Contains Duplicate - Leetcode 217 - Python
Summary
TLDRIn this video, the host explores the problem of detecting duplicates in an array, emphasizing its importance for beginners and the variety of solutions available. The discussion begins with a brute force approach, highlighting its O(n²) time complexity and O(1) space complexity. Next, the host presents a sorting method, which reduces the time complexity to O(n log n). Finally, the optimal solution using a hash set is demonstrated, achieving O(n) time complexity at the cost of O(n) space. The video concludes with a clear Python code implementation, aiming to help viewers understand efficient strategies for solving this common problem.
Takeaways
- 😀 The 'Contains Duplicate' problem asks whether any value in a list appears at least twice.
- 😀 A brute force solution involves comparing each number against every other number, resulting in a time complexity of O(n²).
- 😀 Sorting the array is another approach that allows duplicates to be adjacent, reducing the problem to a single pass check with a time complexity of O(n log n).
- 😀 While sorting reduces the number of comparisons, it may require additional time due to the sorting process itself.
- 😀 Using a hash set allows for checking duplicates in O(1) time, significantly improving efficiency with an overall time complexity of O(n).
- 😀 The hash set method requires extra space, leading to a space complexity of O(n) as it can store up to n elements.
- 😀 The brute force method has no additional memory requirements, making its space complexity O(1).
- 😀 The hash set approach is often the most efficient, balancing time and space complexity effectively.
- 😀 Each of the discussed methods has its advantages and trade-offs, depending on the specific constraints of the problem.
- 😀 The implementation of the hash set approach in Python is straightforward and efficient for solving the 'Contains Duplicate' problem.
Q & A
What is the main problem addressed in the video?
-The main problem addressed is 'Contains Duplicate,' which asks whether any value in a given array of numbers appears at least twice.
What is the brute force method to solve this problem?
-The brute force method involves comparing each element in the array with every other element to check for duplicates, resulting in a time complexity of O(n²).
What is the time complexity of the brute force solution?
-The time complexity of the brute force solution is O(n²), where n is the number of elements in the array.
How does sorting help in finding duplicates?
-Sorting the array places duplicates next to each other, allowing for a single pass through the array to check adjacent elements for duplicates, which improves efficiency.
What is the time complexity of the sorting approach?
-The time complexity of the sorting approach is O(n log n) due to the sorting step, while the iteration through the array takes O(n).
What is the role of a hash set in the optimized solution?
-A hash set allows for O(1) average time complexity for insertions and lookups, enabling efficient checking for duplicates as we iterate through the array.
What is the time and space complexity of the hash set approach?
-The hash set approach has a time complexity of O(n) and a space complexity of O(n) since it may require additional space for storing elements.
Can you provide an example of input and output for the contains duplicate problem?
-For the input array [1, 2, 3, 1], the output would be true because the number 1 appears more than once.
What Python code is provided to implement the solution?
-The provided Python code initializes a hash set, iterates through the input array, checks for duplicates, and returns true or false based on whether duplicates are found.
Why is it beneficial to consider multiple approaches to a coding problem?
-Considering multiple approaches allows for a deeper understanding of the problem, helps identify the most efficient solution in terms of time and space complexity, and enhances problem-solving skills.
Outlines
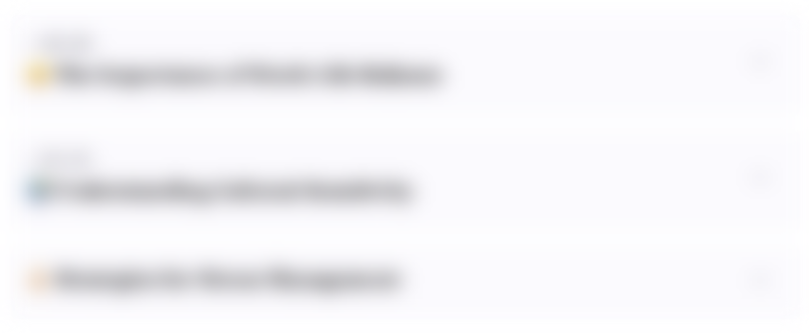
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
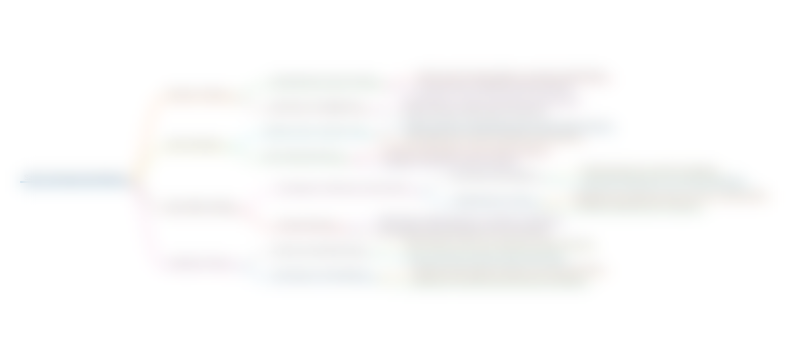
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
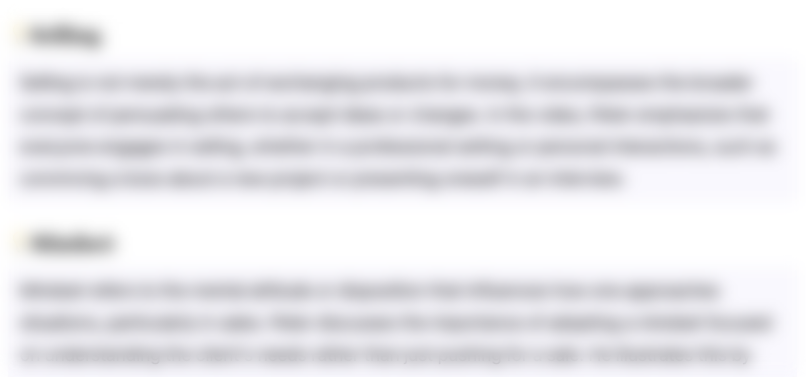
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
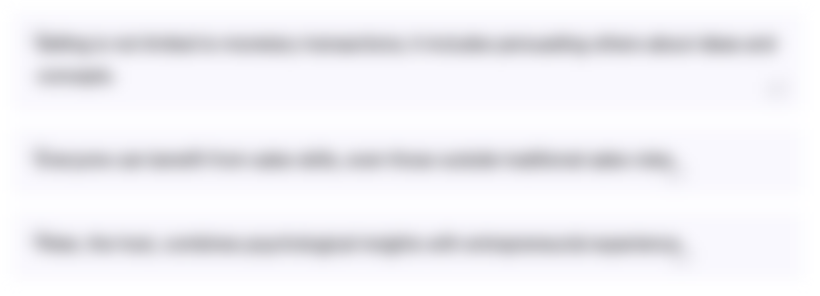
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
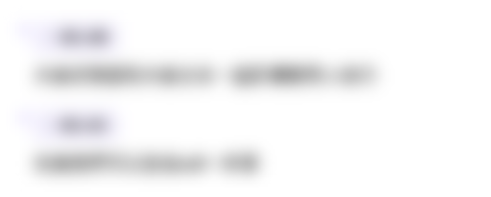
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen
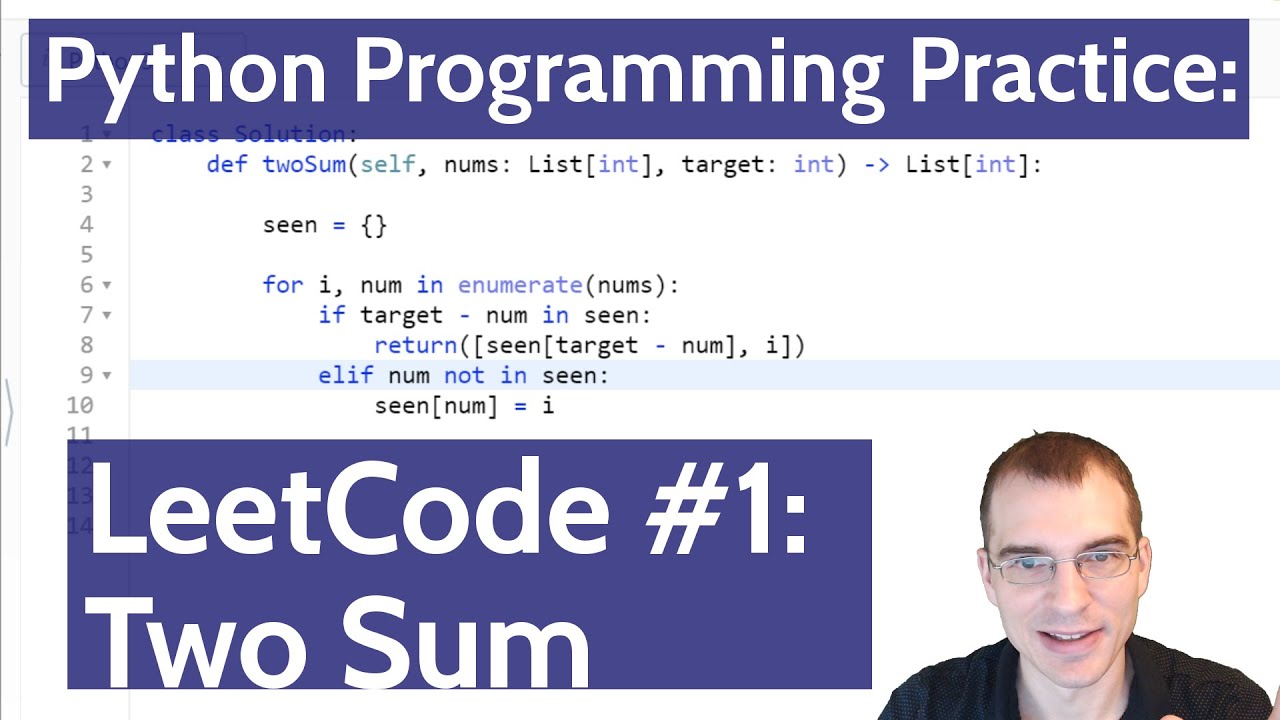
Python Programming Practice: LeetCode #1 -- Two Sum

Majority Element I | Brute-Better-Optimal | Moore's Voting Algorithm | Intuition 🔥|Brute to Optimal

1.4 Frequency Count Method

Two Sum - Leetcode 1 - HashMap - Python
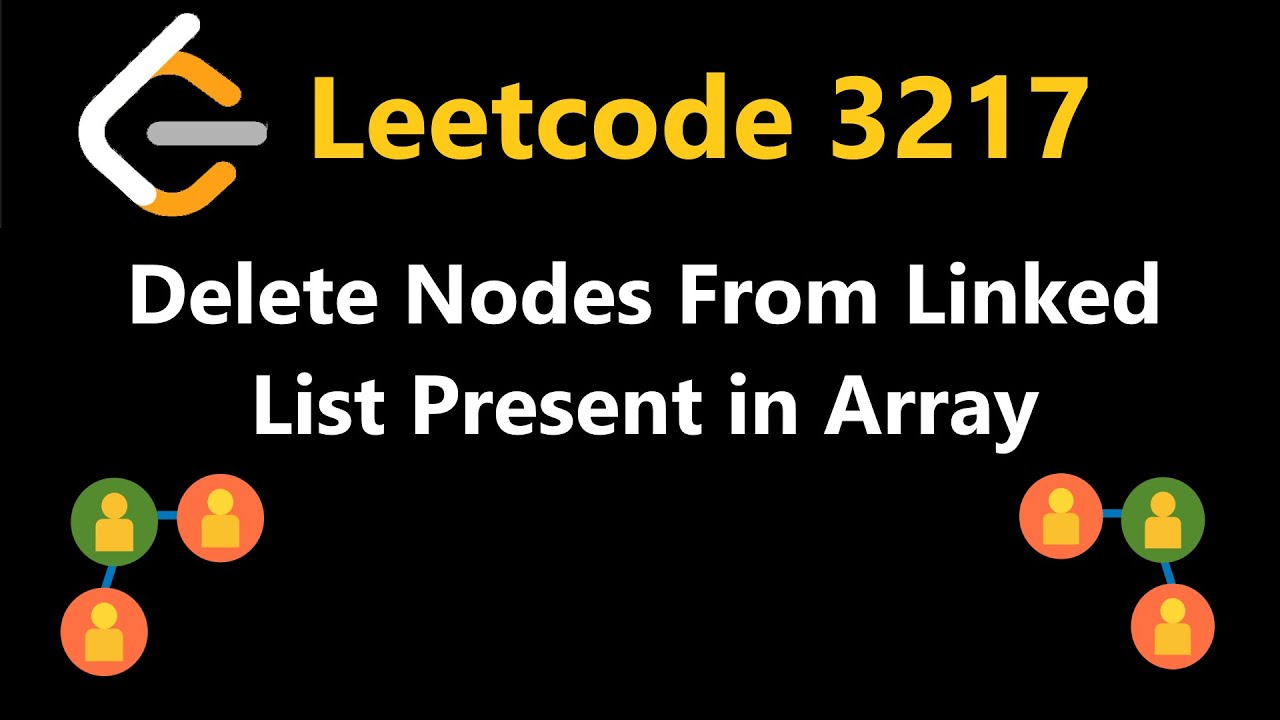
Delete Nodes From Linked List Present in Array - Leetcode 3217 - Python
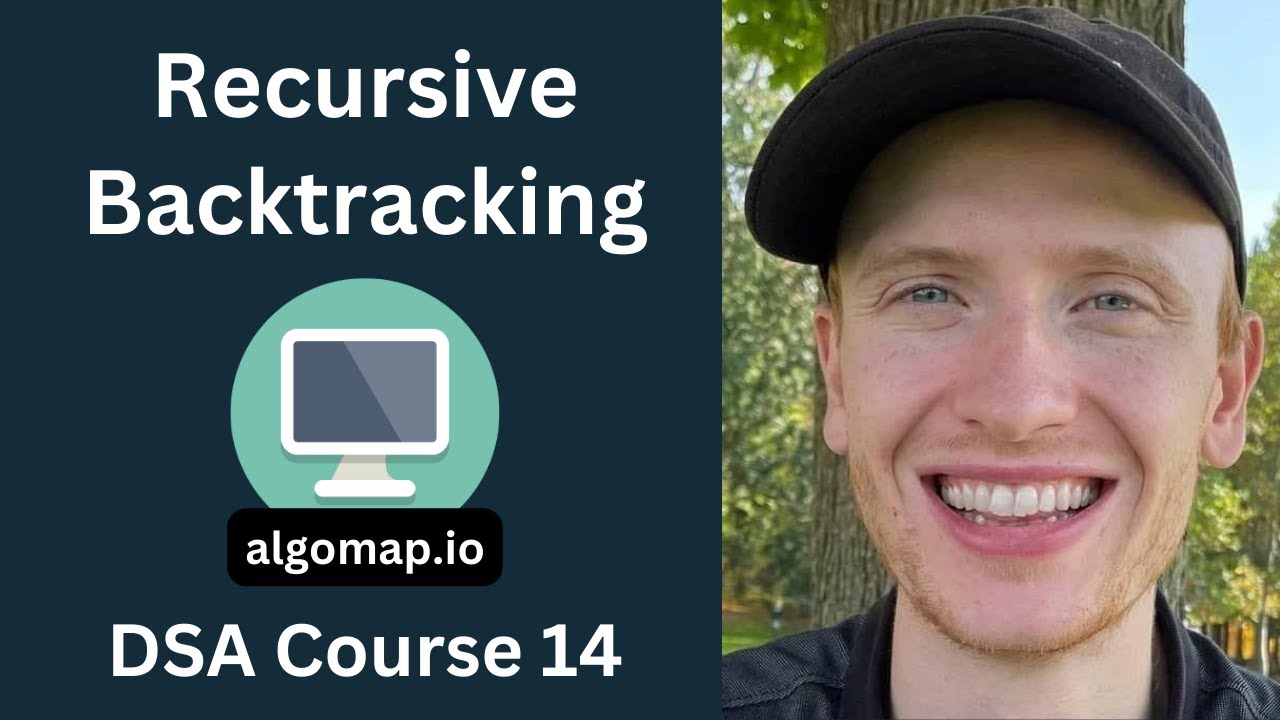
Recursive Backtracking - DSA Course in Python Lecture 14
5.0 / 5 (0 votes)