Senior Frontend Developer Interview Questions 2024
Summary
TLDRIn this video, Senior Dev and bden explore advanced front-end topics, focusing on webpack, CSS-in-JS, React, and web performance. They discuss webpack's role in module bundling and tree shaking, the dynamic capabilities of CSS-in-JS, and React's component patterns including error boundaries and hooks. The conversation delves into state management strategies, the balance between essential and derived state, and the implications of using React context for state. Testing approaches for React applications are examined, emphasizing the importance of a mix between unit, integration, and end-to-end tests. The discussion concludes with web performance optimization, particularly focusing on improving First Contentful Paint (FCP) and considering server-side rendering for faster load times.
Takeaways
- 🔧 Webpack is a module bundler primarily used to combine multiple JavaScript files into a single bundle for efficient loading.
- 🌳 Tree shaking is a technique used by Webpack to remove unused code from the final bundle, optimizing the application size.
- 🚀 Webpack's tree shaking requires ES6 static imports and won't work with CommonJS dynamic imports, which are evaluated at runtime.
- 🌐 Dependency graph is a structure built by Webpack starting from the entry point, tracing all imports to form a tree of modules.
- 💄 CSS-in-JS allows for dynamic styling by writing CSS inside JavaScript files, enabling style changes based on component state or props.
- 🚨 CSS-in-JS can lead to larger bundles, difficulty in caching styles, and challenges in debugging due to generated class names with unique hashes.
- ⚖️ React's PureComponent helps in preventing unnecessary re-renders by shallowly comparing props and state, but is less necessary with Hooks.
- 🛡️ Error boundaries in React catch JavaScript errors in child components, prevent the error from propagating, and allow UI recovery.
- ⚙️ useEffect Hook in React is used for handling side effects, such as API calls or subscriptions, in function components.
- 🔄 React's Context API is suitable for managing global state like authentication and user settings that affect the entire application.
- 📊 Code coverage is important but should be balanced; aiming for 60-70% in front-end applications can be practical while avoiding the pitfalls of excessive testing for the sake of coverage.
Q & A
What is Webpack and what is it used for?
-Webpack is a module bundler primarily used for bundling JavaScript files into a single file. It was necessary because browsers required a script tag for each JavaScript file, which was impractical for large applications with many files.
What is tree shaking in the context of Webpack?
-Tree shaking in Webpack refers to the process of eliminating unused code from the final bundle during production. It identifies and removes modules or parts of modules that are imported but not actually used in the code.
How does tree shaking work with ES6 static imports in Webpack?
-Tree shaking works with ES6 static imports because Webpack can understand and track these imports to build a dependency tree, allowing it to determine which parts of the code are not used and can be removed.
Why can't CommonJS modules with 'require' be tree shaken by Webpack?
-CommonJS modules with 'require' cannot be tree shaken because they are dynamic and evaluated at runtime. Webpack cannot use these to build a dependency tree to determine which code to drop, as it cannot predict what will be executed.
What is a dependency graph in Webpack and how is it used?
-A dependency graph in Webpack is a structure that Webpack builds from the entry point of the application. It traverses all import statements to form a tree structure that represents all modules. This graph is used to optimize the bundle by determining which modules to include or exclude.
What is CSS-in-JS and why is it used?
-CSS-in-JS is a technique where CSS is written inside JavaScript files. It allows for dynamic styling based on JavaScript variables and state, enabling styles to change in response to user interactions or other runtime events.
What are some disadvantages of using CSS-in-JS?
-Disadvantages of CSS-in-JS include the inability to perform certain CSS optimizations like caching, making the CSS non-cacheable and potentially increasing load times. It can also complicate debugging due to unique hash class names generated by tools like Webpack and may lead to performance issues like cumulative layout shift.
What is the difference between a Pure Component and a Functional Component in React?
-A Pure Component in React is a class component that avoids unnecessary re-renders by comparing incoming props with existing props. A Functional Component is a component written as a JavaScript function, which can use Hooks to manage state and side effects without the need for a class-based approach.
What is an Error Boundary in React and why is it used?
-An Error Boundary in React is a component that catches JavaScript errors anywhere in its child component tree, logs those errors, and displays a fallback UI instead of the component tree that crashed. It helps in isolating errors and preventing the entire application from crashing.
Can you explain the useEffect Hook in React and its purpose?
-The useEffect Hook in React is used to perform side effects in function components. It allows you to perform operations such as data fetching, subscribing to subscriptions, or manually changing the DOM in response to state or prop changes.
Why shouldn't you use an async function as a callback to useEffect?
-You shouldn't use an async function as a callback to useEffect because useEffect expects a cleanup function to be returned, which allows for cleaning up side effects. An async function implicitly returns a promise, which React cannot use as a cleanup function, leading to potential memory leaks or unintended behavior.
Outlines
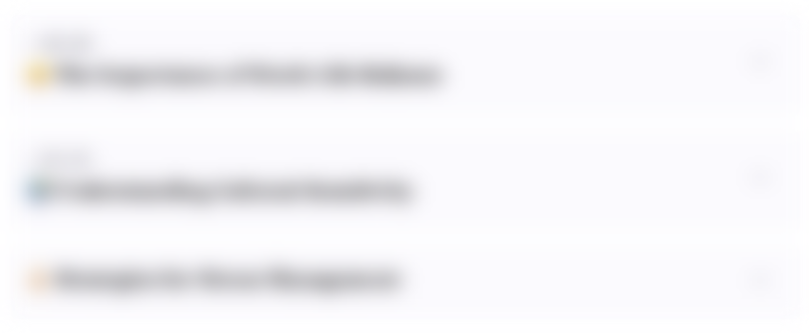
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
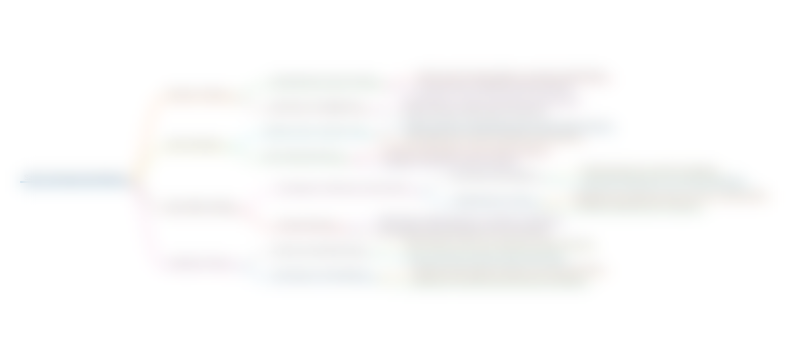
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
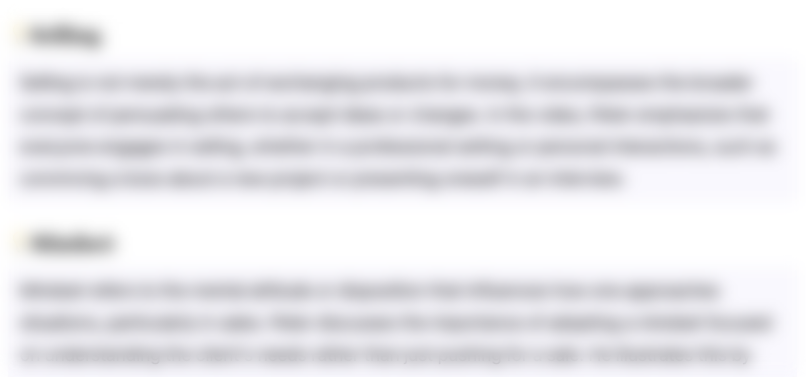
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
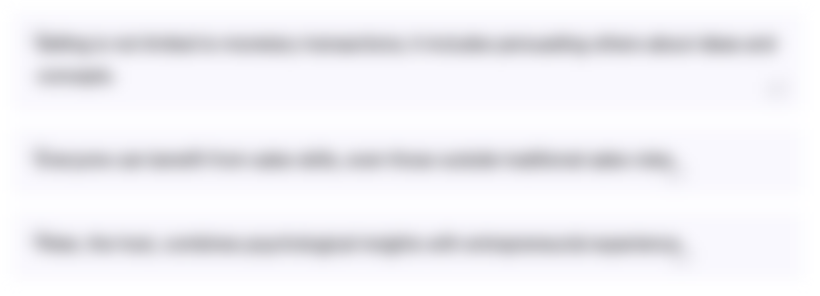
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
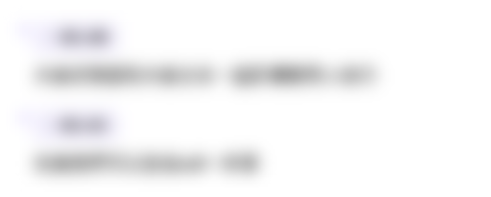
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados

The Ultimate 2025 Front-End developer Roadmap
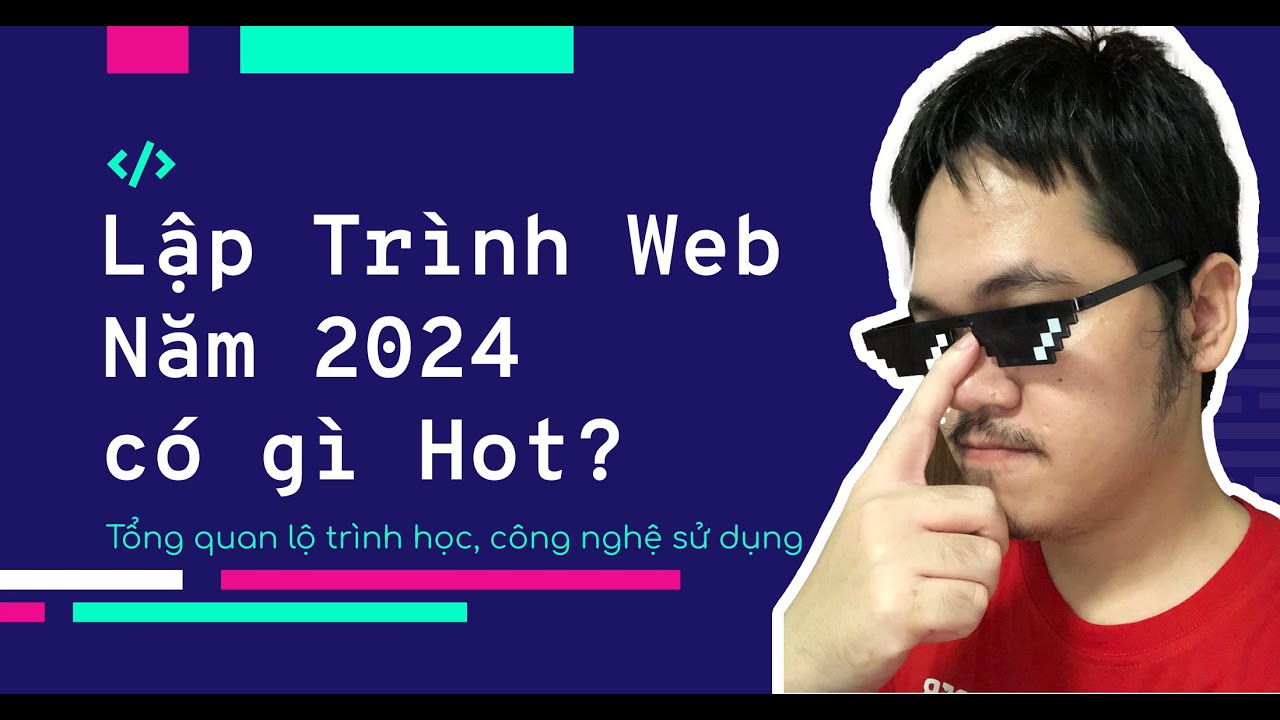
Tổng hợp Full Lộ Trình và các Công Nghệ Web nên học năm 2024
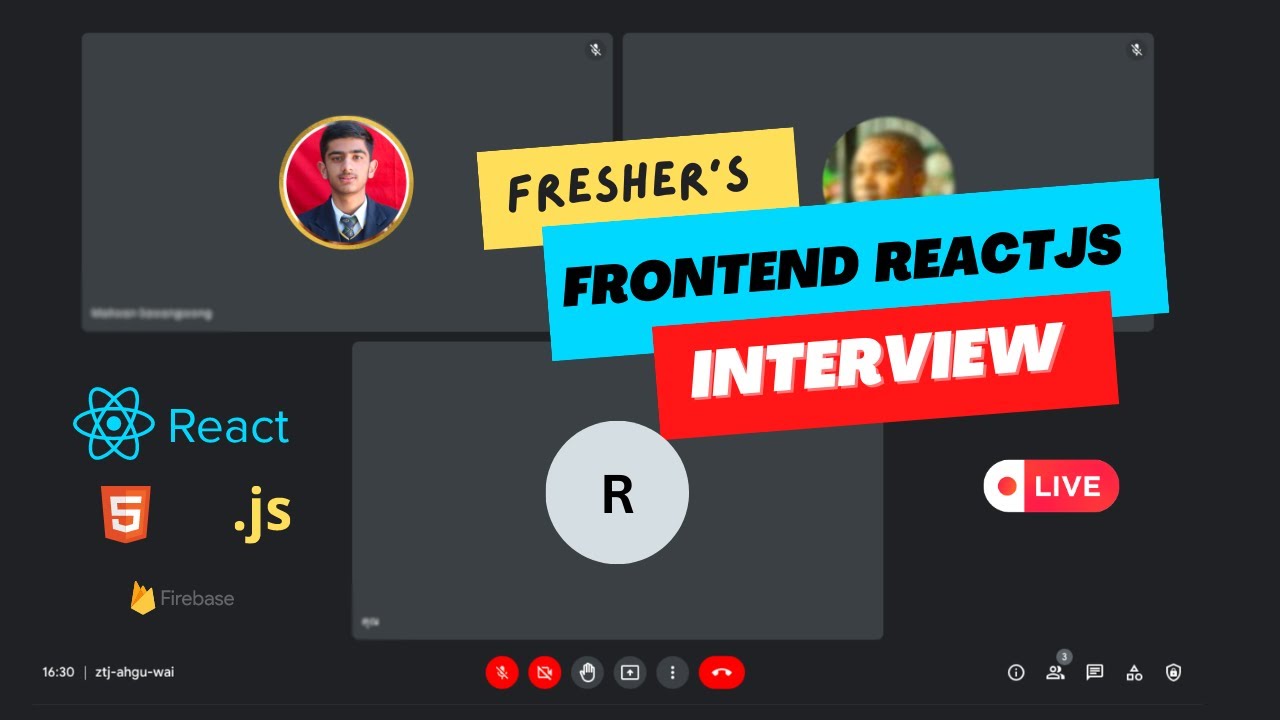
Fresher's Frontend Interview 🎉 | JavaScript | #reactjs (Mock) [Most Asked Questions -2023]
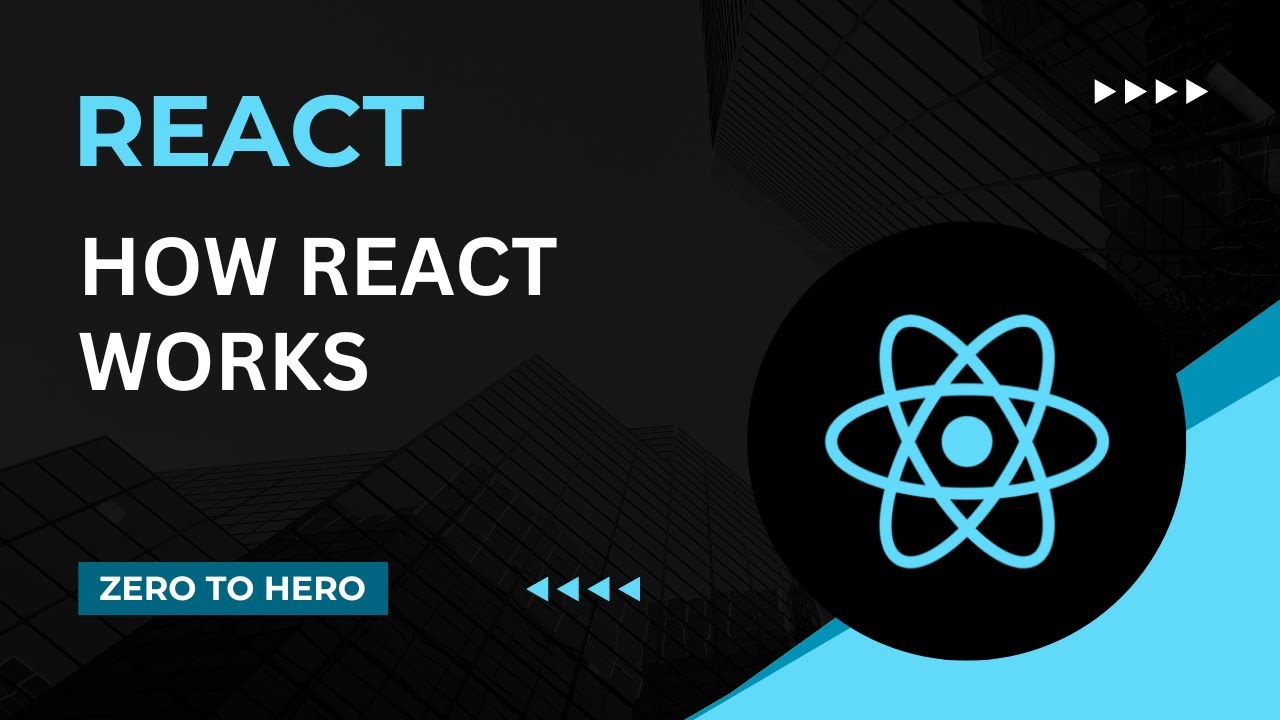
How React Works | Mastering React: An In-Depth Zero to Hero Video Series
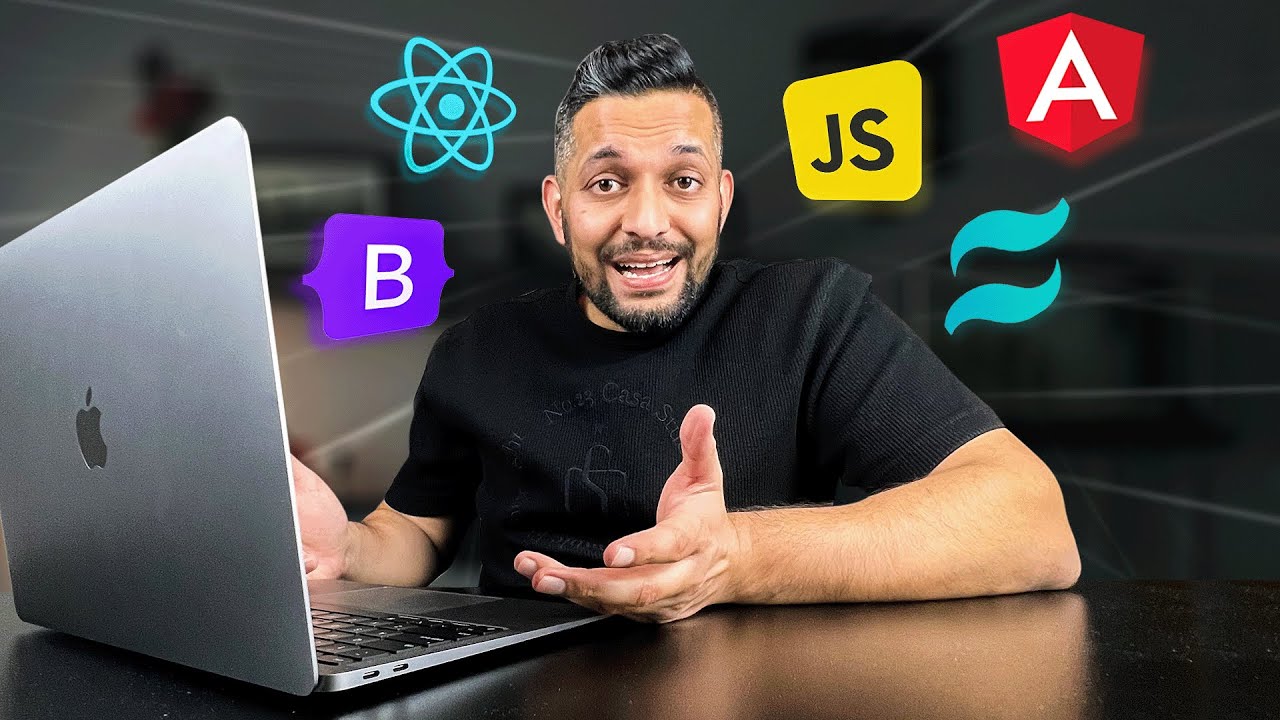
Fastest way to become a Web Developer in 2024
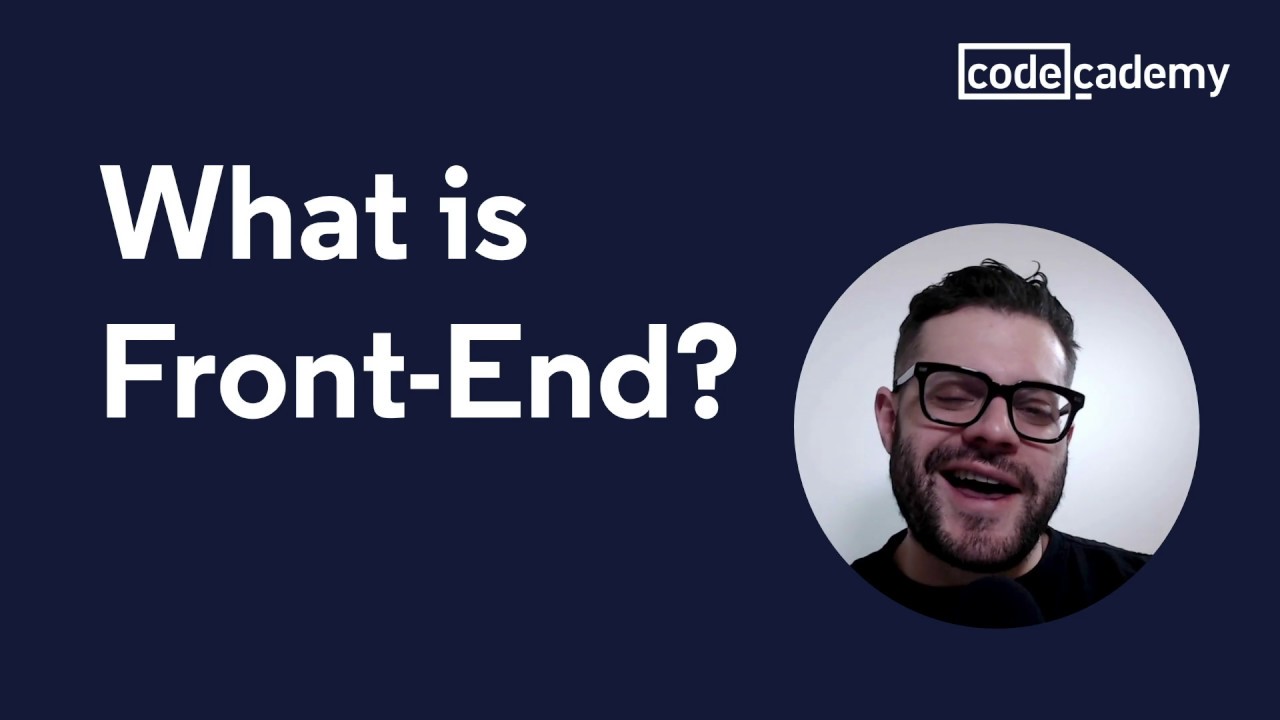
What is front-end?
5.0 / 5 (0 votes)