Data Types in Python | Python for Beginners
Summary
TLDRThis educational video script delves into Python's data types, essential for understanding how data is stored and manipulated. It covers numeric types like integers, floats, and complex numbers, explaining their characteristics and operations. The script progresses to Boolean values, emphasizing true or false states, and sequence types including strings, lists, and tuples, highlighting their indexing and mutability. It distinguishes between lists and tuples, noting the latter's immutability. Sets are introduced as unordered collections without duplicates, and their operations for unique value comparison are discussed. The script concludes with dictionaries, Python's key-value pair data structures, illustrating how they store and update data. The comprehensive overview is designed to solidify viewers' grasp of Python's foundational data types.
Takeaways
- 🔢 Data types in Python are essential for understanding how data is stored and manipulated, with numeric, sequence, set, Boolean, and dictionary types being the main categories.
- 📏 Numeric types include integers (whole numbers), floats (decimal numbers), and complex numbers (used for imaginary numbers).
- 🌟 Boolean type represents one of two values: True or False, and is often used in conditional statements and comparison operations.
- 📝 Sequence types consist of strings, lists, and tuples, with strings being arrays of bytes representing Unicode characters, and lists being ordered, mutable collections of items.
- 🍦 Tuples are similar to lists but are immutable, meaning they cannot be changed after creation, and are often used for data that should not change.
- 🔑 Sets are unordered collections of unique elements, without indices, and are useful for membership testing and eliminating duplicates.
- 📖 Dictionaries store data in key-value pairs, allowing for fast retrieval of values based on keys, and are mutable, allowing for updates and deletions.
- 🔍 Indexing in Python is used to access elements in data structures like strings and lists, starting from position 0, and can include negative indexing for reverse access.
- 🔄 Operations like addition, multiplication, and concatenation can be performed on strings and lists, but not on tuples due to their immutability.
- 🏷 Sets support operations like union, intersection, and difference, which are useful for comparing and manipulating collections of unique items.
- 🛠️ Dictionaries can be dynamically updated and elements can be added, modified, or removed using keys, providing a flexible way to handle data.
Q & A
What are the main data types in Python discussed in the script?
-The main data types in Python discussed are numeric, sequence, set, Boolean, and dictionary.
What are the three different types of numeric data types in Python?
-The three different types of numeric data types in Python are integers, floats, and complex numbers.
How can you verify the data type of a variable in Python?
-You can verify the data type of a variable in Python by using the 'type()' function, such as 'type(12)' to check if it's an integer.
What is the difference between an integer and a float in Python?
-An integer in Python is a whole number, positive or negative, without a decimal point. A float is a number with a decimal point, representing a fractional part.
What is a complex number in Python and how is it represented?
-A complex number in Python represents imaginary numbers and is represented by using 'j' as the imaginary unit, such as '12 + 3j'.
What are the two built-in values of the Boolean data type in Python?
-The two built-in values of the Boolean data type in Python are 'True' and 'False'.
How do you create a string in Python and what are the different ways to enclose it?
-You create a string in Python by enclosing characters in either single quotes (' '), double quotes (
What is the significance of indexing in Python strings and how does it work?
-Indexing in Python strings allows you to access specific characters or a range of characters within the string. It starts at 0, and you can use positive or negative indices to access characters from the beginning or end of the string.
How do lists differ from strings in Python, and what is a key feature of lists?
-Lists in Python differ from strings in that they can store multiple values, which can be of different data types. A key feature of lists is that they are mutable, meaning you can change, add, or remove items after creation.
What is a tuple in Python and how does it differ from a list?
-A tuple in Python is a collection of values, similar to a list, but it is immutable, meaning its values cannot be changed after creation. Tuples are defined using parentheses.
What is a set in Python and how does it differ from a list?
-A set in Python is an unordered collection of unique elements, which means it does not allow duplicate values. Unlike lists, sets do not support indexing and are defined using curly braces.
How can you compare two sets in Python to find common or unique elements?
-You can compare two sets in Python using set operations like union ('|'), intersection ('&'), difference ('-'), and symmetric difference ('^') to find common or unique elements between them.
What is a dictionary in Python and how is it different from other data types?
-A dictionary in Python is a collection of key-value pairs, where each key is unique. It is different from other data types in that it uses keys to access values, rather than using indices like in lists or tuples.
How do you access values in a dictionary in Python?
-In Python, you access values in a dictionary by using the keys, such as 'dictionary_name['key_name']'.
Can you update or delete values in a Python dictionary? If so, how?
-Yes, you can update values in a Python dictionary by assigning a new value to an existing key or by using the 'update()' method. You can delete values using the 'del' statement followed by the key or the 'pop()' method.
Outlines
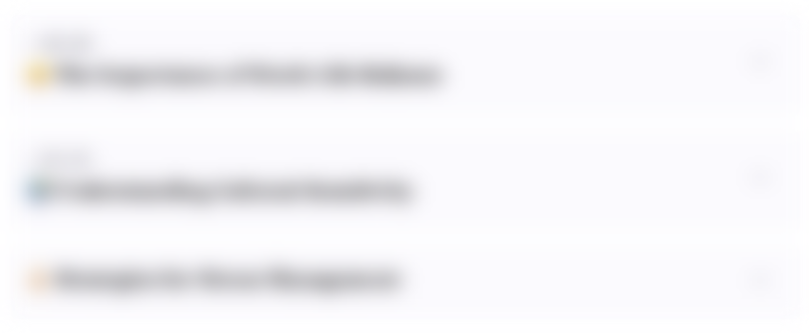
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
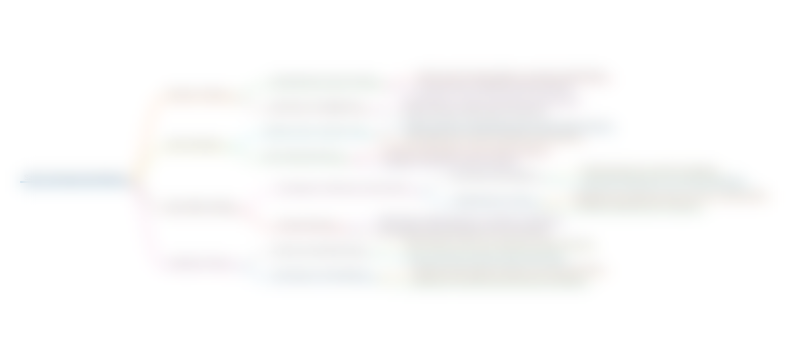
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
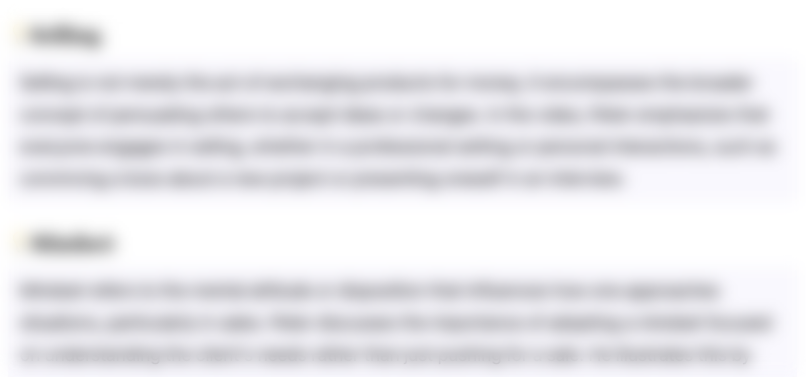
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
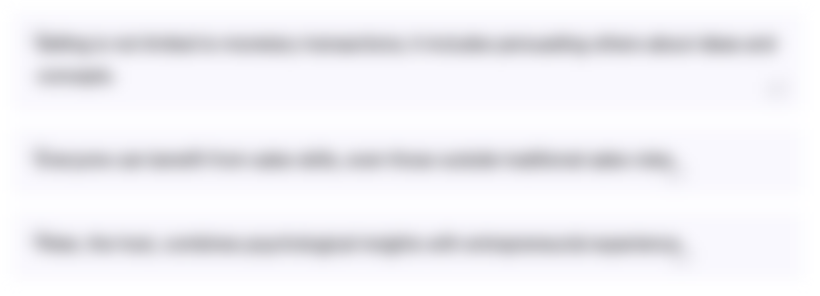
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
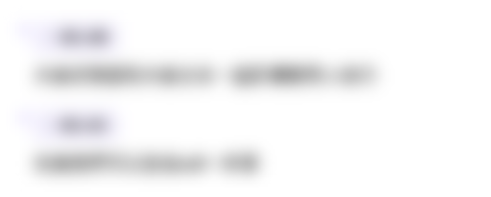
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen
5.0 / 5 (0 votes)