5 Good Python Habits
Summary
TLDRThis video discusses five good habits for Python programming. It covers avoiding module execution on import, defining a main function, creating reusable functions, using type annotations for clarity and error prevention, and utilizing list comprehensions for efficiency and readability.
Takeaways
- 📚 Always check if `__name__` is equal to 'main' before running certain code to ensure it only executes when the script is run directly.
- 🔍 Use a main function as an entry point to organize code and combine all necessary functionality in a clean and readable manner.
- 🚫 Avoid having a single function handle too much functionality; instead, create separate functions for each task to improve reusability and maintainability.
- 👤 Implement checks for specific conditions, such as age verification and ID validation, in separate functions to keep the code modular and reusable.
- 🚫 Avoid hardcoding values directly in functions; use parameters to make functions more flexible and reusable.
- 📝 Utilize type annotations to provide clarity on what types of data a function expects and returns, improving code readability and reducing errors.
- 👀 Type annotations can act as self-documentation, helping developers understand what the function does without needing to read external documentation.
- 🛠️ Install a static type checker like `mypy` to catch type-related errors before running the script, ensuring type consistency and reducing runtime errors.
- 📈 Use list comprehensions for cleaner and potentially faster code when creating lists based on conditions, improving readability and reducing the amount of code.
- 🧩 Break down complex operations into simpler, reusable functions to make the code more maintainable and easier to understand.
- 💬 Consider using descriptive variable names in list comprehensions to enhance readability and make the code self-explanatory.
Q & A
What is the main purpose of the video?
-The main purpose of the video is to discuss five good habits that can be built in Python programming.
Why should you check if the script name is 'main' before running certain functions?
-Checking if the script name is 'main' ensures that the code will only be executed when the module is run directly, preventing accidental execution when the module is imported.
What is the benefit of defining a main entry point in your script?
-Defining a main entry point helps in organizing the code by consolidating all the functionality in one place, making it more readable and easier to manage.
Why is it recommended to separate functionality into different functions?
-Separating functionality into different functions increases the reusability of the code, making it easier to maintain and modify without affecting other parts of the script.
How does using type annotations help in Python programming?
-Type annotations help in providing clarity about the expected data types of variables and function parameters, reducing the chances of type-related errors and improving code readability.
What is the purpose of using 'if __name__ == "__main__"' in a Python script?
-The 'if __name__ == "__main__"' block is used to ensure that certain parts of the code are only executed when the script is run directly, not when it is imported as a module in another script.
Why is it important to keep functions simple and reusable?
-Keeping functions simple and reusable makes the code more maintainable and easier to understand, as it reduces the complexity and allows for easier debugging and modification.
What are the advantages of using list comprehensions in Python?
-List comprehensions offer a more concise and often more readable way to create lists, especially when filtering or transforming elements based on certain conditions.
How can type annotations help in avoiding type-related errors in Python?
-Type annotations provide a way to explicitly declare the expected data types of variables and function parameters, which can help in catching type-related errors at an early stage, often before the code is even run.
What is the role of a static type checker like mypy in Python programming?
-A static type checker like mypy helps in identifying potential type-related errors in the code by analyzing the type annotations, providing warnings and suggestions to improve code reliability.
Outlines
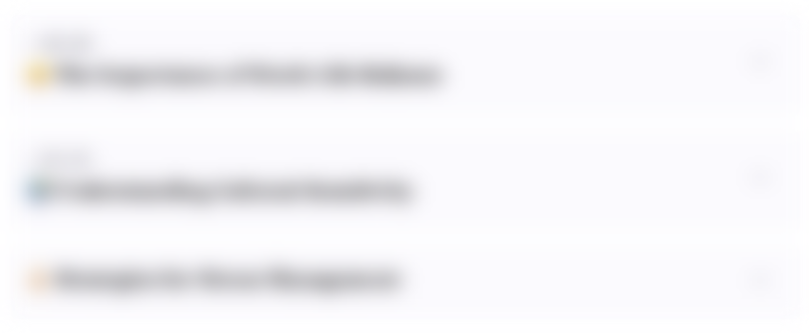
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
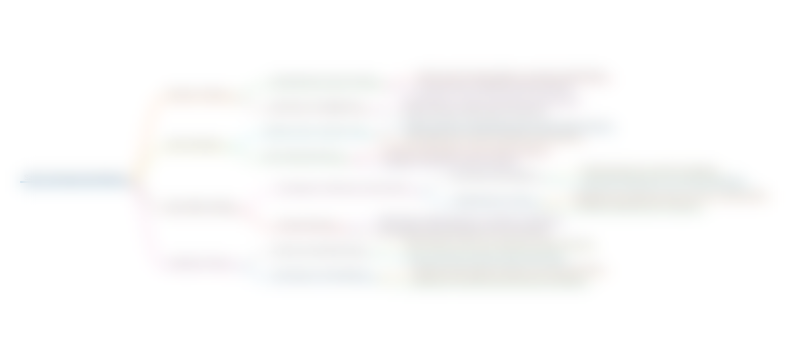
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
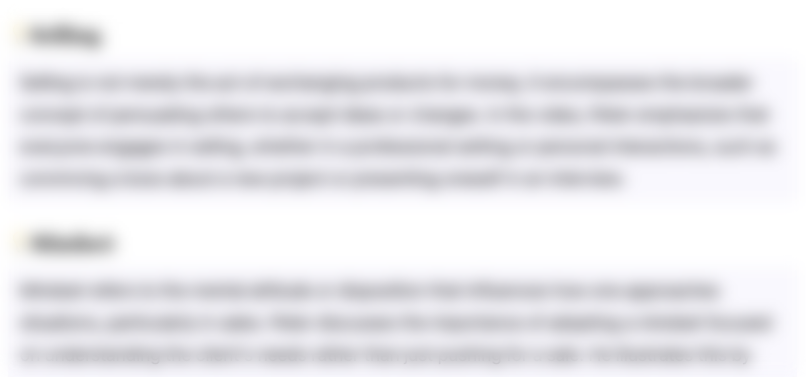
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
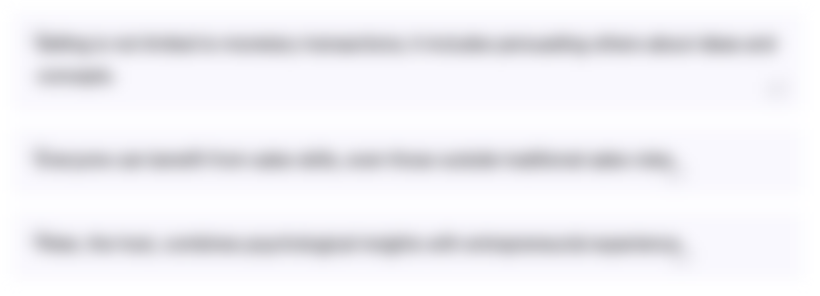
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
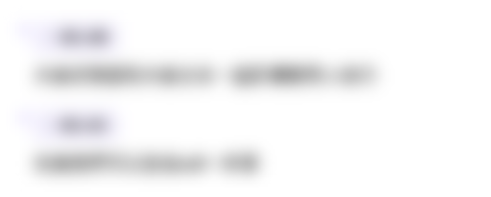
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes

The ONLY 5 Languages You Need For AI Development (Before It's Too Late!)

I’ve read 40 programming books. Top 5 you must read.
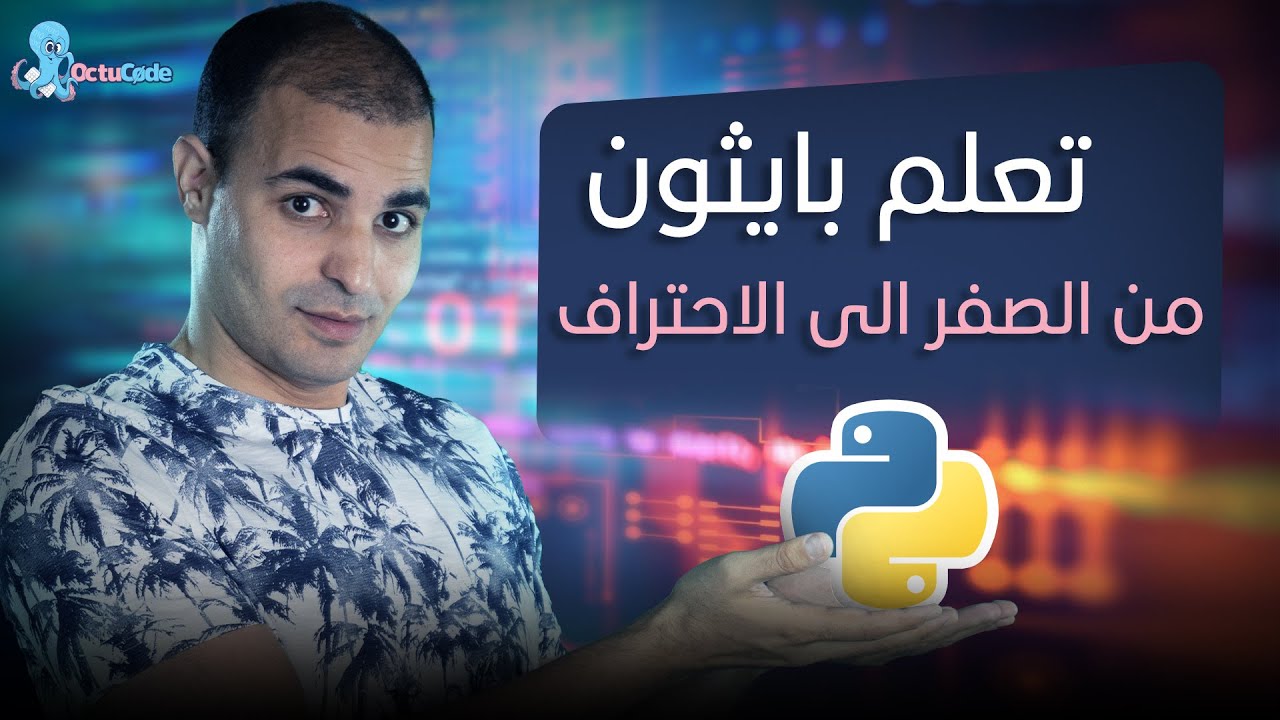
تعلم بايثون من الصفر الى الاحتراف : كورس بايثون كامل للمبتدئين مجانا: ١
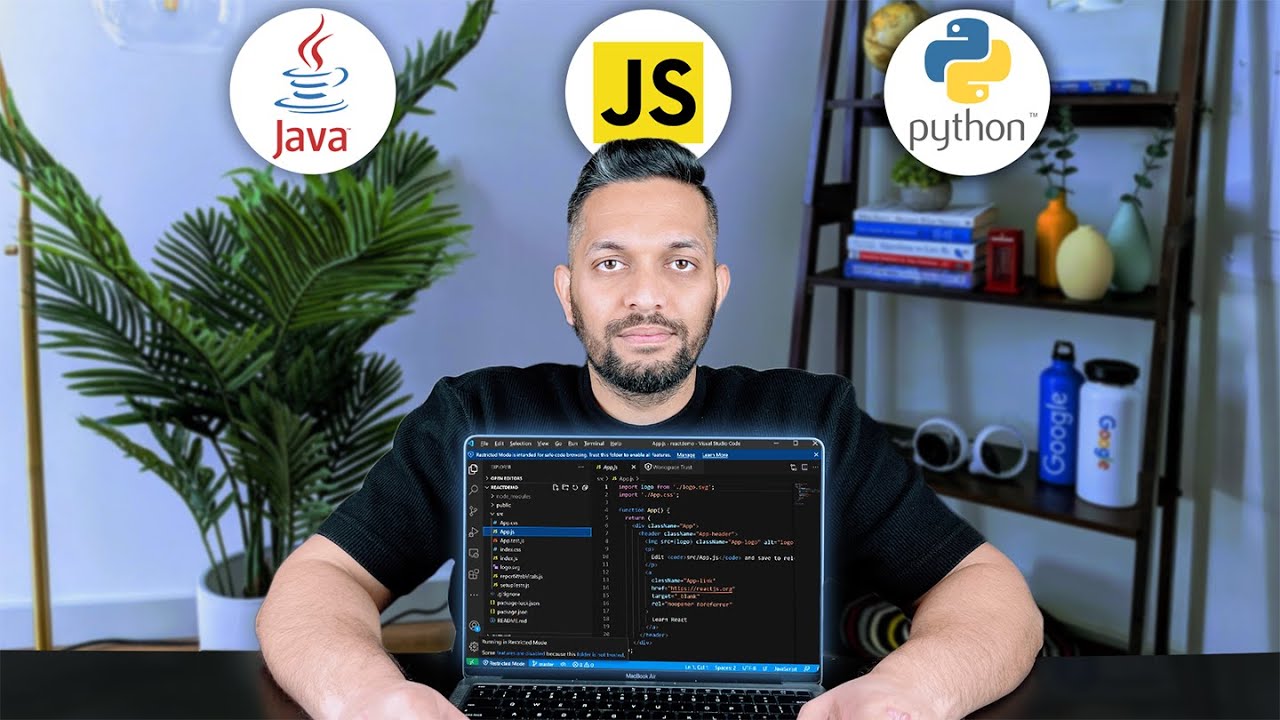
3 Developer Roadmaps That Actually Work
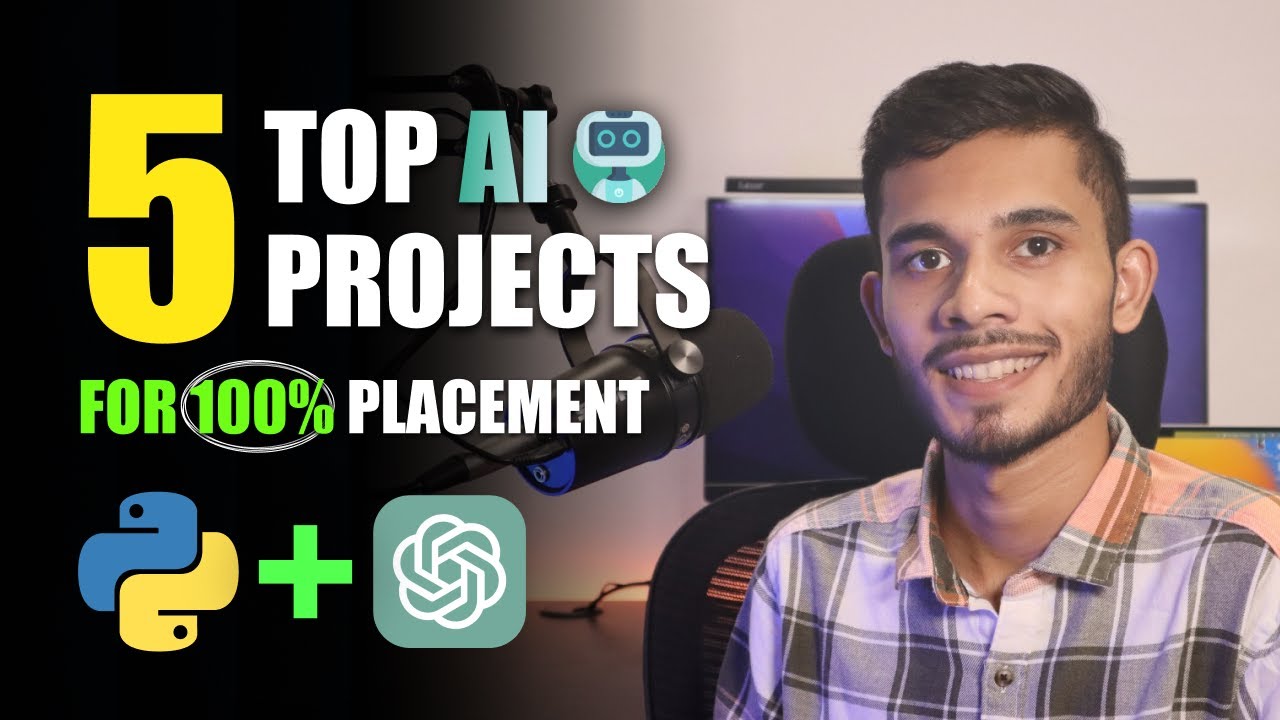
Top 5 Artificial Intelligence Project Ideas 2023 | Best AI Projects Ideas For 100% Placement
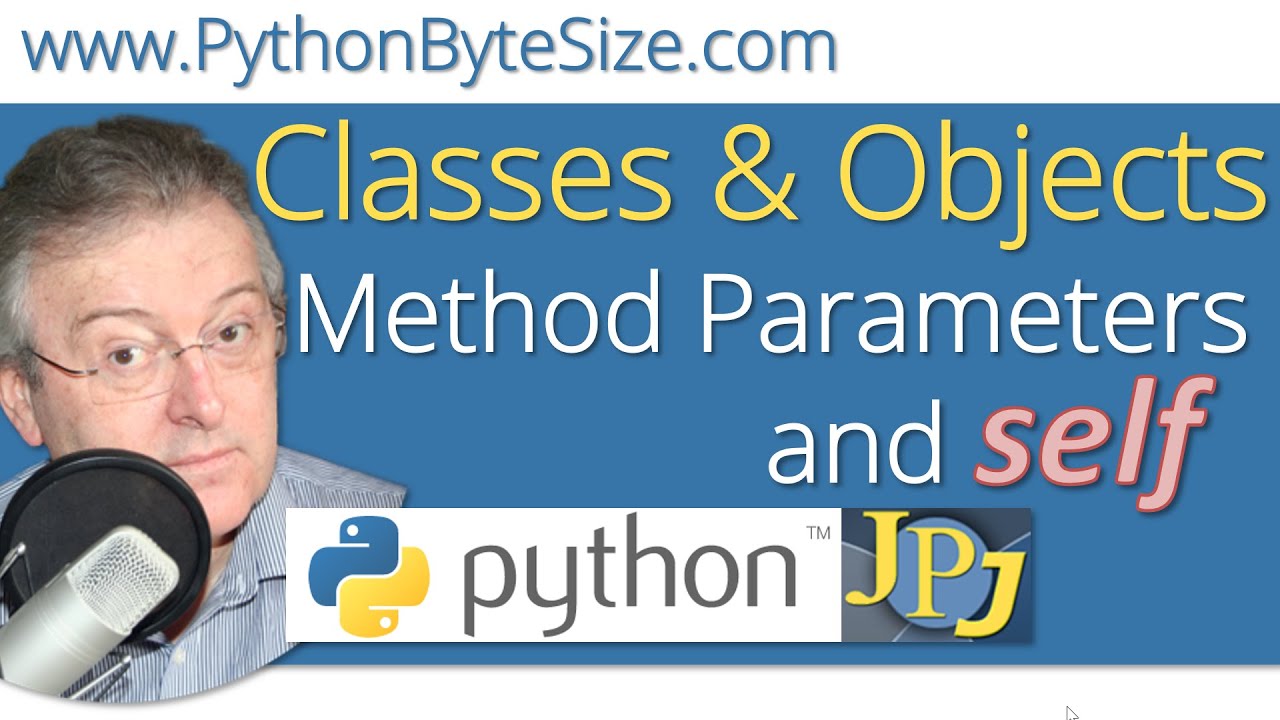
Python Method Parameters and self
5.0 / 5 (0 votes)