The Pattern You MUST Learn in .NET
Summary
TLDRThe video introduces the transactional outbox pattern, an important design pattern in messaging and microservices. It allows atomic operations between a database and a message queue by using a database transaction to make changes and write events to an outbox table. A background process then pushes those events to a message queue. The video demonstrates implementing this pattern simply in .NET with MassTransit to achieve reliable messaging. It also discusses alternatives like distributed transactions and the presenter's preferred approach of using serverless architectures.
Takeaways
- 💬 The video introduces the transactional outbox pattern in messaging within .NET applications, focusing on its importance for ensuring reliable message delivery in distributed systems.
- 🔥 Demonstrates the use of Amazon SQS (Simple Queue Service) and MassTransit for implementing the outbox pattern, but emphasizes that the approach is not limited to SQS and can work with other messaging services like RabbitMQ or Azure Service Bus.
- 📦 Explains that the outbox pattern helps solve the problem of ensuring that a database operation and a message publication to a queue are treated as a single atomic operation, preventing data inconsistencies.
- 🛠️ Outlines the steps to implement the outbox pattern in .NET, including the addition of specific MassTransit and Entity Framework Core packages and configuration changes in the application.
- 📈 Uses a practical example of a customer API to show how the outbox pattern can be applied to a real-world scenario, including creating, updating, and deleting customer records and publishing messages related to these events.
- 💻 Highlights the importance of idempotence in message processing to handle potential duplicate messages that could arise due to the nature of distributed systems and the outbox pattern.
- ✅ Provides code snippets and explanations for setting up the transactional outbox pattern, including database migrations for outbox-related tables and configuring MassTransit to use these tables.
- 📚 Mentions the availability of a complete code example in the video description, offering viewers a resource to explore the implementation details further.
- 📲 Discusses alternative approaches to solving the problem addressed by the outbox pattern, hinting at the presenter's preference for a different method based on his experience with cloud and serverless architectures.
- ❓ Encourages viewers to share their own experiences and approaches to implementing reliable messaging patterns in their .NET applications, fostering a community dialogue.
Q & A
What is the main topic of the video introduced by Nick?
-The main topic of the video is the transactional outbox pattern in messaging systems, particularly its application in .NET environments.
Why is the outbox pattern considered important in messaging systems?
-The outbox pattern is considered important because it ensures reliable messaging and transactional consistency between database operations and message queuing, which is crucial for distributed systems.
What messaging service is used as an example in the video?
-Amazon SQS (Simple Queue Service) is used as the queuing mechanism example in the video.
How does the transactional outbox pattern help in messaging systems?
-The transactional outbox pattern helps by storing messages in a database outbox as part of the same transaction as the business operation. This ensures that messages are reliably published to the message queue once the transaction commits, avoiding data inconsistencies.
What is MassTransit and how is it used in the context of the video?
-MassTransit is an abstraction layer over queueing mechanisms or pub/sub systems that simplifies messaging in .NET applications. In the video, it's used to demonstrate the implementation of the transactional outbox pattern with various transport layers, like Amazon SQS.
Why is the outbox pattern necessary, according to the video?
-The outbox pattern is necessary to ensure atomic operations between database changes and message publishing, solving the problem of ensuring both occur successfully or neither, to maintain data integrity across distributed systems.
What problem does the transactional outbox pattern solve regarding message publishing?
-It solves the problem of ensuring reliable message delivery even when an application is scaled out, by atomically storing messages in a database outbox before publishing them to the queue, thus avoiding lost messages or inconsistencies.
What alternative approaches to the transactional outbox pattern are mentioned?
-While specific alternatives are not detailed, the video mentions that there are other options if one does not want to use the outbox pattern, implying the existence of different messaging and transaction consistency strategies.
How does MassTransit support the transactional outbox pattern?
-MassTransit supports the transactional outbox pattern through integration with Entity Framework Core, allowing for the configuration of outbox and inbox state entities to manage message transactions and reliability.
What is idempotency, and why is it important in the context of the outbox pattern?
-Idempotency ensures that even if a message is delivered or processed multiple times, the effect on the system is the same as if it were processed once. It's important in the outbox pattern to handle duplicate messages without causing unintended effects.
Outlines
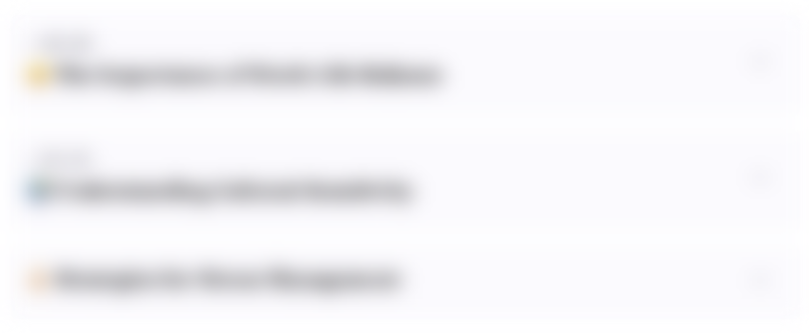
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
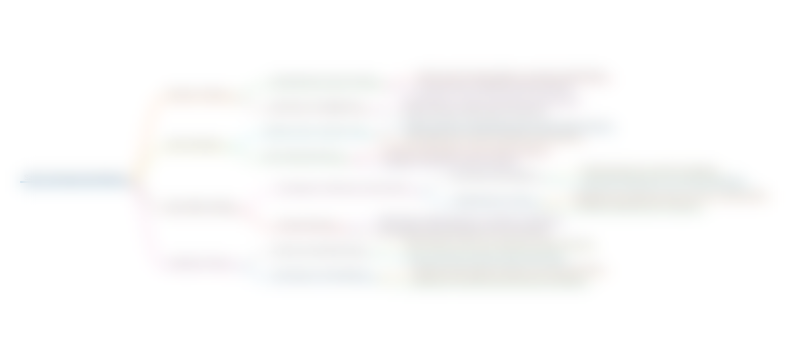
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
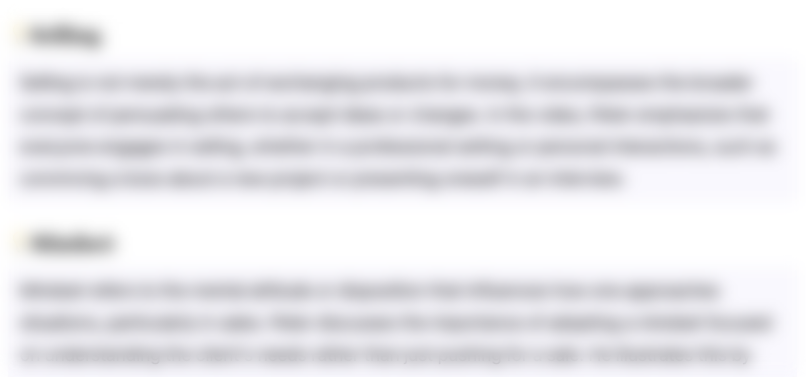
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
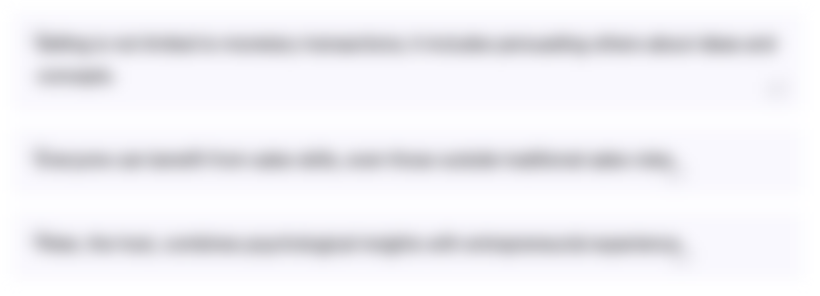
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
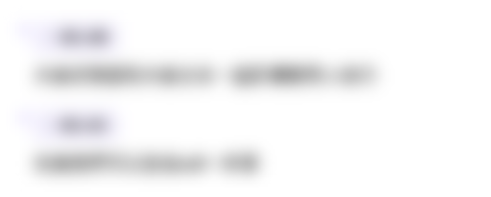
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados
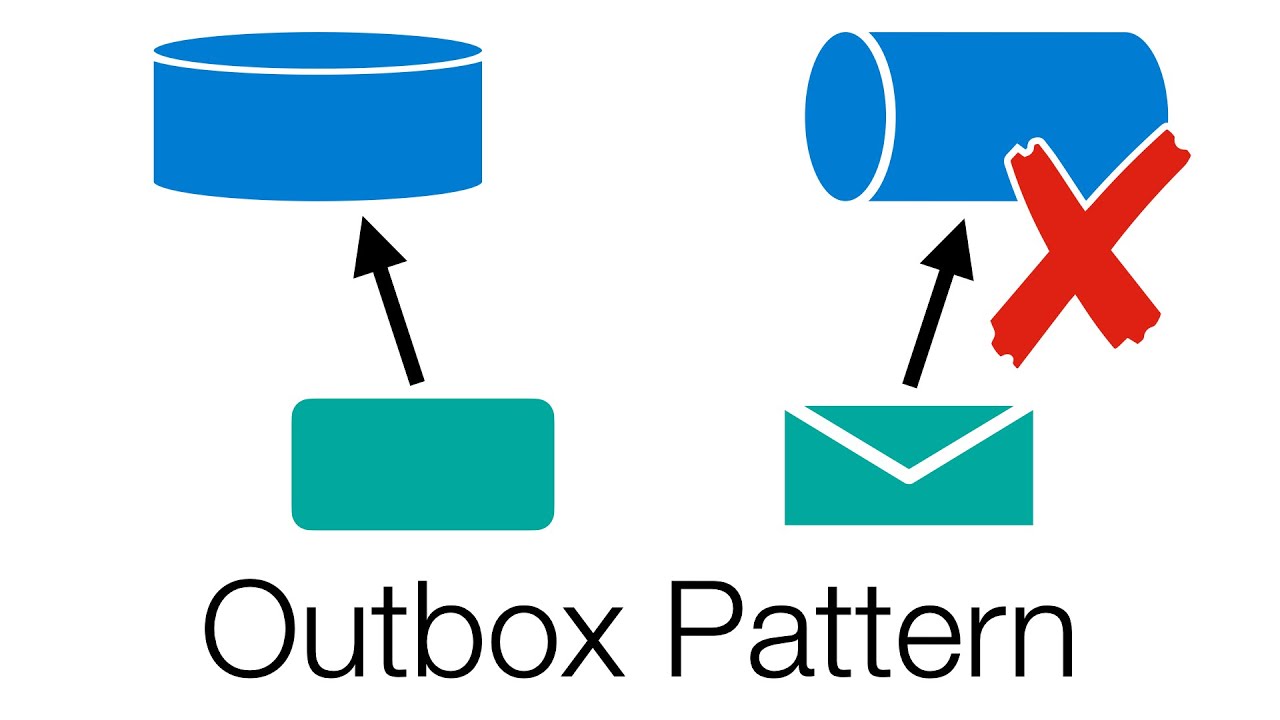
Outbox Pattern: Fixing event failures in an event-driven architecture
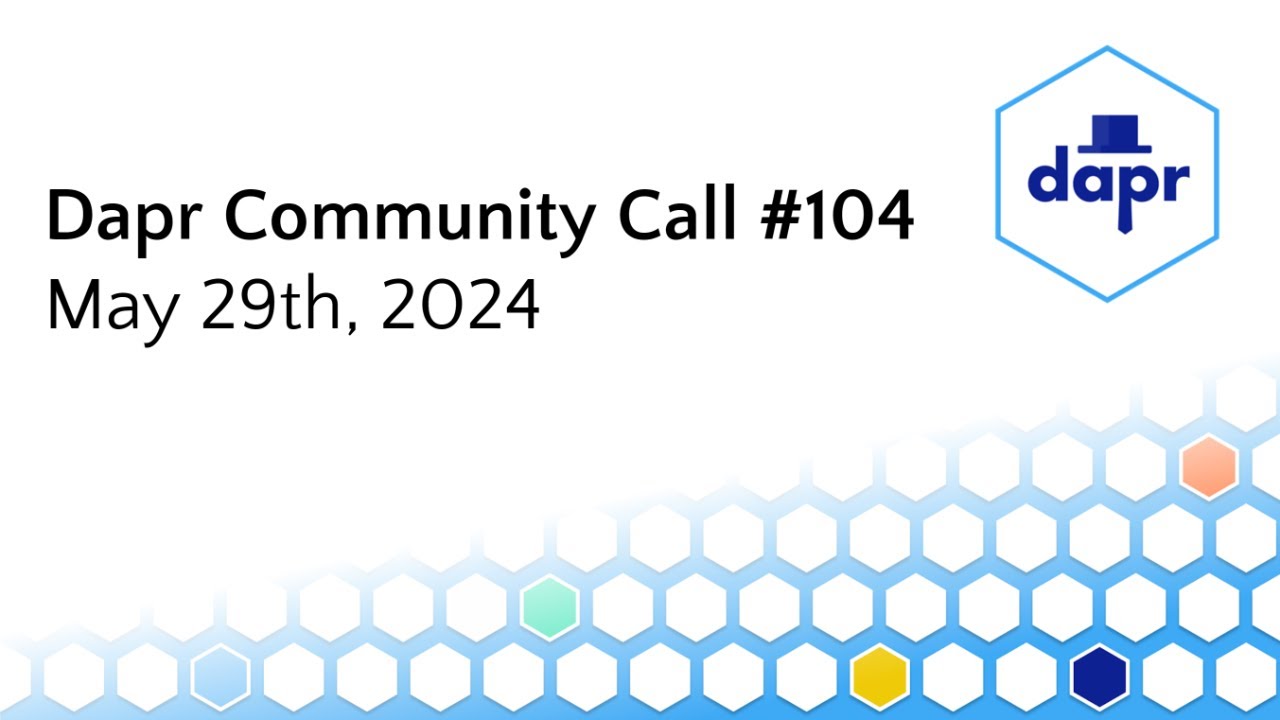
Dapr Community Call - May 29th 2024 (#104)

The Biggest Issue With Event-Based Architecture (Day 10 of 100 to Scale)
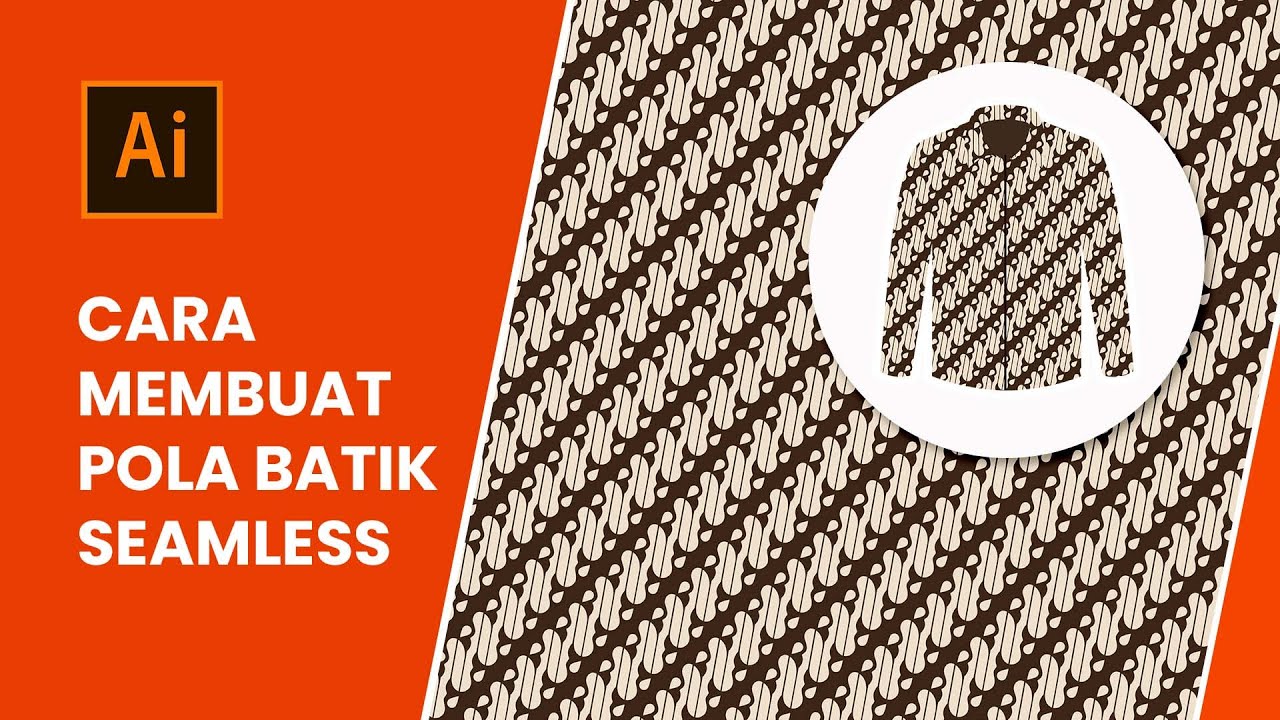
Cara Membuat Batik di Adobe Illustrator | Desainer Indonesia

Microservices with Databases can be challenging...
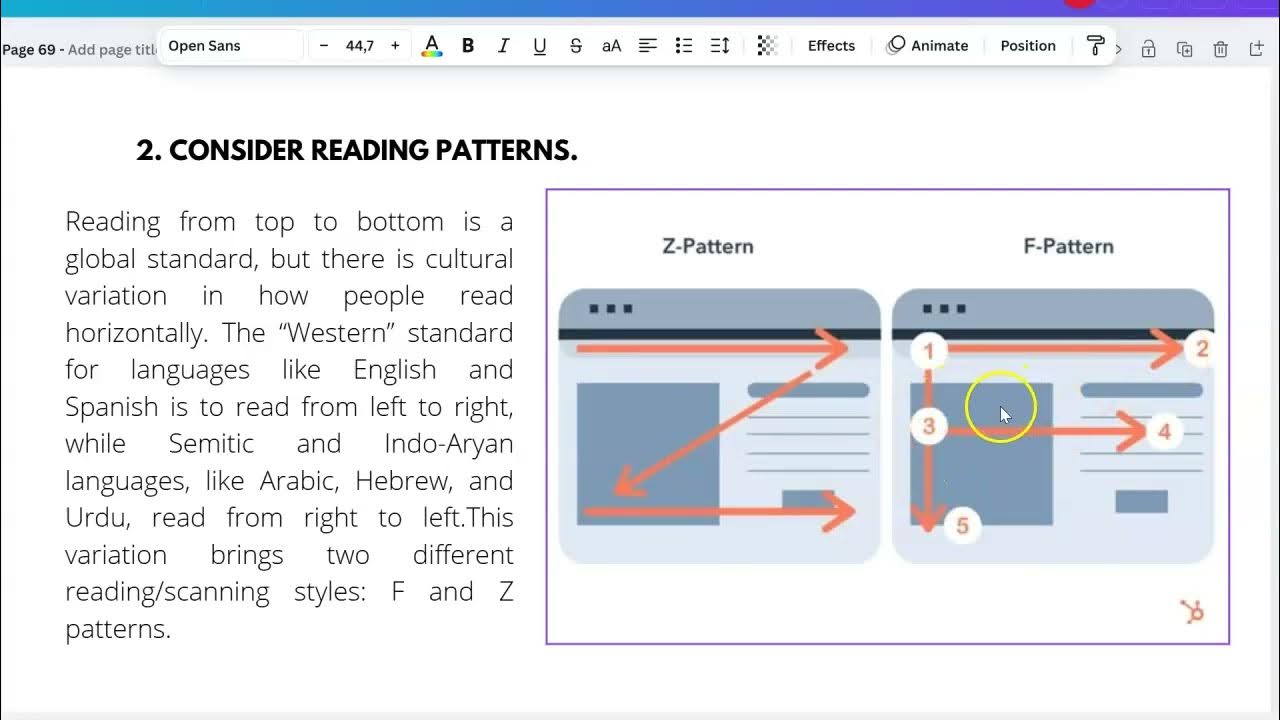
Visual Hierarchy Part 2
5.0 / 5 (0 votes)