The call and apply Methods | JavaScript 🔥 | Lecture 124
Summary
TLDRIn this informative lecture, we delve into the intricacies of the 'this' keyword in JavaScript, particularly focusing on how to manually set it and understand its significance in different contexts. Using Lufthansa, the largest European airline group, as an example, we explore creating a simple booking system. We illustrate how the 'this' keyword can dynamically point to different objects, such as Lufthansa and Eurowings, to handle bookings. Through practical examples, we introduce the use of JavaScript's first-class functions and the methods 'call', 'apply', and 'bind' to explicitly set the 'this' keyword, demonstrating how to efficiently manage function contexts across different objects without duplicating code.
Takeaways
- 📚 The 'this' keyword in JavaScript refers to the object it belongs to, and its value varies depending on how the function is called.
- 💻 Enhanced object literal syntax allows for simpler method definitions in JavaScript objects.
- ✈️ Example given involves creating a booking system for airlines like Lufthansa and Eurowings, demonstrating practical use of 'this'.
- 📈 Methods within objects can access the object's properties and array of bookings using 'this' keyword.
- ⚡️ Directly copying methods between objects is discouraged due to bad practice; instead, functions should be stored externally and reused.
- 🔧 JavaScript's first-class functions enable the storage of functions in variables for reuse across different contexts.
- 🔒 Regular function calls set 'this' to undefined in strict mode, illustrating the dynamic nature of 'this'.
- 🔨 The 'call' method allows explicit setting of 'this' for a function, enabling flexibility across different objects.
- 👨🔧 The 'apply' method is similar to 'call', but takes an array of arguments instead.
- 🔫 Modern JavaScript favors the 'call' method with spread operator over 'apply' for passing an array of arguments.
- 🔔 'bind' method offers another way to set 'this' keyword but is left for further discussion in the next lecture.
Q & A
What is the main topic being discussed in this lecture?
-The main topic being discussed is how to manually set the 'this' keyword in JavaScript using the call, apply, and bind methods, and why you would want to do that.
Why is it considered a bad practice to copy and paste methods across different objects?
-It is considered a bad practice because it violates the DRY (Don't Repeat Yourself) principle of programming. Instead, it's better to create a separate function that can be reused across different objects.
What happens when you call a regular function, and how does it affect the value of the 'this' keyword?
-When you call a regular function (not a method), the 'this' keyword inside that function points to undefined in strict mode. This is because the function is not bound to any specific object.
How does the call() method allow you to manually set the 'this' keyword?
-The call() method allows you to manually set the 'this' keyword by passing the object you want 'this' to reference as the first argument. The remaining arguments are passed to the function being called.
What is the difference between the call() and apply() methods?
-The call() method accepts the arguments to be passed to the function individually, while the apply() method accepts an array of arguments to be passed to the function.
How can you achieve the same functionality as apply() in modern JavaScript?
-In modern JavaScript, you can use the spread operator (...) with the call() method to spread the elements of an array as individual arguments, achieving the same functionality as apply().
Why is the bind() method considered more important than call() and apply()?
-The bind() method is considered more important because it allows you to create a new function with the 'this' keyword permanently bound to a specific object, which can be useful in certain scenarios like event handlers or currying.
What are the property names that the 'book' method expects to exist on the airline objects?
-The 'book' method expects the airline objects to have properties named 'airline', 'iataCode', and 'bookings'.
Why is it important to understand the dynamics of the 'this' keyword in JavaScript?
-It is important to understand the dynamics of the 'this' keyword because its value can change depending on how a function is called, and understanding this behavior is crucial for writing correct and maintainable code.
What is the purpose of creating the 'book' function separately and assigning it to a variable?
-The purpose of creating the 'book' function separately and assigning it to a variable is to enable code reuse across different airline objects, rather than copying and pasting the method into each object.
Outlines
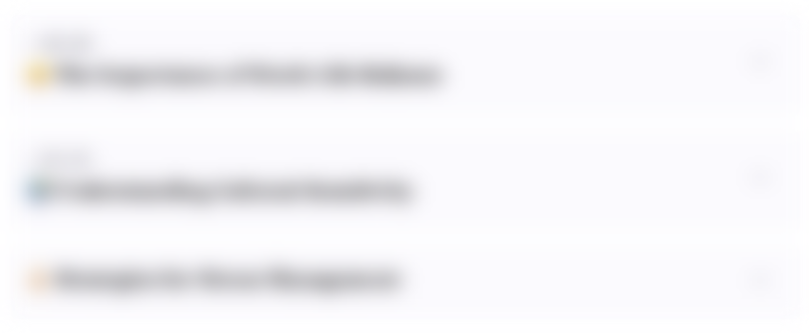
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
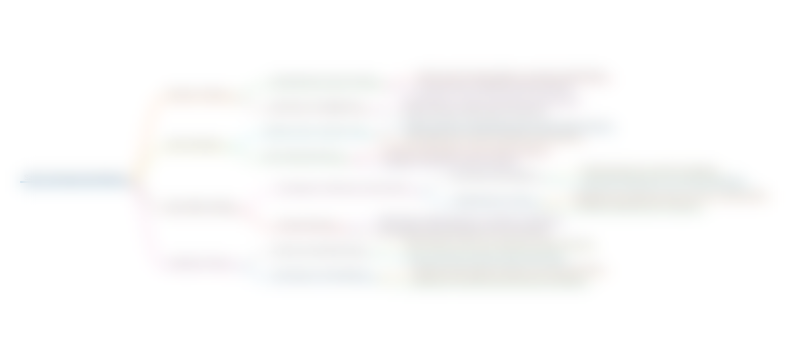
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
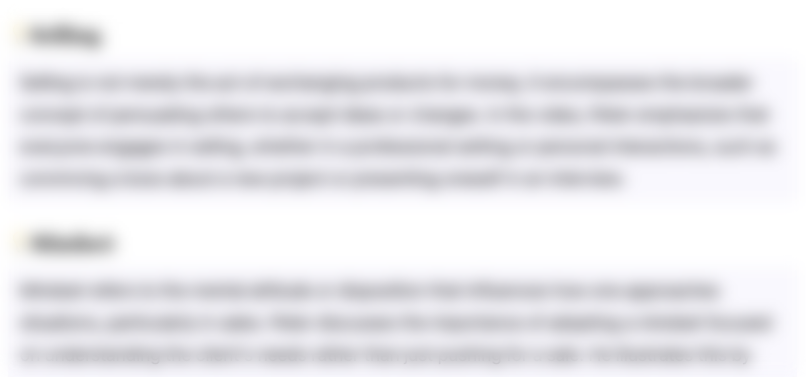
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
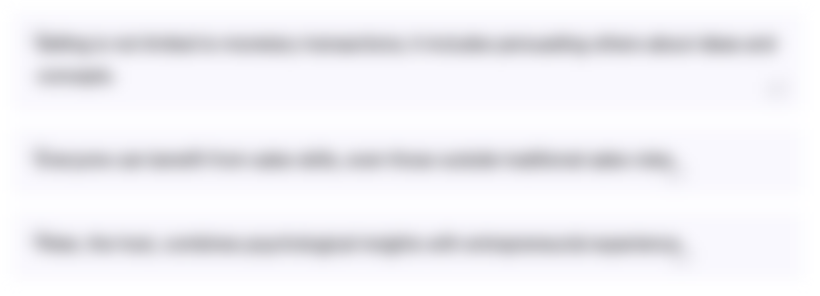
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
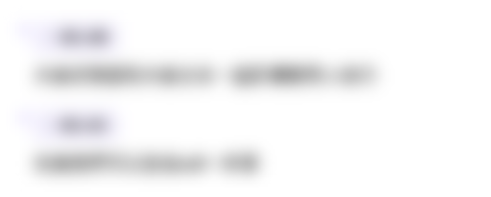
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示

Hoisting in JavaScript 🔥(variables & functions) | Namaste JavaScript Ep. 3
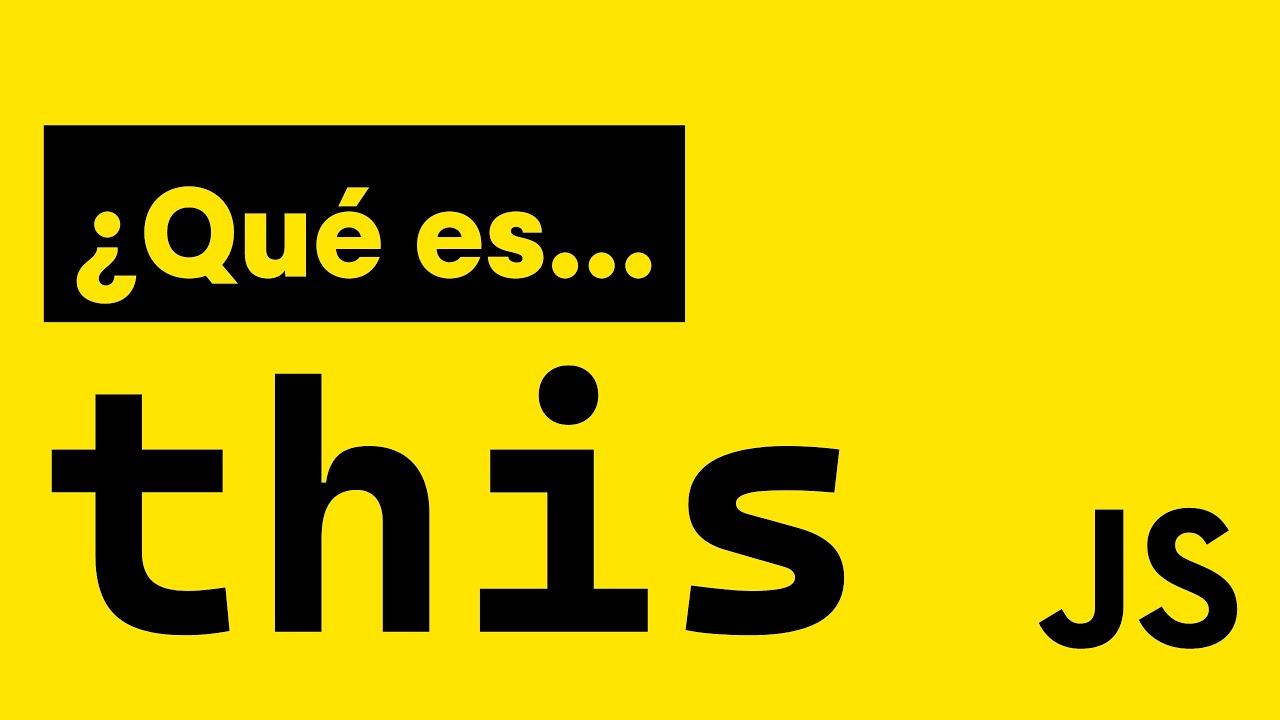
📚 ¿Qué es this en JavaScript? Explicación fácil, directa y sencilla con ejemplos

undefined vs not defined in JS 🤔 | Namaste JavaScript Ep. 6
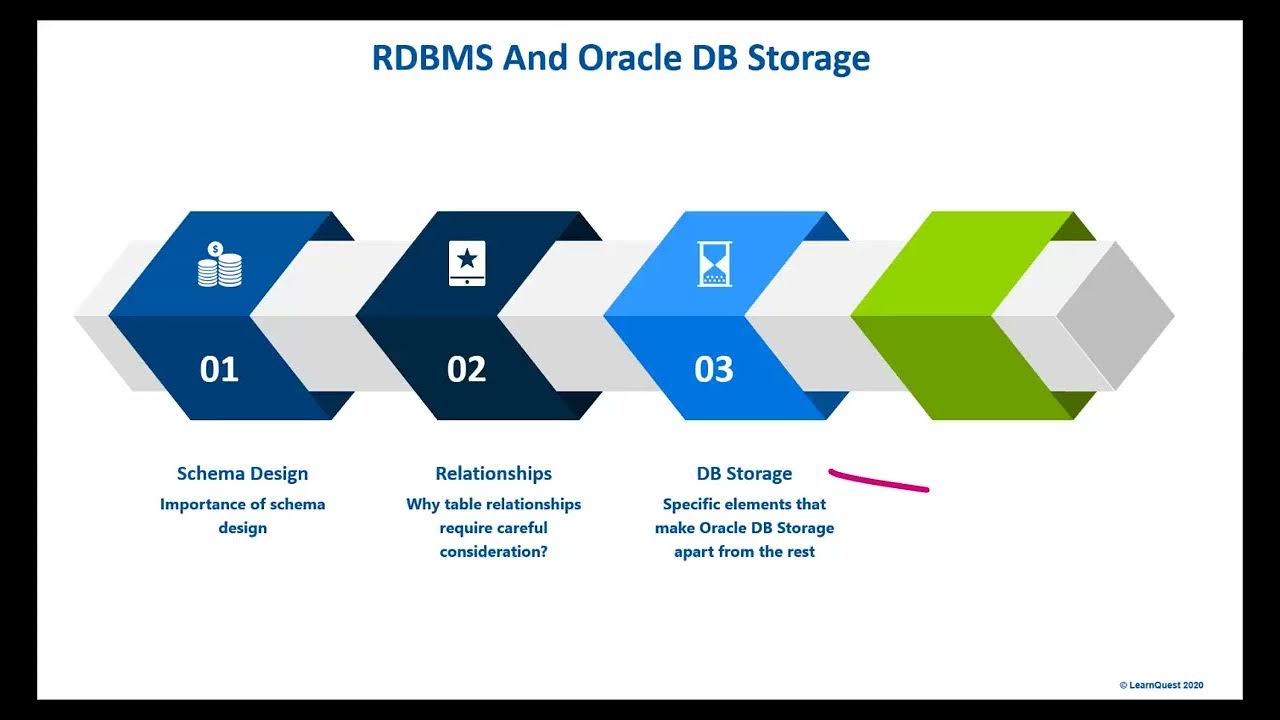
What You Will Learn in Module 4

How JavaScript Code is executed? ❤️& Call Stack | Namaste JavaScript Ep. 2

Quantum Mechanics by a Physicist | IIT- kharagpur, CMI
5.0 / 5 (0 votes)