Javascript Promises vs Async Await EXPLAINED (in 5 minutes)
Summary
TLDRIn this informative video, Chris Roberts explores JavaScript Promises, explaining their necessity for handling asynchronous operations. He demonstrates how to use 'then' and 'catch' methods to manage promises and introduces the 'async' and 'await' keywords for cleaner, more sequential code execution. With a practical example involving an API request, he illustrates the process of fetching data and handling errors, ultimately simplifying complex asynchronous code into a more readable format.
Takeaways
- 🌟 JavaScript Promises are used for handling asynchronous operations, representing a future completion or failure of a task.
- 🔗 The 'then' method is used to handle the successful completion of a Promise, allowing access to the result of the asynchronous operation.
- 🚫 The 'catch' method is used to handle any errors that occur during the execution of the Promise, providing a way to deal with failures.
- 📚 Promises are compared to a real-world scenario, like ordering coffee at a restaurant, where you must wait for the coffee to be brought to you.
- 🛠 The 'axios' library is used in the example to demonstrate how to make API requests and handle the returned Promises.
- 📝 The script explains that using Promises can lead to 'callback hell', where nested callbacks make the code difficult to read and maintain.
- 🔄 The 'async' and 'await' keywords are introduced as a way to simplify asynchronous code, making it more readable and sequential.
- 🛑 The 'await' keyword is used to pause the execution of the function until the Promise is resolved, allowing for a more straightforward code flow.
- 🔀 The 'try...catch' block is used in conjunction with 'async' functions to handle errors, similar to how it's used in synchronous code.
- 🎥 The video aims to demystify the use of Promises and async/await in JavaScript, providing practical examples and clear explanations.
Q & A
What is the main topic of the video by Chris Roberts?
-The main topic of the video is an explanation of JavaScript Promises, including why they are needed, how to use the 'then' and 'catch' methods, and how to convert code using these to using 'async' and 'await' keywords.
Why are JavaScript Promises used in programming?
-JavaScript Promises are used to handle asynchronous operations, such as database calls, file operations, or API requests, which do not return results immediately but instead return a promise that will eventually complete or fail.
What is the real-world analogy used in the video to explain Promises?
-The real-world analogy used is ordering coffee at a restaurant. Just as you wait for the waiter to bring your coffee, in JavaScript, you wait for a promise to be fulfilled with the result of an asynchronous operation.
What is the purpose of the 'then' method in Promises?
-The 'then' method is used to specify what should happen when the promise is fulfilled. It receives the result of the task as a parameter and allows you to run code with that result.
What is the purpose of the 'catch' method in Promises?
-The 'catch' method is used to handle any errors that occur while processing the promise. It receives the error as a parameter and allows you to run error-handling code.
How does the 'await' keyword work with Promises?
-The 'await' keyword is used to wait for a promise to be resolved before moving on to the next line of code. It allows for a cleaner and more synchronous-looking code structure when dealing with asynchronous operations.
What is required for a function to use the 'await' keyword?
-The 'await' keyword must be used inside a function that is marked with the 'async' keyword, indicating that the function contains asynchronous code.
How does the video demonstrate the use of Promises with an example?
-The video uses an example of an app that suggests activities when you are bored, making a request to a 'board' API and logging the suggested activity to the console, demonstrating the use of 'then', 'catch', and 'await'.
What is the issue with the initial code example in the video?
-The initial code example fails because it tries to access properties ('data' and 'activity') on a response object that is not structured as expected, leading to an error.
How does the video address the problem of sequential code execution with Promises?
-The video introduces the 'async' and 'await' keywords as a solution to make the code neater and more readable, allowing for sequential execution of asynchronous code.
How does the video handle errors in the context of 'async' and 'await'?
-With 'async' and 'await', errors are handled using a standard try-catch block. If an error occurs, it is caught and can be logged or handled within the catch block.
Outlines
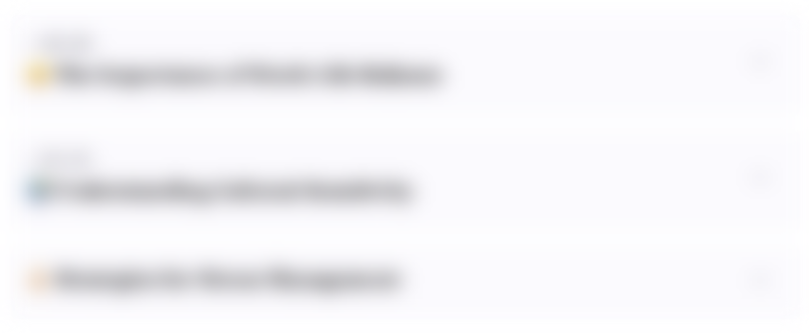
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
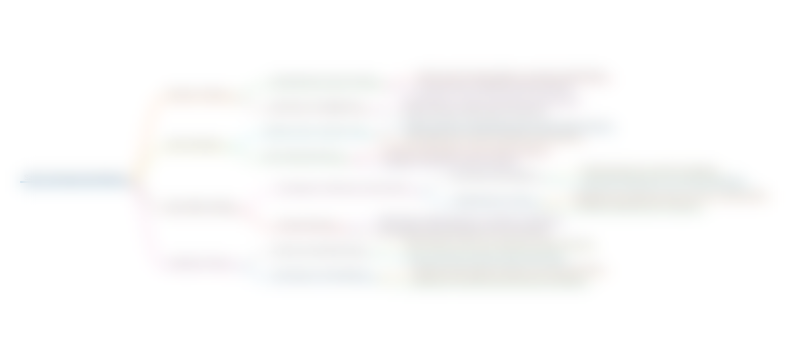
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
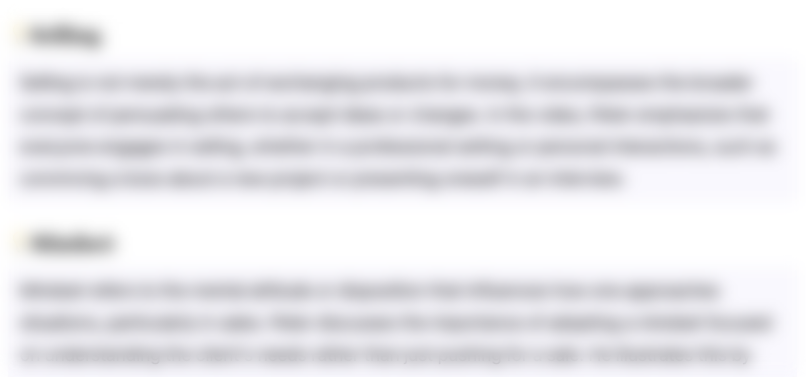
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
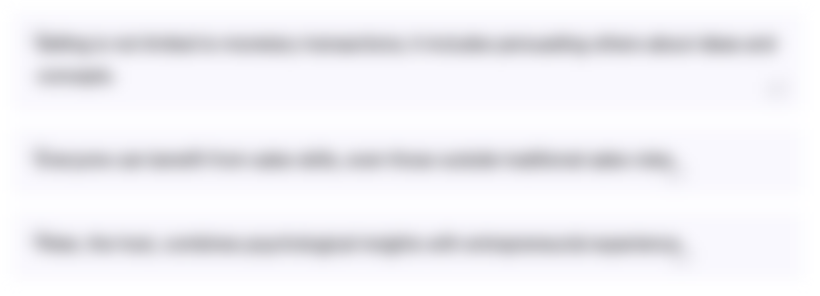
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
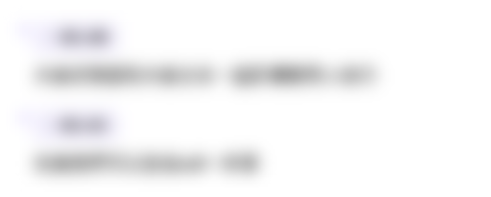
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
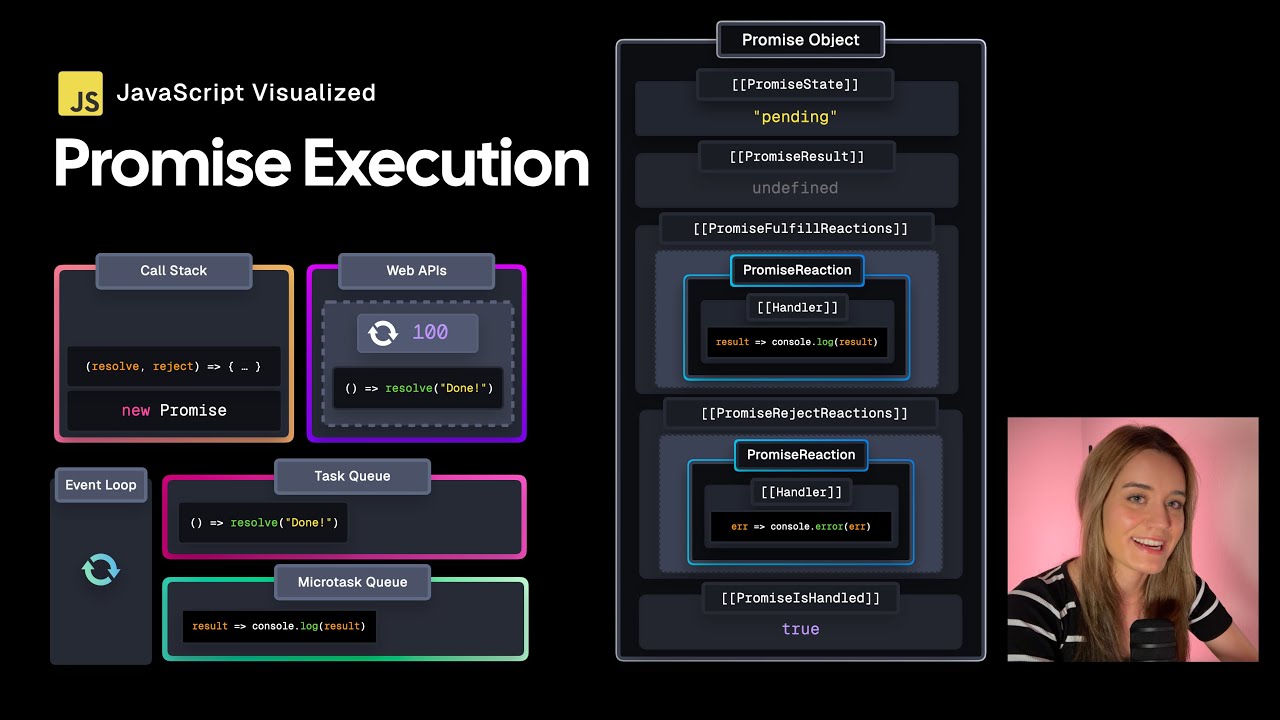
JavaScript Visualized - Promise Execution
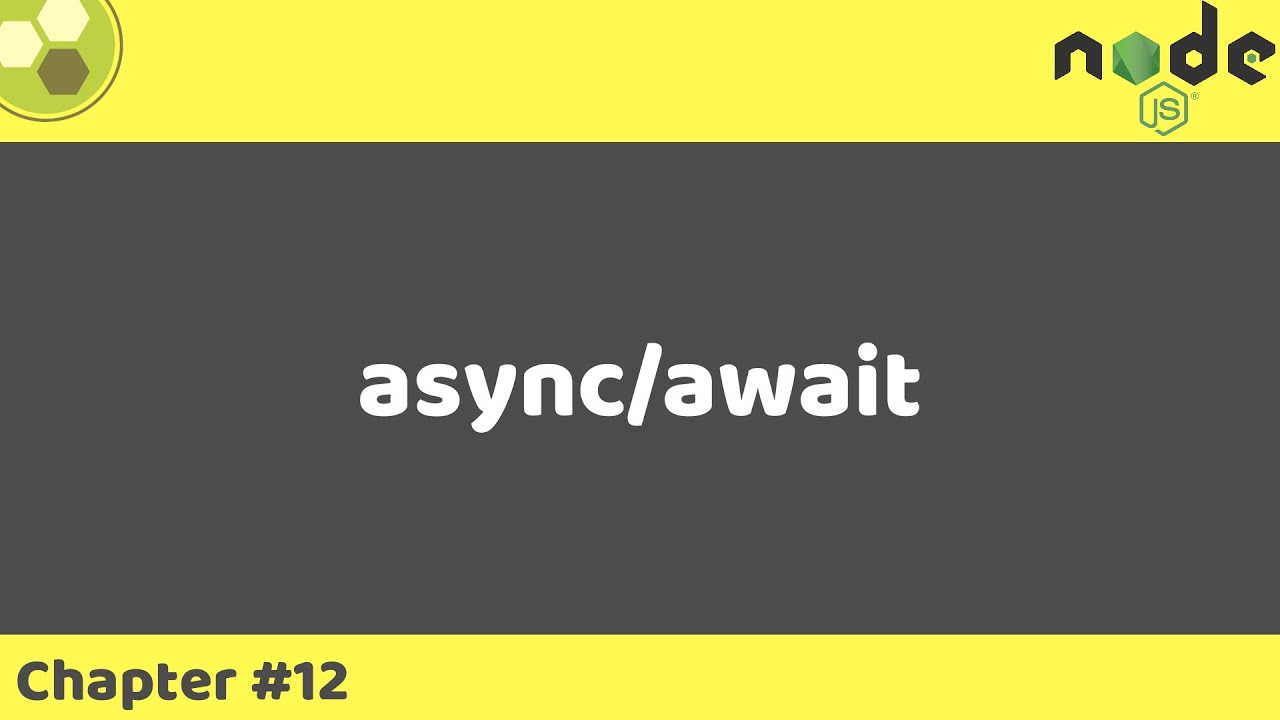
Node.js Tutorial #12 | async/await
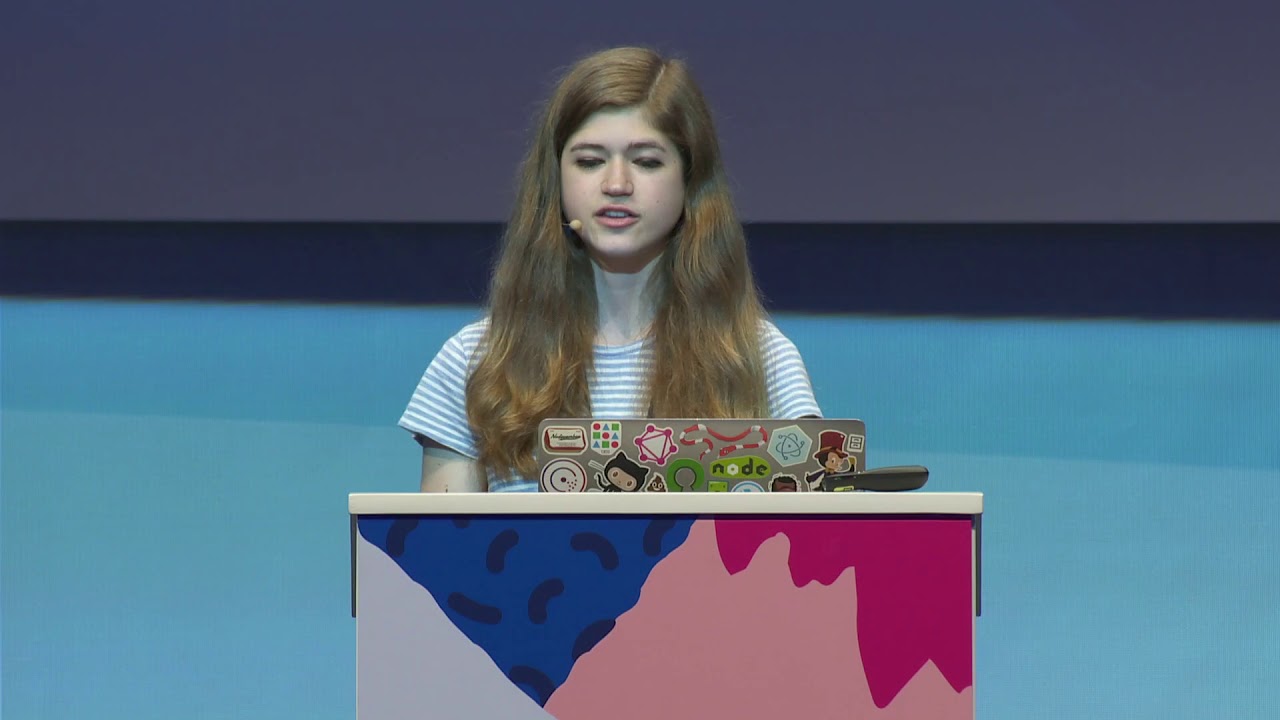
Asynchrony: Under the Hood - Shelley Vohr - JSConf EU
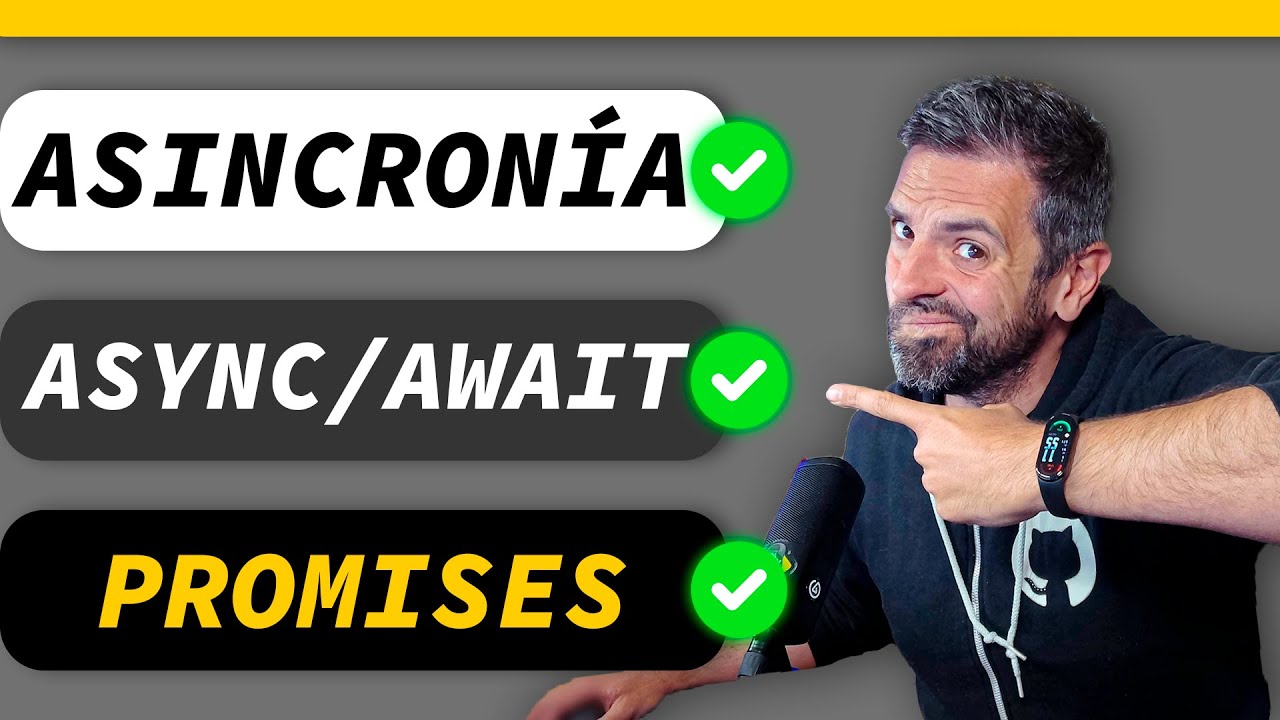
Asincronía en JavaScript: Todo lo que necesitas saber

What the heck is the event loop anyway? | Philip Roberts | JSConf EU
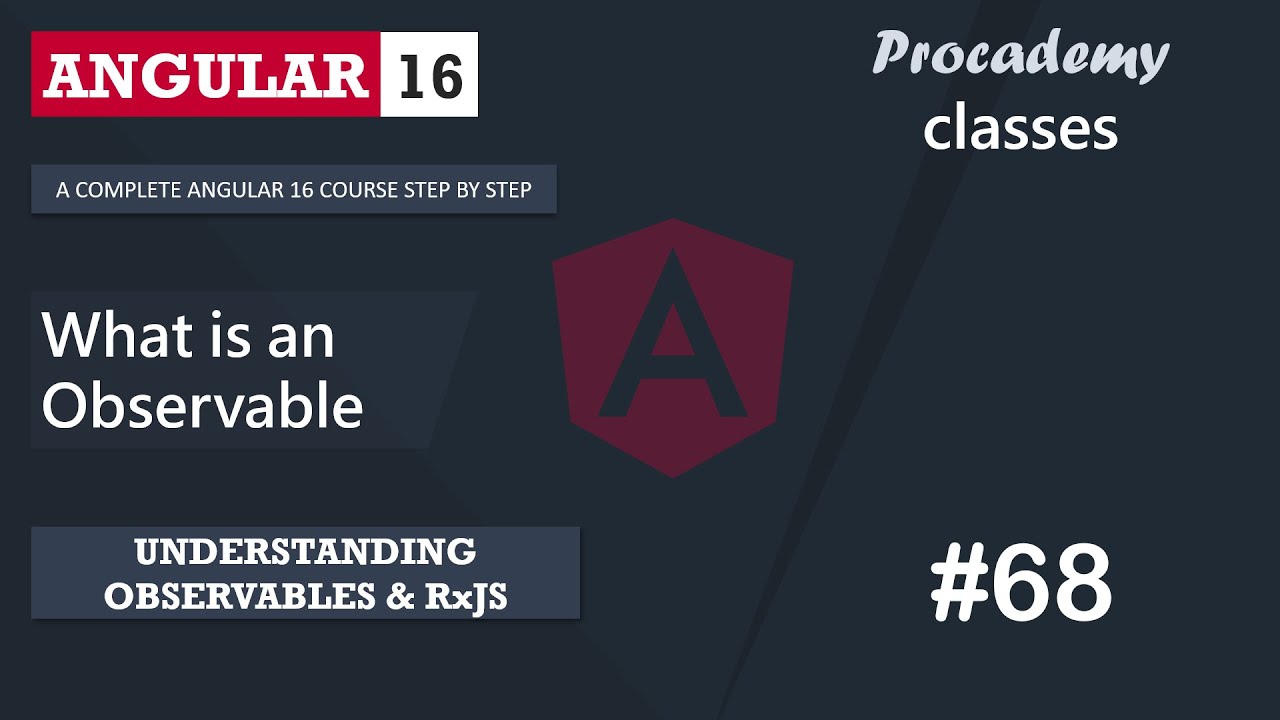
#68 What is an Observable | Understanding Observables & RxJS | A Complete Angular Course
5.0 / 5 (0 votes)