Python Tutorial for Beginners 2: Strings - Working with Textual Data
Summary
TLDRThis video tutorial offers an in-depth exploration of Python's string data type, demonstrating how to create, manipulate, and utilize strings effectively. It covers variable naming conventions, string indexing, slicing, and methods like `lower()`, `upper()`, `count()`, `find()`, and `replace()`. The tutorial also introduces advanced string operations, including formatted strings, f-strings for Python 3.6+, and using `dir()` and `help()` functions to discover available string methods. Aimed at beginners, the video simplifies complex concepts, making Python's string handling accessible and engaging.
Takeaways
- 📝 Python strings are used to represent textual data and are created using single or double quotes.
- 🔡 Python does not require semicolons to end statements; it relies on whitespace for structure.
- 🔑 Variable names in Python should be lowercase with words separated by underscores for readability.
- 📐 The `len()` function in Python is used to find the length of a string.
- 👉 To access individual characters in a string, use indexing with square brackets, starting from index 0.
- ❗ Attempting to access an index that doesn't exist in a string will result in an IndexError.
- 🔄 String methods like `lower()`, `upper()`, `count()`, `find()`, and `replace()` allow for manipulation and analysis of string data.
- 🔑 The `dir()` function can be used to list all methods and attributes available for a given object in Python.
- 📚 The `help()` function provides detailed information about Python's built-in functions and methods.
- 💬 String concatenation can be achieved using the `+` operator or by using formatted strings for more complex constructions.
- 🆕 Python 3.6 introduced f-strings, which simplify the process of inserting variables into strings with ease.
Q & A
What is the purpose of the video?
-The video aims to teach viewers about Python data types, specifically focusing on how to work with textual data using strings.
How do you create a variable to hold a text value in Python?
-You can create a variable to hold a text value by assigning a string to it, such as `message = 'hello world'`.
Why does Python not require semicolons to end each line?
-Python operates on whitespace, making it a clean language to work with, and does not require semicolons to end each line.
What are the conventions for naming variables in Python?
-Variables are usually named in lowercase, and if they consist of multiple words, the words are separated by underscores. The names should be descriptive to indicate the value they hold.
How can you include a single quote within a string that uses single quotes?
-You can either escape the single quote with a backslash (`\`), or you can use double quotes to enclose the string.
What is the difference between using single quotes and double quotes for strings in Python?
-There is no functional difference between using single quotes and double quotes for strings in Python; it depends on the content of the string. If the string contains a single quote, use double quotes to avoid syntax errors and vice versa.
How do you create a multi-line string in Python?
-You can create a multi-line string by enclosing the string in triple quotes (`'''` or `"""`).
How do you find the length of a string in Python?
-You can find the length of a string using the `len` function, such as `len(message)`.
How do you access individual characters in a string?
-You can access individual characters in a string using the index notation with square brackets, such as `message[0]` for the first character.
What is string slicing and how is it done?
-String slicing is a way to access a range of characters in a string. It is done using the notation `string[start:stop]`, where `start` is inclusive and `stop` is exclusive.
What are some common string methods in Python?
-Common string methods include `.lower()`, `.upper()`, `.count()`, `.find()`, and `.replace()`.
How do you concatenate strings in Python?
-You can concatenate strings using the `+` operator, such as `greeting + ', ' + name`, or using formatted strings with placeholders.
What are formatted strings and how are they used?
-Formatted strings allow you to create a string with placeholders that are replaced with variable values using the `.format()` method or f-strings (for Python 3.6+).
What are f-strings and how do they work?
-F-strings are a way to embed expressions inside string literals using curly braces `{}`. They are prefixed with an `f` or `F` and allow for expressions within the placeholders, like `f'{name.upper()}'`.
How can you find all methods and attributes available to a string variable?
-You can use the `dir(variable)` function to list all methods and attributes available to a string variable.
How can you get detailed information about a specific string method?
-You can use the `help()` function with the string class and method name, such as `help(str.lower)`, to get detailed information about a specific string method.
What should you do if you want to replace a substring in a string?
-Use the `.replace()` method, which takes two arguments: the substring to be replaced and the new substring, such as `message.replace('world', 'universe')`.
Outlines
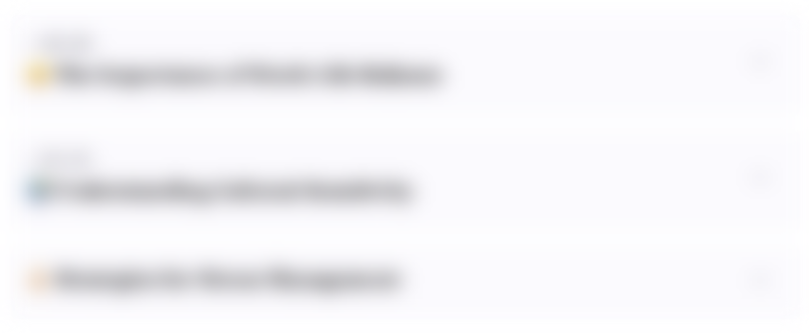
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
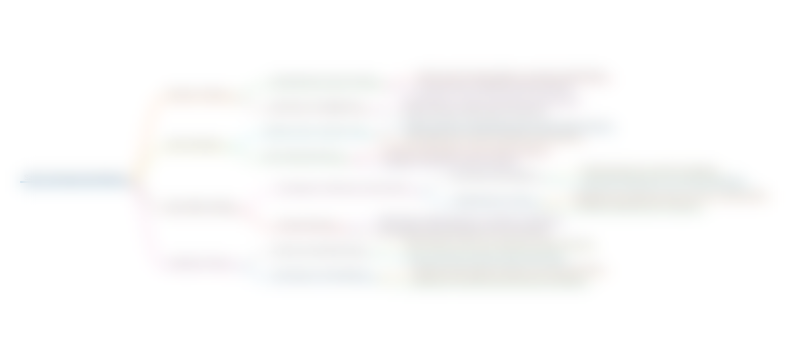
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
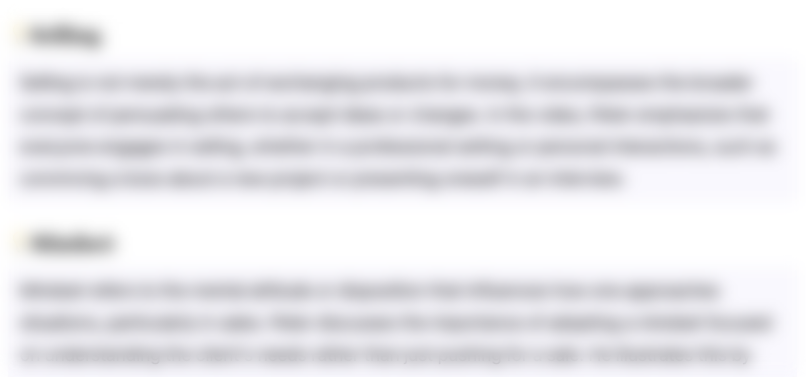
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
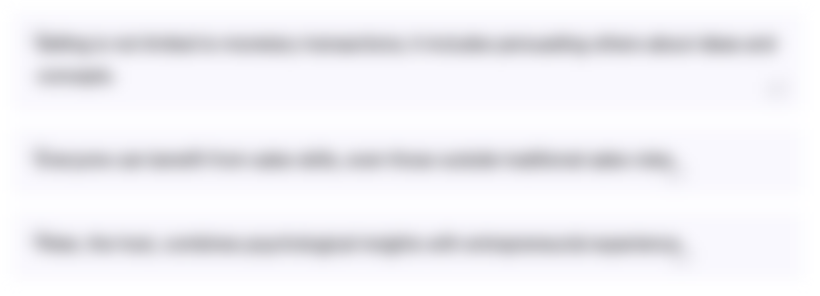
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
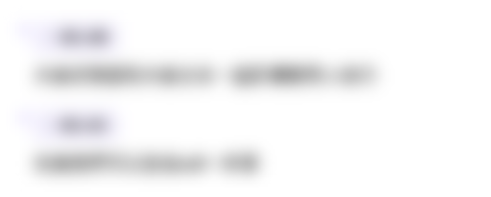
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführen5.0 / 5 (0 votes)