Declaring Variables without Var, Let, Const - What Would Happen?
Summary
TLDRIn this video, the speaker explores the consequences of declaring variables in JavaScript without using 'var', 'let', or 'const'. They explain that such variables are attached to the global object, which can lead to accidental overwrites of existing properties and affect the browser's default behavior. The speaker suggests using 'var', 'let', or 'const', creating a custom global object, or employing strict mode to avoid these issues. Additionally, they discuss the global execution context and how variables are managed within it, emphasizing the importance of understanding the call stack and function scope.
Takeaways
- 🌐 In JavaScript, variables declared without `var`, `let`, or `const` are attached to the global object, which can lead to unintended behavior.
- 🛠️ The global object in a browser environment is `window`, and modifying its properties can disrupt the browser's default functionality.
- 🚫 Overwriting properties of the global object can weaken the resiliency of a program, making it harder to manage and extend.
- 🔑 To avoid conflicts, it's recommended to use `var`, `let`, or `const` for variable declarations or to create a custom global object for application-wide properties.
- 📚 Understanding the global object and its properties is crucial for preventing accidental overwrites and ensuring compatibility with third-party libraries.
- 🔍 The `this` keyword in JavaScript refers to the global object, providing a way to access its properties and methods.
- 🔒 Using strict mode in JavaScript can prevent the accidental creation of global variables and enforce more rigorous coding practices.
- 📈 The call stack in JavaScript is a data structure that manages the execution of functions and helps visualize where variables are scoped.
- 🛑 Functions in JavaScript are both executable code and objects, with the object part containing properties that can be accessed and manipulated.
- 🔬 Tools like `console.dir` can be used to inspect the object properties of functions, including the global object.
Q & A
What happens if you declare a variable without using var, let, or const in JavaScript?
-If you declare a variable without using var, let, or const, the variable gets placed onto the global object, which can lead to overwriting existing properties or creating new ones, potentially interfering with the runtime environment's native functionalities.
What is the global object in JavaScript?
-The global object is an object that contains data and functionality allowing the runtime environment, such as a browser or Node.js, to perform various processes. In a browser, this is typically the 'window' object.
Why is it bad to overwrite properties of the global object?
-Overwriting properties of the global object can change the default behavior of the runtime environment, leading to unexpected results and potentially conflicting with third-party libraries or future code.
What is global abatement and how does it help in managing global variables?
-Global abatement is a process where you create a custom global object with properties and functionality that are accessible throughout your application. This prevents overwriting or creating new properties on the native global object.
How can you prevent accidentally writing variables onto the global object?
-To prevent accidentally writing variables onto the global object, always use a variable declaration keyword (var, let, or const), use global abatement, or enable strict mode which prevents variable declarations without a keyword.
What is the call stack in JavaScript and how does it relate to variable scope?
-The call stack is a data structure that follows a last-in, first-out rule and is used to manage the execution of functions. Variables declared with var, let, or const exist within the function portion of the global execution context or the function's scope.
How does strict mode prevent variables from being attached to the global object?
-Strict mode prevents variables from being attached to the global object by throwing an error when a variable is declared without a keyword, thus enforcing the use of var, let, or const.
What are the different ways to access the global object in JavaScript?
-You can access the global object in JavaScript using the 'this' keyword, by directly referencing 'window' in a browser environment, using 'global' in Node.js, or by inspecting the 'global' property in the 'scopes' tab of a function's object representation in the console.
Why is it important to understand the difference between function execution context and the global execution context?
-Understanding the difference between function execution context and the global execution context helps in managing variable scope and avoiding conflicts or unintended side effects when declaring variables.
How can you ensure that your variables do not conflict with existing properties on the global object?
-To ensure that your variables do not conflict with existing properties, use naming conventions that avoid common property names, always declare variables with var, let, or const, and consider using a custom global object for your application's global variables.
Outlines
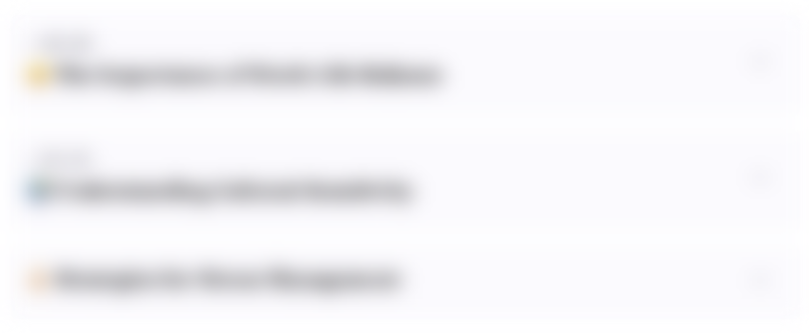
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
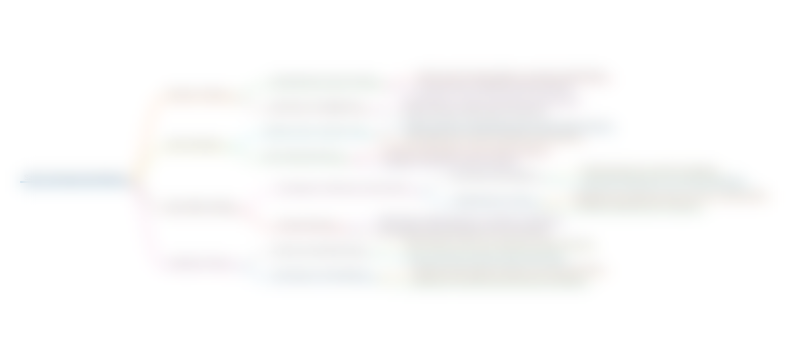
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
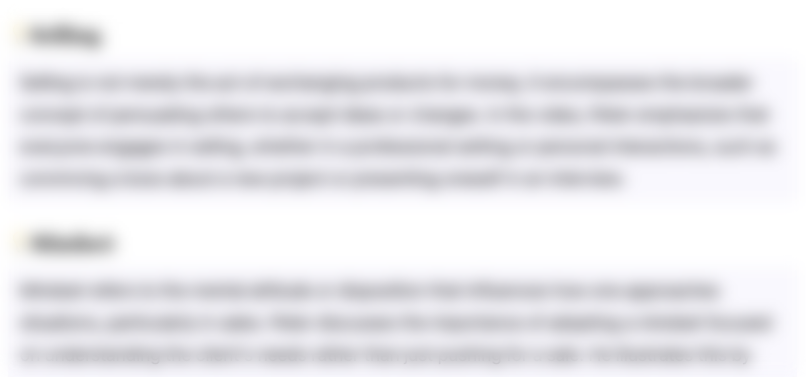
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
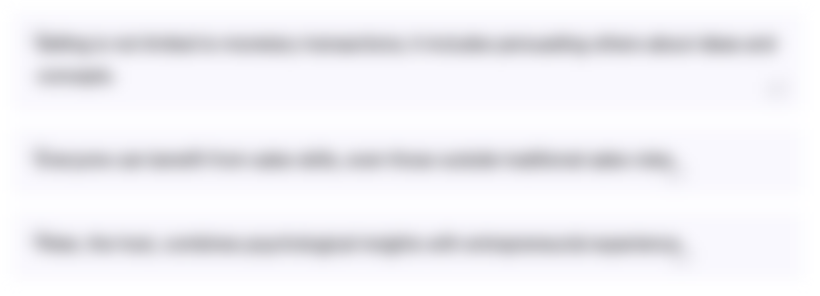
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
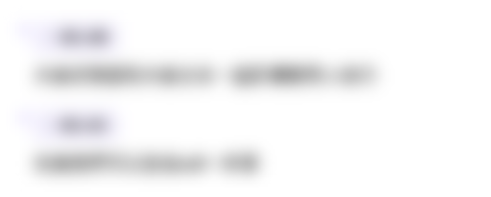
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados
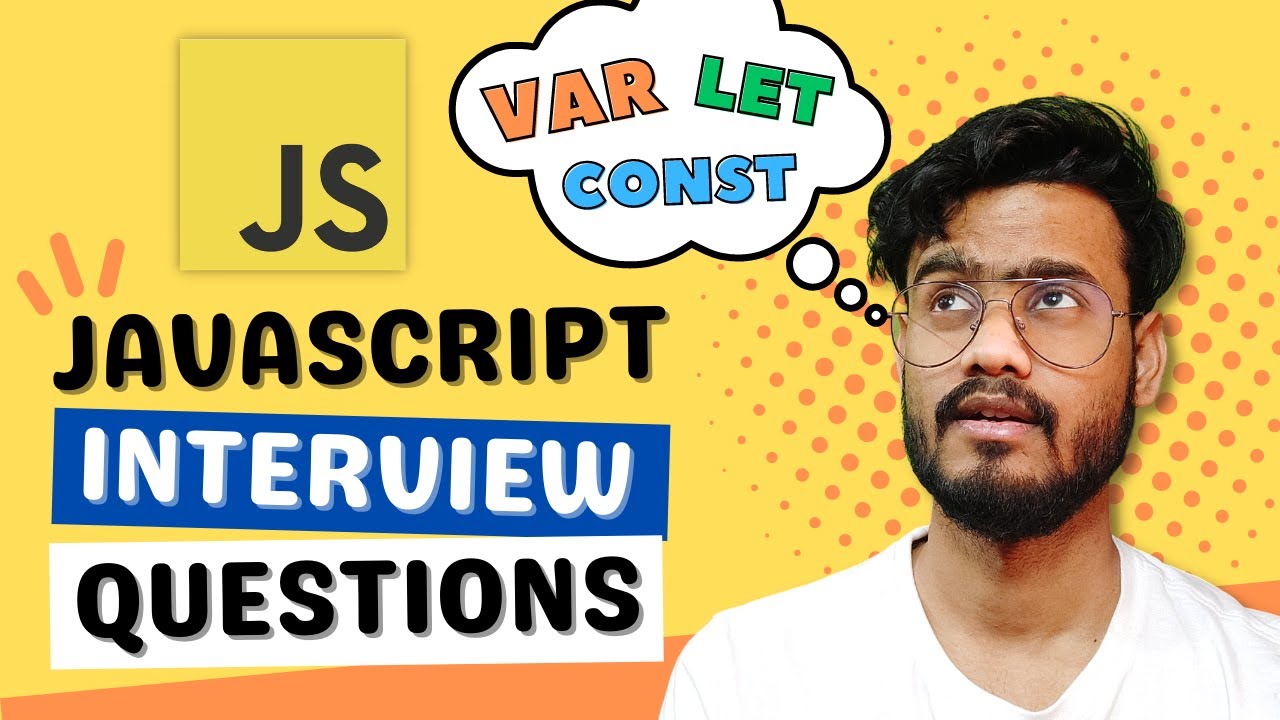
Javascript Interview Questions ( Var, Let and Const ) - Hoisting, Scoping, Shadowing and more
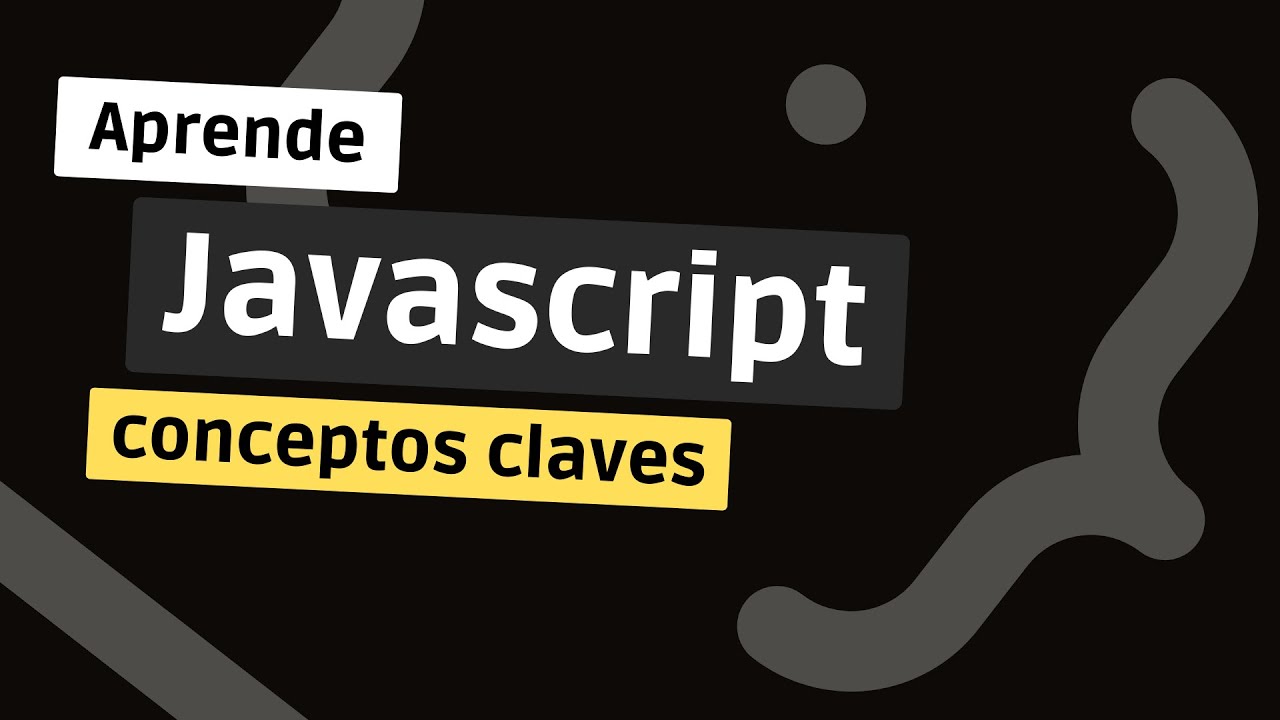
Curso Javascript - #03 const vs let vs var

JavaScript Visualized - Execution Contexts
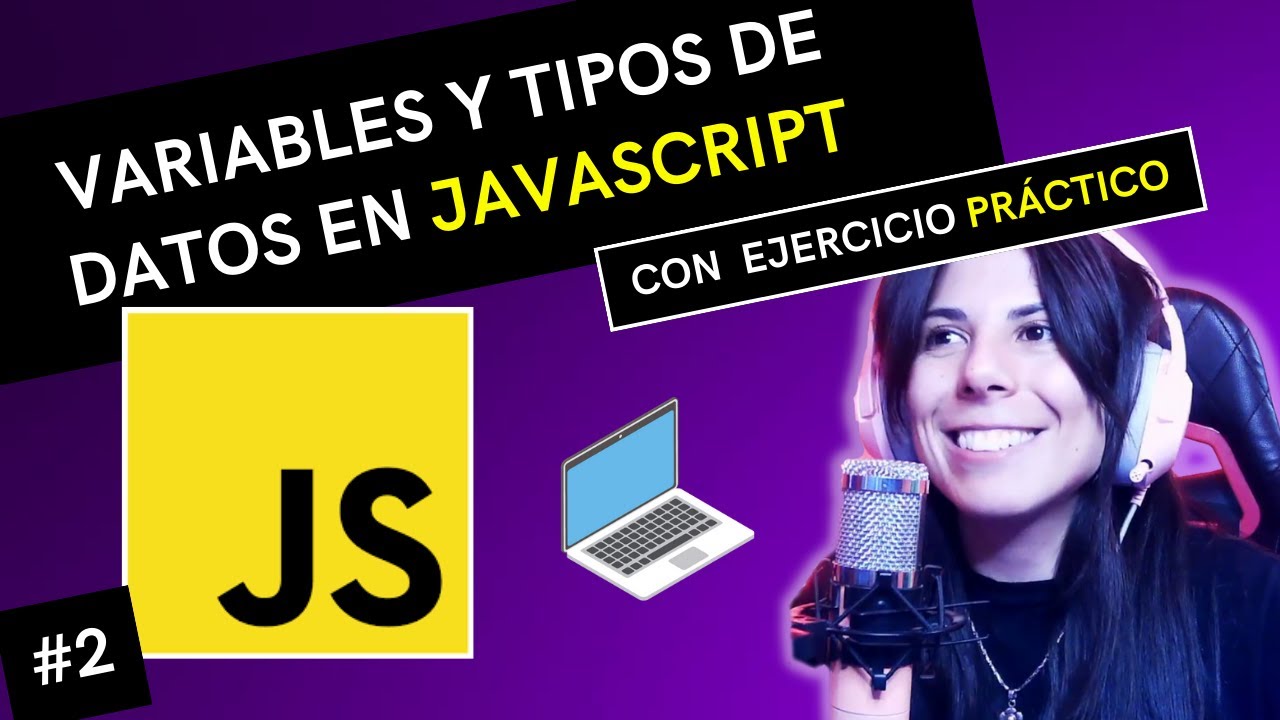
👩🏻💻 VARIABLES y TIPOS DE DATOS en JAVASCRIPT | ⭐ Curso JAVASCRIPT DESDE CERO 🚀 #2
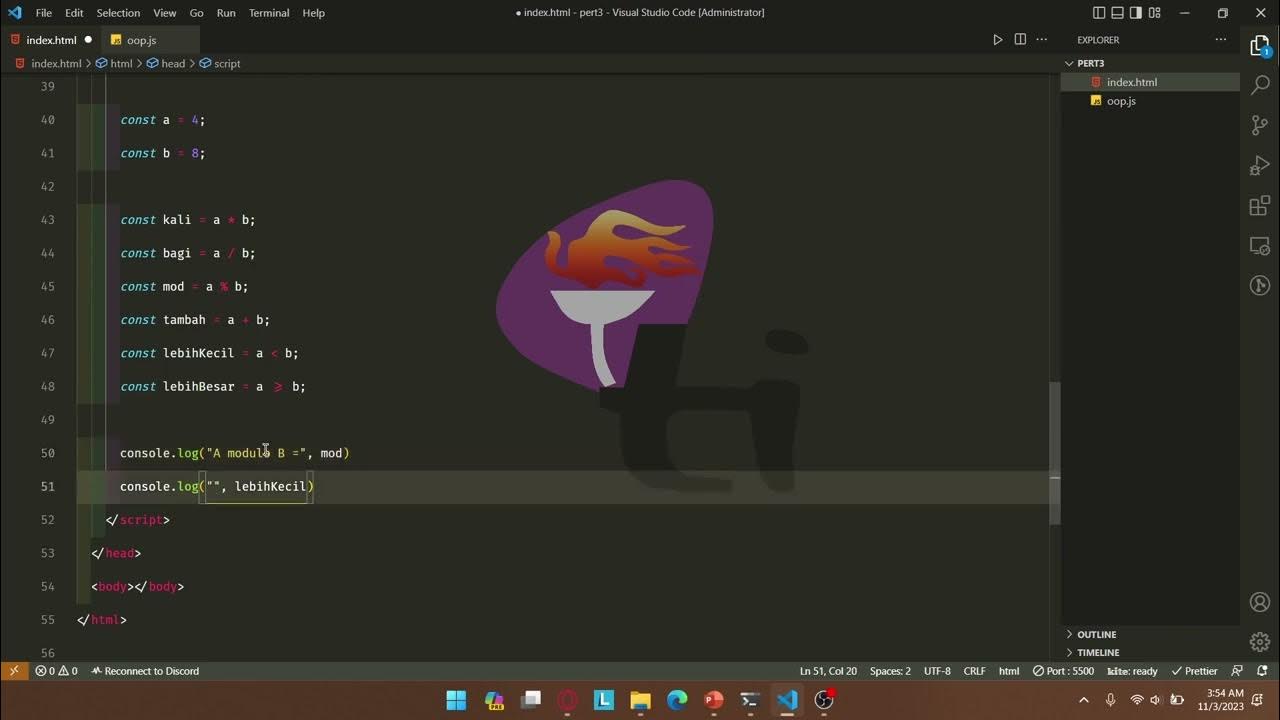
PWEB 3IA01 3IA02 | Pertemuan 3 | PJ Fierza
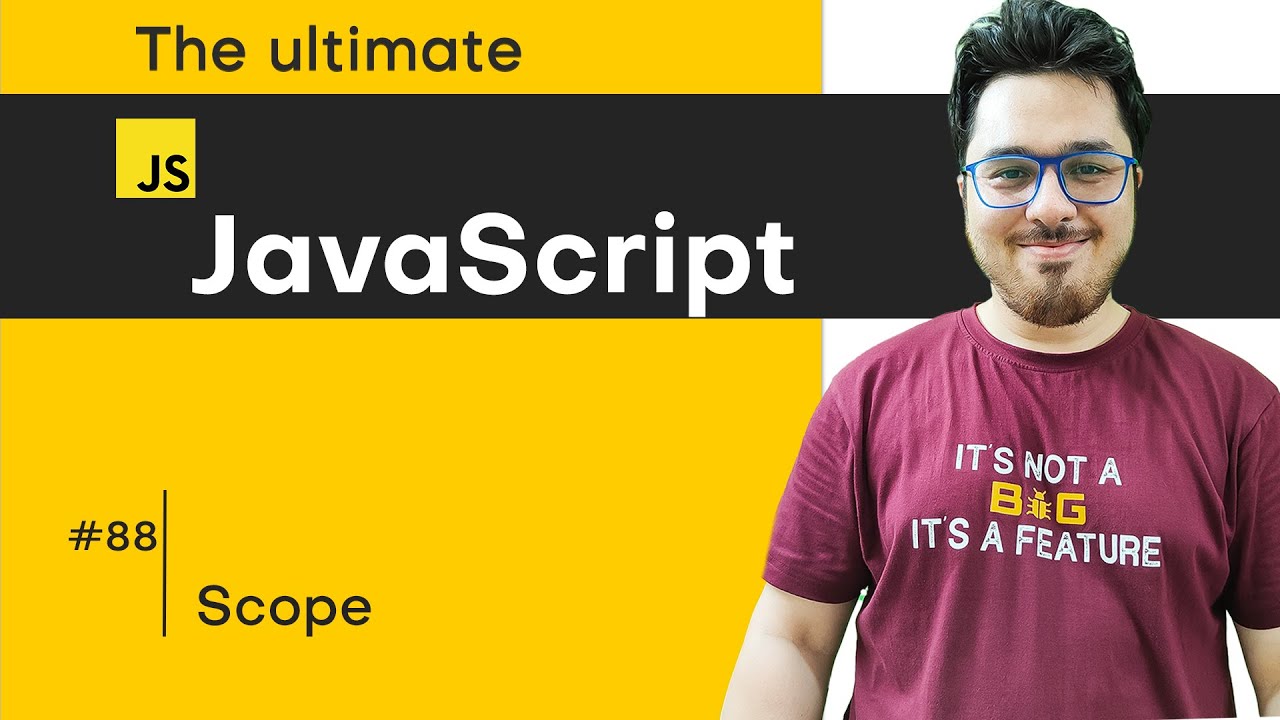
local and global Scope in JavaScript | JavaScript Tutorial in Hindi #88
5.0 / 5 (0 votes)