Understanding the basic structure of a SwiftUI app – WeSplit SwiftUI Tutorial 1/11
Summary
TLDRThis video script offers a detailed introduction to creating a new Swift UI project in Xcode. It explains the structure of the project, including the main files and their purposes, such as 'AppDelegate.swift' for app lifecycle management and 'ContentView.swift' for the initial user interface. The script delves into the Swift UI framework, highlighting the use of view modifiers and the 'VStack' for layout. It also introduces the concept of previews in Xcode, which allows developers to see a live version of their project and interact with it directly within the canvas, emphasizing the importance of this feature for design-time previews without affecting the final app.
Takeaways
- 📁 When starting a new Swift UI project in Xcode, you get multiple files and code lines, with the Project Navigator on the left showing all the files.
- 🚀 The 'App.Swift' file contains the main code to launch your app and maintain data while the app runs.
- 💻 'ContentView.Swift' is where the initial user interface is coded and where all the project's coding will occur.
- 🎨 The 'Assets' catalog is where you can add images, colors, app icons, and other visual elements for the app.
- 🛠️ The 'Preview Content' group has its own asset catalog specifically for previewing data within Xcode.
- 🔍 File extensions might be hidden depending on Xcode settings; you can change this in the General tab under settings.
- 📜 The 'ContentView' struct conforms to the 'View' protocol, which is essential for displaying any data on the screen.
- 🖼️ The 'body' property in 'ContentView' returns a view layout, which can include images, text, and other elements.
- ⚙️ Swift UI's view modifiers, like 'padding' and 'foregroundStyle', allow you to modify views while returning new ones.
- 🖥️ The 'Canvas' in Xcode allows you to preview your app's UI in real-time and is interactive, making it easier to test and adjust your design.
Q & A
What is the purpose of the 'WeitApp.swift' file in a new Swift UI project?
-The 'WeitApp.swift' file contains the main code to launch the app and display the initial user interface. It also serves as the place to initialize and maintain data that should persist throughout the app's runtime.
Where do you write most of your code in a new Swift UI project?
-Most of the coding for a new Swift UI project is done in the 'ContentView.swift' file, which contains the initial user interface for the app.
What is the role of the 'Assets' folder in an Xcode project?
-The 'Assets' folder is the asset catalog where you can add pictures, colors, app icons, and other resources like iMessage stickers, which can be used in your app.
How can you preview your UI design in Xcode?
-You can preview your UI design using the canvas in Xcode, which renders a live version of your project. The canvas can be enabled or disabled through the 'Editor' menu.
What is the 'SwiftUI' framework used for in a Swift UI project?
-The 'SwiftUI' framework provides the functionality needed to create user interfaces in Swift. It includes tools for working with text, images, buttons, and more.
What does the 'ContentView' struct do in a Swift UI project?
-The 'ContentView' struct defines the initial view of the app and conforms to the 'View' protocol, which is essential for displaying content on the screen.
What is the significance of the 'body' property in a Swift UI view?
-The 'body' property in a Swift UI view is a computed property that returns some type of view conforming to the 'View' protocol. It dictates the layout and appearance of the view's content.
How does the 'View Modifier' work in Swift UI?
-A 'View Modifier' in Swift UI is a method that returns a new view by applying modifications to the original data. For example, it can change an image's scale or add padding.
What is the purpose of the '#Preview' code in a Swift UI project?
-The '#Preview' code allows you to render a live preview of your app's UI in Xcode. It doesn't affect the final app but is crucial for design-time visualization.
How can you change the device used in the Xcode canvas preview?
-You can change the device in the Xcode canvas preview by selecting a different device from the list that appears when you click on the current device name. This will also change the simulator used when running the app.
Outlines
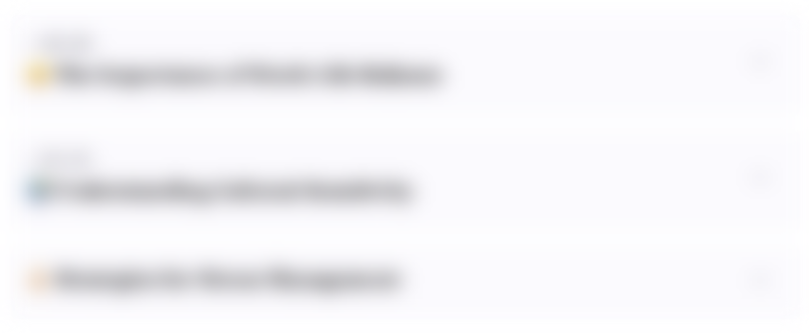
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
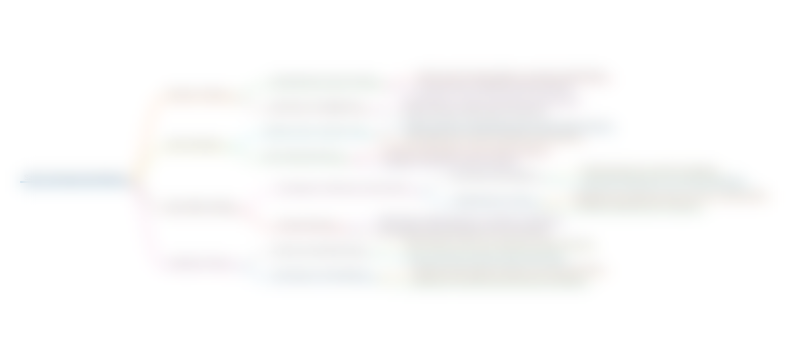
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
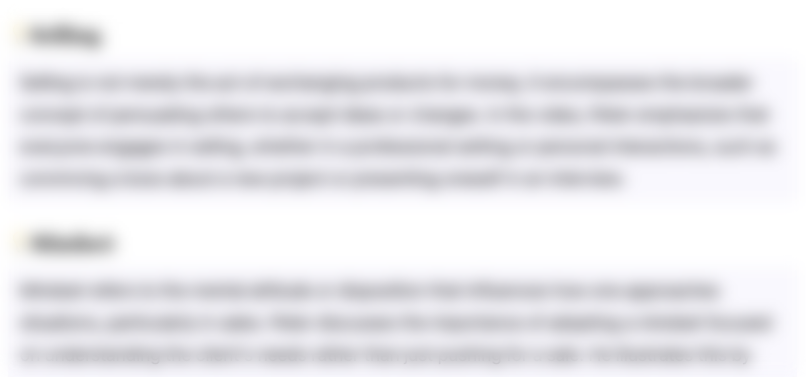
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
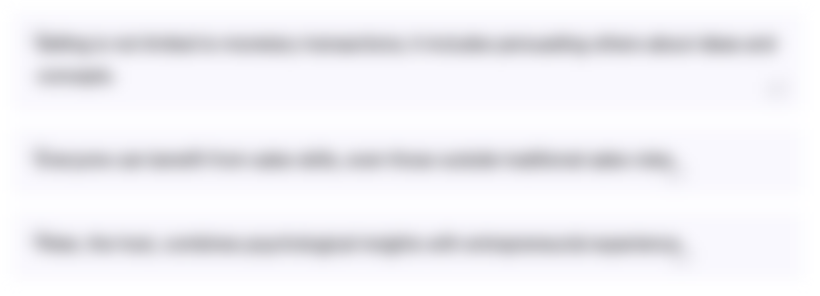
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
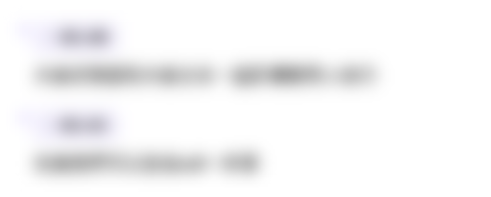
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen
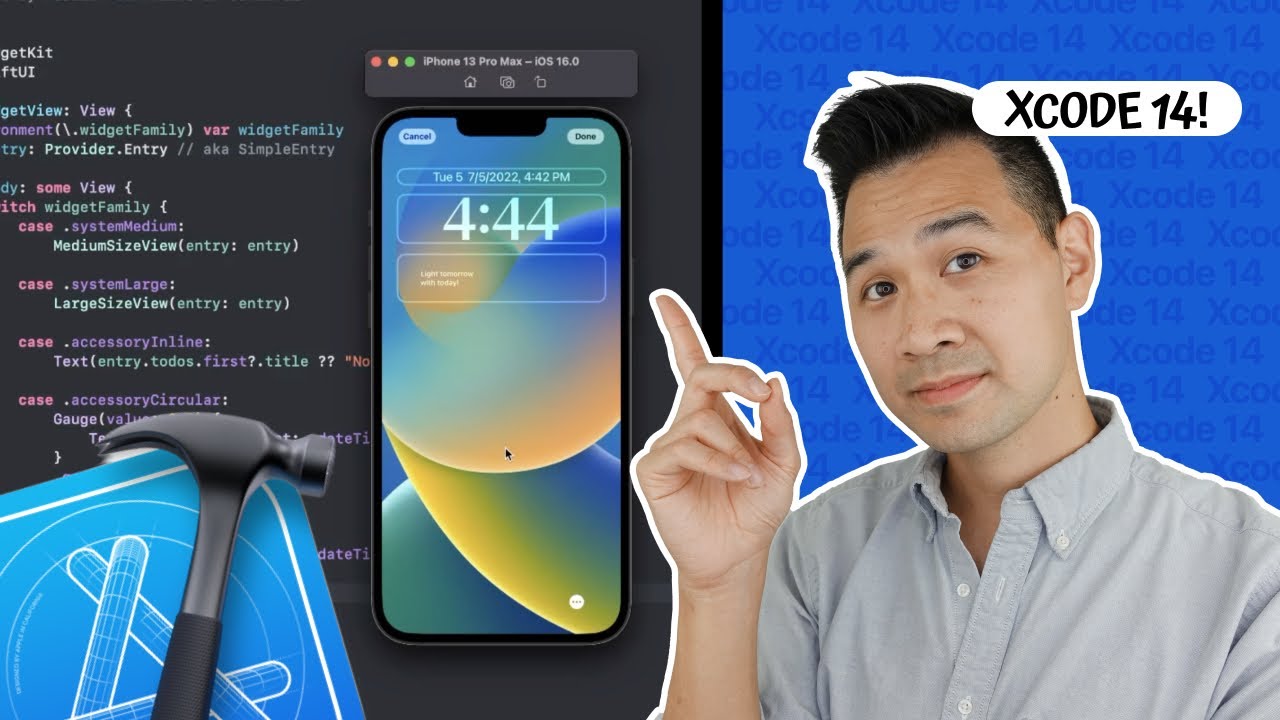
Xcode Tutorial - Step by Step for Beginners
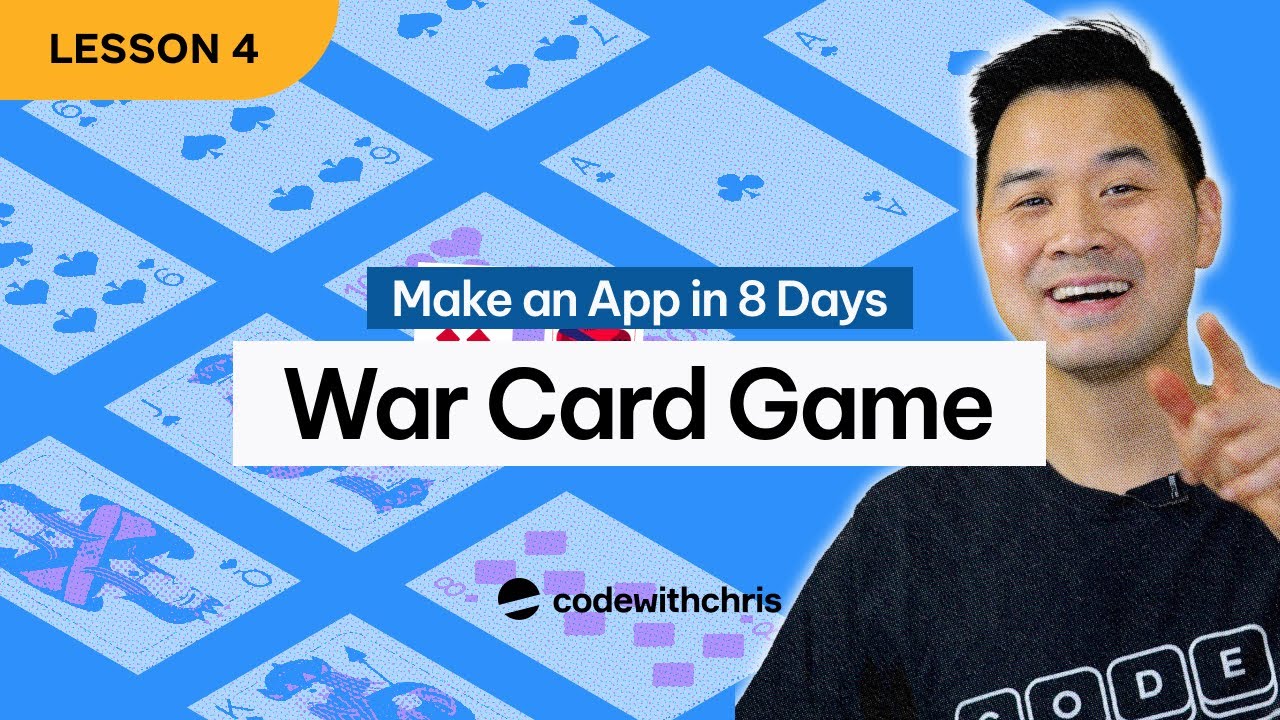
Starting The War Card Game App - Lesson 4 (2024 / SwiftUI)
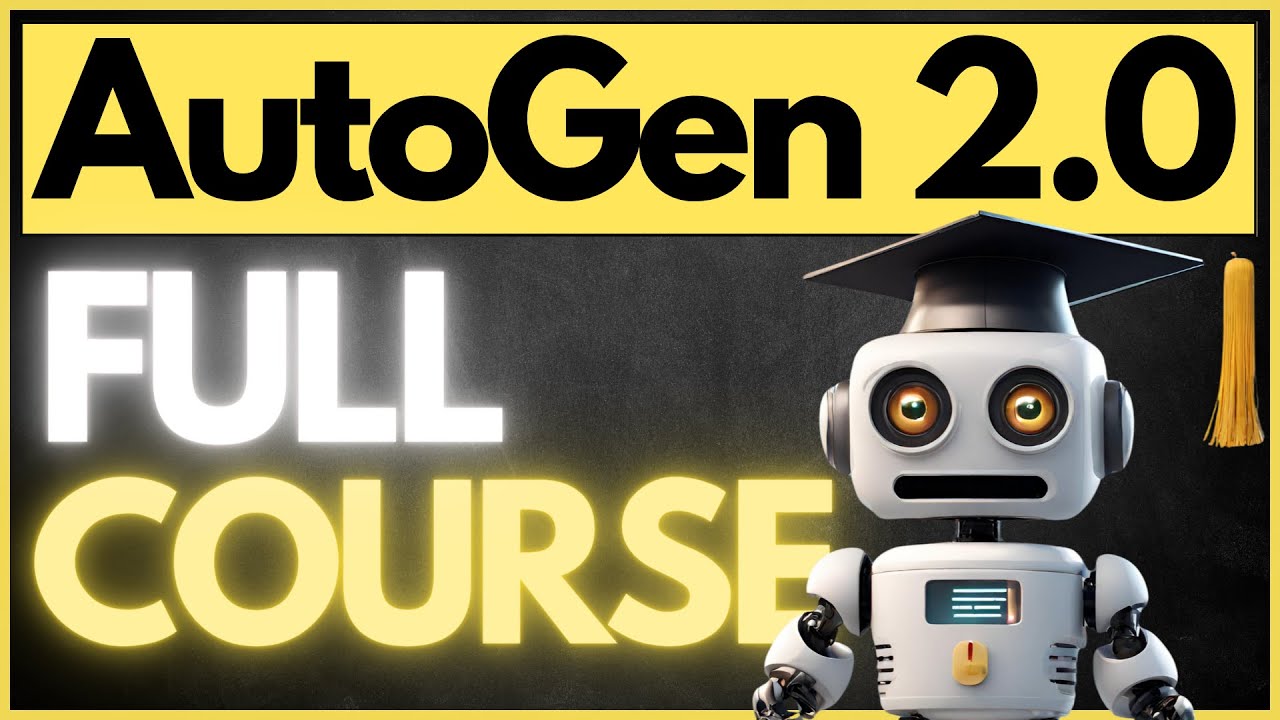
AutoGen Studio 2.0 Full Course - NO CODE AI Agent Builder
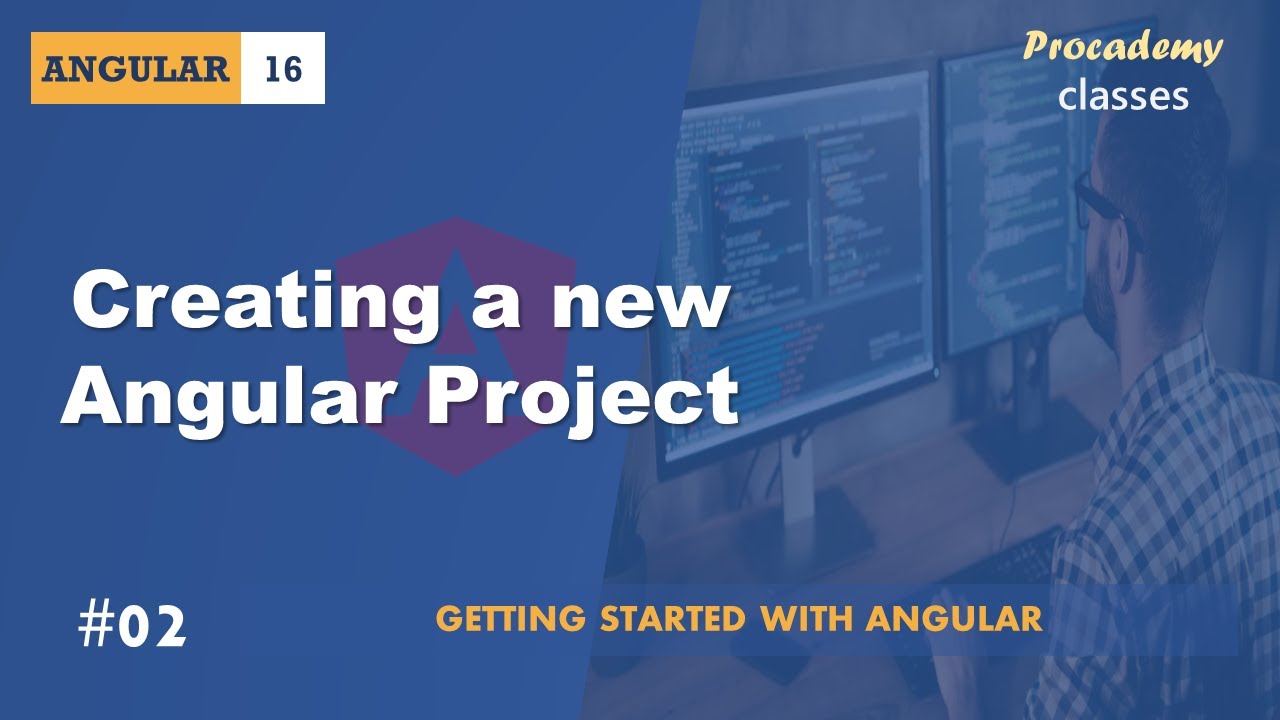
#02 Creating a new Angular Project | Getting Started with Angular | A Complete Angular Course
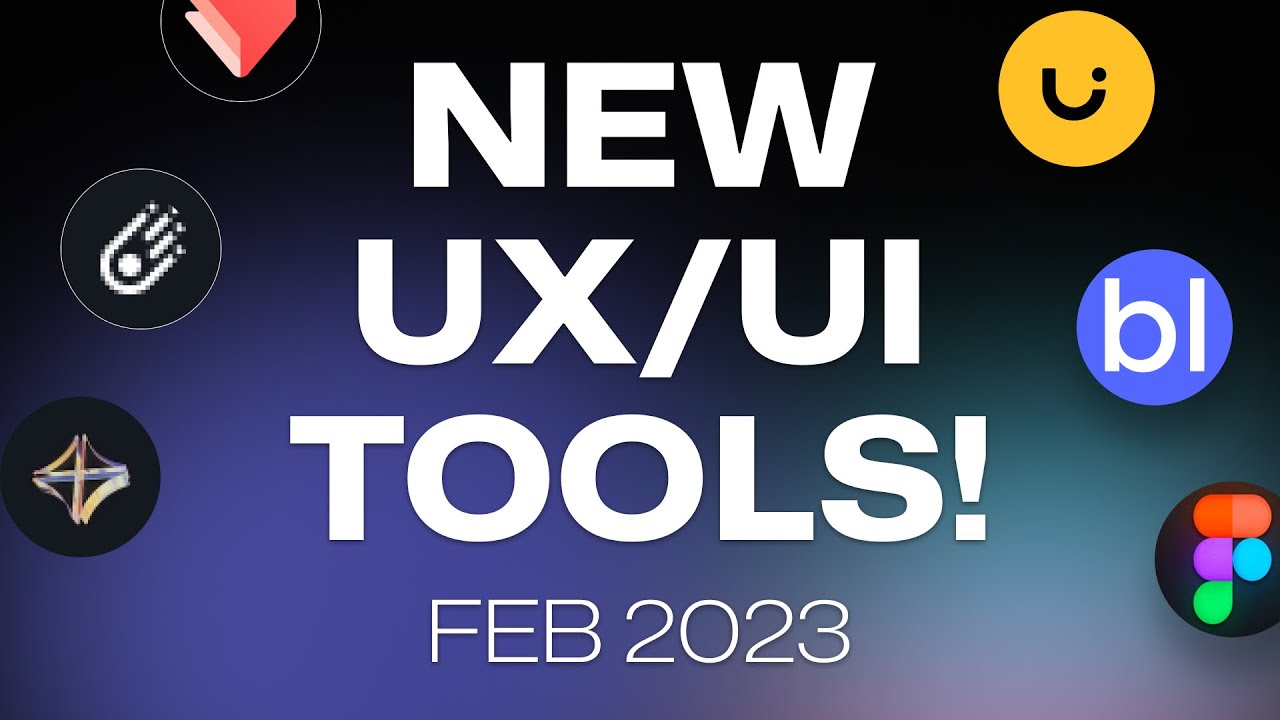
Surprising New UX/UI Design Tools: A.I. UI Design Tool, Premium Giveaway, Portfolio Tools – Feb 2023

Video Pertemuan 3 (Pemrograman Mobile) - Jelly Leliana Purba 2 SI A
5.0 / 5 (0 votes)