[CS61C FA20] Lecture 07.3 - RISC-V Intro: RISC-V add/sub Instructions
Summary
TLDRThe video script introduces basic RISC-V assembly instructions, focusing on addition (ADD) and subtraction (SUB) between registers. It explains the rigid syntax of these instructions, where the operation, destination, and sources are clearly defined. The professor discusses how addition is commutative, while subtraction is not, and demonstrates how to perform more complex arithmetic operations using temporary registers. The script emphasizes that higher-level C instructions often compile into multiple lines of RISC-V assembly. The session concludes with examples of how to implement these operations in RISC-V and promises more instruction types after the break.
Takeaways
- 🧠 RISC-V instructions for addition and subtraction are being introduced in this lesson.
- 🎬 The professor mentions Bruce Lee's movies as a childhood influence but notes they can't use his tricks in RISC-V instruction.
- 🎯 Assembly instructions have a rigid format, making them easier for hardware to decode.
- ➕ Addition syntax in assembly: `add x1, x2, x3` is equivalent to `a = b + c` in C language.
- ➖ Subtraction syntax in assembly: `sub x3, x4, x5` is equivalent to `d = e - f` in C.
- 🔄 Addition is a commutative operation, meaning operand order doesn't matter, but subtraction is not.
- ⚙️ Complex arithmetic operations may require the use of temporary registers for multiple operands.
- 📏 A single high-level line in C can translate to multiple RISC-V assembly instructions.
- 💬 Comments in assembly (starting with #) are not compiled and serve as annotations.
- 🔀 Temporary registers are used in more complex examples to handle operations like `f = g + h - (i + j)`.
Q & A
What is the format of RISC-V arithmetic instructions?
-RISC-V arithmetic instructions follow a rigid format: the operation name, followed by three operands. The first is the destination register, and the next two are source registers, separated by commas. For example: `add x1, x2, x3`.
What does the command `add x1, x2, x3` mean in assembly?
-In assembly, `add x1, x2, x3` means the value in register x2 is added to the value in register x3, and the result is stored in register x1. It is equivalent to the C code `a = b + c`.
How does subtraction differ from addition in RISC-V?
-Subtraction differs from addition in that it is not commutative. In RISC-V, `sub x3, x4, x5` subtracts the value in x5 from the value in x4 and stores the result in x3. The order of operands matters for subtraction, unlike addition.
What is the equivalent C instruction for `sub x3, x4, x5` in RISC-V?
-The equivalent C instruction for `sub x3, x4, x5` is `d = e - f`, where `d` is stored in register x3, `e` in register x4, and `f` in register x5.
Why is the format of RISC-V instructions kept rigid?
-The format is kept rigid to simplify the hardware’s decoding process. By ensuring that operands are always in the same positions, the hardware can easily identify sources and destinations, making it efficient and straightforward.
What happens when you need to perform more complex operations involving multiple operands in RISC-V?
-When more complex operations involving multiple operands need to be performed, they are broken into several steps using temporary registers. For example, to calculate `a = b + c + d - e`, you use intermediate additions and subtractions, storing intermediate results in temporary registers.
How would you implement `a = b + c + d - e` in RISC-V assembly?
-You would implement it by breaking the operation into steps: first adding b and c, then adding d, and finally subtracting e, using a temporary register to accumulate the intermediate results.
Why is addition considered commutative but subtraction is not?
-Addition is commutative because the order of the operands does not matter; `a + b` is the same as `b + a`. Subtraction, however, is not commutative because the order affects the result; `a - b` is not the same as `b - a`.
How are comments added in RISC-V assembly code?
-Comments in RISC-V assembly code are added using the hash symbol (#). Everything after the hash on a line is ignored by the compiler and serves as a comment.
How can temporary registers be used to handle more complex arithmetic operations?
-Temporary registers are used to store intermediate results. For instance, in a more complex arithmetic operation like `f = g + h - (i + j)`, you would first store the results of `g + h` and `i + j` in temporary registers, and then subtract the two to get the final result.
Outlines
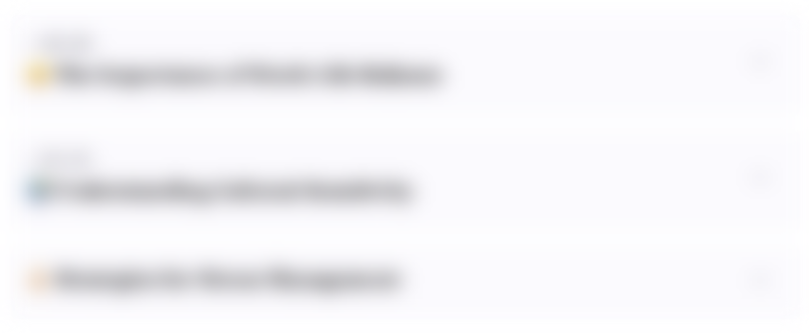
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
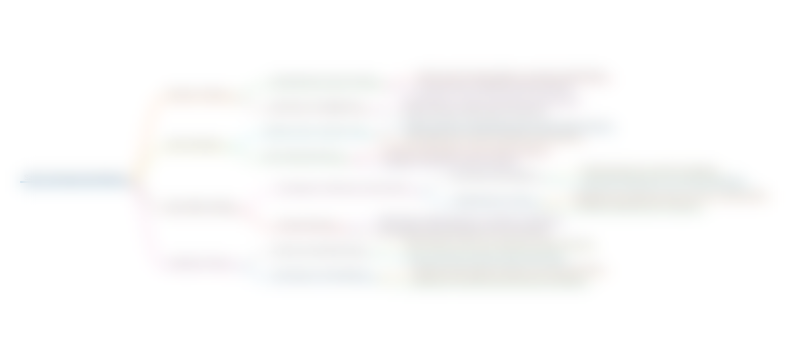
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
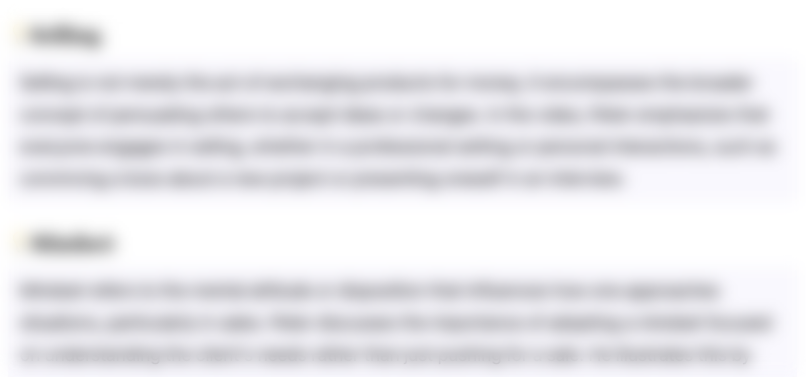
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
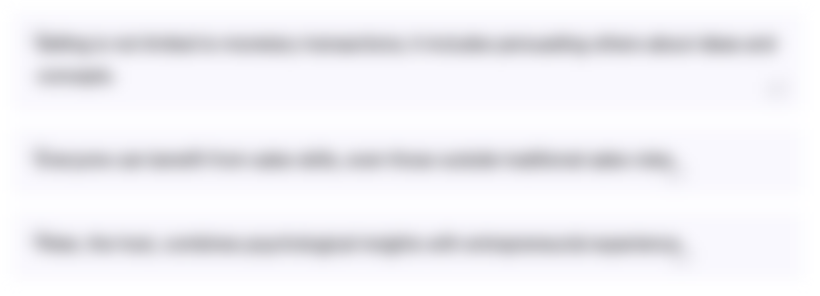
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
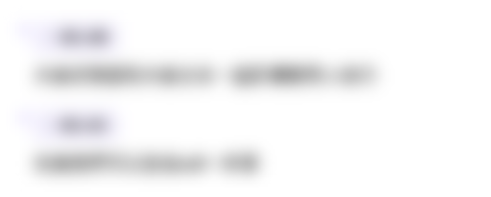
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados

Assembly Programming with RISC-V: Part 1

RISC vs CISC | RISC | Reduced Instruction Set Computer | CISC | Complex Instruction Set Computer

L-1.9: Arithmetic Instructions(Data Manipulation) in Computer Organisation and Architecture

CH01_VID02_CPU Components & Lifecycle

Addition and Subtraction of Small Numbers
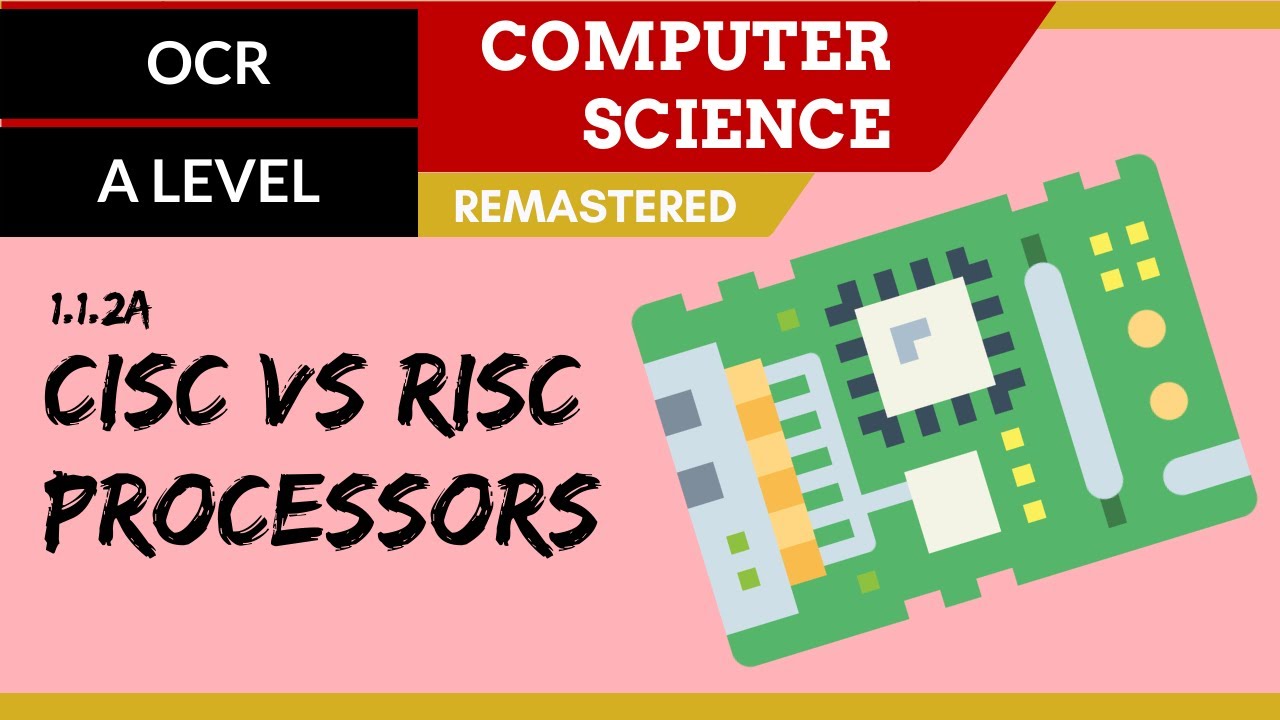
6. OCR A Level (H046-H446) SLR2 - 1.1 CISC vs RISC
5.0 / 5 (0 votes)