Bytes and Values in Java
Summary
TLDRIn this Java-focused lecture, the presenter explores bytes and values, explaining that a bit is the smallest unit of memory and a byte consists of eight bits. They detail the memory allocation for different integer data types like byte, short, int, and long. The lecture demonstrates how to calculate the range of values for these data types using a formula involving powers of two. It advises choosing the appropriate data type based on the size of numbers needed to avoid memory wastage. The presenter also shows how to programmatically find the range of data types in Java using predefined classes and constants, emphasizing that memorization of these ranges is unnecessary.
Takeaways
- 😀 A bit is the smallest unit of memory measurement, and a byte consists of eight bits.
- 🔢 Memory is divided into bytes, with each variable in Java reserving a specific number of bytes.
- 📏 More bytes allocated to a variable mean it can store larger values.
- 💾 A long variable in Java occupies more bytes (8 bytes) than an int variable (4 bytes).
- 📦 Each box in memory represents a byte, and an int variable allocates four such boxes.
- 🔢 The size of different integer data types in Java is as follows: byte (1 byte), short (2 bytes), int (4 bytes), and long (8 bytes).
- 📉 To calculate the range of values for a data type, use the formula: min value = -2^(number of bits - 1) and max value = 2^(number of bits - 1) - 1.
- 🔄 For example, a short (16 bits) has a range from -2^15 to 2^15 - 1.
- 🚫 Avoid wasting memory by choosing the appropriate data type based on the size of numbers you are working with.
- 💡 You don't need to memorize the ranges of data types; you can find them programmatically using classes like Integer, Short, Byte, and Long in Java.
Q & A
What is the smallest unit of memory measurement in Java?
-The smallest unit of memory measurement in Java is a bit.
How many bits are there in a byte?
-There are eight bits in a byte.
What is the significance of the number of bytes a variable reserves in memory?
-The number of bytes a variable reserves in memory determines the range of values it can hold, with more bytes allowing for larger values.
How many bytes does an int variable allocate in memory?
-An int variable allocates four bytes in memory.
What is the difference between a byte and a short in terms of memory allocation?
-A byte allocates one byte (8 bits) in memory, whereas a short allocates two bytes (16 bits).
How can you calculate the range of values for different data types in Java?
-You can calculate the range of values using the formula: minimum value = -2^(number of bits - 1) and maximum value = 2^(number of bits - 1) - 1.
What is the range of values for a short data type?
-The range of values for a short data type is from -32768 to 32767.
Why is it important to choose the right data type for a variable?
-Choosing the right data type is important to avoid wasting memory and to ensure that the variable can store the required range of values.
How can you find the range of an integer programmatically in Java?
-You can find the range of an integer by accessing the Integer.MIN_VALUE and Integer.MAX_VALUE constants from the Integer class.
What is the purpose of the snake_case convention used for constants in Java?
-The snake_case convention is used for constants in Java to indicate that the value of the variable should not change and is considered a constant.
What happens if you try to assign a value outside the range of a data type?
-If you try to assign a value outside the range of a data type, the compiler will give you an error.
Outlines
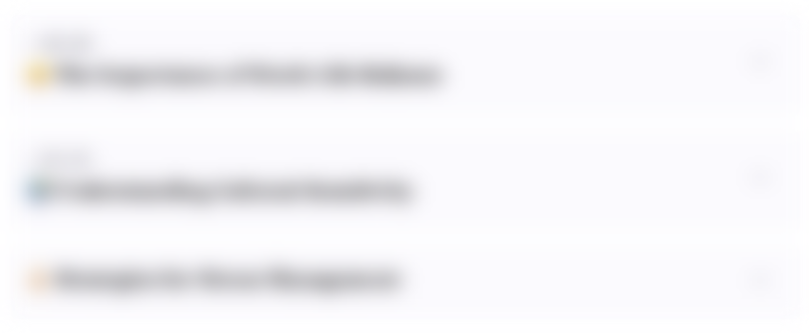
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
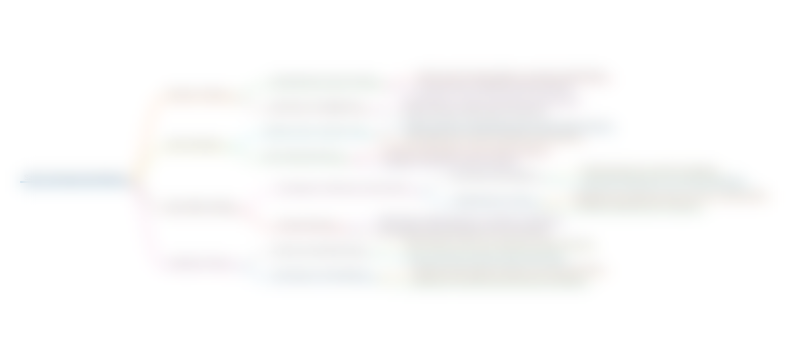
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
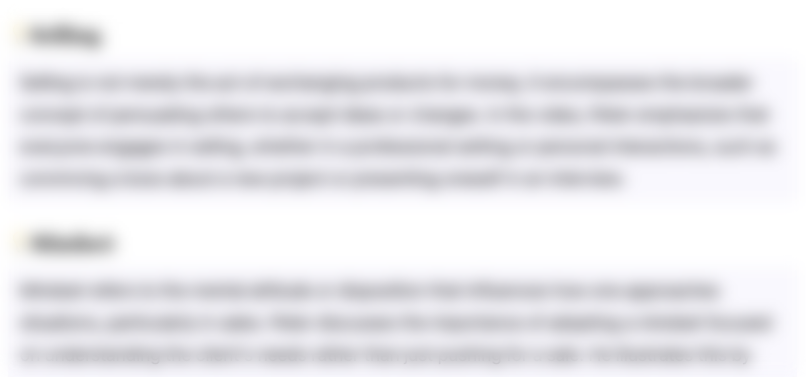
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
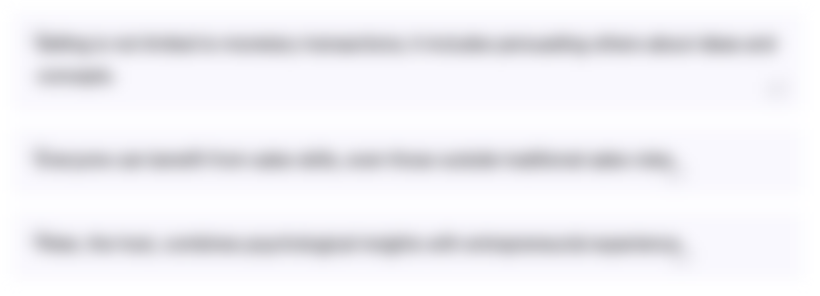
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
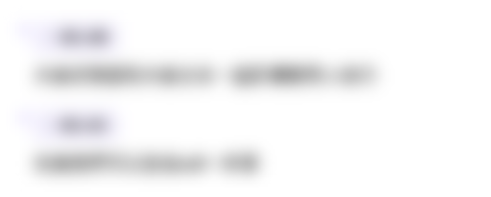
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados

Computer Skills Course: Bits, Bytes, Kilobytes, Megabytes, Gigabytes, Terabytes (OLD VERSION)
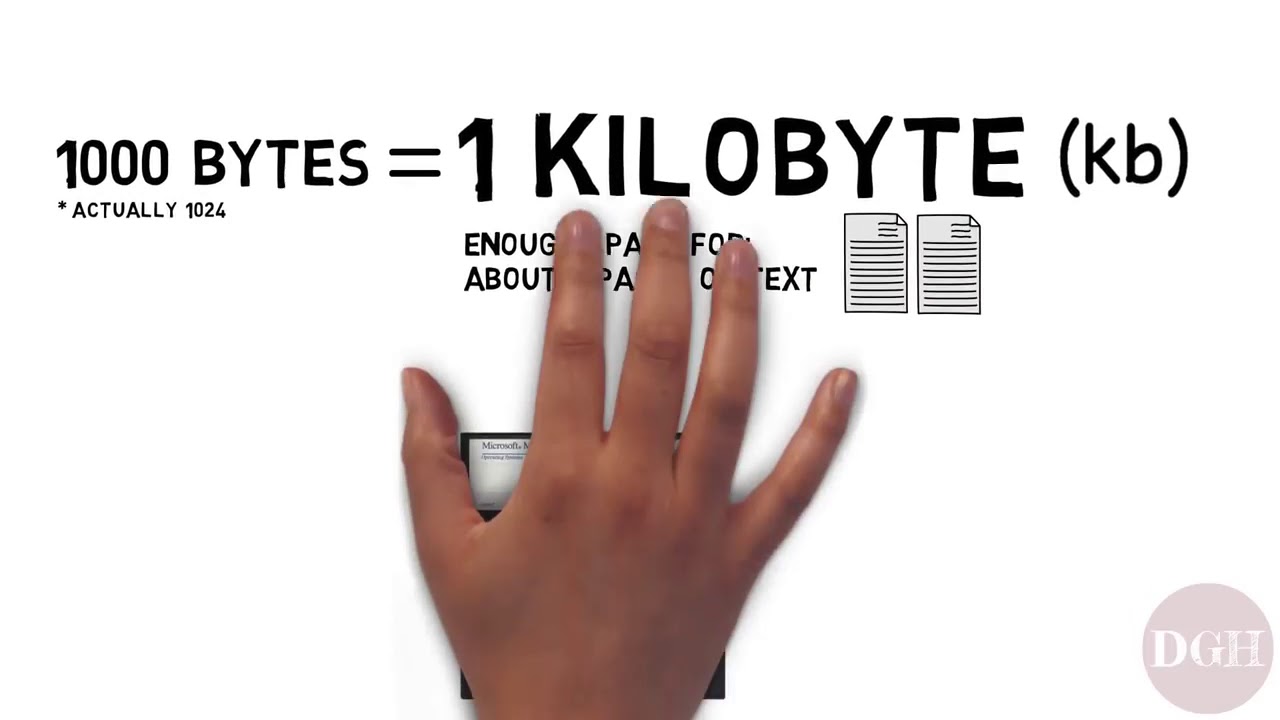
Units of Storage
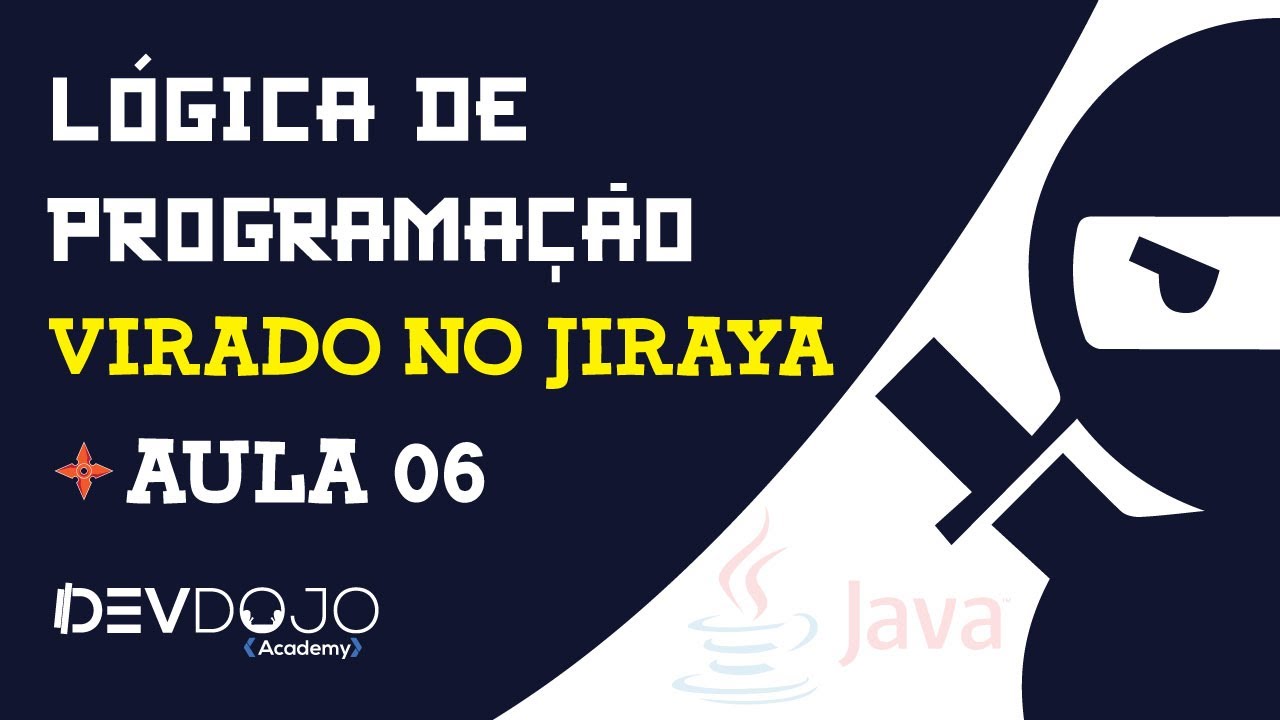
Lógica Virado no Jiraya 06 - Memória volátil
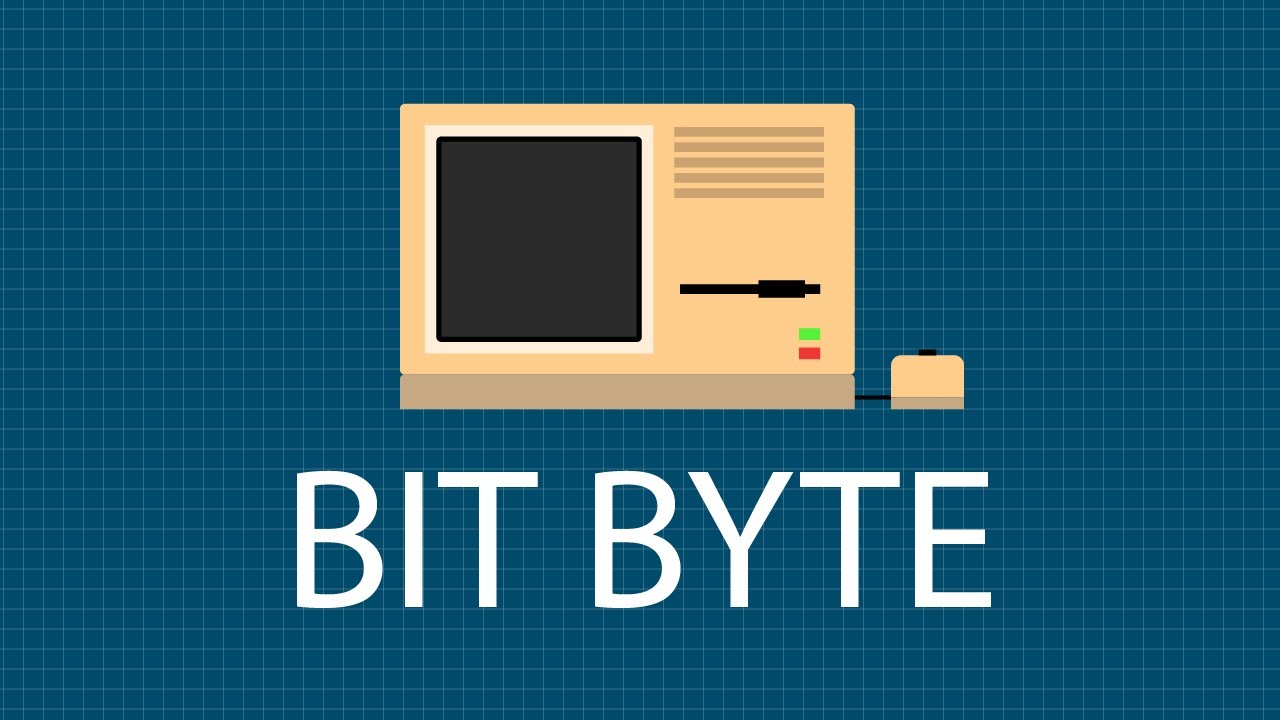
Diferencias entre Bit y Byte
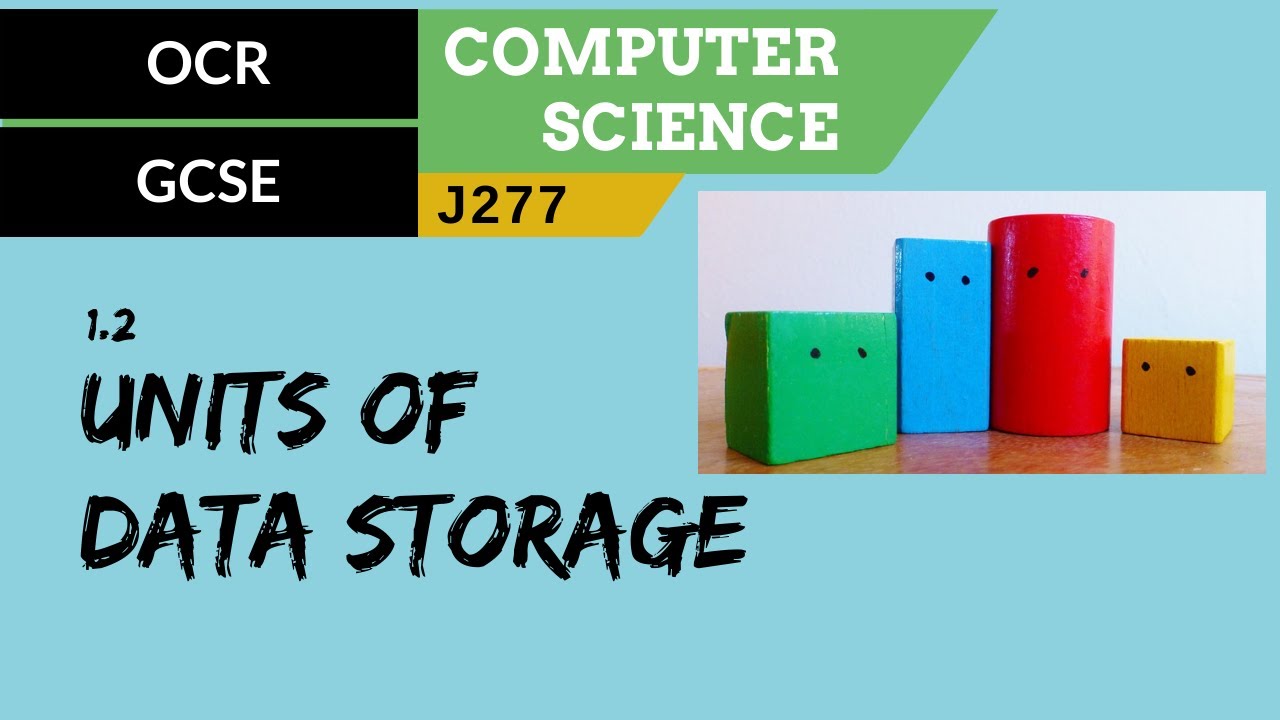
12. OCR GCSE (J277) 1.2 Units of data storage

Internal RAM Structure of 8051 | Bit Addressable Area & General Purpose Area of Internal RAM in 8051
5.0 / 5 (0 votes)