Learn JavaScript in 12 Minutes
Summary
TLDRThis video script offers a concise introduction to JavaScript, covering its role as a client-side scripting language, its basic syntax, and various functionalities. It explains how JavaScript differs from Java and demonstrates creating a simple HTML file with embedded JavaScript. The tutorial delves into variables, operators, string manipulation, arrays, functions, and control statements like 'if' conditions and 'for' loops. Aimed at beginners, it provides a foundational understanding to build upon.
Takeaways
- 🌐 JavaScript is a client-side scripting language that runs in the user's browser after the HTML file is sent by the server.
- 🔑 JavaScript is distinct from Java and has various uses including form validation, web page effects, and interactive content.
- 📄 JavaScript code is embedded within HTML files and can be saved with a '.html' extension.
- 📝 To output text in JavaScript, use `document.write` inside a script tag.
- 📏 Variables in JavaScript are declared with the `var` keyword and do not require a data type to be specified.
- 🔗 The `+` operator is used for both string concatenation and numeric addition in JavaScript.
- 🔄 Unary operators like `++` can increment a variable's value, and can be placed before or after the variable.
- 🔑 Strings in JavaScript are object types with properties like `length` and methods like `substring`.
- 🗃 Arrays can store multiple values and are defined using square brackets or the `new Array()` constructor.
- 🎯 Functions in JavaScript are defined with the `function` keyword and can accept parameters to make them reusable.
- 🔄 Flow control statements like `if` and `for` loops help control the execution of code based on conditions.
Q & A
What is JavaScript and where is it typically used?
-JavaScript is a client-side scripting language used primarily for web development. It is executed by the user's browser when they visit a website, allowing for interactive content and dynamic effects on web pages.
How does JavaScript differ from Java?
-JavaScript and Java are not the same language despite the similar names. JavaScript is a scripting language for web development, while Java is a general-purpose programming language used for a wide range of applications.
What is the purpose of the 'script' tag in HTML?
-The 'script' tag in HTML is used to embed JavaScript code into an HTML document. It allows the browser to execute the JavaScript code when processing the page.
Why is the 'document.write' function used in JavaScript?
-The 'document.write' function is used to output text to the browser, which is a simple way to test if JavaScript is working correctly within an HTML document.
What is a variable in JavaScript and how is it declared?
-A variable in JavaScript is an item of data that has a name and a value. It is declared using the 'var' keyword followed by the variable name. JavaScript is loosely typed, so the type does not need to be specified.
How can you define a variable with a value in JavaScript?
-You can define a variable with a value in JavaScript by using the assignment operator (=), like this: `myVariable = 5;` or by combining the declaration and assignment: `var myVariable = 5;`.
What is the significance of the semicolon in JavaScript statements?
-The semicolon in JavaScript is used to terminate a statement. While it can be omitted in many cases due to Automatic Semicolon Insertion (ASI), it is considered good practice to include it for clarity and to avoid potential errors.
How do you comment in JavaScript?
-In JavaScript, you can create single-line comments using two forward slashes (//). Comments are not executed and are used to describe what is happening in the code.
What is concatenation in JavaScript and how is it done?
-Concatenation in JavaScript is the process of combining two or more strings. It is done using the plus sign (+) operator. For example, if you have two variables `words` and `moreWords`, you can concatenate them with `sentence = words + moreWords;`.
How can you access the length property of a string in JavaScript?
-You can access the length property of a string in JavaScript by using the dot notation, like this: `var length = alpha.length;` where `alpha` is the string variable.
What is the difference between a property and a method in JavaScript?
-A property in JavaScript is a piece of information or a value that describes an object, while a method is a function that can take input, perform operations, and return a result. Properties are accessed using dot notation, while methods are called using parentheses.
How do you create an array in JavaScript?
-You can create an array in JavaScript using the 'new Array()' constructor, or more simply with square brackets to define the elements directly, like this: `var myArray = ['element1', 'element2', ...];`.
What is the purpose of a function in JavaScript?
-A function in JavaScript is a block of code designed to perform a specific task. It allows for code reuse and can take parameters, making it a versatile tool for organizing and managing code.
How does an 'if' statement work in JavaScript?
-An 'if' statement in JavaScript executes a block of code if a specified condition is true. It is used for flow control and can include 'else' clauses to handle cases where the condition is false.
What is a 'for' loop used for in JavaScript?
-A 'for' loop in JavaScript is used to execute a block of code multiple times. It is typically used when the number of iterations is known beforehand and is useful for iterating over arrays or performing repeated tasks.
Outlines
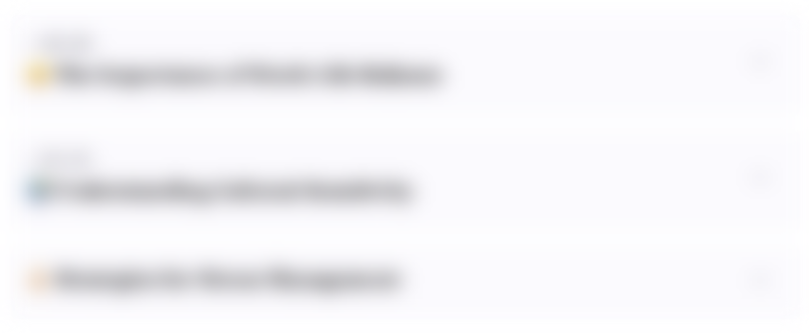
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
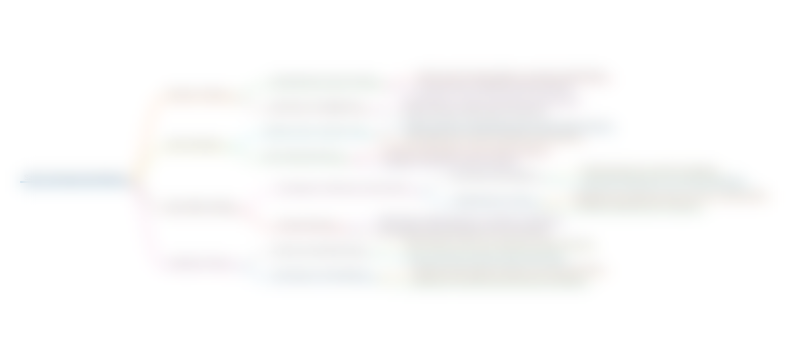
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
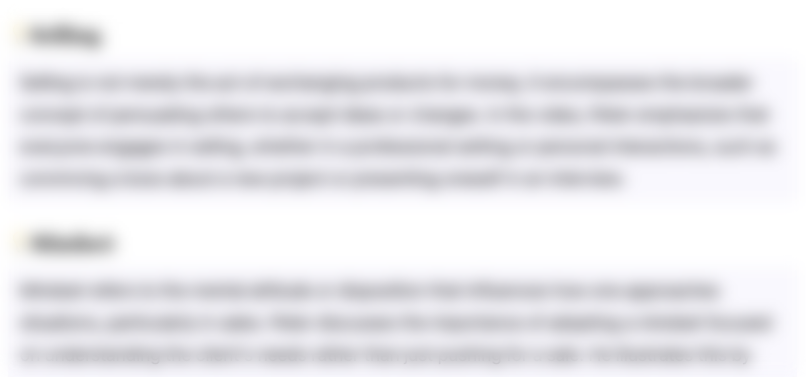
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
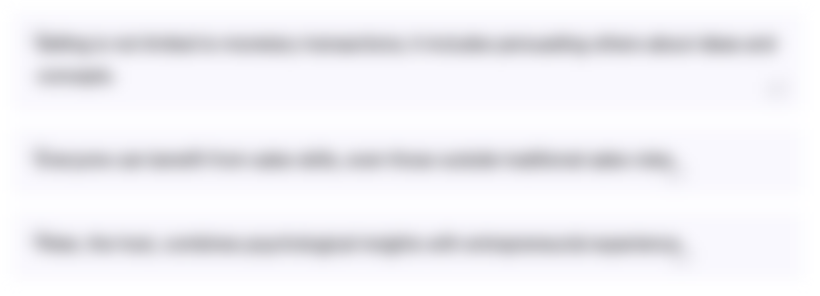
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
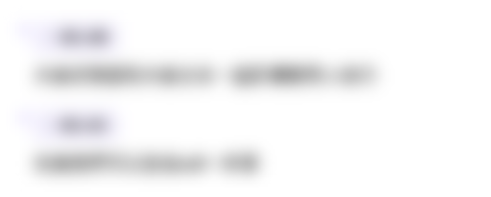
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen
5.0 / 5 (0 votes)