There's Way More to JSON.Stringify Than You Think
Summary
TLDRIn this video, Dom explores the intricacies of JavaScript's JSON.stringify method, revealing its hidden capabilities. He demonstrates how to prettify JSON output with indentation and new lines using the third argument, and delves into the second argument's role in filtering object properties for JSON conversion. Dom also explains the use of replacer functions to filter or transform data, showcasing practical examples. The video is an insightful guide for developers looking to harness the full potential of JSON.stringify.
Takeaways
- 🔍 The `JSON.stringify` method in JavaScript converts objects or arrays into JSON strings.
- 📄 To make the JSON output more readable, you can use the third argument of `JSON.stringify` to add indentation with a specified number of spaces or a string like tabs.
- 🚫 The second argument of `JSON.stringify` can be an array specifying a whitelist of properties to include in the JSON output, useful for filtering sensitive data.
- 👨💻 Passing a function as the second argument allows for custom filtering and transformation of the JSON output, acting as a replacer function that runs for each property.
- 🔑 The replacer function is first called for the entire object, and then for each property, making it possible to manipulate the entire structure or individual values.
- 🛠️ Using the replacer function, you can filter out properties by returning `undefined` for certain conditions or transform values, such as multiplying numbers by a factor.
- 📊 The replacer function provides a powerful way to control the serialization process, allowing for both inclusion/exclusion of data and value transformations.
- 💡 While the replacer function is versatile, it's often simpler to create a new object with the desired properties before stringifying, especially for complex transformations.
- 👍 The video provides practical examples of how to use `JSON.stringify` with different arguments, demonstrating the method's flexibility in generating JSON strings.
Q & A
What is the primary function of JSON.stringify in JavaScript?
-JSON.stringify in JavaScript is used to convert an object or an array into a JSON string.
How can you prettify the JSON string output using JSON.stringify?
-You can prettify the JSON string by using the third argument of JSON.stringify, which allows you to specify the number of spaces for indentation or a string like a tab character.
What is the maximum indentation level allowed when using the third argument in JSON.stringify?
-The maximum indentation level allowed is 10 spaces, regardless of the number specified.
What does the second argument of JSON.stringify do when it's an array?
-When the second argument is an array, it acts as a whitelist to specify which properties of an object to include in the JSON string output.
What happens if you provide an empty array as the second argument in JSON.stringify?
-Providing an empty array as the second argument results in no properties being included in the JSON string output.
Can you use a function as the second argument in JSON.stringify, and if so, what does it do?
-Yes, a function can be used as the second argument, and it acts as a filter to determine which values are included in the JSON string by returning them, or it can transform the values by returning modified versions.
What is the behavior of the replacer function when it encounters the initial call with an empty key?
-The replacer function is initially called with an empty key for the entire object, and if it returns the object itself, it proceeds to process each property.
How can you use the replacer function to filter out properties from the JSON string?
-You can use the replacer function to filter out properties by returning 'undefined' for the properties you want to exclude from the JSON string.
Is it possible to modify the values during the JSON.stringify process using the replacer function?
-Yes, you can modify the values by returning a transformed version of the value within the replacer function.
Why might someone choose to create a new object before using JSON.stringify instead of using the replacer function?
-Creating a new object before using JSON.stringify might be preferred for clarity and simplicity, especially when the transformations or filtering are complex or numerous.
Outlines
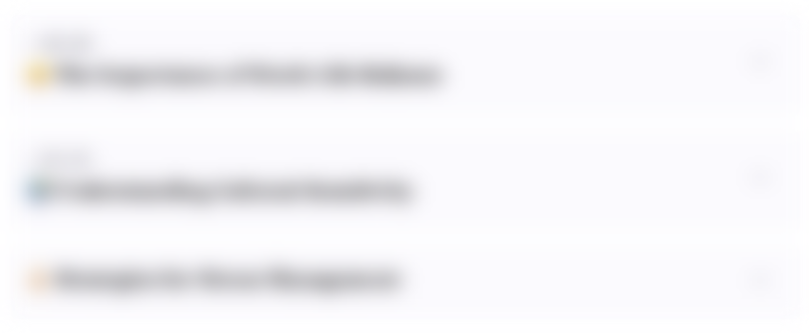
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
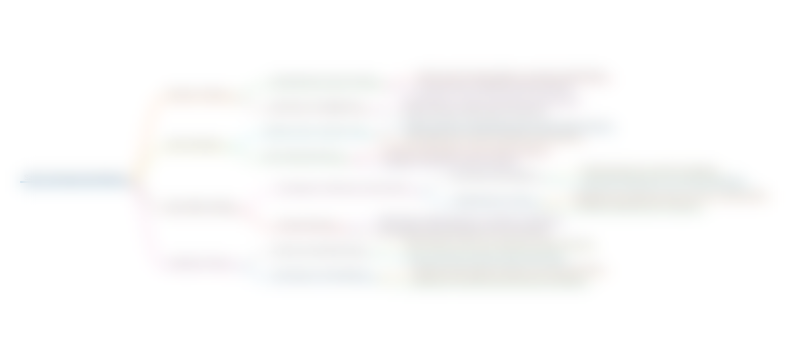
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
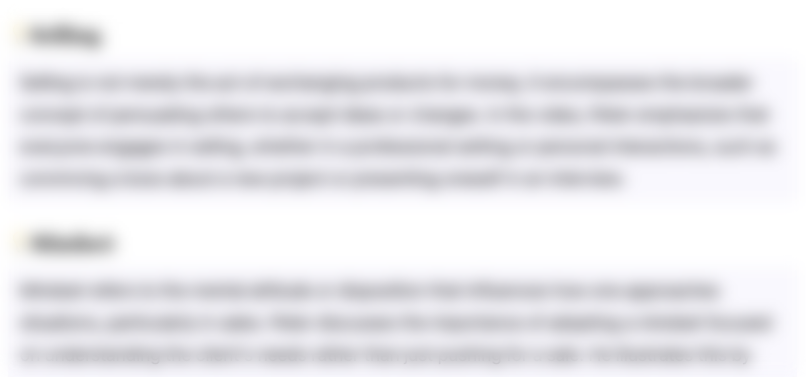
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
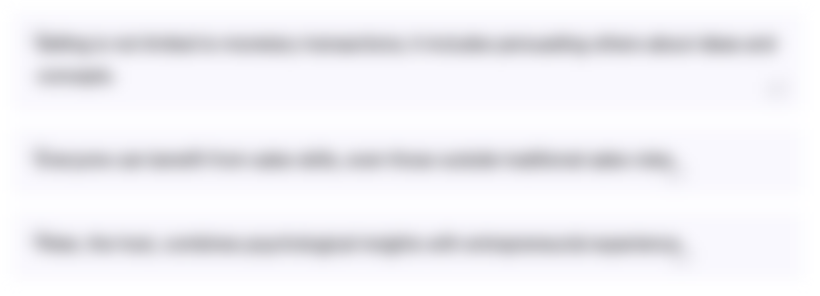
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
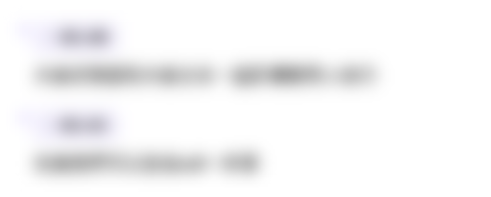
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen

Why does JavaScript's fetch make me wait TWICE?
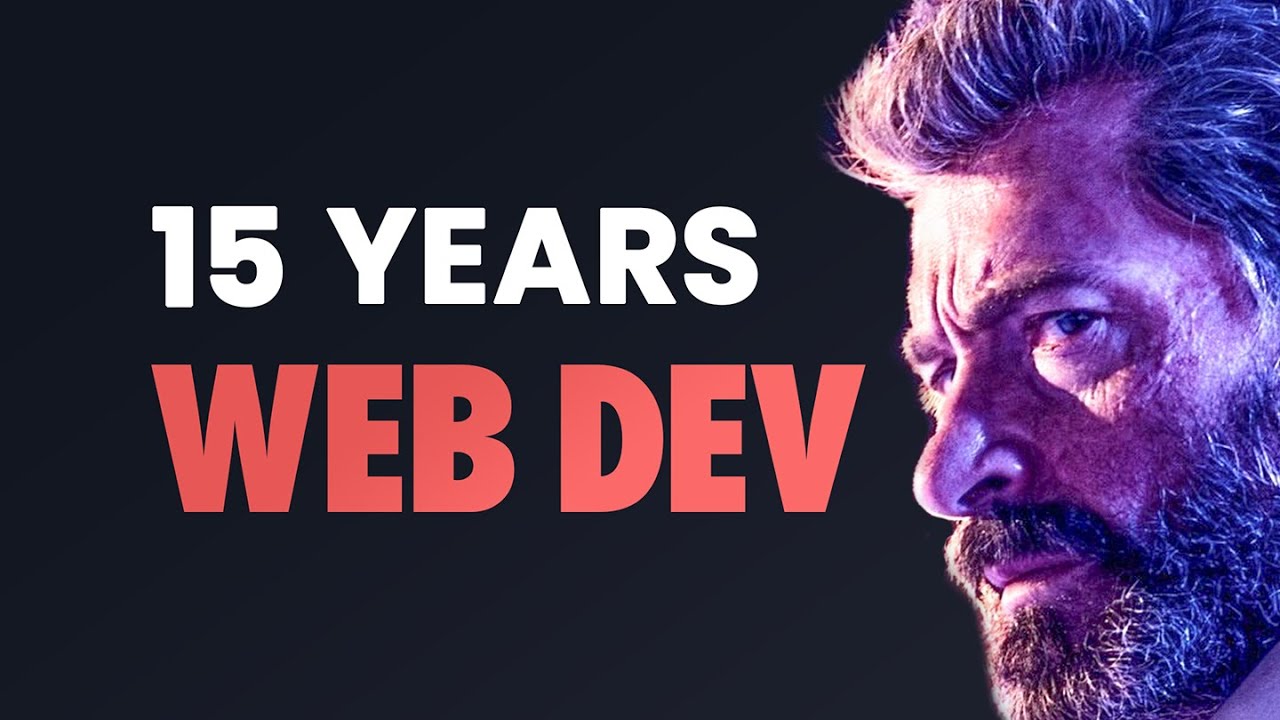
Web Dev The Right Way

What the heck is the event loop anyway? | Philip Roberts | JSConf EU

JavaScript Fetch API - One Mistake I ALWAYS MAKE!
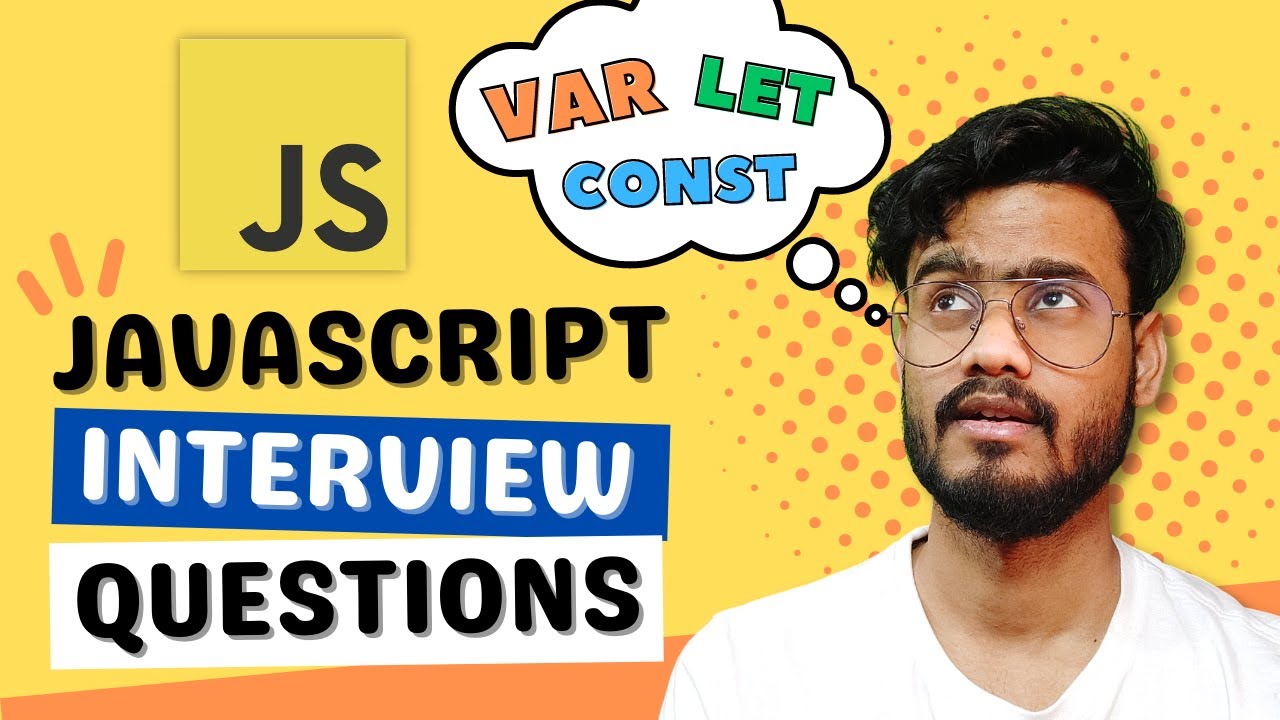
Javascript Interview Questions ( Var, Let and Const ) - Hoisting, Scoping, Shadowing and more

PERÍODO REGENCIAL: ENTENDA DE UMA VEZ POR TODAS (Débora Aladim)
5.0 / 5 (0 votes)