C 2D arrays ⬜
Summary
TLDRThis video explains the concept of two-dimensional arrays in C programming. It covers how to create, initialize, and manipulate these arrays, which are useful for representing grids, tables, or matrices. The video demonstrates how to define a two-dimensional array, assign values using indices, and access array elements through nested loops. It also addresses dynamic size calculation using the 'sizeof' operator and how to update the array’s size. With practical examples, the video emphasizes the flexibility and functionality of two-dimensional arrays for handling structured data in programming.
Takeaways
- 😀 A two-dimensional array is an array of arrays, useful for storing structured data like matrices, grids, or tables.
- 😀 To create a two-dimensional array, you need two sets of square brackets: one for rows and one for columns.
- 😀 You can initialize a two-dimensional array with specific values, such as a 2x3 matrix: {{1, 2, 3}, {4, 5, 6}}.
- 😀 Arrays can have a fixed size for rows and columns, but you must specify the maximum number of elements per row.
- 😀 When accessing an element in a two-dimensional array, use two indices: one for the row and one for the column.
- 😀 Looping through a two-dimensional array can be done with nested `for` loops, where the outer loop handles rows and the inner loop handles columns.
- 😀 You can initialize a two-dimensional array without assigning values immediately and later assign values using two indices.
- 😀 The `sizeof` operator can be used to dynamically calculate the number of rows and columns in the array.
- 😀 If the size of the array changes (e.g., adding more rows), you can dynamically calculate the new dimensions using `sizeof`.
- 😀 A practical example shows how to initialize, modify, and display a 2D array with values like a 3x3 matrix.
- 😀 The `printf` function can be used to display the elements of a two-dimensional array, printing each row on a new line.
Q & A
What is a two-dimensional array in C?
-A two-dimensional array in C is an array of arrays, where each element is itself an array. It is useful for representing matrices, grids, or tables of data.
How do you declare a two-dimensional array in C?
-A two-dimensional array is declared using two sets of square brackets, the first for the number of rows and the second for the number of columns. For example: 'int numbers[2][3];' represents an array with 2 rows and 3 columns.
How do you initialize a two-dimensional array in C?
-To initialize a two-dimensional array, you use curly braces to define the rows and columns. For example: 'int numbers[2][3] = {{1, 2, 3}, {4, 5, 6}};' initializes a 2x3 array.
Can you specify the size of each row in a two-dimensional array in C?
-Yes, when declaring a two-dimensional array, you must specify the size of each row. For example, 'int numbers[2][3];' sets each row to have a maximum of 3 elements.
How do you access an element in a two-dimensional array?
-To access an element in a two-dimensional array, you use two indices: one for the row and one for the column. For example: 'numbers[0][1]' accesses the element in the first row, second column.
What are nested loops used for in relation to two-dimensional arrays?
-Nested loops are used to iterate over all elements of a two-dimensional array. The outer loop handles the rows, and the inner loop handles the columns. This allows you to access every element in the array.
How do you modify an element in a two-dimensional array?
-You modify an element by accessing it using its row and column indices and assigning a new value. For example: 'numbers[1][0] = 7;' changes the element in the second row, first column to 7.
How can you calculate the number of rows and columns dynamically in C?
-You can use the 'sizeof' operator to calculate the number of rows and columns. For example: 'int rows = sizeof(numbers) / sizeof(numbers[0]);' calculates the number of rows, and 'int columns = sizeof(numbers[0]) / sizeof(int);' calculates the number of columns.
What happens if you change the dimensions of the array?
-If the dimensions of the array change, the array needs to be reinitialized, and the loops must be adjusted to reflect the new number of rows and columns.
What is the significance of zero-based indexing in two-dimensional arrays?
-In C, arrays are zero-indexed, meaning that both the row and column indices start at 0. This affects how you access and modify array elements, with the first row being 'numbers[0]' and the first column being 'numbers[0][0]'.
Outlines
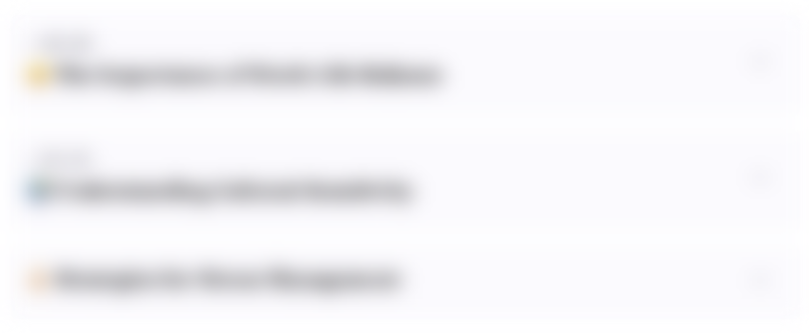
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
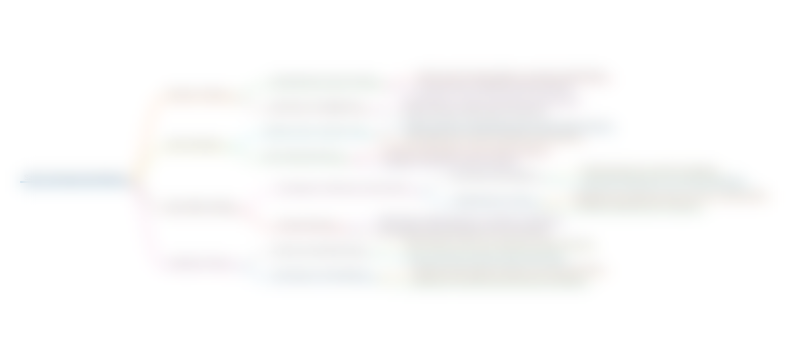
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
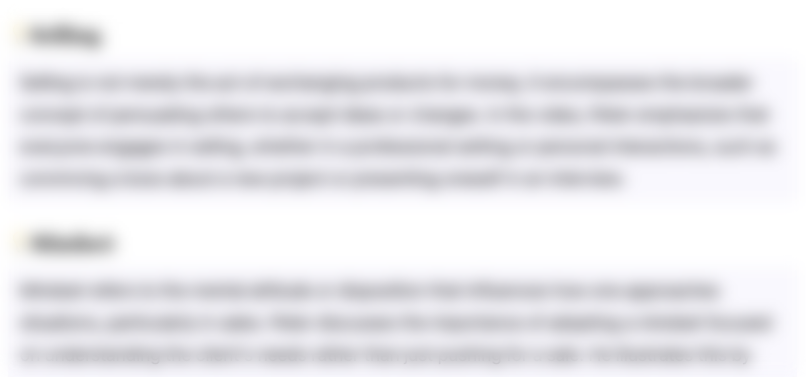
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
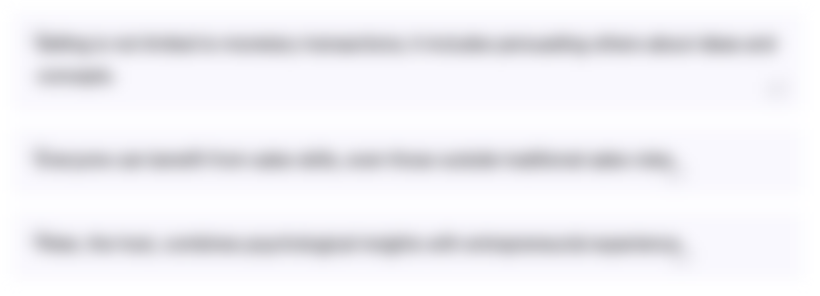
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
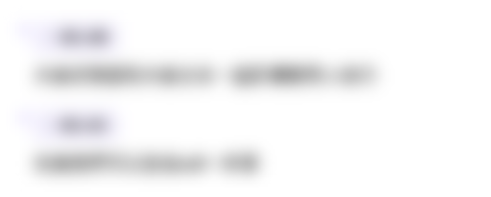
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
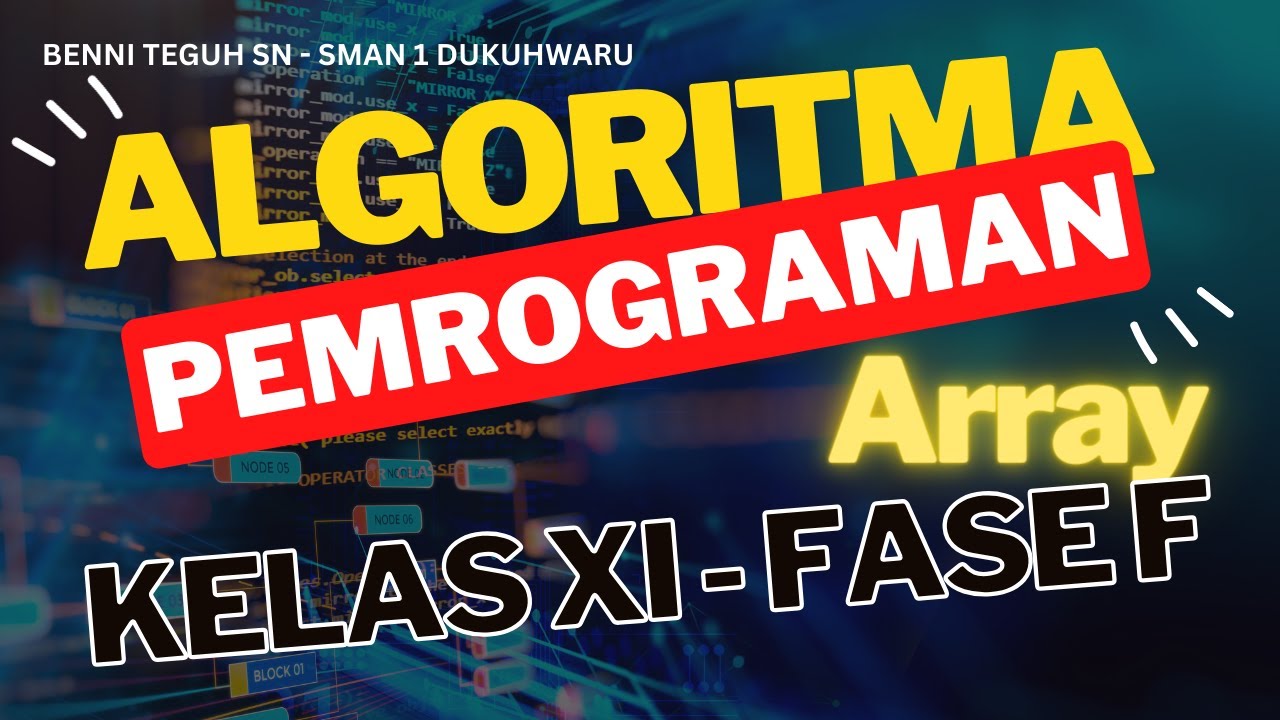
Larik (Array) - Algoritma dan Pemrograman
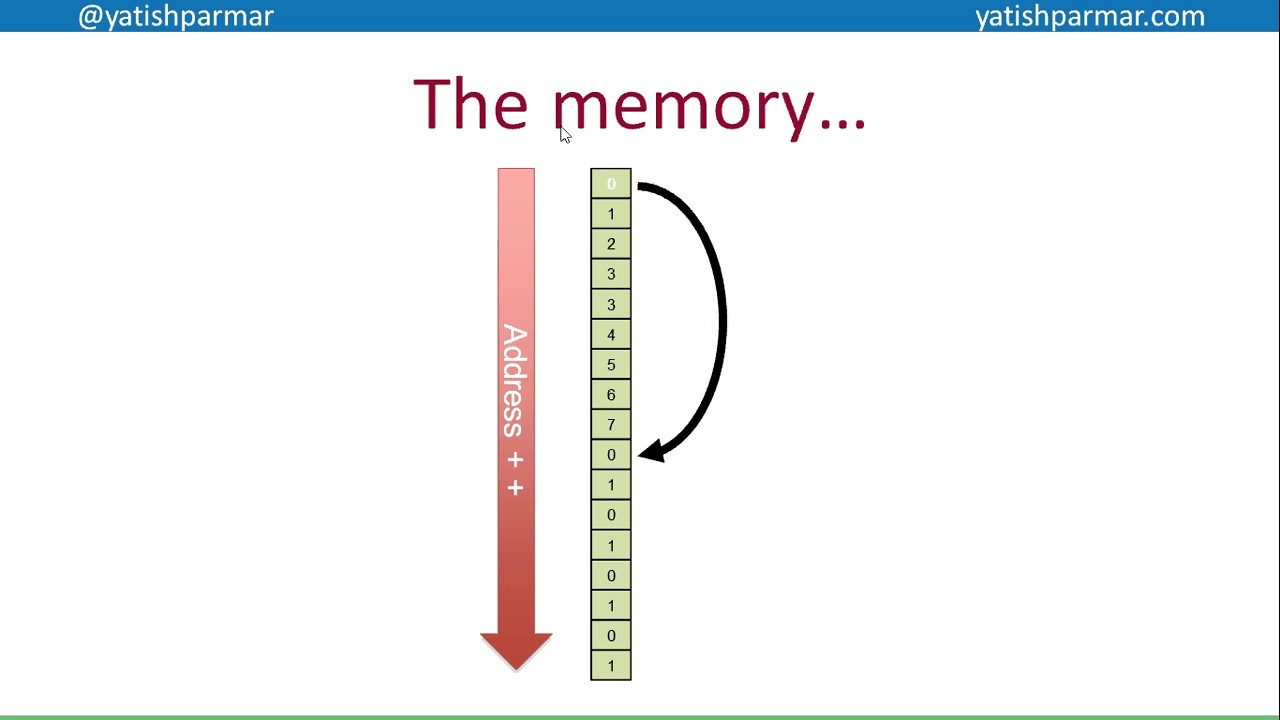
2D arrays - A Level Computer Science
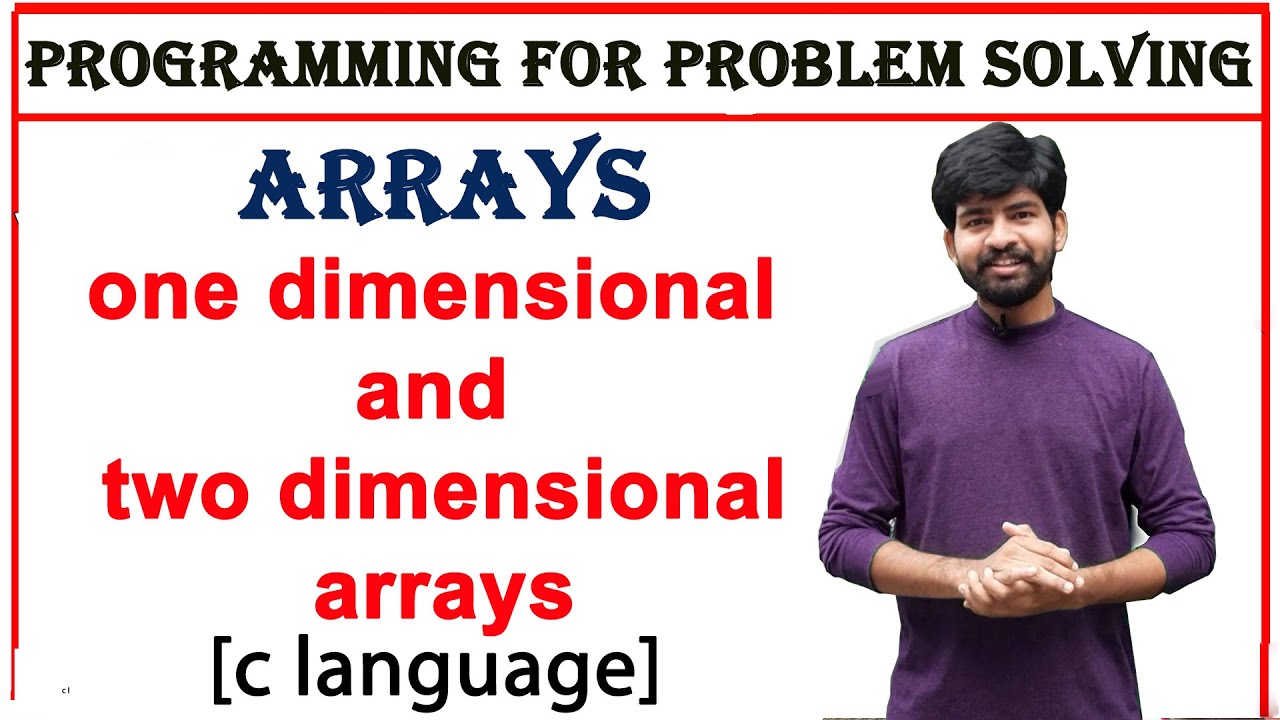
arrays in c, one dimensional array, two dimensional array |accessing and manipulating array elements
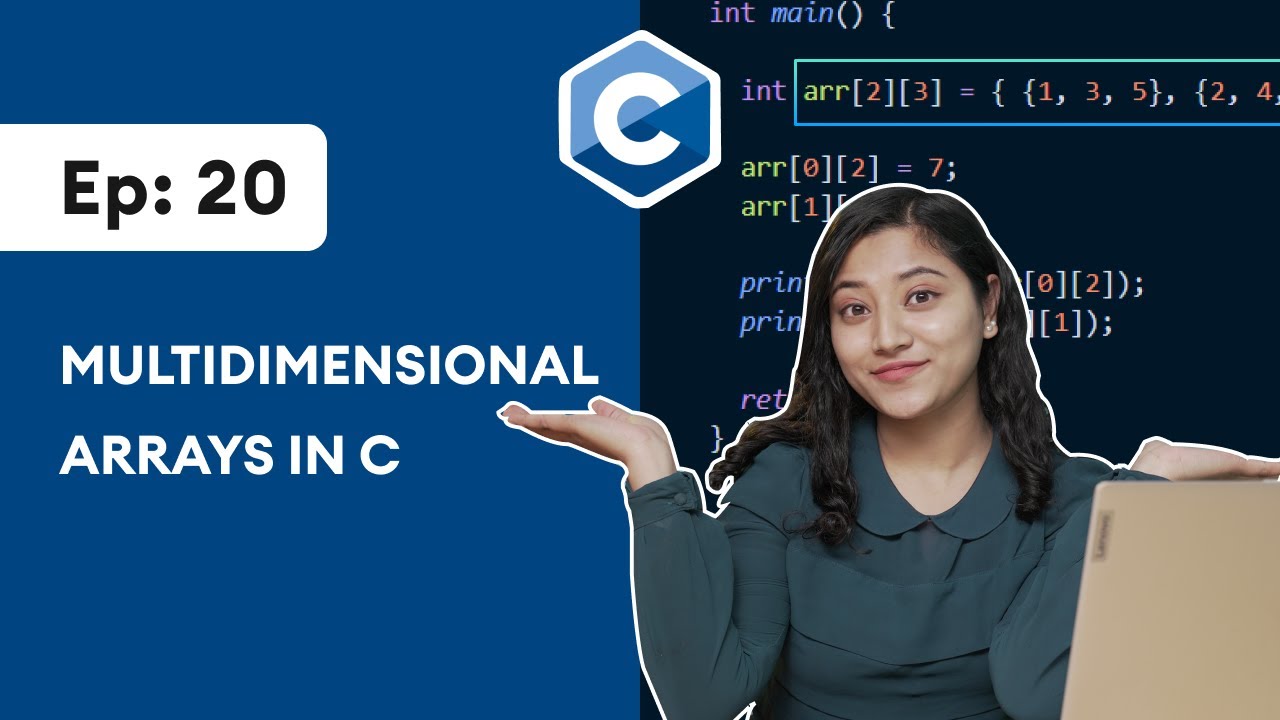
#20 C Multidimensional Arrays | C Programming For Beginners

NumPy Python - What is NumPy in Python | Numpy Python tutorial in Hindi
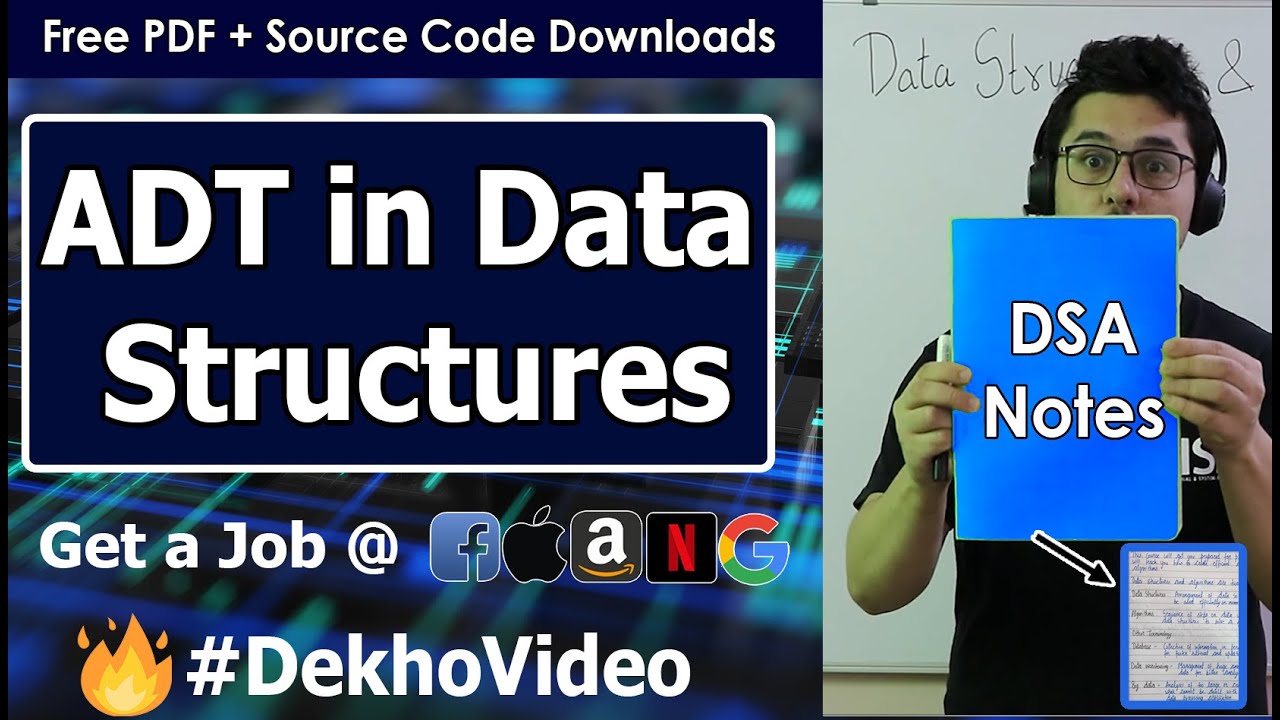
Arrays and Abstract Data Type in Data Structure (With Notes)
5.0 / 5 (0 votes)