ReactJS Tutorial - 25 - Fragments
Summary
TLDRThis video tutorial delves into React Fragments, a feature that allows developers to group child elements without adding extra nodes to the DOM. The instructor begins by creating a 'Fragment Demo' component, illustrating how fragments solve the issue of JSX requiring a single parent element for multiple children. They demonstrate the use of fragments in a table example, showing how fragments can eliminate unwanted div tags and resolve console warnings about improper DOM nesting. The video also covers the key attribute in fragments for rendering lists and the shorthand syntax for fragments, highlighting its limitation of not allowing key attributes. The tutorial is a practical guide for React developers looking to streamline their JSX structures.
Takeaways
- đ React Fragments are used to group a list of children elements without adding extra nodes to the DOM.
- đ In React, when returning multiple elements, they must be enclosed within a single parent element.
- đ The error message 'JSX expressions must have one parent element' indicates that JSX elements need to be wrapped in an enclosing tag.
- đ React Fragments can prevent the addition of unnecessary DOM nodes by replacing enclosing tags like div.
- đ The DOM tree can be cleaned up by using React Fragments, as they do not add extra nodes like divs.
- đ React Fragments can be used in scenarios where multiple elements are returned, such as in table rows with multiple cells.
- đ Console warnings like 'validate DOM nesting TD cannot appear as a child of the div' can be resolved by using React Fragments.
- đ The key attribute can be passed to React Fragments when rendering lists of items, which is useful for identifying elements in a collection.
- đ Currently, the key attribute is the only attribute that can be passed to a React Fragment, with potential for more attributes to be supported in the future.
- đ There is a shorthand syntax for React Fragments using empty opening and closing tags, but this does not support passing the key attribute.
Q & A
What is the primary purpose of React Fragments?
-React Fragments allow you to group a list of children elements without adding extra nodes to the DOM.
How do you create a React Fragment?
-You can create a React Fragment by using either `<React.Fragment>` or the shorthand syntax `<>...</>`.
What is the issue when returning multiple JSX elements without using a Fragment?
-If you return multiple JSX elements without using a Fragment, React will throw an error stating that JSX expressions must have one parent element.
Why is it important to avoid extra nodes in the DOM?
-Avoiding extra nodes in the DOM is important for performance optimization and to prevent unnecessary nesting that can lead to styling and layout issues.
Can you pass the 'key' attribute to a React Fragment?
-Yes, you can pass the 'key' attribute to a React Fragment, which is especially useful when rendering lists of items.
What is the limitation of using the shorthand syntax for React Fragments?
-The limitation of using the shorthand syntax for React Fragments is that you cannot pass in the 'key' attribute.
How do you fix the warning 'validateDOMNesting TD cannot appear as a child of a div'?
-You can fix this warning by replacing the enclosing `div` tag with a `<React.Fragment>` to prevent the extra node from being added to the DOM.
What is the correct way to include a React Fragment in a component?
-You include a React Fragment in a component by replacing the enclosing parent tag (like `div`) with `<React.Fragment>` or `<>...</>`.
What is the significance of the 'key' attribute in the context of React Fragments?
-The 'key' attribute is significant in React Fragments when rendering lists as it helps React identify which items have changed, are added, or are removed, which is crucial for performance optimization.
Can React Fragments accept attributes other than 'key'?
-As of the knowledge cutoff in 2023, React Fragments can only accept the 'key' attribute. However, React plans to allow additional attributes in the future.
What is the benefit of using React Fragments over a simple enclosing tag like 'div'?
-Using React Fragments over a simple enclosing tag like 'div' prevents the addition of unnecessary DOM elements, which can lead to cleaner HTML structure, better performance, and fewer potential layout issues.
Outlines
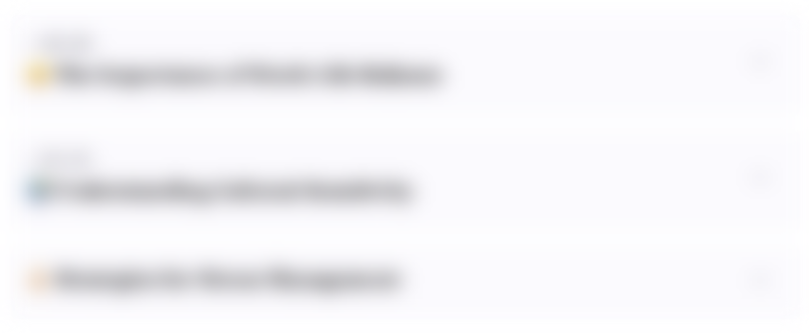
Dieser Bereich ist nur fĂŒr Premium-Benutzer verfĂŒgbar. Bitte fĂŒhren Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchfĂŒhrenMindmap
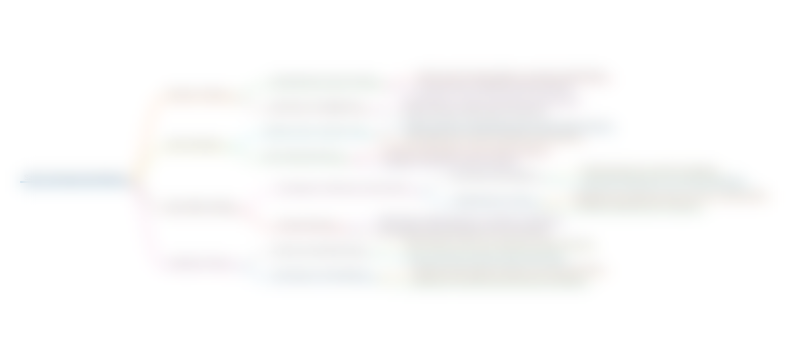
Dieser Bereich ist nur fĂŒr Premium-Benutzer verfĂŒgbar. Bitte fĂŒhren Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchfĂŒhrenKeywords
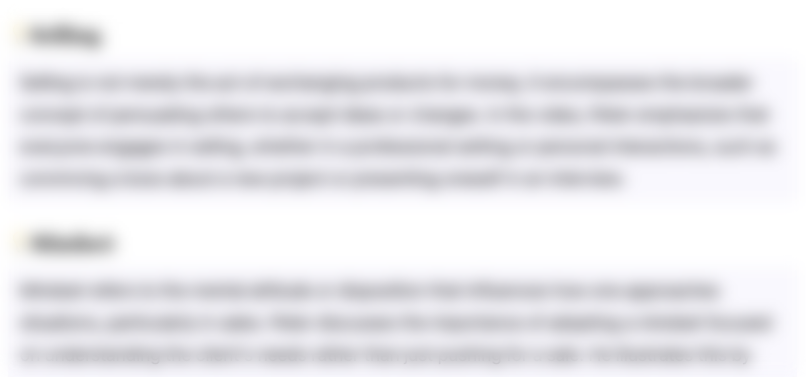
Dieser Bereich ist nur fĂŒr Premium-Benutzer verfĂŒgbar. Bitte fĂŒhren Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchfĂŒhrenHighlights
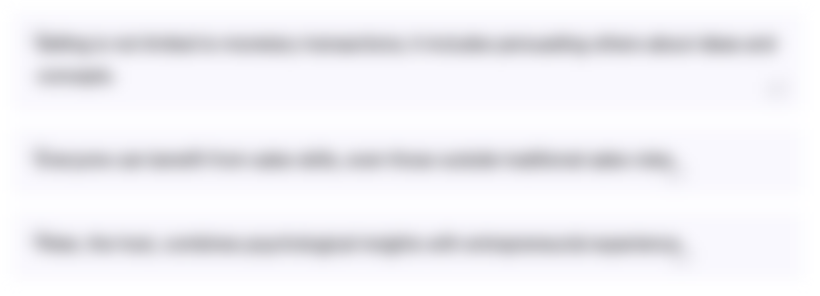
Dieser Bereich ist nur fĂŒr Premium-Benutzer verfĂŒgbar. Bitte fĂŒhren Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchfĂŒhrenTranscripts
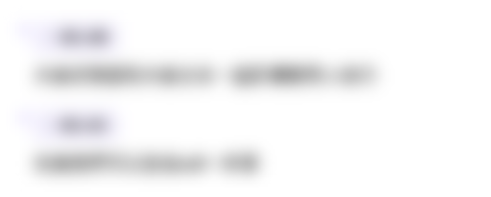
Dieser Bereich ist nur fĂŒr Premium-Benutzer verfĂŒgbar. Bitte fĂŒhren Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchfĂŒhrenWeitere Ă€hnliche Videos ansehen
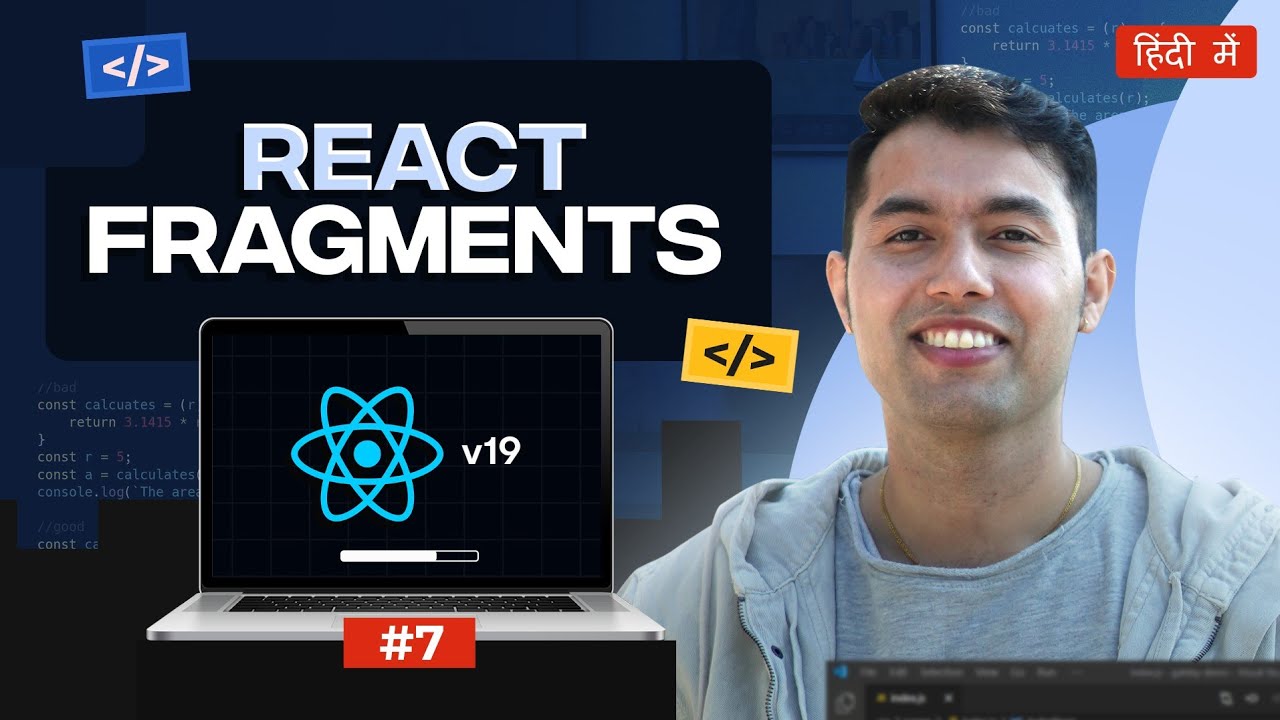
#7: React Fragments: Remove unwanted Nodes & Speed Up Rendering | React v19 Tutorial in Hindi
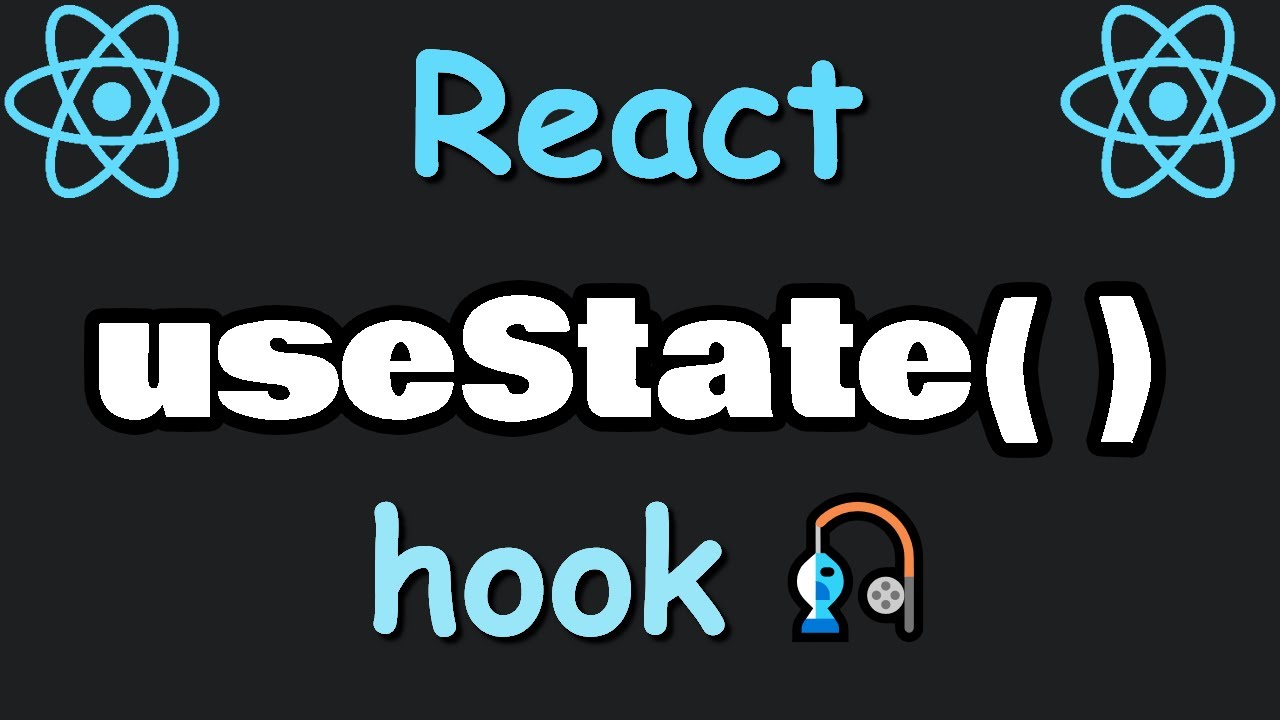
React useState() hook introduction đŁ
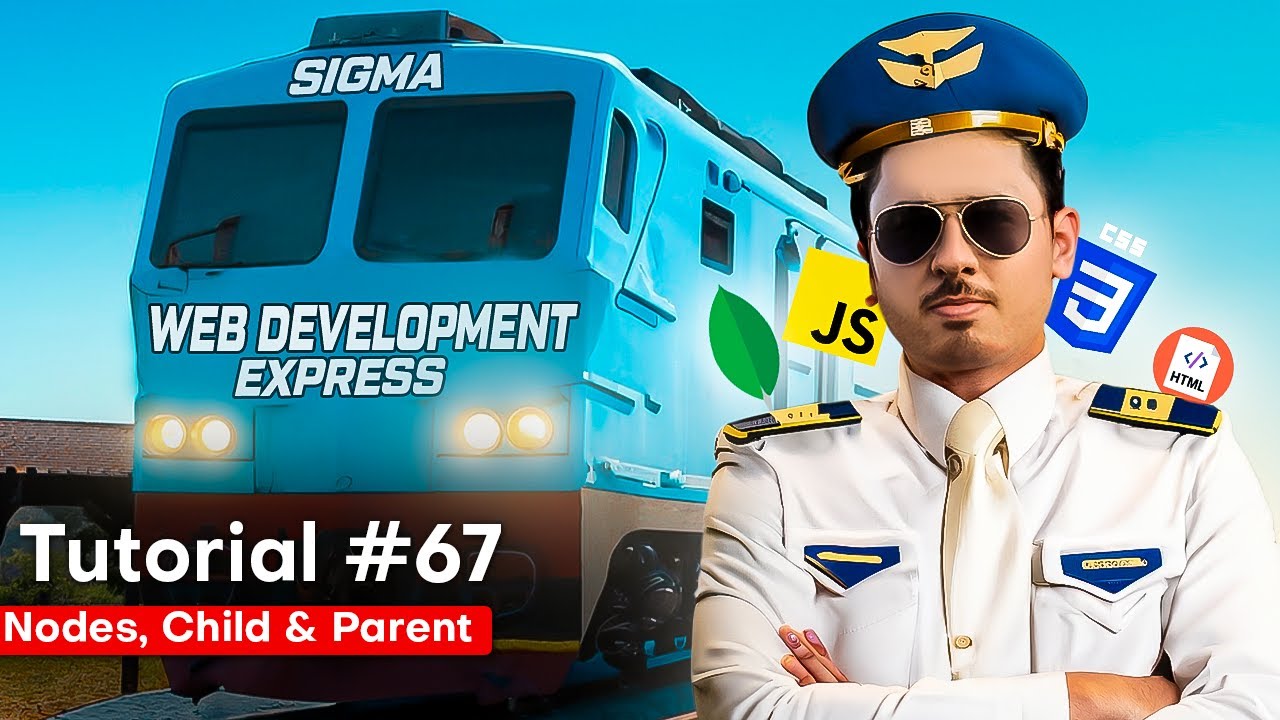
JavaScript DOM - Children, Parent & Sibling Nodes | Sigma Web Development Course - Tutorial #67

Components, Instances, and Elements | Lecture 121 | React.JS đ„

How to Create a Cloud-Based POS System Using ChatGPT AI | HTML, CSS, JS, PHP, MySQL | website
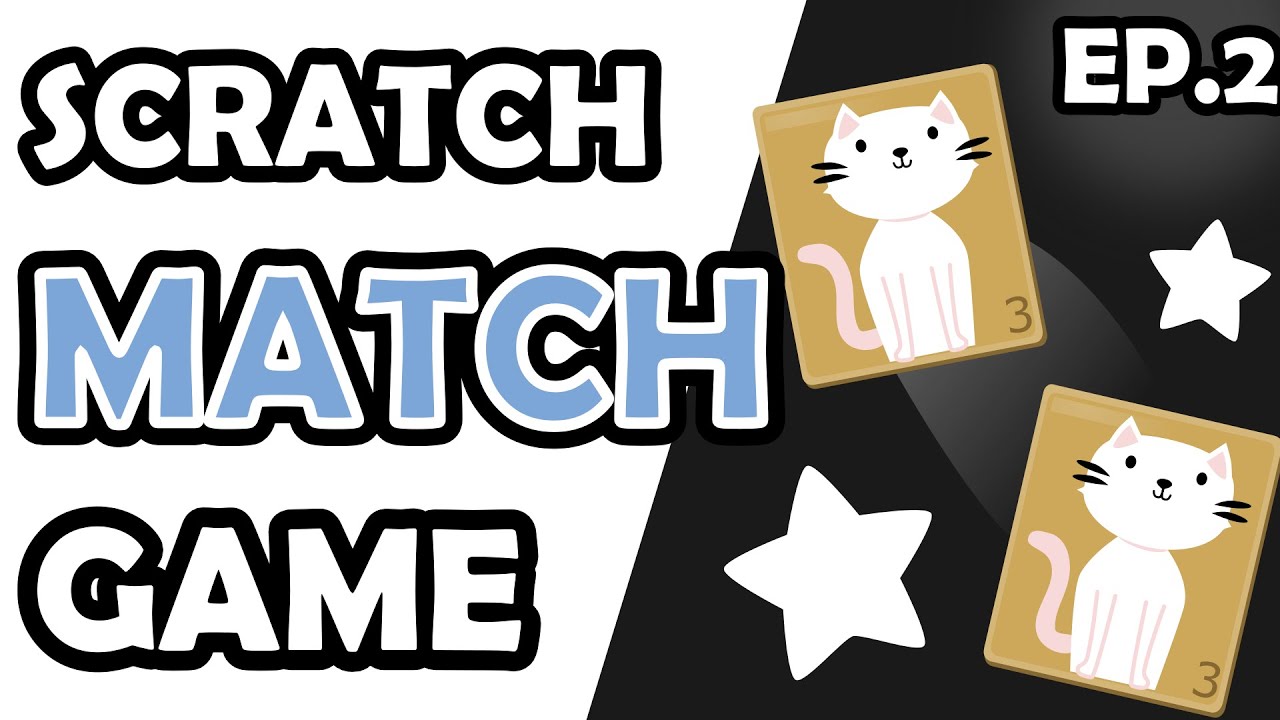
Scratch Memory Game Tutorial (Ep2)
5.0 / 5 (0 votes)