1.1 Arrays in Data Structure | Declaration, Initialization, Memory representation
Summary
TLDRThis video delves into the fundamentals of arrays in programming, focusing on their declaration, memory representation, and methods of initialization. It covers the basic structure of arrays, explaining how they store multiple values of the same data type in consecutive memory locations. The video emphasizes array size limitations, introduces dynamic memory allocation, and discusses accessing array elements using indices. The importance of fixed-size arrays and the efficiency of random access are highlighted, alongside practical demonstrations of compile-time and runtime initialization. This content provides a thorough introduction to one-dimensional arrays, ideal for beginners.
Takeaways
- đ An array is a collection of elements of the same data type, useful for storing multiple values under a single variable name.
- đ To declare an array in C, specify the data type, array name, and size (constant), e.g., `int a[60];`.
- đ Arrays can be initialized at compile time (static initialization) by directly assigning values during declaration.
- đ At runtime, arrays can be initialized by taking input from the user, typically using loops and functions like `scanf`.
- đ Memory for an array is allocated contiguously, with each element occupying a fixed number of bytes depending on the data type.
- đ The address of each array element can be calculated using the formula: `base_address + (index * size_of_data_type)`.
- đ Array indexing starts from 0 in C, and elements can be accessed in constant time `O(1)` by specifying the index.
- đ Arrays have a fixed size that cannot be changed at runtime, which may lead to wasted memory if the array is larger than needed.
- đ If more space is required for an array, a new memory block is allocated, and the existing data is copied to the new location.
- đ The key limitation of arrays is that they cannot dynamically adjust their size after declaration, potentially causing inefficiencies.
- đ Although arrays are efficient for accessing elements, other data structures like linked lists may be used for dynamic size allocation.
Q & A
What is an array in programming?
-An array is a collection of elements of the same data type, stored in contiguous memory locations. It allows you to store multiple values under a single variable, making it easier to manage and access related data.
How do you declare an array in C?
-In C, an array is declared by specifying the data type, followed by the array name and the size. For example, to declare an array of 5 integers: `int a[5];`.
How does memory representation of an array work?
-Memory for an array is allocated in consecutive locations. For example, if an array has 5 elements of type `int`, and each `int` takes 4 bytes, the memory manager will allocate 20 bytes in consecutive memory locations.
How is the address of an array element calculated?
-The address of an element in an array is calculated using the formula: `Address of a[i] = Base address + (i * size of element)`. The base address is where the array starts in memory, and `i` is the index of the element.
What are the types of arrays?
-Arrays can be one-dimensional (1D), two-dimensional (2D), or multi-dimensional. A 1D array stores a single row of elements, while a 2D array stores data in rows and columns, and multi-dimensional arrays store data across more than two dimensions.
What is the difference between compile-time and runtime array initialization?
-Compile-time initialization happens when you specify the values of the array at the time of declaration. For example: `int a[5] = {1, 2, 3, 4, 5};`. Runtime initialization occurs during program execution, where values are input by the user or generated dynamically.
Can you change the size of an array at runtime in most programming languages?
-No, arrays in most programming languages, such as C, have a fixed size once declared. To change the size, you would typically need to allocate a new array and copy the old values to it.
What is a major drawback of static arrays?
-A major drawback of static arrays is that their size is fixed at compile-time. If you allocate too much space, you waste memory; if you allocate too little, you cannot store additional elements.
How is random access possible in arrays?
-Arrays provide random access, meaning any element can be accessed directly using its index. Since the elements are stored in contiguous memory locations, accessing any element takes constant time, O(1).
Why can't arrays store elements of different data types?
-Arrays cannot store elements of different data types because they are designed to hold elements of the same data type. Mixing different data types would violate the array's structural consistency, which is why arrays are type-specific.
Outlines
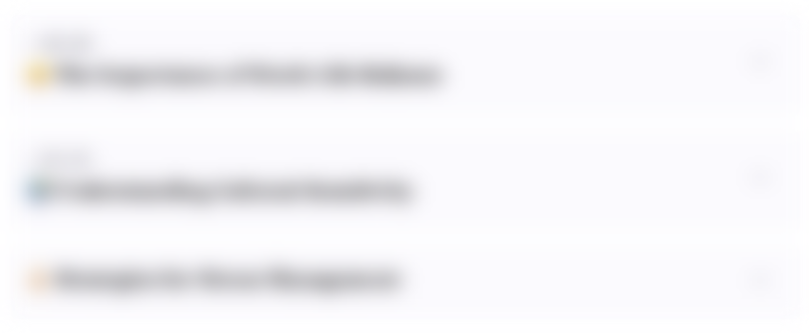
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
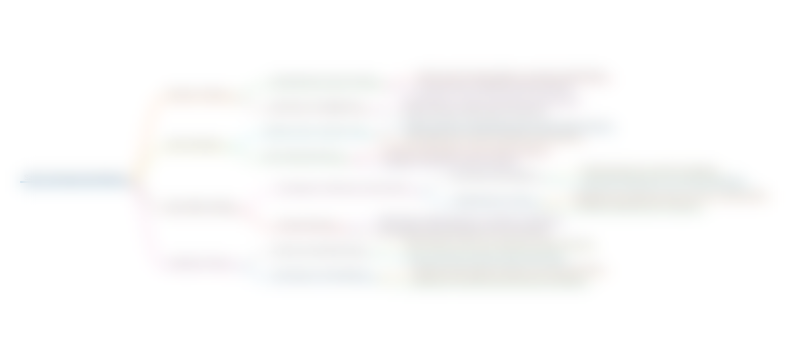
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
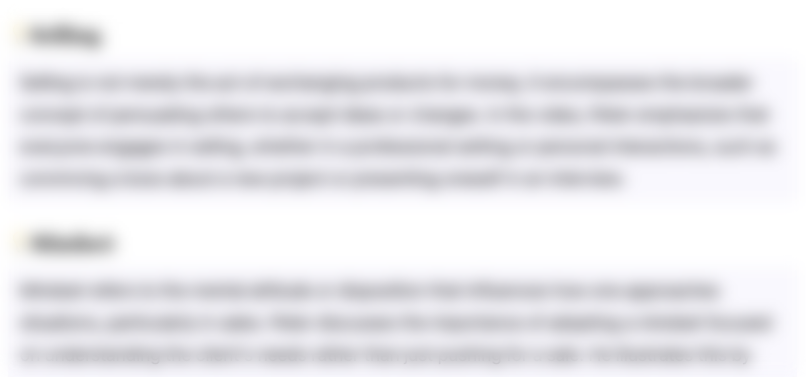
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
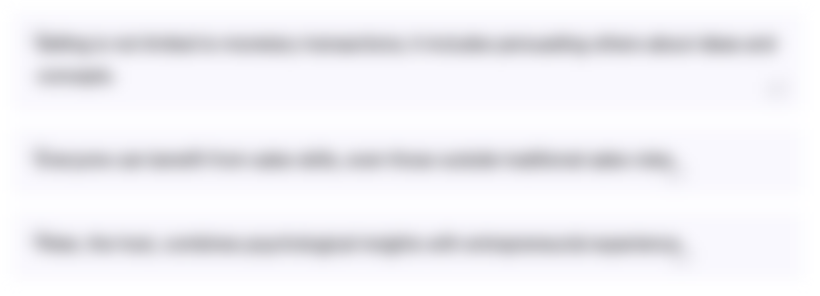
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
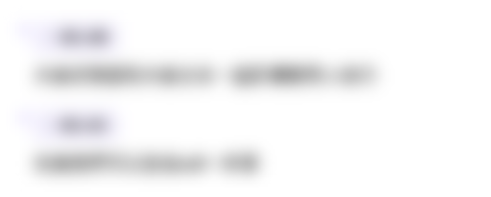
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes
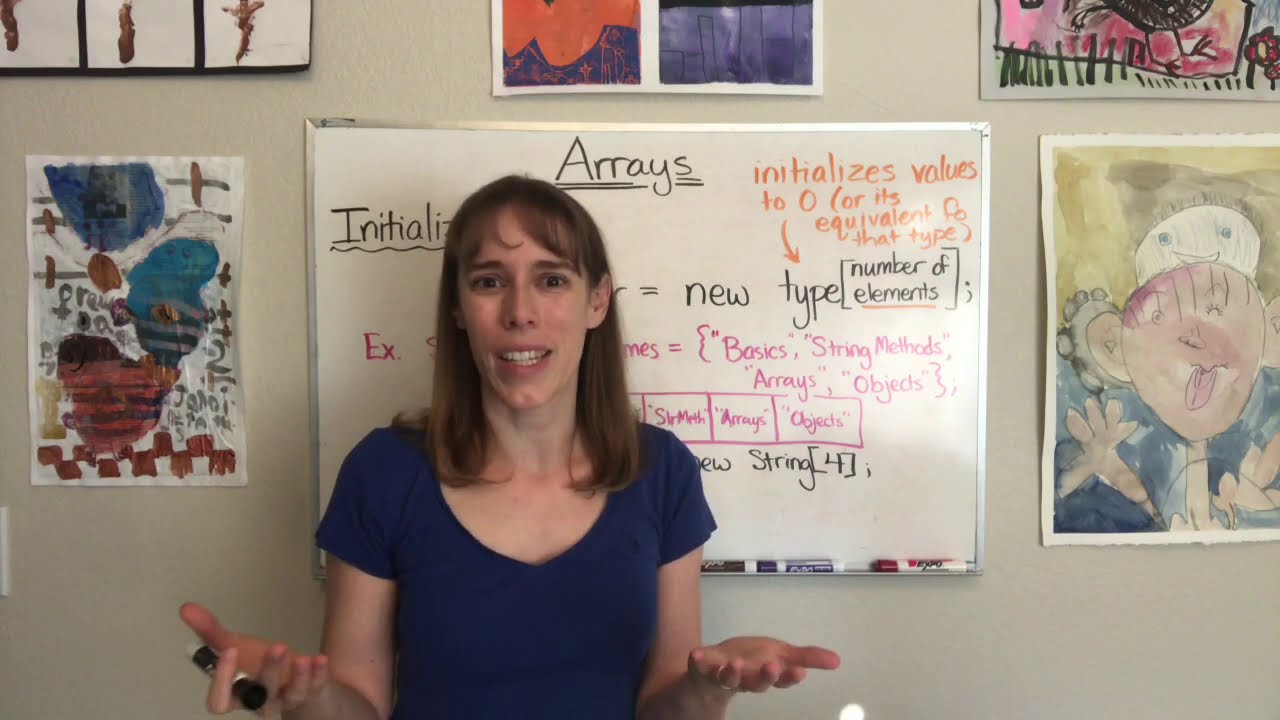
Intro to Arrays
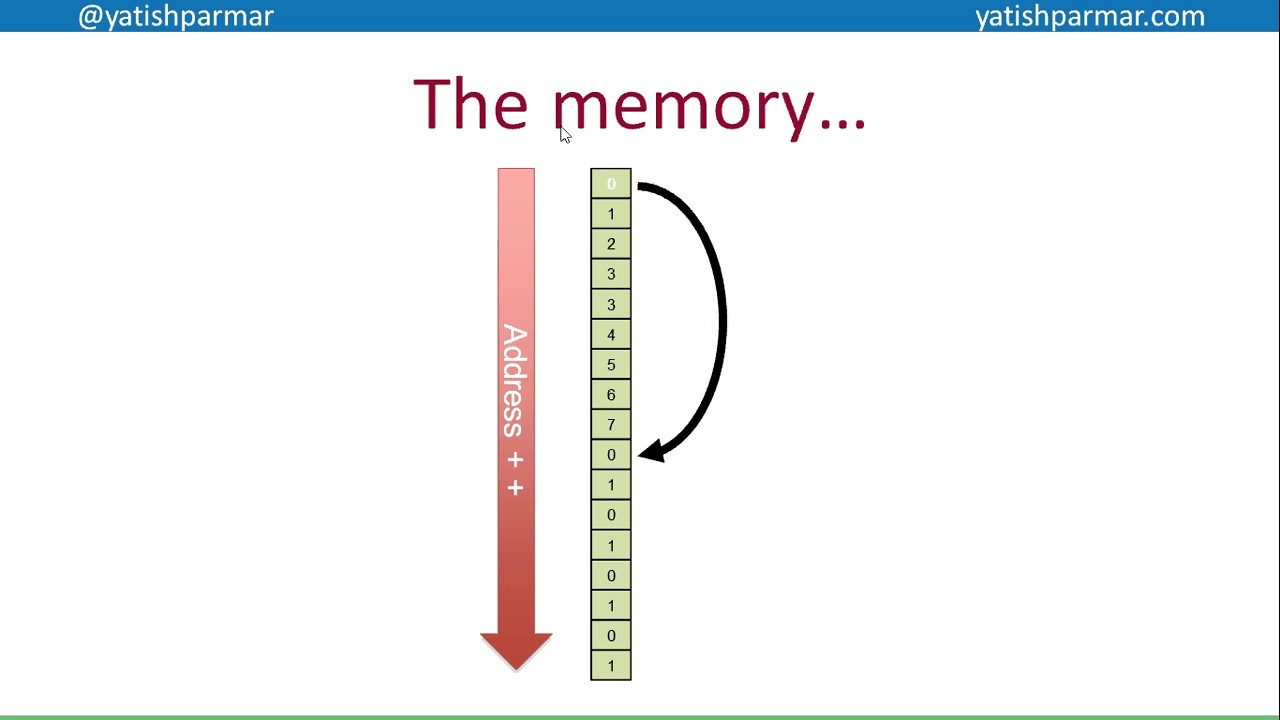
2D arrays - A Level Computer Science
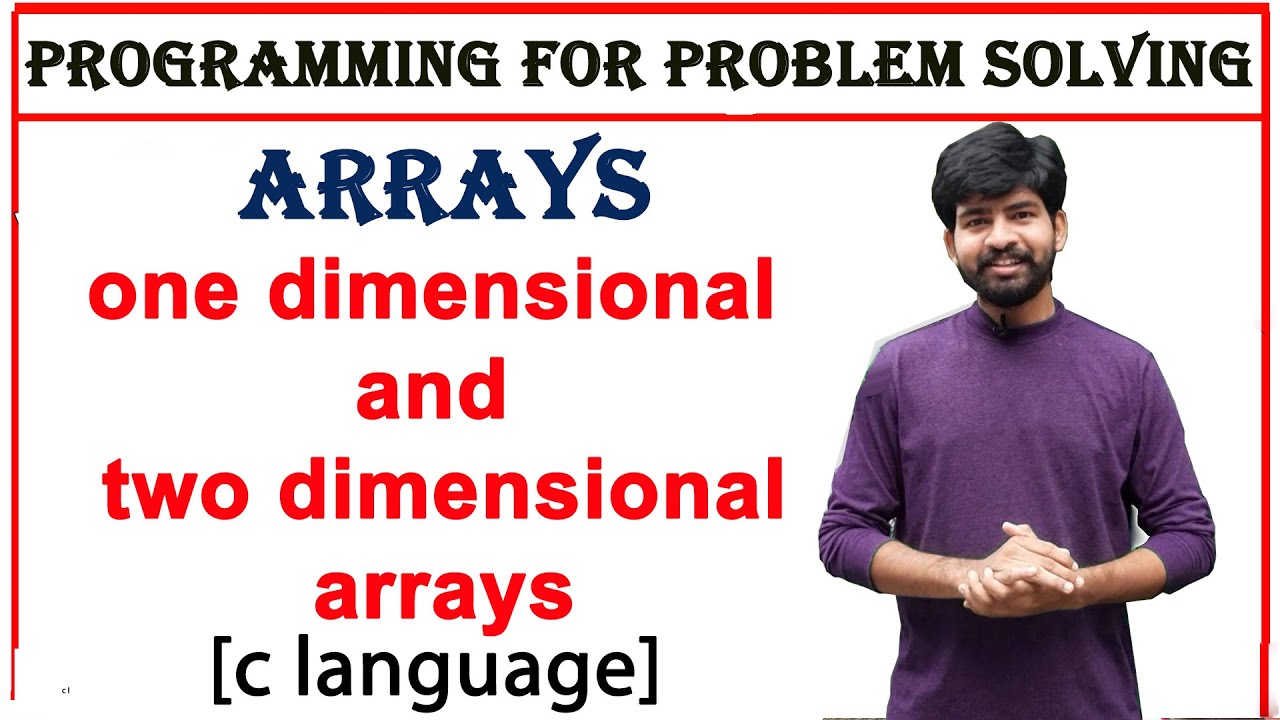
arrays in c, one dimensional array, two dimensional array |accessing and manipulating array elements
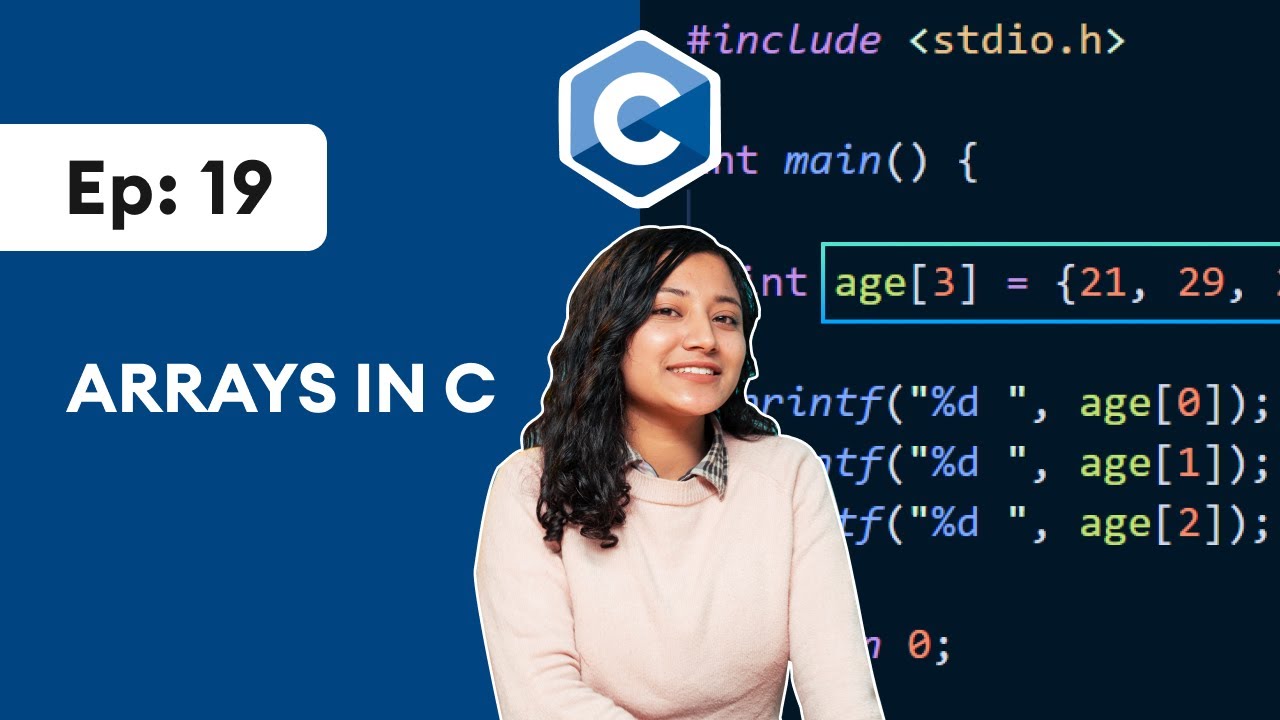
#19 C Arrays | C Programming For Beginners

9.1: What is an Array? - Processing Tutorial
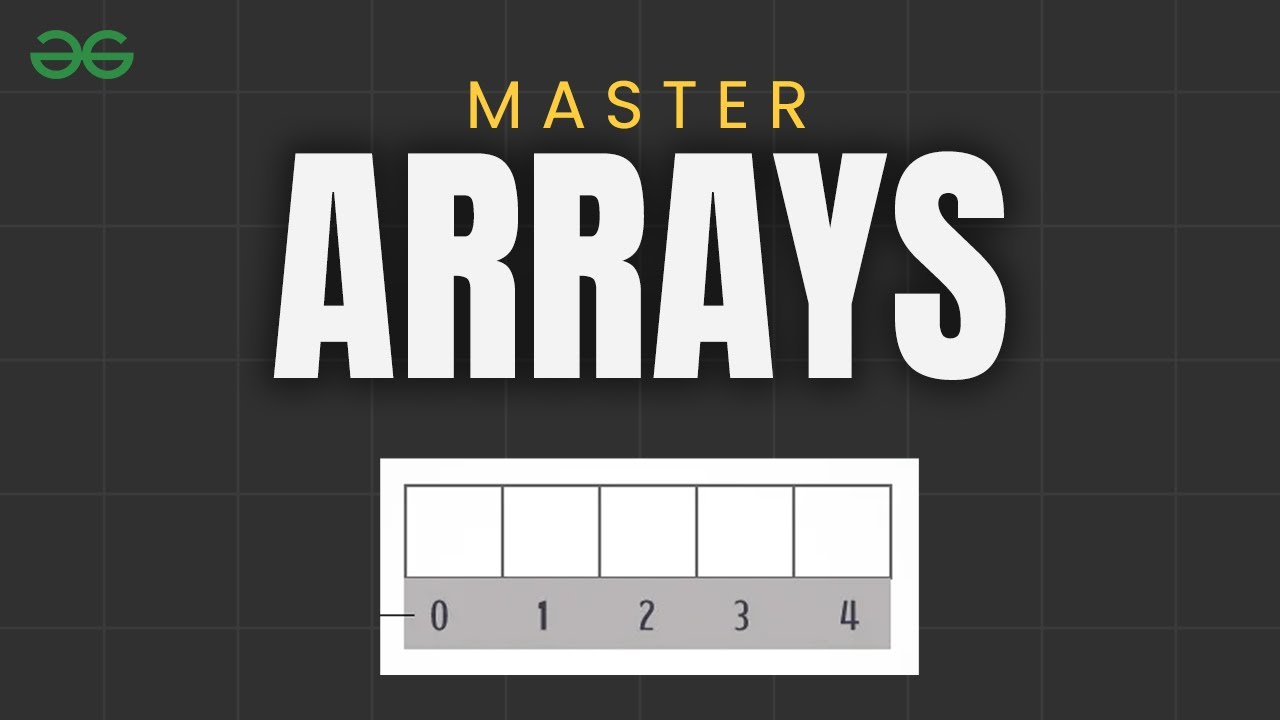
WHAT IS ARRAY? | Array Data Structures | DSA Course | GeeksforGeeks
5.0 / 5 (0 votes)