Java arrays 🚗
Summary
TLDRIn this video, the concept of arrays in Java is explained in a clear and beginner-friendly way. The tutorial covers how to create arrays, access and modify array elements, handle errors like `ArrayIndexOutOfBoundsException`, and declare arrays with predefined sizes. The video also introduces the use of loops to iterate through array elements and print them out. By the end of the video, viewers will have a solid understanding of arrays, including how to use them to store and manage multiple values efficiently in Java programs.
Takeaways
- 😀 Arrays are used to store multiple values in a single variable in Java.
- 😀 Arrays are declared by specifying a data type followed by square brackets and initializing values with curly braces.
- 😀 Arrays are typically named in plural to reflect the multiple values they store, e.g., 'cars' instead of 'car'.
- 😀 Array elements are accessed using an index, starting at 0. For example, 'cars[0]' accesses the first element.
- 😀 If you attempt to access an element that doesn't exist in the array, it will result in an 'ArrayIndexOutOfBoundsException'.
- 😀 The size of an array is fixed once declared. However, you can add or modify elements within its bounds.
- 😀 Data types in an array must be consistent. For example, you cannot mix strings with integers in a single array.
- 😀 Arrays can be declared with a predefined size and values can be assigned later in the program using indices.
- 😀 A 'for loop' can be used to iterate through and display all the elements in an array.
- 😀 The 'length' property of an array helps in defining the loop condition when iterating through an array, ensuring you don't go out of bounds.
- 😀 The video offers code examples and encourages viewers to comment, like, and subscribe for further tutorials.
Q & A
What is an array in Java?
-An array in Java is used to store multiple values in a single variable. These values must be of the same data type, such as integers, strings, or objects.
How do you declare an array in Java?
-To declare an array in Java, you specify the data type followed by square brackets and the array name, like this: `String[] cars;`.
How do you initialize an array with values in Java?
-To initialize an array with values, you can use curly braces to enclose the values, like this: `String[] cars = {"Camaro", "Corvette", "Tesla"};`.
What happens if you try to access an array element that doesn't exist?
-If you try to access an array element that doesn't exist, you will encounter an `ArrayIndexOutOfBoundsException` because the array only contains a certain number of elements.
How do you modify an element in an array?
-You can modify an element in an array by accessing it using its index and assigning a new value. For example: `cars[0] = "Mustang";`.
Can you store different data types in a single array in Java?
-No, an array in Java can only store values of the same data type. For instance, if you have an array of strings, all elements must be strings.
How do you declare an array with a specified size in Java?
-To declare an array with a specified size, you use the `new` keyword followed by the data type and the number of elements you want. For example: `String[] cars = new String[3];`.
What is the role of the `for` loop when working with arrays?
-The `for` loop is useful for iterating over all the elements in an array. You can use it to perform actions on each element, such as printing them or modifying values.
Why is it important to start array indices at 0 in Java?
-Array indices in Java start at 0 because it reflects the memory allocation system. The first element is stored at the beginning of the array, which corresponds to index 0.
What is the purpose of the `cars.length` property in a `for` loop?
-The `cars.length` property gives the total number of elements in the array. It is often used in a `for` loop condition to ensure the loop runs for the correct number of iterations, preventing out-of-bounds errors.
Outlines
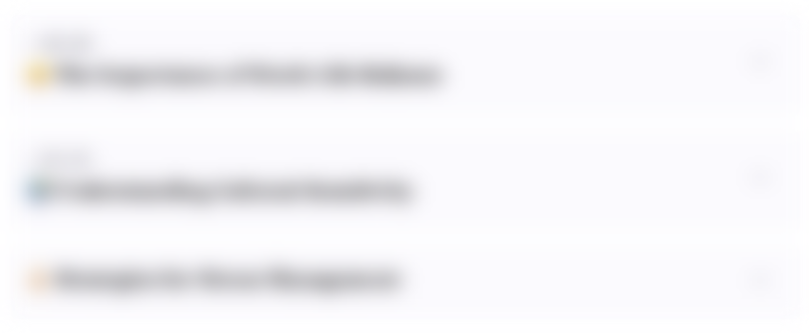
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
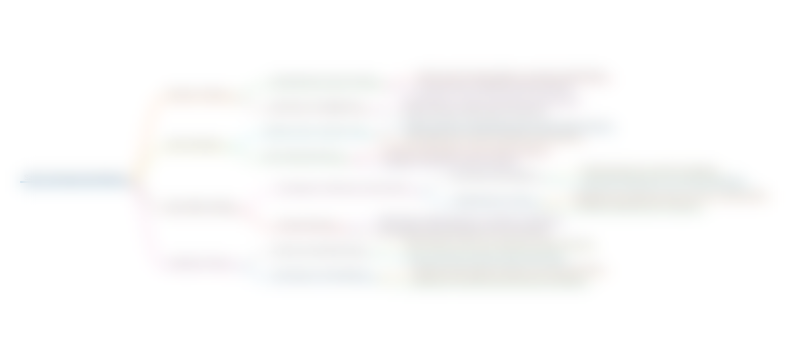
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
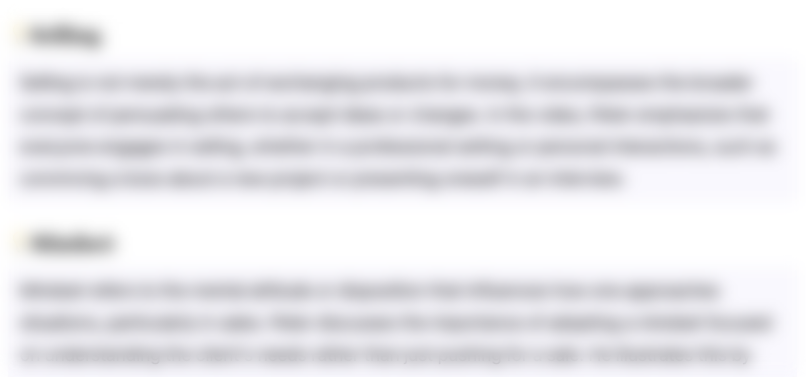
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
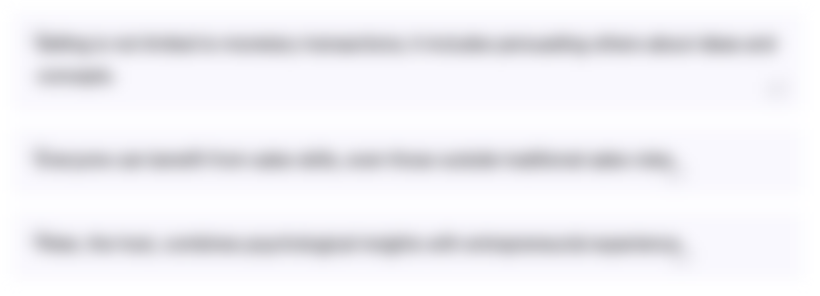
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
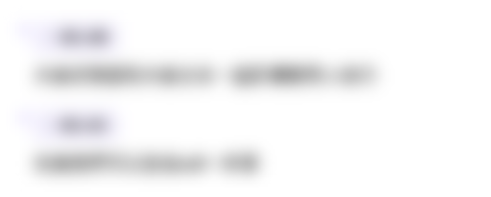
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados
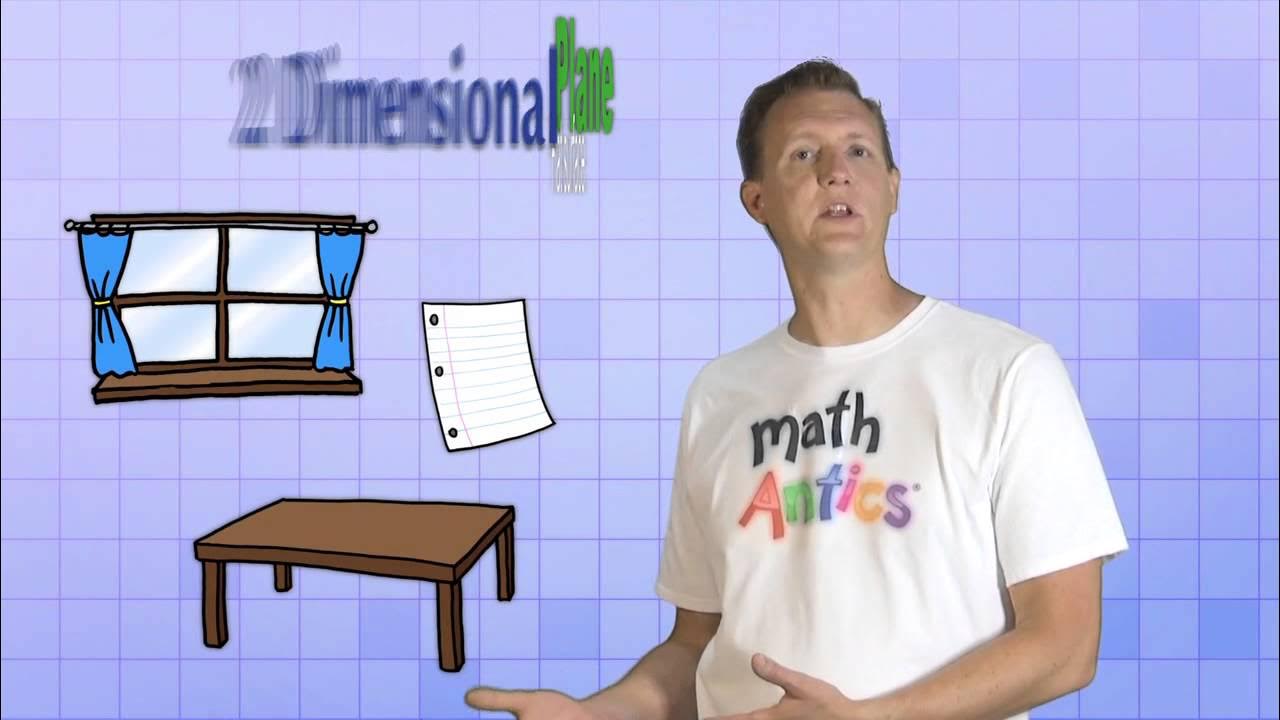
Math Antics - Points, Lines, & Planes
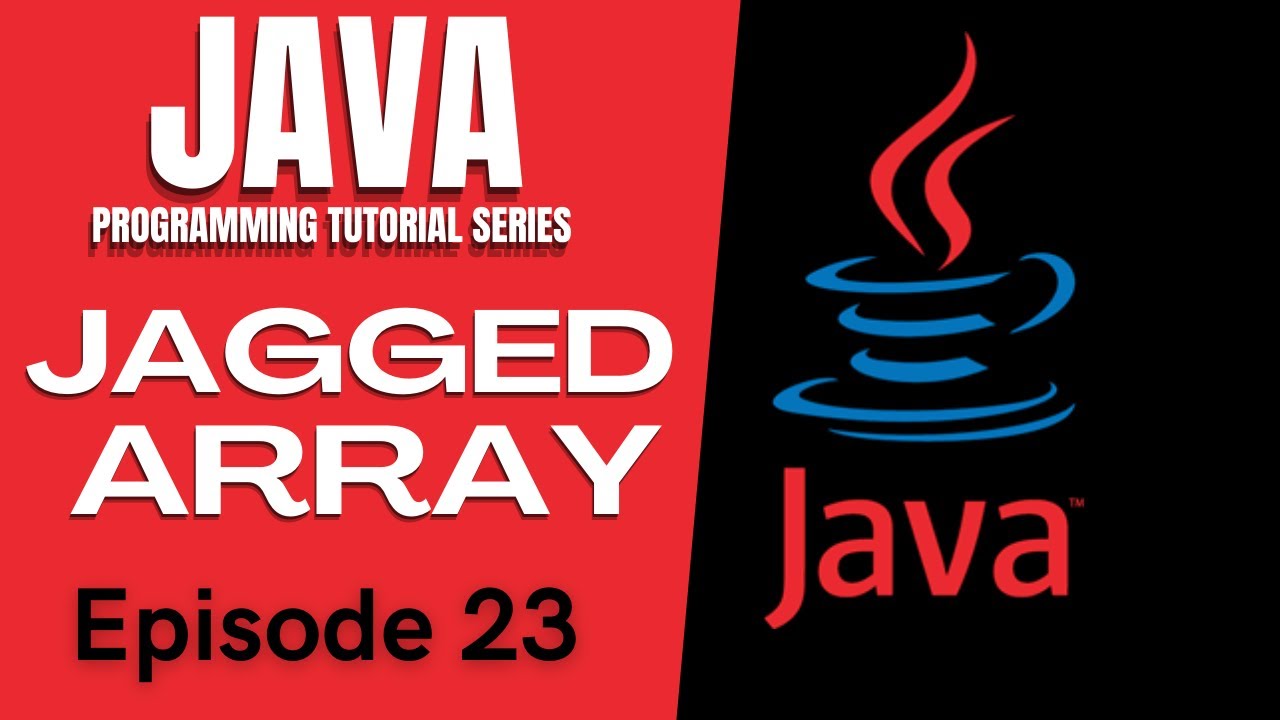
Java Tutorial #23 | 2D JAGGED ARRAYS IN JAVA | RAGGED |Tagalog | English | Filipino | 2021
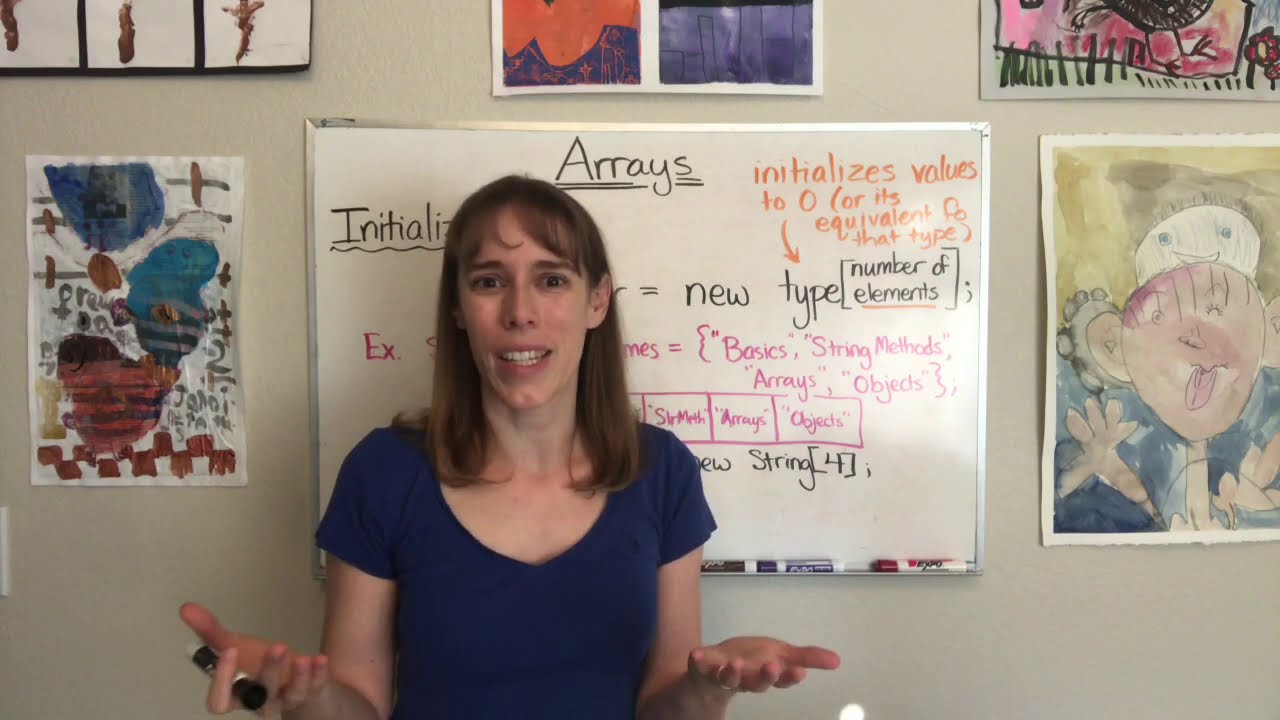
Intro to Arrays

G-19. Detect cycle in a directed graph using DFS | Java | C++
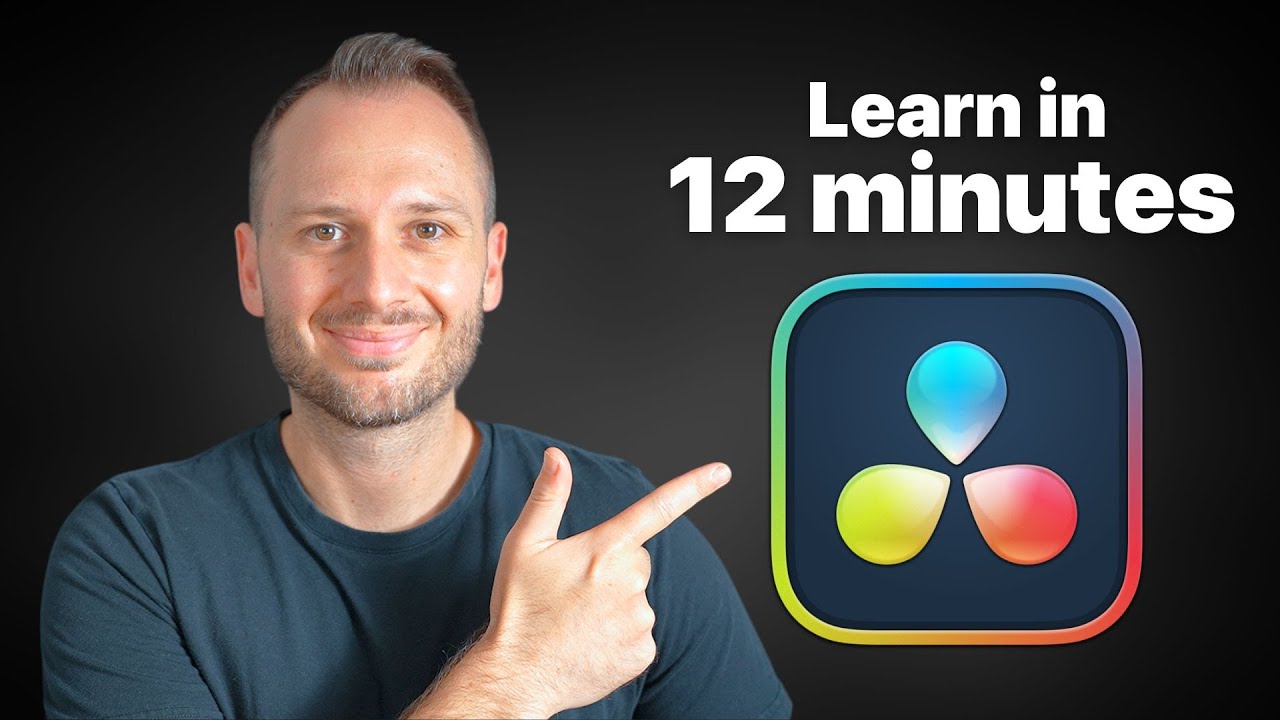
DaVinci Resolve Beginners Tutorial 2024: Edit like a PRO for FREE!
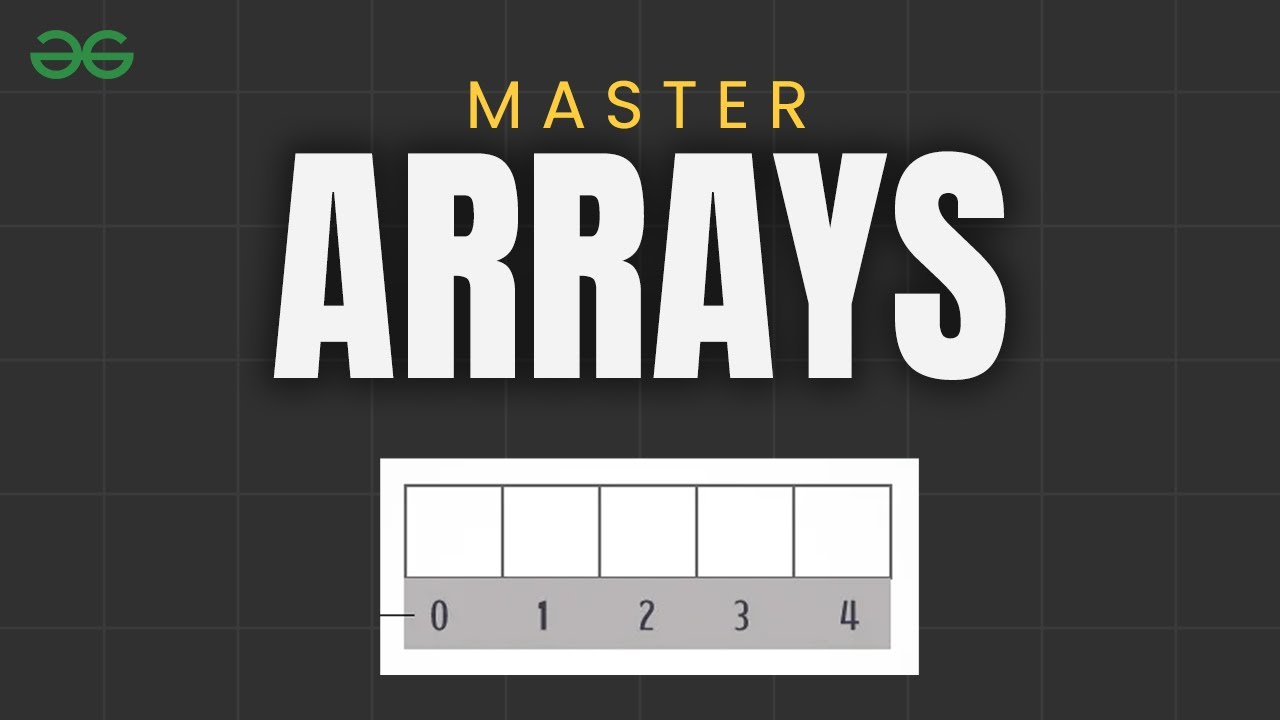
WHAT IS ARRAY? | Array Data Structures | DSA Course | GeeksforGeeks
5.0 / 5 (0 votes)