used to determine the grade based on the marks.
- 📝 The video provides a step-by-step guide on how to write the code for the exercise.
- 👨🏫 The instructor encourages viewers to pause the video and attempt the exercise before watching the solution.
- 🔗 The video concludes with a mention of future videos that will cover nested dictionaries and lists.
Q & A
What is the main topic of the video?
-The main topic of the video is a coding exercise involving Python dictionaries, specifically converting student marks into grades.
What is the structure of the student marks dictionary discussed in the video?
-The student marks dictionary has student names as keys and their corresponding marks as values.
What is the purpose of creating a new dictionary called 'student_grade'?
-The purpose of creating 'student_grade' is to store the student names as keys and their corresponding grades as values based on the marks obtained.
What are the grading criteria mentioned in the video?
-The grading criteria are: 91-100 for A+, 81-90 for A, 71-80 for B+, and below 40 for F. The video suggests using if-else conditions to assign these grades.
How does the video suggest looping through the student marks dictionary?
-The video suggests using a for loop to iterate over the keys (student names) in the student marks dictionary.
What is the significance of the variable 'marks' in the coding exercise?
-The variable 'marks' is used to store the value (marks) associated with each key (student name) in the student marks dictionary during the loop.
Why is it unnecessary to use 'and' conditions for the grade ranges in the if-else statements?
-It is unnecessary to use 'and' conditions because the if-else structure naturally progresses to the next condition if the previous one is not met, ensuring only one grade is assigned per student.
What is the final step in the coding exercise after assigning grades?
-The final step is to print the 'student_grade' dictionary which contains the student names and their corresponding grades.
How does the video handle students with marks below 40?
-For students with marks below 40, the grade 'F' is assigned in the else part of the if-else condition.
What is the recommendation for viewers who are confused about the if-else conditions?
-The video recommends writing down the code and manually testing it with different marks to better understand the logic.
Outlines
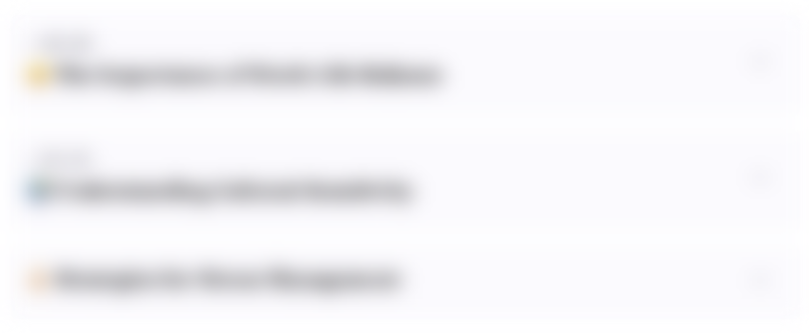
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
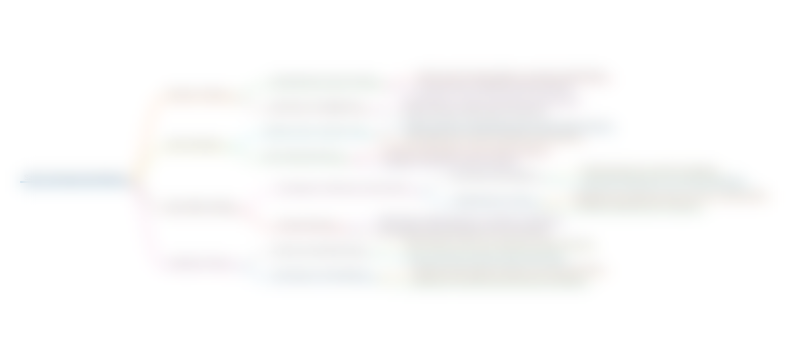
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
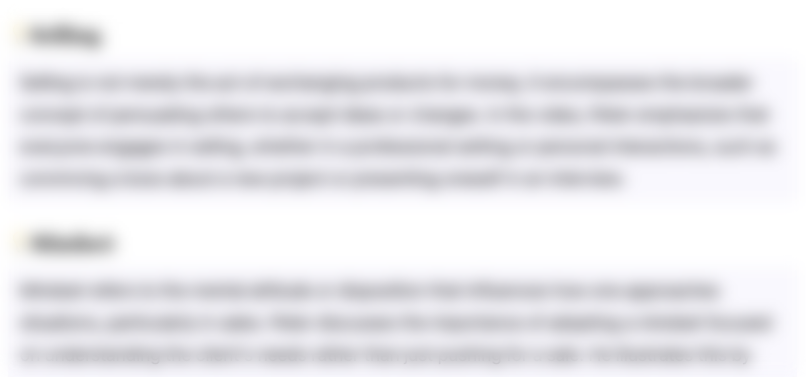
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
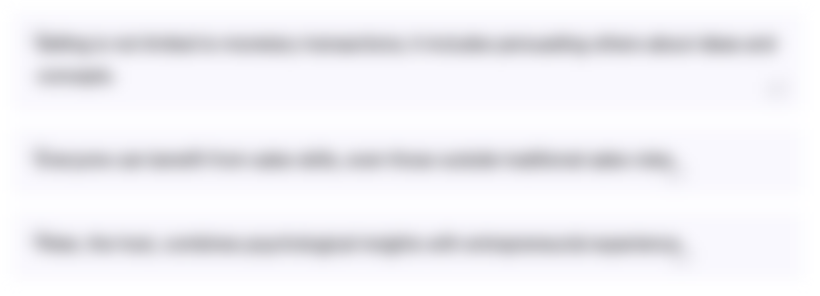
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
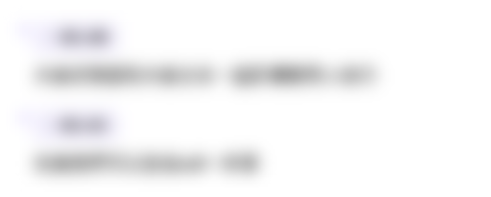
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード5.0 / 5 (0 votes)