For Statement (Contd.)
Summary
TLDRThis script discusses the use of the for loop in programming, exploring its structure including initialization, condition checking, and modulator expressions. It highlights the ability to use arithmetic expressions within the loop and the potential for infinite loops. Examples are provided to illustrate how to compute factorials and sum of squares using for loops. The script also touches on the use of the break and continue statements to control loop execution, emphasizing best practices for readability and logic in programming.
Takeaways
- 🔁 The for loop is a control structure used for creating loops in a program, consisting of initialization, condition checking, and a modulator (alteration) expression.
- 📐 Arithmetic expressions are used within the for loop for initialization and condition checking, allowing for complex conditions and variable manipulation.
- 🔄 The modulator expression can be used to increment or decrement the loop control variable, or to modify it in other ways, hence it's sometimes better referred to as an alteration expression.
- ⏪ The for loop can be used to create loops that count down, not just up, by using a decrementing modulator expression.
- 🚫 If the loop continuation condition is false from the start, the body of the for loop will not be executed even once.
- 🎲 Examples provided in the script demonstrate how to compute factorials and sum of squares using for loops, showcasing their versatility.
- 🔀 The comma operator allows for multiple initialization or alteration expressions within the for loop, although it's advised against for beginners due to potential readability issues.
- 🔁 Infinite loops can be created using for loops by omitting the initialization, condition, and modulator expressions, resulting in a loop that runs indefinitely.
- 🛑 The break statement can be used to exit a for loop prematurely when a specific condition is met, allowing for early termination of the loop.
- 🔁 The continue statement is used to skip the remaining part of the loop body and proceed to the next iteration of the loop.
Q & A
What is the purpose of the 'for' statement in programming?
-The 'for' statement is used to create loops in a program, allowing for repeated execution of a block of code as long as a specified condition is true.
What are the three main components of a 'for' loop?
-The three main components of a 'for' loop are the initialization expression, the loop continuation condition, and the alteration expression.
Can the increment in a 'for' loop be negative?
-Yes, the increment in a 'for' loop can be negative, allowing the loop to count down or decrement the loop control variable.
What is an infinite loop and how can it be created using a 'for' loop?
-An infinite loop is a loop that continues to execute indefinitely because the loop continuation condition never becomes false. It can be created using a 'for' loop by omitting the initialization, condition, and alteration parts, or by setting the condition to always be true.
What is the role of the 'break' statement in loops?
-The 'break' statement is used to exit a loop prematurely when a certain condition is met, preventing further iterations of the loop.
How does the 'continue' statement function within a loop?
-The 'continue' statement is used to skip the remaining part of the loop body and proceed to the next iteration of the loop.
What is the significance of the comma operator in the context of 'for' loops?
-The comma operator allows multiple expressions to be included in the initialization or alteration part of a 'for' loop, separating them with commas.
Why might a programmer choose to use a 'do while' loop instead of a 'for' loop?
-A programmer might choose to use a 'do while' loop instead of a 'for' loop when they want to ensure that the loop body executes at least once before the condition is checked.
Can you provide an example of how a 'for' loop can be used to compute the factorial of a number?
-A 'for' loop can be used to compute the factorial of a number by initializing a variable to 1, using a loop to multiply this variable by each integer up to the number, and breaking the loop when the factorial exceeds a certain value.
What is the difference between a 'for' loop and a 'while' loop in terms of their syntax and usage?
-A 'for' loop is more compact and is typically used when the number of iterations is known beforehand, while a 'while' loop is used when the number of iterations is not known and depends on a condition that is checked before each iteration.
Outlines
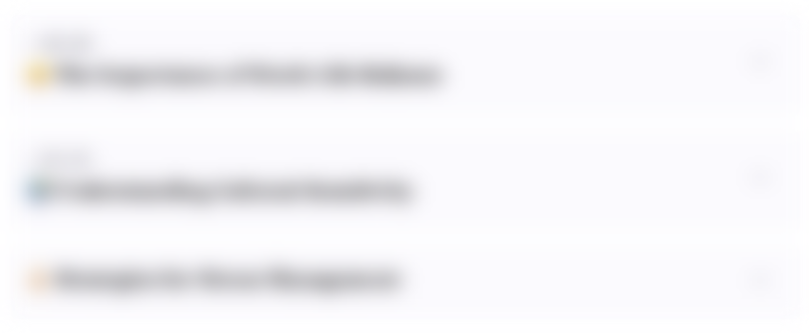
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
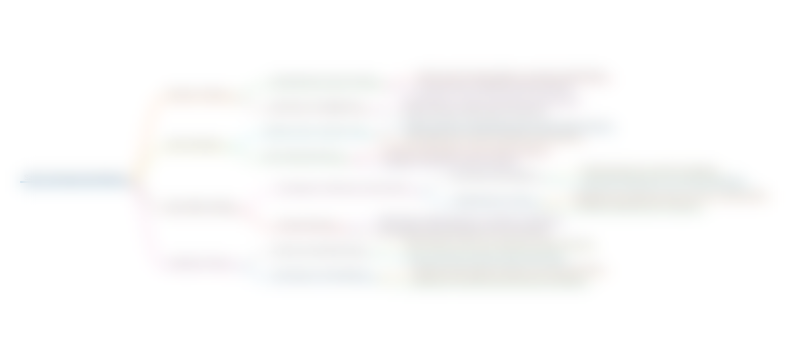
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
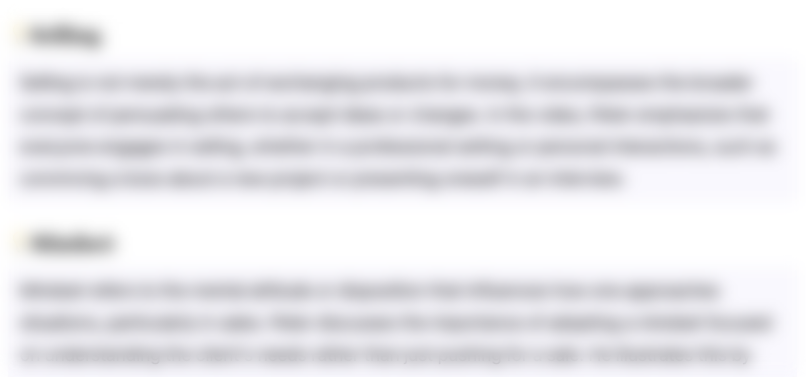
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
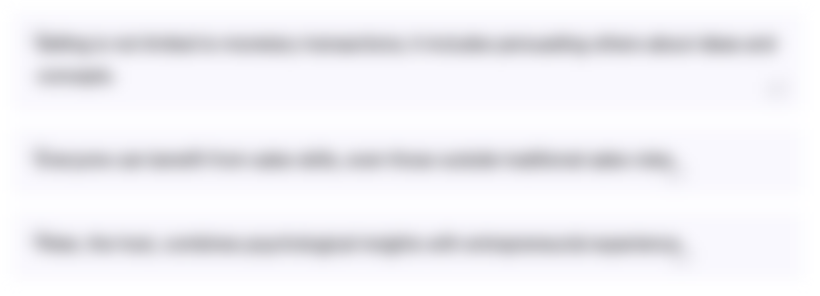
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
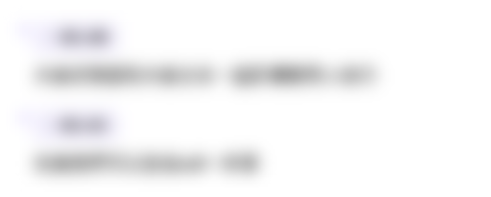
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahora5.0 / 5 (0 votes)