Using Arrays
Summary
TLDRThis video covers the essentials of working with arrays in Java. It explains how to iterate over arrays using loops, sum array elements, and handle exceptions like ArrayIndexOutOfBounds. The tutorial also demonstrates the use of arrays in object-oriented programming, including their role as instance variables in classes. Key concepts such as array references, the fixed size of arrays, and how changes in one reference affect others are also discussed. The video wraps up with examples of handling student and classroom data, showcasing the practical use of arrays in real-world applications.
Takeaways
- đ Iterating over an array is done using a for loop, where the loop goes through each element by accessing the array with its index.
- đ Arrays can store both primitive data types and objects, and they can be used as instance variables or method parameters/return types.
- đ A runtime error, specifically an ArrayIndexOutOfBoundsException, occurs if you attempt to access an index outside the bounds of the array.
- đ When assigning arrays to another array (e.g., arr2 = arr), both arrays refer to the same object in memory, meaning changes to one array affect the other.
- đ Summing an array requires iterating over it with a loop, adding each element to a sum variable, and printing the final result.
- đ Arrays have a fixed size, so you must manually track how many elements are used, especially when working with arrays of objects.
- đ In object-oriented programming, arrays can be used in classes to represent collections of related data, like storing student objects in a classroom.
- đ Array indices start at 0, so when creating an array with a certain size, valid indices range from 0 to length - 1.
- đ It's essential to be aware of array bounds and use appropriate methods or checks to avoid exceptions related to invalid indices.
- đ When manipulating arrays, remember that arrays are reference types. Modifying an element in one array will affect all other arrays that reference the same array.
Q & A
What does 'iterating over an array' mean in programming?
-Iterating over an array means accessing each element of the array one by one, typically using a loop. In the script, this is done using a `for` loop to print each element of the array.
How do you sum the elements of an array in Java?
-To sum the elements of an array, you create a variable to hold the sum (e.g., `int sum = 0`), then loop through the array, adding each element to the sum within the loop.
What exception occurs when you try to access an invalid index in an array?
-Attempting to access an invalid index results in an `ArrayIndexOutOfBoundsException`, which is a runtime error.
How do arrays behave when assigned to another array in Java?
-When one array is assigned to another, both variables point to the same array in memory. This means that modifying one array will also modify the other, as they reference the same object.
Why is it important to track the indices when working with arrays?
-Itâs crucial to track indices because array indices in Java are zero-based, meaning the first element is at index 0. Trying to access an index that is out of bounds will result in an error.
What is an example of using an array as an instance variable in a class?
-In the script, the `Classroom` class has an instance variable `private Student[] students;` which is an array of `Student` objects. This is an example of using an array as an instance variable.
How can you use arrays in method parameters and return types?
-Arrays can be passed as parameters to methods, or methods can return arrays. For example, the method `private int[] getBestScores(Student[] students)` takes an array of `Student` objects as a parameter and returns an array of `int` values.
What is the significance of the 'arr2 = arr' operation in the script?
-'arr2 = arr' means that `arr2` is now pointing to the same array as `arr`. Changes to `arr` (like modifying an element) will also be reflected in `arr2` because they both reference the same array object.
What role does the `Student` class play in the example?
-The `Student` class represents a student with properties like `firstName`, `lastName`, and `examScores`. It includes methods to calculate the average exam score and print out individual exam scores.
What does the method `getAverage()` do in the `Student` class?
-The `getAverage()` method calculates the average score of a student by summing all their exam scores and dividing by the number of exams taken.
Outlines
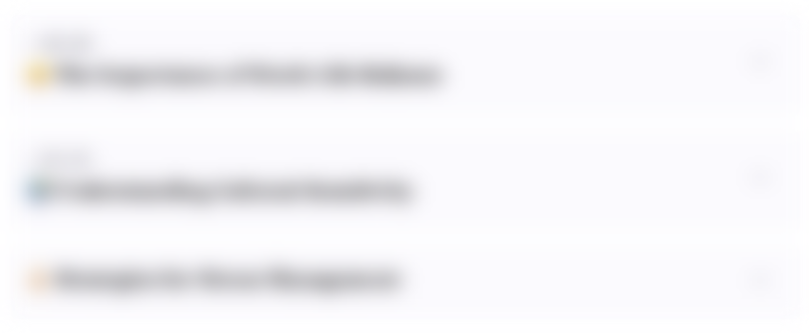
Dieser Bereich ist nur fĂŒr Premium-Benutzer verfĂŒgbar. Bitte fĂŒhren Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchfĂŒhrenMindmap
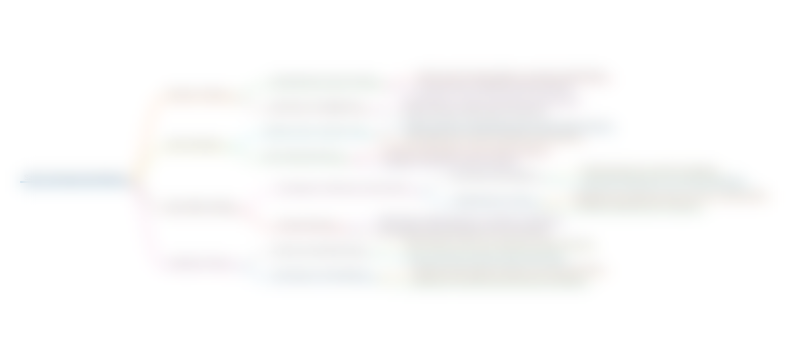
Dieser Bereich ist nur fĂŒr Premium-Benutzer verfĂŒgbar. Bitte fĂŒhren Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchfĂŒhrenKeywords
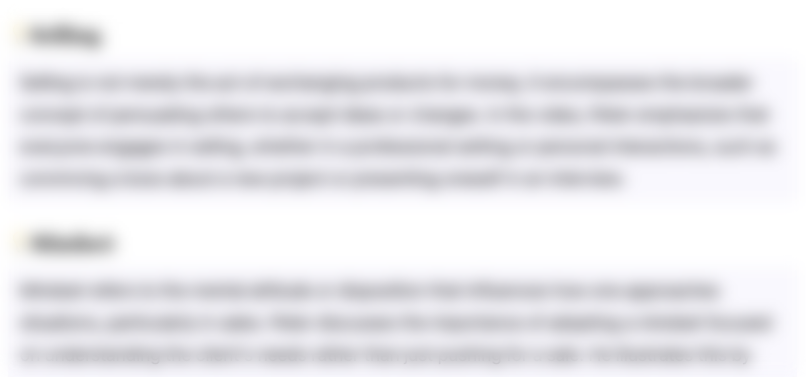
Dieser Bereich ist nur fĂŒr Premium-Benutzer verfĂŒgbar. Bitte fĂŒhren Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchfĂŒhrenHighlights
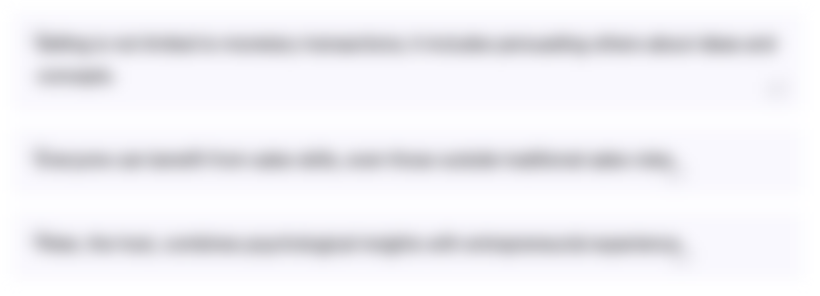
Dieser Bereich ist nur fĂŒr Premium-Benutzer verfĂŒgbar. Bitte fĂŒhren Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchfĂŒhrenTranscripts
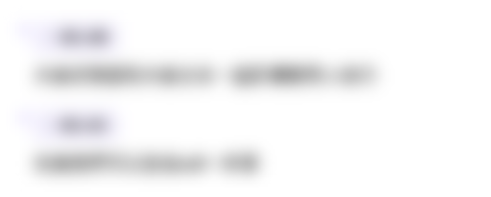
Dieser Bereich ist nur fĂŒr Premium-Benutzer verfĂŒgbar. Bitte fĂŒhren Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchfĂŒhrenWeitere Ă€hnliche Videos ansehen
5.0 / 5 (0 votes)