How to write tuple in python | Python Tutorials | Lesson 10
Summary
TLDRIn this engaging Python tutorial, Aman introduces tuples, highlighting their similarities to lists but emphasizing their immutability. He demonstrates how to create tuples, access elements using indexing, and perform slicing to retrieve specific ranges of values. Aman clarifies that, unlike lists, the elements of tuples cannot be modified after creation. He also shows how to combine tuples to create new ones and explores the concept of nested tuples. Additionally, the tutorial explains that tuples can contain mutable lists, allowing changes within the lists. This informative session encourages viewers to practice Python for various career opportunities in data science and AI.
Takeaways
- 😀 Python tuples are similar to lists but are immutable, meaning their elements cannot be changed after creation.
- 📁 To create a tuple in Python, use parentheses. For example, tuple_t = (5, 1, 7, 3).
- 🔢 Tuples can be accessed using indexing, e.g., tuple_t[0] returns the first element.
- 📊 You can slice tuples to retrieve a range of elements, e.g., tuple_t[0:2] returns elements from index 0 to 1.
- ❌ Attempting to change a tuple's elements results in an error, as tuples do not support item assignment.
- ➕ You can create a new tuple by adding elements to an existing tuple, e.g., new_tuple = tuple_t + (10, 20, 30).
- 📚 Tuples can contain other tuples or mutable objects like lists, allowing for complex data structures.
- 🔍 Indexing into a tuple containing other tuples allows you to access nested elements easily.
- 🔄 Lists are mutable, allowing element changes, while tuples maintain their original state once created.
- 💼 Learning Python, especially through practices like working with tuples, opens doors to careers in data analysis, machine learning, and AI.
Q & A
What is a tuple in Python?
-A tuple is a data structure that is similar to a list but is immutable, meaning its elements cannot be changed after creation.
How do you create a tuple in Python?
-You can create a tuple by placing elements inside parentheses, such as `t = (5, 1, 7, 3)`.
Can you change the elements of a tuple after it has been created?
-No, tuples are immutable. Attempting to change an element by assignment will raise a TypeError.
How do you access elements in a tuple?
-You can access elements in a tuple using their index, such as `t[0]` to access the first element.
What will happen if you try to assign a new value to an index in a tuple?
-It will result in a TypeError, indicating that tuple objects do not support item assignment.
Can you create a new tuple by combining existing tuples?
-Yes, you can concatenate tuples to create a new one, like `t2 = t1 + (10, 20, 30)`.
Is it possible to have a tuple within a tuple?
-Yes, tuples can contain other tuples. For example, `x = ((5, 1, 7), (3, 4, 5))`.
What happens if a tuple contains a mutable object like a list?
-You can modify the elements of the mutable object within the tuple. For instance, changing an element in a list contained in a tuple is allowed.
What are the key differences between lists and tuples?
-The key differences are that tuples are immutable, while lists are mutable, allowing changes to their elements.
Why is it important to understand tuples in Python?
-Understanding tuples is crucial as they are used in various programming scenarios, including data integrity and functional programming.
Outlines
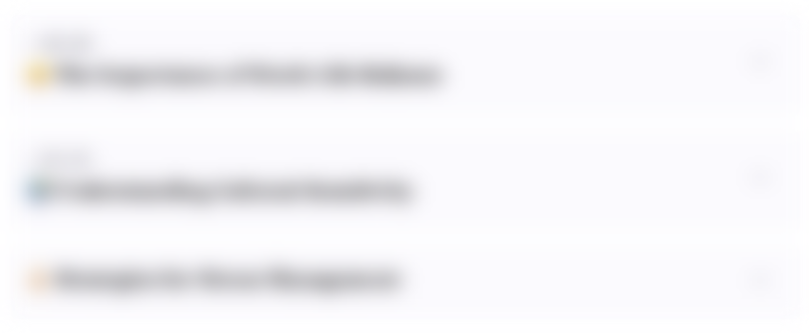
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
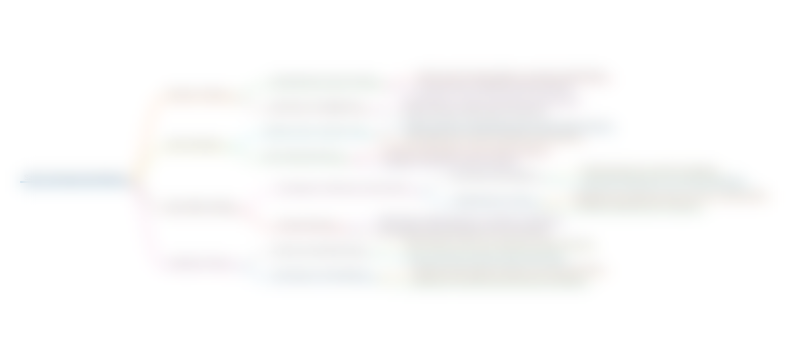
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
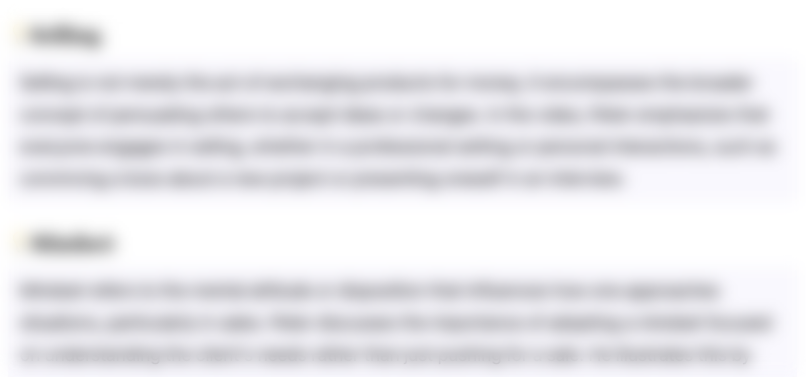
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
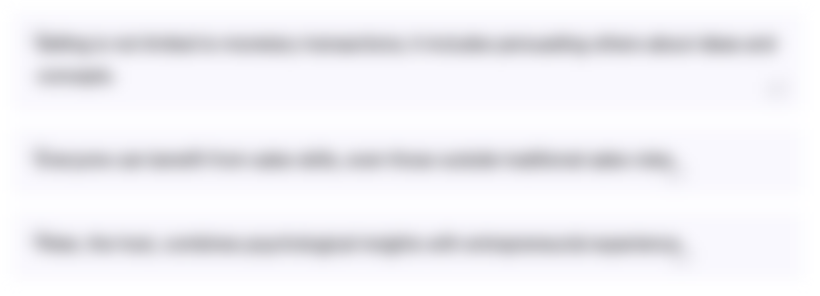
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
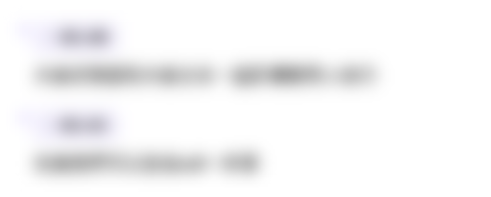
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführen5.0 / 5 (0 votes)