#19 C Arrays | C Programming For Beginners
Summary
TLDRIn this C programming tutorial, you'll learn how to work with arrays, a powerful way to store multiple values of the same type in a single variable. The video covers array declaration, initialization, accessing elements, and modifying values using both direct assignment and user input. Additionally, it explains the concept of array indices, the importance of staying within array bounds, and how to use loops for efficient array manipulation. The tutorial concludes with a programming task and quiz to test your understanding of arrays.
Takeaways
- 😀 Arrays in C are used to store multiple values of the same data type in a single variable.
- 😀 You can declare an array by specifying the data type, array name, and size (number of elements).
- 😀 Once declared, the size and data type of an array cannot be changed.
- 😀 Array elements can be assigned values at declaration or later using the array index.
- 😀 Array indexing starts at 0, so the first element is accessed at index 0.
- 😀 When assigning values to an array, the values are stored in sequential memory locations.
- 😀 Arrays can be initialized without specifying the size, and the compiler automatically determines it based on the number of elements.
- 😀 Accessing an array element requires specifying the array name and its index inside square brackets.
- 😀 We can modify array values by directly assigning new values to specific indices.
- 😀 To simplify input and output operations, loops can be used to process all elements of an array, such as taking input or printing values.
- 😀 It’s important to avoid accessing array elements outside its bounds, as this leads to unpredictable behavior or errors.
Q & A
What is an array in C programming?
-An array is a collection of elements of the same data type, stored in contiguous memory locations. It allows you to store multiple values under a single variable name.
Why should we use arrays instead of individual variables?
-Using arrays simplifies code and reduces redundancy. For example, if you need to store data for 100 people, it's impractical to create 100 separate variables. Arrays allow you to store all the values in one place.
How do you declare an array in C?
-To declare an array, you specify the data type, the array name, and the size of the array. For example: `int ages[5];` declares an array of integers with 5 elements.
Can you change the size of an array after it is declared in C?
-No, in C, once an array is declared with a specific size, it cannot be resized or its data type changed. The size and type must remain fixed.
What happens if you omit the size in an array declaration?
-If you omit the size, the compiler will automatically determine the size based on the number of elements provided in the initialization. For example: `int ages[] = {21, 29, 25, 32, 17};` will create an array of 5 integers.
How are elements accessed in an array?
-Array elements are accessed using an index. Array indices in C start from 0. For example, `ages[0]` accesses the first element of the array.
What is an array index, and how does it work?
-An array index is the position of an element within an array. In C, array indices start from 0, meaning the first element is at index 0, the second at index 1, and so on.
How can you modify an element in an array?
-You can modify an array element by directly assigning a new value to a specific index. For example: `ages[2] = 26;` changes the value at index 2 of the `ages` array.
Why is it important not to exceed the bounds of an array?
-Accessing an array element outside its bounds (i.e., using an index that is greater than or equal to the array size) leads to undefined behavior, which can cause errors or unexpected results in your program.
How can you improve efficiency when working with arrays and loops in C?
-Instead of using individual `scanf` and `printf` statements for each array element, you can use loops to process array elements efficiently. A `for` loop can handle input and output for all array elements sequentially.
Outlines
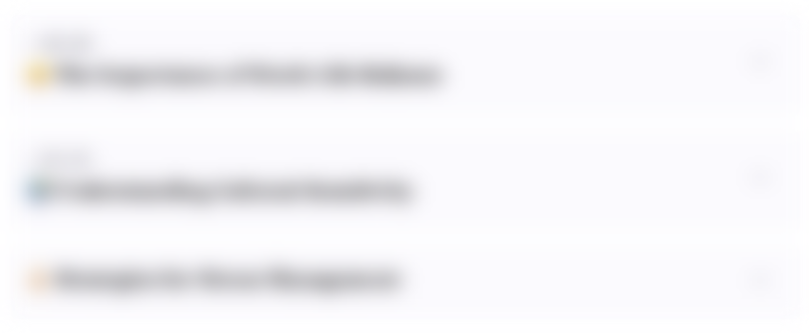
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
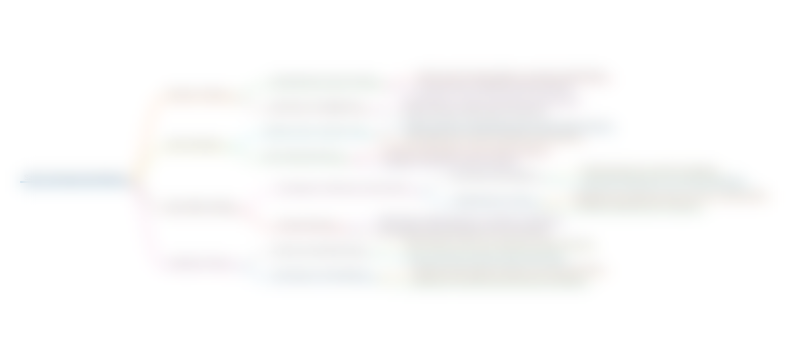
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
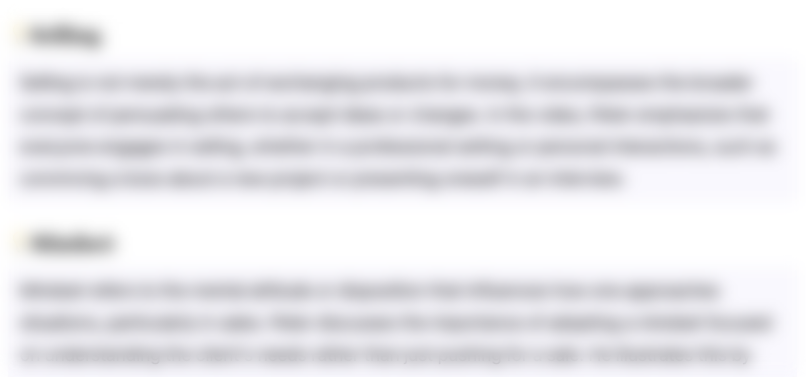
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
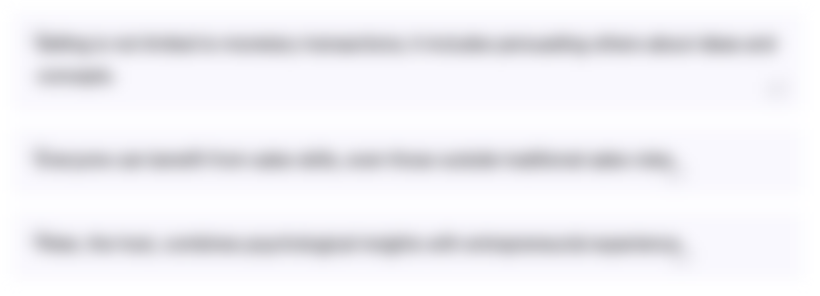
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
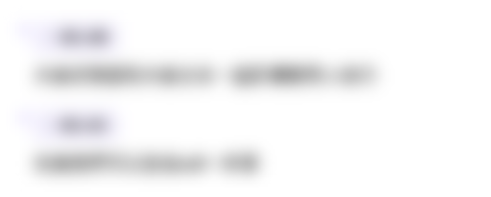
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes
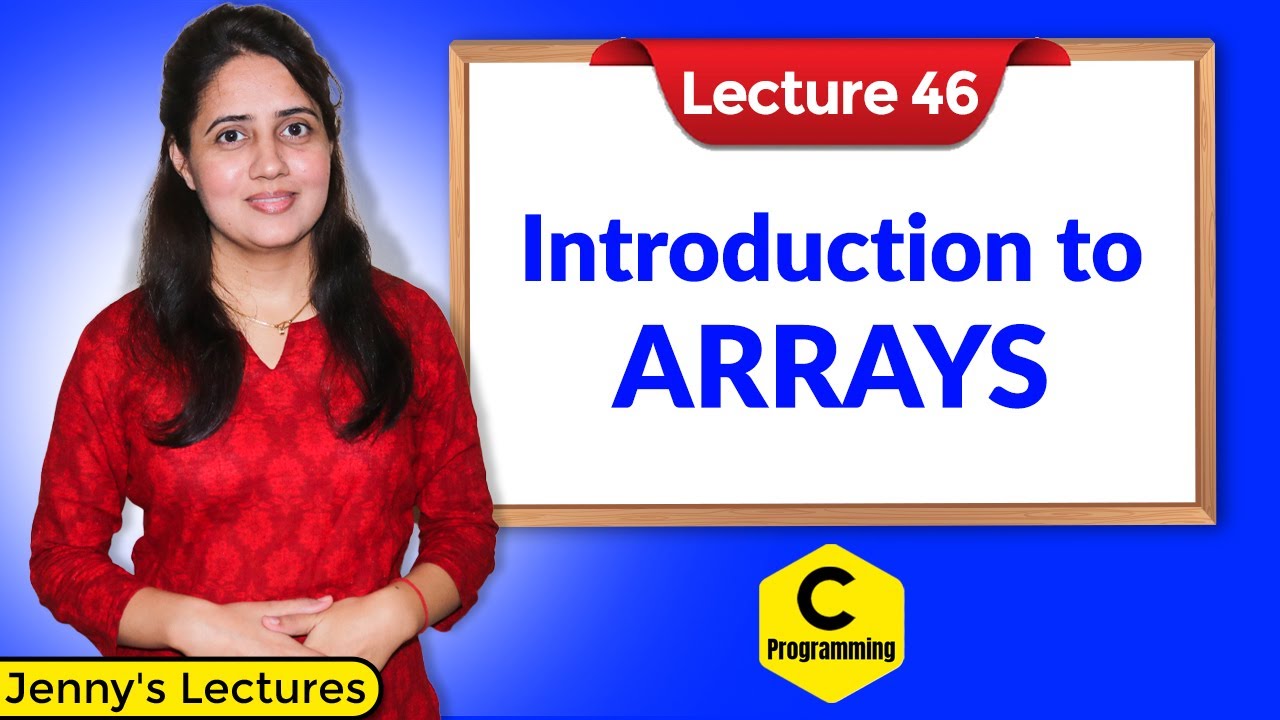
C_46 Arrays in C - part 1 | Introduction to Arrays
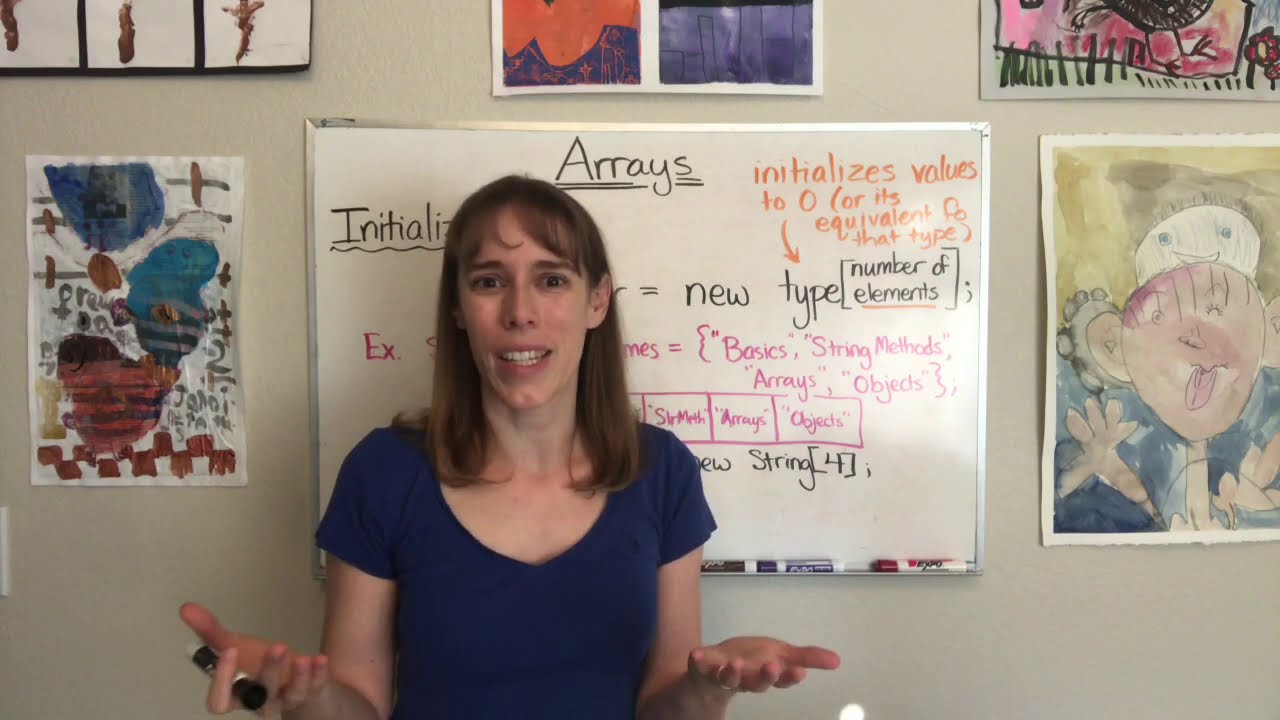
Intro to Arrays
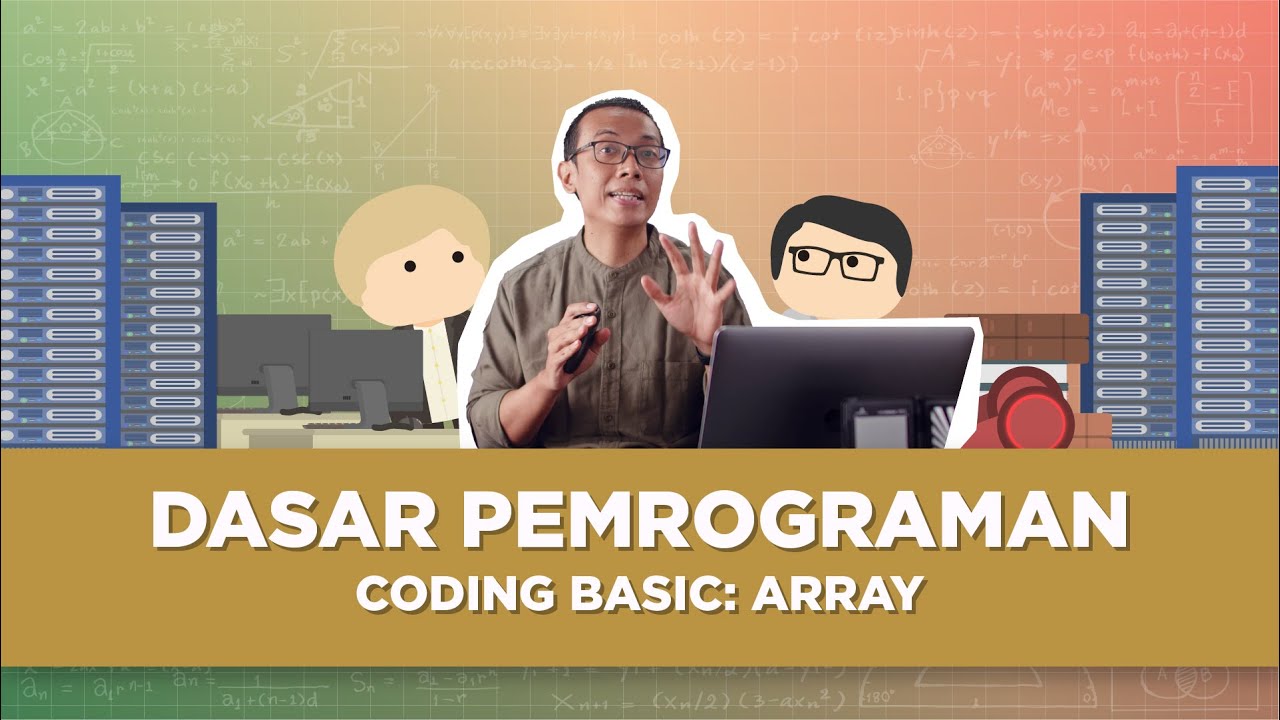
ARRAY pada Programming
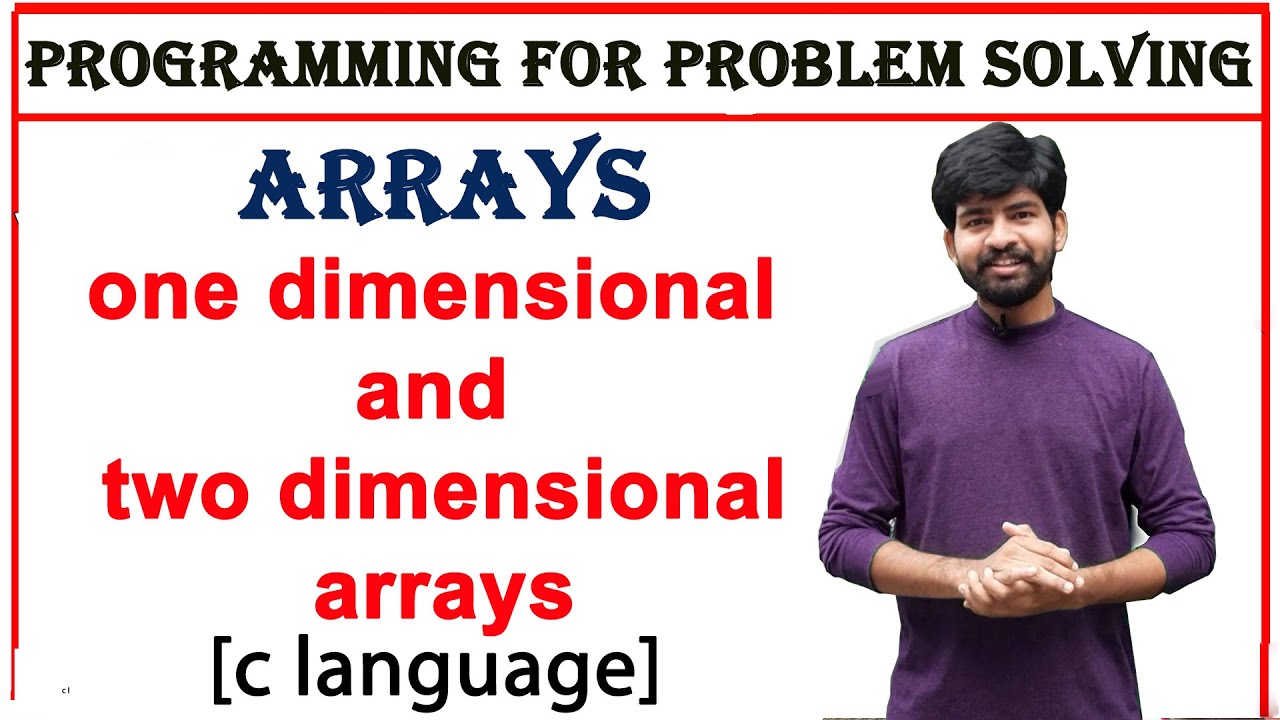
arrays in c, one dimensional array, two dimensional array |accessing and manipulating array elements
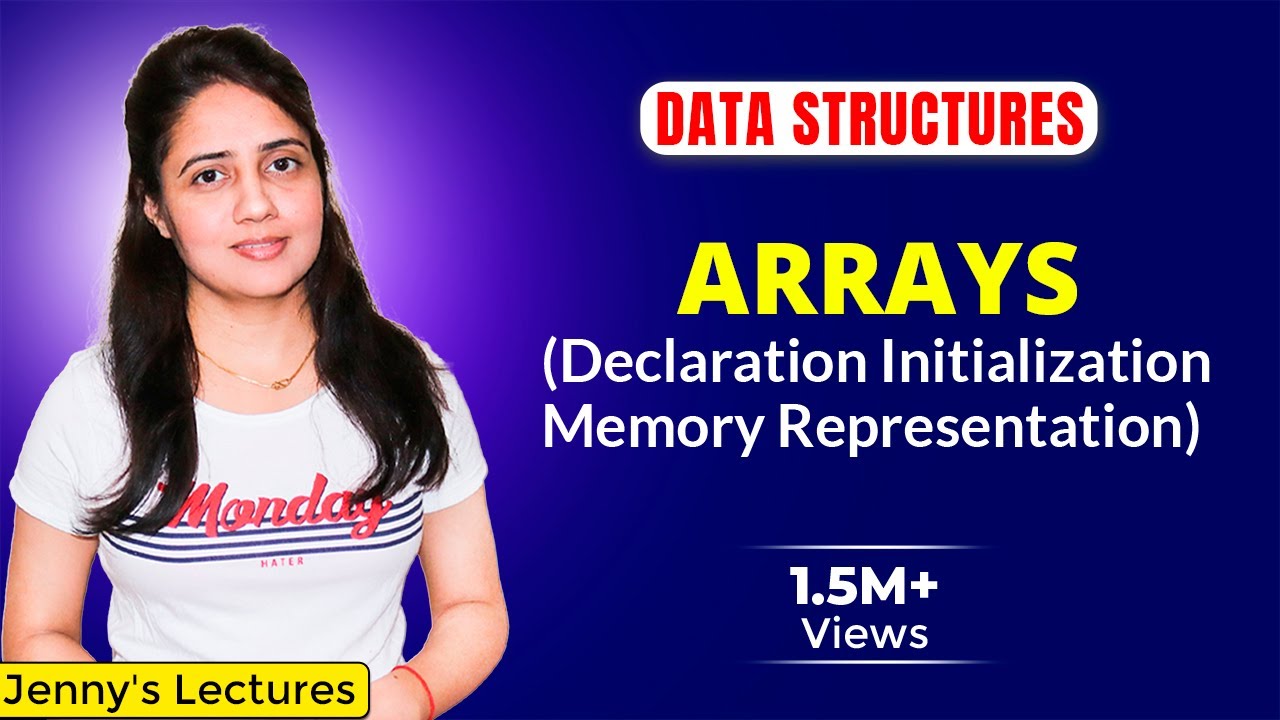
1.1 Arrays in Data Structure | Declaration, Initialization, Memory representation
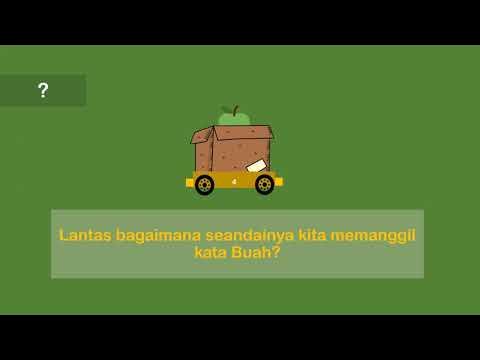
Pengenalan Array di Struktur Data
5.0 / 5 (0 votes)