C_19 Operators in C - Part 7 (Bitwise Operators-II) | C Programming Tutorials
Summary
TLDRThis video explains the remaining bitwise operators in C: left shift, right shift, and bitwise NOT (one's complement). The presenter demonstrates how each operator works with examples, showing how bitwise operations manipulate data at the bit level. The left shift and right shift operators adjust bit positions, while bitwise NOT inverts the bits, converting positive numbers to negative in two's complement. Shortcuts for quickly calculating results using multiplication and division (for shift operators) and negation (for bitwise NOT) are also shared. This video is a practical guide for understanding and applying these operators efficiently.
Takeaways
- 😀 Bitwise operators work at the bit level, allowing manipulation of individual bits in a number.
- 😀 The left shift (`<<`) operator shifts bits to the left by a specified number of positions, effectively multiplying the number by powers of 2.
- 😀 The right shift (`>>`) operator shifts bits to the right by a specified number of positions, effectively dividing the number by powers of 2.
- 😀 Bitwise NOT (`~`) inverts the bits of a number, turning `1`s into `0`s and vice versa, often used to find the one's complement of a number.
- 😀 The left shift operator is used to multiply a number by `2^n`, where `n` is the number of positions to shift.
- 😀 The right shift operator divides a number by `2^n`, where `n` is the number of positions to shift.
- 😀 Bitwise NOT (`~`) produces a negative number in two's complement representation, which is a standard way to store negative numbers in computers.
- 😀 A shortcut to calculate the result of a left shift is: `result = a * (2^b)`, where `a` is the number and `b` is the number of positions to shift.
- 😀 A shortcut to calculate the result of a right shift is: `result = a / (2^b)`, where `a` is the number and `b` is the number of positions to shift.
- 😀 When using bitwise NOT, the result can be obtained by the formula: `result = -(a + 1)`, where `a` is the number being negated.
Q & A
What is the function of the left shift operator (`<<`) in C?
-The left shift operator shifts the bits of a number to the left by a specified number of positions. Each left shift operation is equivalent to multiplying the number by 2 raised to the power of the specified number of positions.
How can you calculate the result of a left shift operation without converting to binary?
-To calculate the result of a left shift without converting to binary, use the formula: `Result = a * (2^n)`, where `a` is the number being shifted and `n` is the number of positions.
What is the right shift operator (`>>`) used for in C?
-The right shift operator shifts the bits of a number to the right by a specified number of positions. Each right shift operation is equivalent to dividing the number by 2 raised to the power of the specified number of positions.
Can you calculate the result of a right shift operation without manually shifting the bits?
-Yes, the result of a right shift can be calculated using the formula: `Result = a / (2^n)`, where `a` is the number being shifted and `n` is the number of positions to shift.
What happens when you apply the bitwise NOT operator (`~`) to a number in C?
-The bitwise NOT operator inverts all the bits of a number, changing 1s to 0s and 0s to 1s. The result is a negative number represented in two's complement format.
How do you calculate the result of a bitwise NOT operation without manually inverting the bits?
-To calculate the result of a bitwise NOT operation, use the formula: `Result = -(a + 1)`, where `a` is the number being inverted.
What is the significance of using two's complement to represent negative numbers in C?
-Two's complement is the standard method used to represent negative numbers in C and most modern computing systems. It avoids the issues associated with sign magnitude and one's complement representations, such as multiple representations of zero.
Why are trailing spaces filled with zeros in a left shift operation?
-In a left shift operation, the bits are shifted to the left, and the vacated positions at the right are filled with zeros because the left shift operation effectively multiplies the number by powers of 2, and the additional zeros represent the increased magnitude.
What would be the result of `a = 10` after shifting left by 4 positions (`a << 4`)?
-Shifting `a = 10` left by 4 positions (`a << 4`) results in `160`. This is because shifting left by 4 positions is equivalent to multiplying `10` by `2^4 = 16`, so `10 * 16 = 160`.
What is the output when applying `~5` (bitwise NOT on 5)?
-When applying `~5` (bitwise NOT on 5), the result is `-6` because the bitwise NOT inverts all the bits of the number. In binary, 5 is `00000101`, and its inverse becomes `11111010`, which is `-6` in two's complement.
Outlines
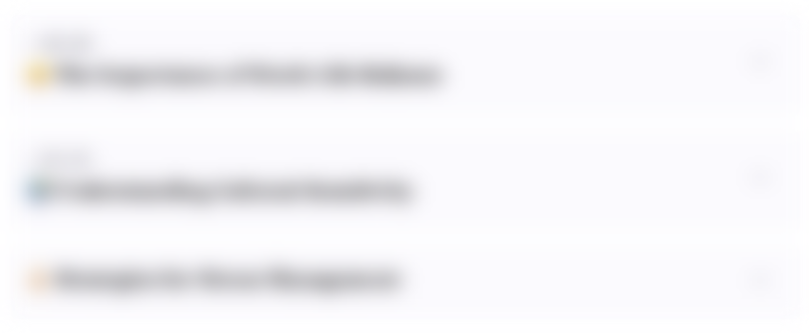
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
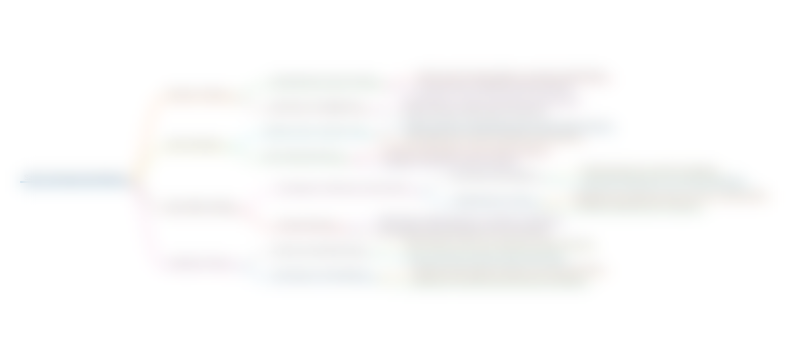
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
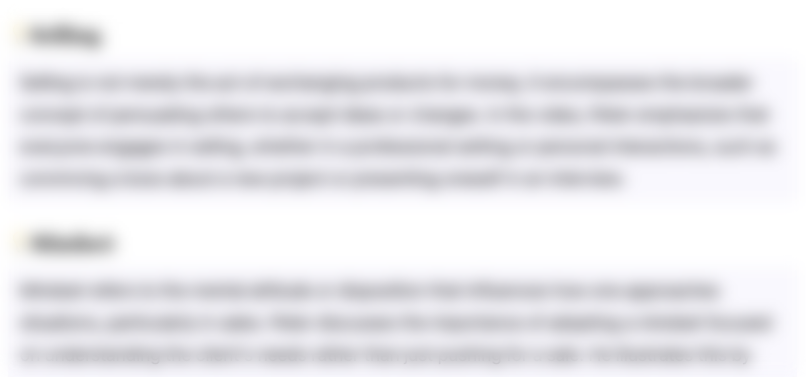
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
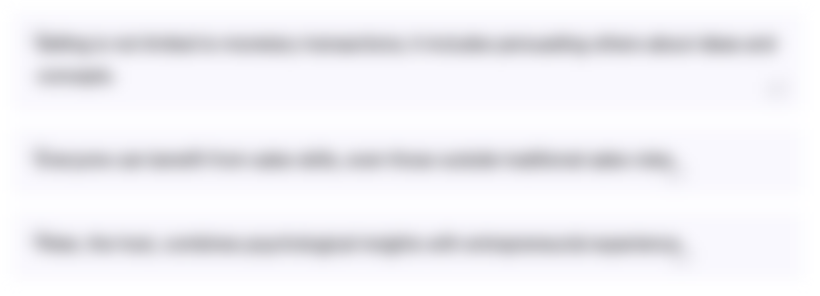
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
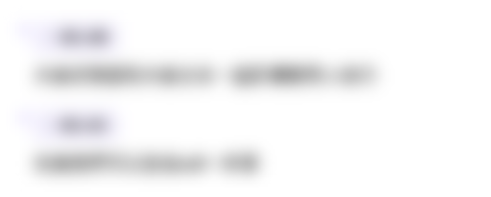
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados
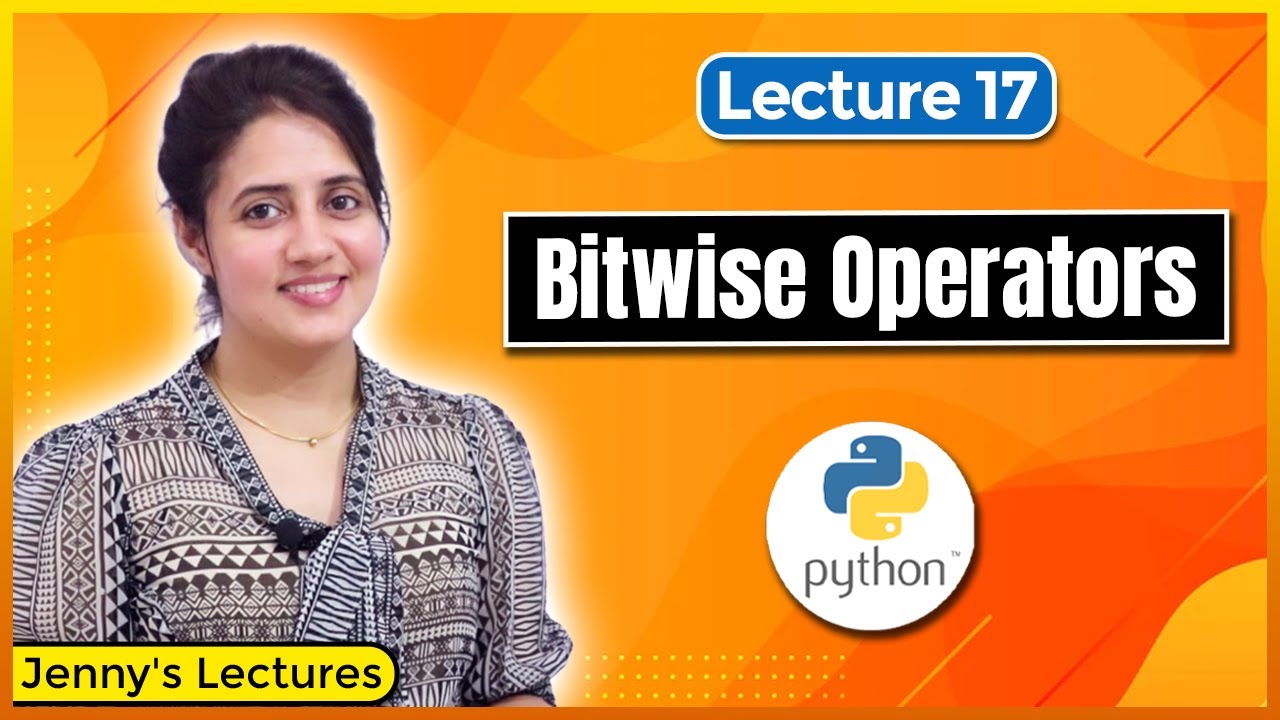
Operators in Python | Bitwise Operators | Python Tutorials for Beginners #lec17
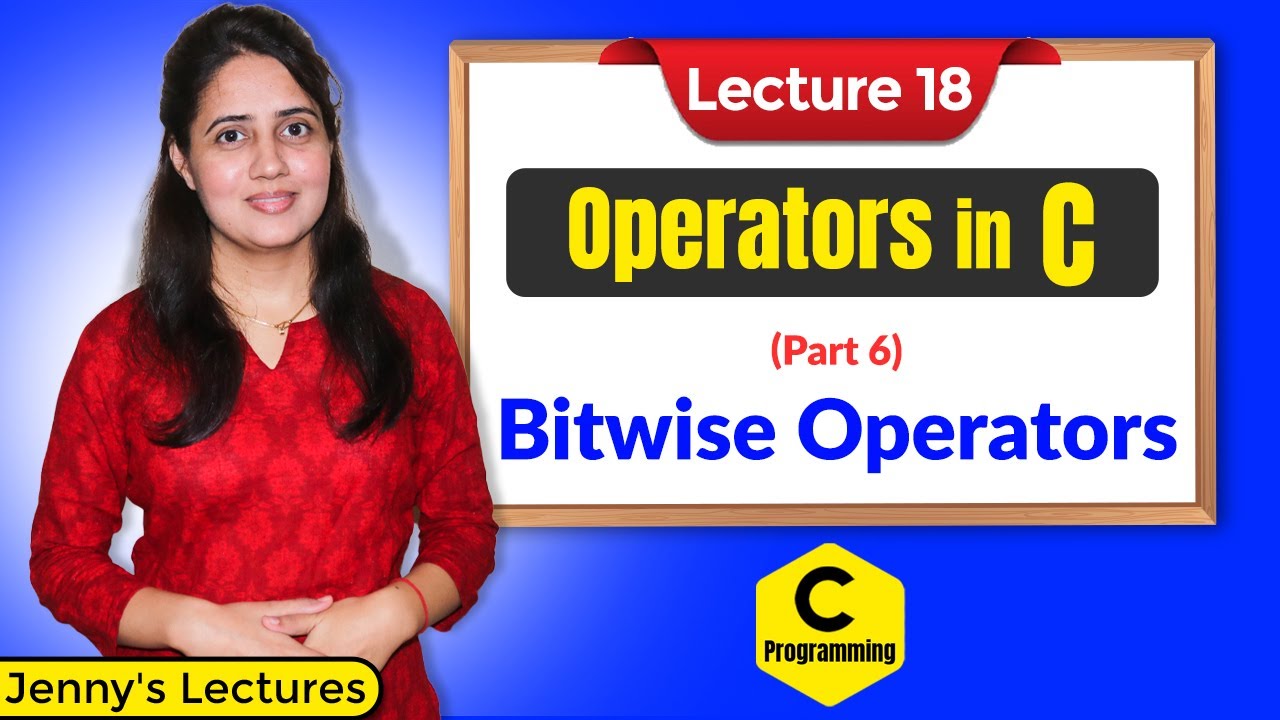
C_18 Operators in C - Part 6 | Bitwise Operators | C Programming Tutorials

C_13 Operators in C - Part 1 | Unary , Binary and Ternary Operators in C | C programming Tutorials
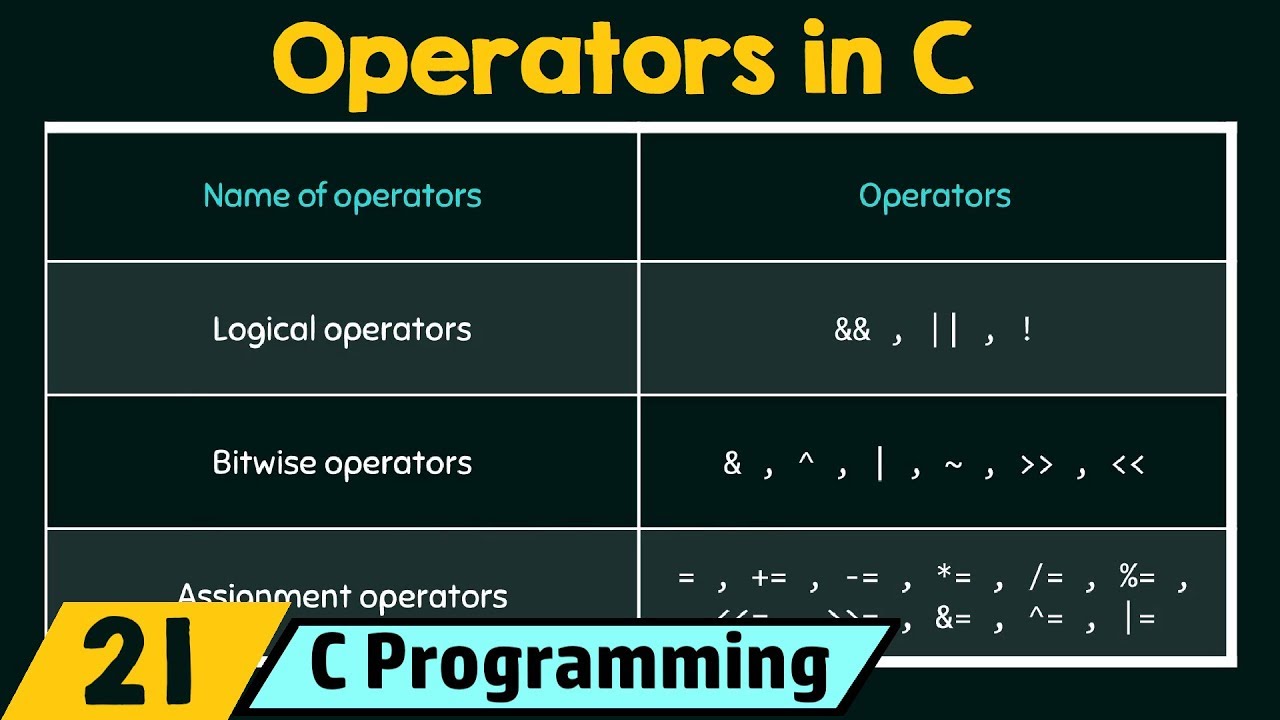
Introduction to Operators in C
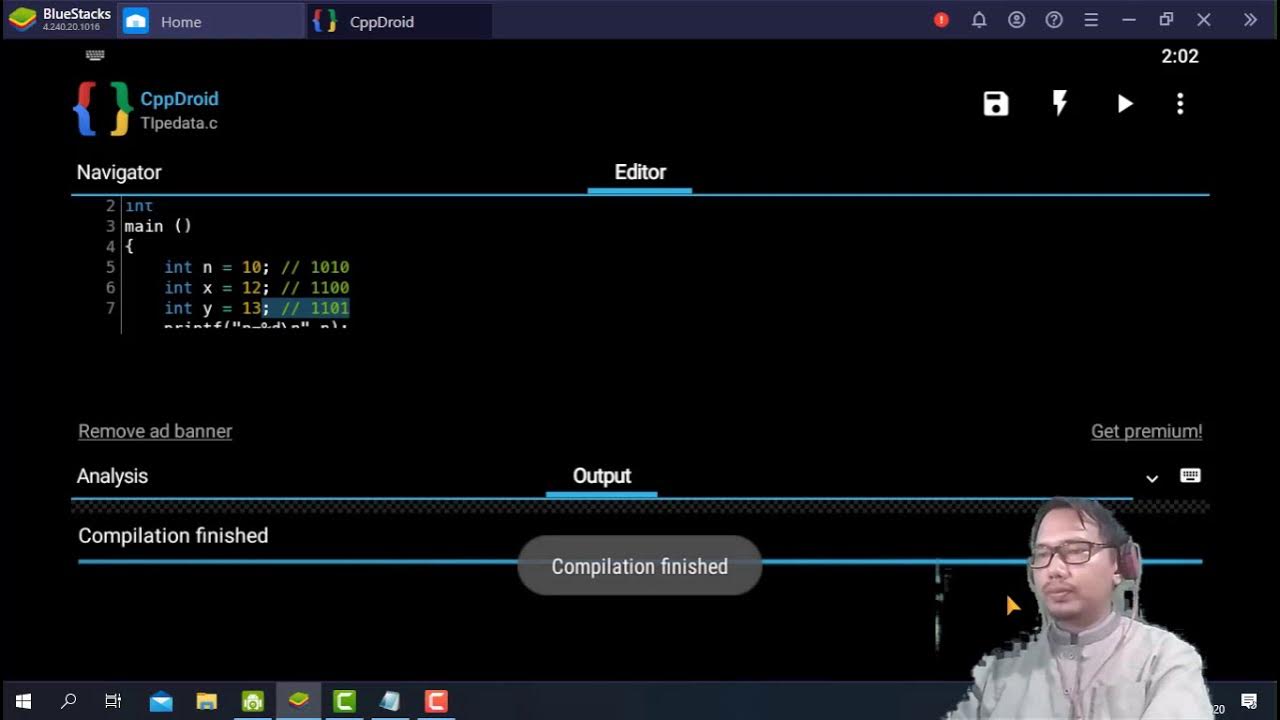
Pergeseran Bit dan Operasi Logika pada Pemrograman Operator Bahasa C
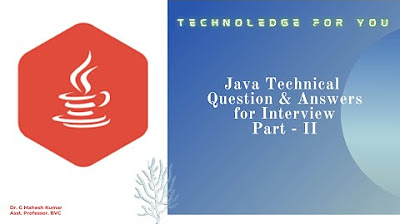
JAVA TECHNICAL QUESTION & ANSWERS FOR INTERVIEW PART - II
5.0 / 5 (0 votes)