Capgemini Java Interview 2024 | Java | Spring Boot | Microservices | Database
Summary
TLDRThe interviewee discusses their Java knowledge, including streams, collections, and immutability. They explain HashMap vs ConcurrentHashMap, thread safety, and the volatile and synchronized keywords. They also cover Java memory model, exception handling, and their experience with Spring Boot and microservices. They detail the shift from monolithic to microservices architecture, challenges like data consistency, and query optimization strategies.
Takeaways
- 💻 The interviewee is proficient in Java and is being tested on Java Stream to find the second highest number in a list.
- 📝 The interviewee explains the process of defining a list, using streams, distinct elements, sorting in descending order, and skipping elements to solve the problem.
- 🔑 The use of 'Optional' in Java is highlighted for handling cases where a value may or may not be present.
- 🗃️ The interviewee has experience with Java collections, differentiating between 'HashMap' and 'ConcurrentHashMap', emphasizing thread safety.
- 🧵 The interviewee discusses Java's threading capabilities, explaining 'synchronized' for critical sections and 'volatile' for variable visibility across threads.
- 🌐 The Java memory model is briefly described, including Young Generation, Old Generation, and Permanent Generation.
- 📝 The interviewee is asked to create an immutable class similar to 'String', emphasizing final class, final fields, and providing only getter methods.
- 🛠️ Exception handling in Java is covered, distinguishing between checked and unchecked exceptions, and the use of try-catch blocks.
- 🌟 The interviewee has experience with Spring Boot and microservices, highlighting advantages like starter dependencies, auto-configuration, and embedded servers.
- 🛡️ In Spring Boot projects, exceptions are handled at both global and method levels using@ControllerAdvice and @ExceptionHandler annotations.
- 🌐 The transition from monolithic to microservices architecture in the interviewee's project is discussed, focusing on scalability, independent teams, and service communication.
Q & A
What is the main objective of the interview?
-The main objective of the interview is to assess the candidate's knowledge and experience in Java, Spring Boot, microservices, and related technologies.
How does the candidate demonstrate their knowledge of Java Streams?
-The candidate demonstrates their knowledge of Java Streams by explaining how to find the second highest number in a list of integers using distinct, sorted, and skip methods.
What is the difference between HashMap and ConcurrentHashMap as explained by the candidate?
-HashMap is not thread-safe, while ConcurrentHashMap is thread-safe. ConcurrentHashMap allows multiple threads to access and modify it without causing inconsistencies by dividing it into segments.
How does the candidate describe the Java memory model?
-The candidate describes the Java memory model as consisting of a Heap memory divided into Young Generation, Old Generation, and Permanent Generation, with different garbage collection frequencies.
What is the candidate's approach to creating an immutable class in Java?
-The candidate suggests making the class final, defining only getter methods for variables, and providing values through a constructor to achieve immutability.
How does the candidate handle exceptions in Java?
-The candidate handles exceptions by using try-catch blocks for checked exceptions and mentions that unchecked exceptions do not require try-catch blocks but can be logged or handled when they occur.
What are the advantages of Spring Boot mentioned by the candidate?
-The candidate mentions that Spring Boot simplifies dependency management with starter dependencies, provides auto-configuration, and includes an embedded Tomcat server for easy deployment.
How does the candidate handle exceptions in a Spring Boot project?
-The candidate handles exceptions at both global and method levels using @ControllerAdvice for global exceptions and @ExceptionHandler for method-level exceptions.
What is the difference between authentication and authorization as explained by the candidate?
-Authentication is about verifying who a user is, while authorization is about determining what resources a user is allowed to access.
How does the candidate describe the transition from a monolithic to a microservices architecture?
-The candidate describes the transition as a move from a single large application to multiple smaller services, each managed by different teams, allowing for independent scaling and updates.
What challenges does the candidate identify with managing multiple databases in a microservices architecture?
-The candidate identifies data consistency and transaction management as major challenges when dealing with multiple databases in a microservices architecture.
Outlines
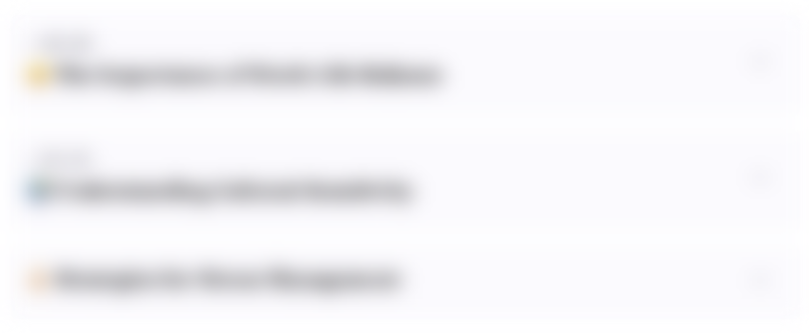
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
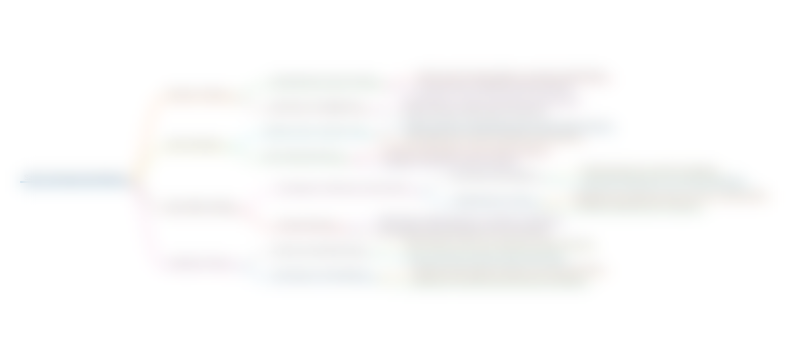
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
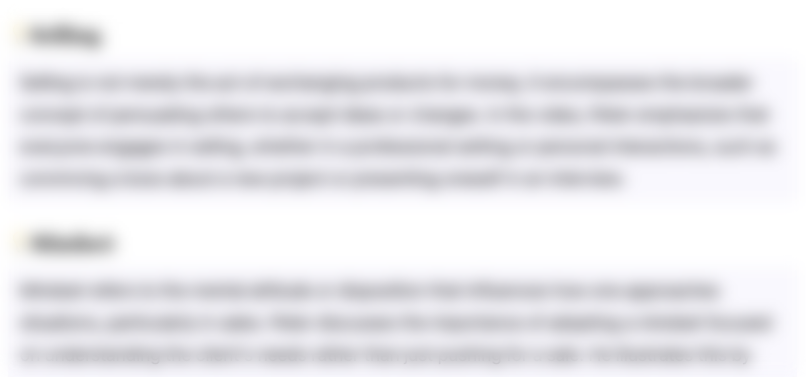
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
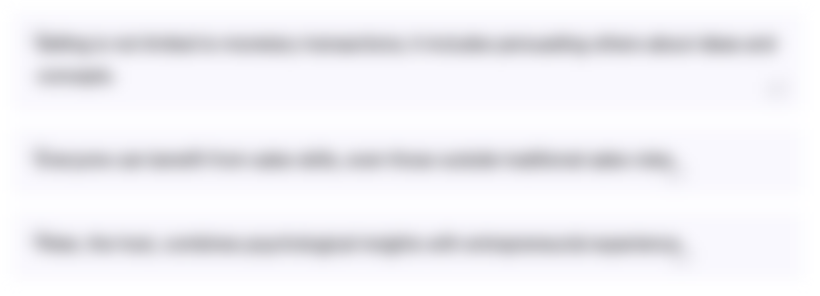
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
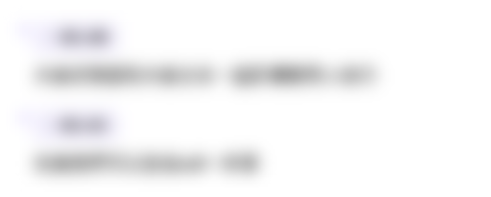
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados

TCS Java | Spring Boot | Microservices | 4 Years | Selected | Mock Interview

Capgemini Java Developer 4 yrs interview Questions and Answers L2 round #capgemini

java microservice telephonic interview of 10 years experienced

3+ years Java Developer Accenture Interview Experience| Java | Spring Boot | Microservices
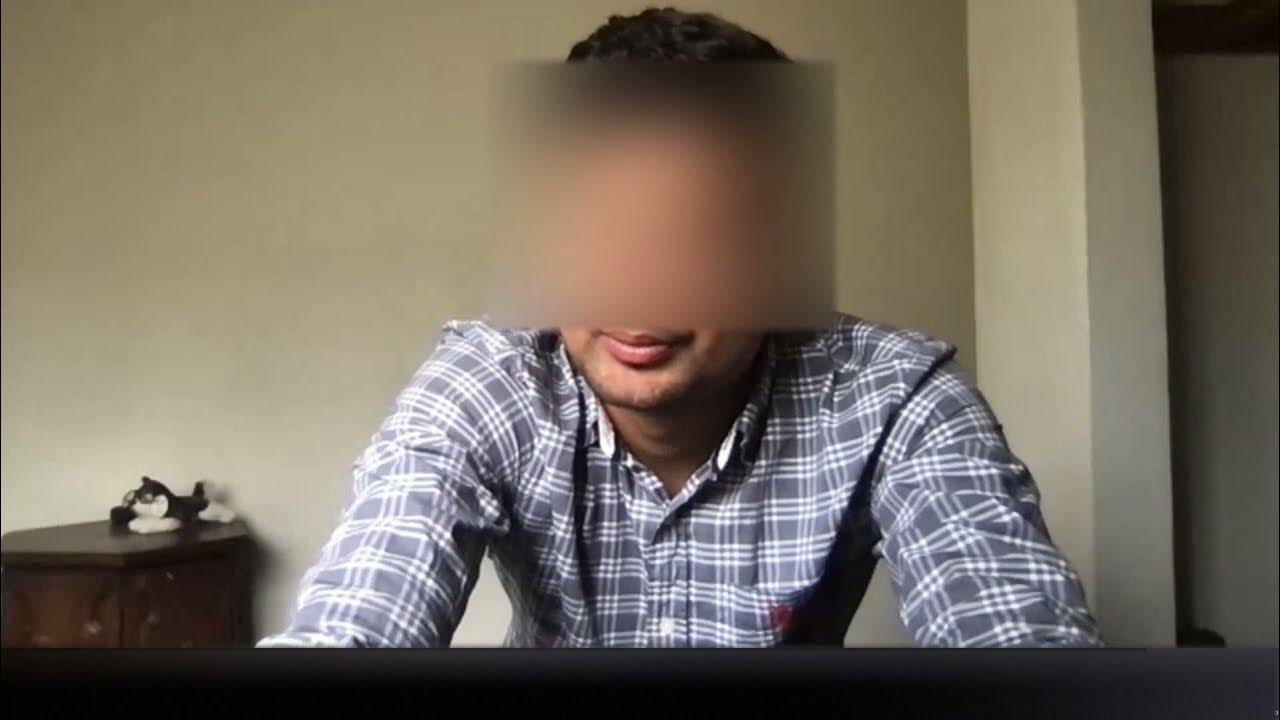
Caught Cheating - SDE Candidate interview unexpectedly terminated | [Software Engineering Interview]
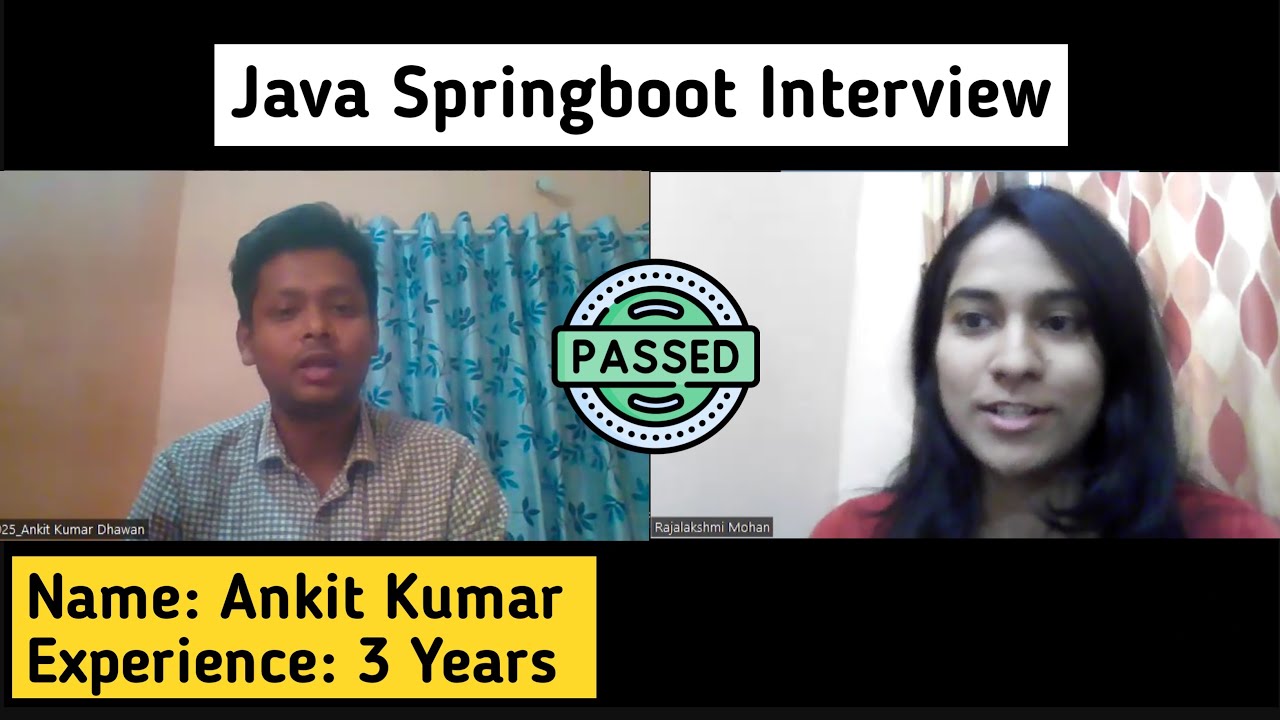
Java Spring Boot 3 Years Experience Interview
5.0 / 5 (0 votes)