61. OCR GCSE (J277) 2.1 Insertion sort
Summary
TLDRThis video tutorial explores the insertion sort algorithm, ideal for small datasets and inserting into sorted lists. It demonstrates the step-by-step process of sorting breakfast cereals alphabetically, emphasizing the algorithm's simplicity despite its complexity in explanation. The video also touches on educational resources, like the 'Essential Algorithms for A Level Computer Science' book, which covers GCSE algorithms and is beneficial for students at any level. The tutorial is designed to help viewers understand and apply insertion sort without needing to memorize code.
Takeaways
- 🔍 The video discusses the insertion sort algorithm, which is a simple and intuitive sorting method.
- 📈 Insertion sort is particularly useful for small datasets and for inserting items into already sorted lists.
- 🚫 It is generally not used for large datasets due to less efficiency compared to other sorting algorithms.
- 🌿 The algorithm sorts items one by one into their correct position in an ordered list, demonstrated using breakfast cereals as an example.
- 🔄 The process involves comparing each item with those already sorted and shifting them to make space for the current item.
- 🟢 The video uses visual aids to distinguish between the sorted (green) and unsorted (orange) parts of the list during the sorting process.
- 📝 The script emphasizes understanding the main steps of algorithms, their prerequisites, and applying them to datasets as key learning objectives.
- 📖 The video references a book titled 'Essential Algorithms for A Level Computer Science' which covers algorithms required for GCSE and A Level studies.
- 💡 The book is designed to help students understand algorithms through high-level introductions, structured English descriptions, diagrams, examples, pseudocode, and actual code in Python and Visual Basic.
- 🎓 The video reassures viewers that memorizing code line by line is not necessary, focusing instead on understanding the algorithm's logic and application.
Q & A
What is the main purpose of the insertion sort algorithm?
-The main purpose of the insertion sort algorithm is to sort a dataset by inserting each item into its correct position one at a time.
Why is insertion sort particularly useful for small datasets?
-Insertion sort is particularly useful for small datasets because it is simple and efficient for a limited number of items, making it a good choice for scenarios where the data size is manageable.
How does insertion sort handle the insertion of items into already sorted lists?
-Insertion sort is adept at inserting items into already sorted lists by comparing each new item with the sorted items and placing it in the correct position to maintain the sorted order.
What is the general approach when performing insertion sort on a list of items?
-The general approach in insertion sort involves comparing each item with the items already sorted and shifting them to create space for the new item to be inserted in its correct position.
Can you explain the process of sorting 'Fruit 'n' Fibre' using the insertion sort algorithm as described in the script?
-In the script, 'Fruit 'n' Fibre' is compared with 'Weetabix' and found to be less, so 'Weetabix' is moved up. 'Fruit 'n' Fibre' is then compared with 'Sugar Puffs' and moved up again, and finally, it is compared with 'Crunchy Nut Clusters', where it finds its correct position and is inserted.
What is the role of the FOR loop in the pseudocode of the insertion sort algorithm?
-The FOR loop in the pseudocode of the insertion sort algorithm is used to iterate over each item in the list, starting from a certain index and moving towards the end of the list.
How does the WHILE loop contribute to the sorting process in the insertion sort algorithm?
-The WHILE loop in the insertion sort algorithm runs as long as the current position is greater than zero and the item below the current position is greater than the current item, allowing for the shifting of items to make space for the correct insertion of the current item.
What is the significance of the green and orange items in the visual representation of the insertion sort algorithm?
-In the visual representation, green items represent the sorted part of the list, while orange items represent the ones currently being sorted and compared. This color coding helps to visualize the progress of the sorting process.
Why might insertion sort be replaced by more efficient algorithms for large datasets?
-Insertion sort might be replaced by more efficient algorithms for large datasets because its performance degrades with larger lists due to its O(n^2) time complexity, making it less suitable for handling large volumes of data efficiently.
What educational resource is recommended in the script for further understanding of algorithms?
-The script recommends a book called 'Essential Algorithms for A Level Computer Science' for further understanding of algorithms, which is available on Amazon and covers every algorithm required for GCSE.
How does the book 'Essential Algorithms for A Level Computer Science' aid in learning algorithms?
-The book 'Essential Algorithms for A Level Computer Science' aids in learning algorithms by introducing them from a high-level perspective, providing structured English descriptions, diagrams, examples, pseudocode, and actual code in Python and Visual Basic.
Outlines
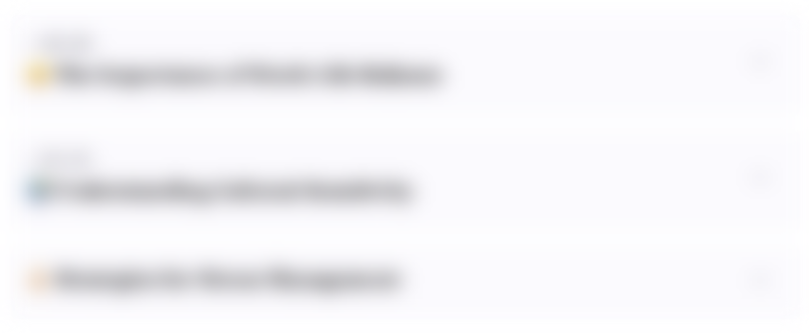
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
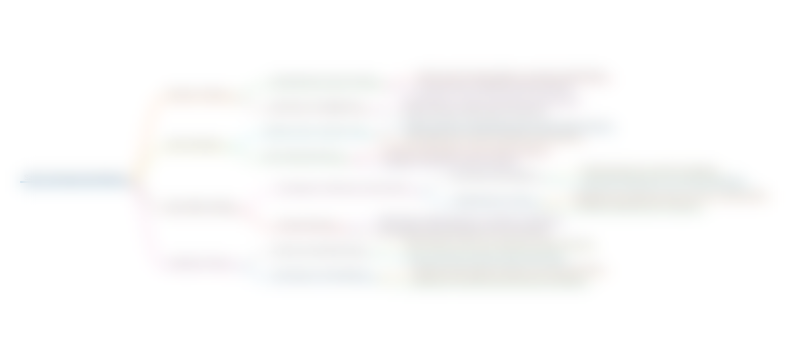
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
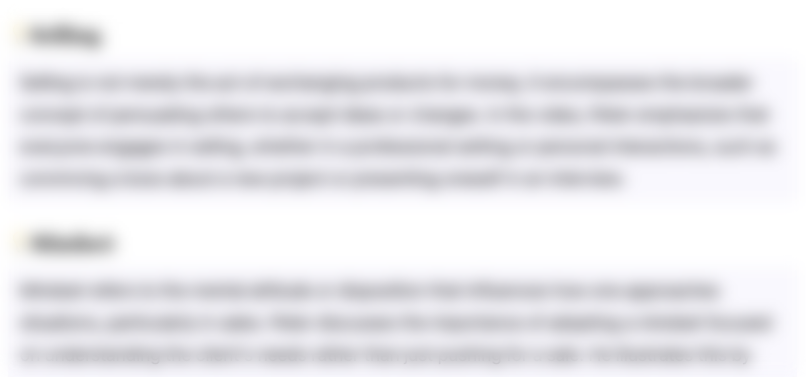
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
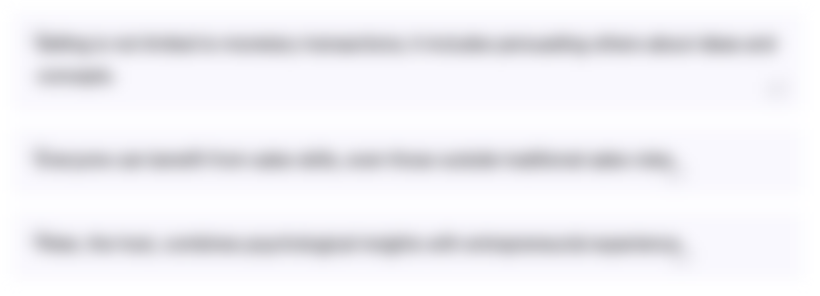
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
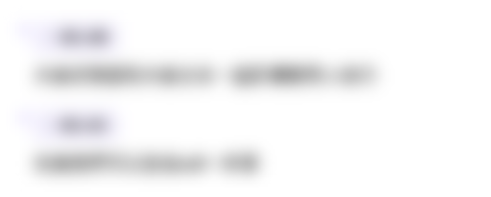
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen
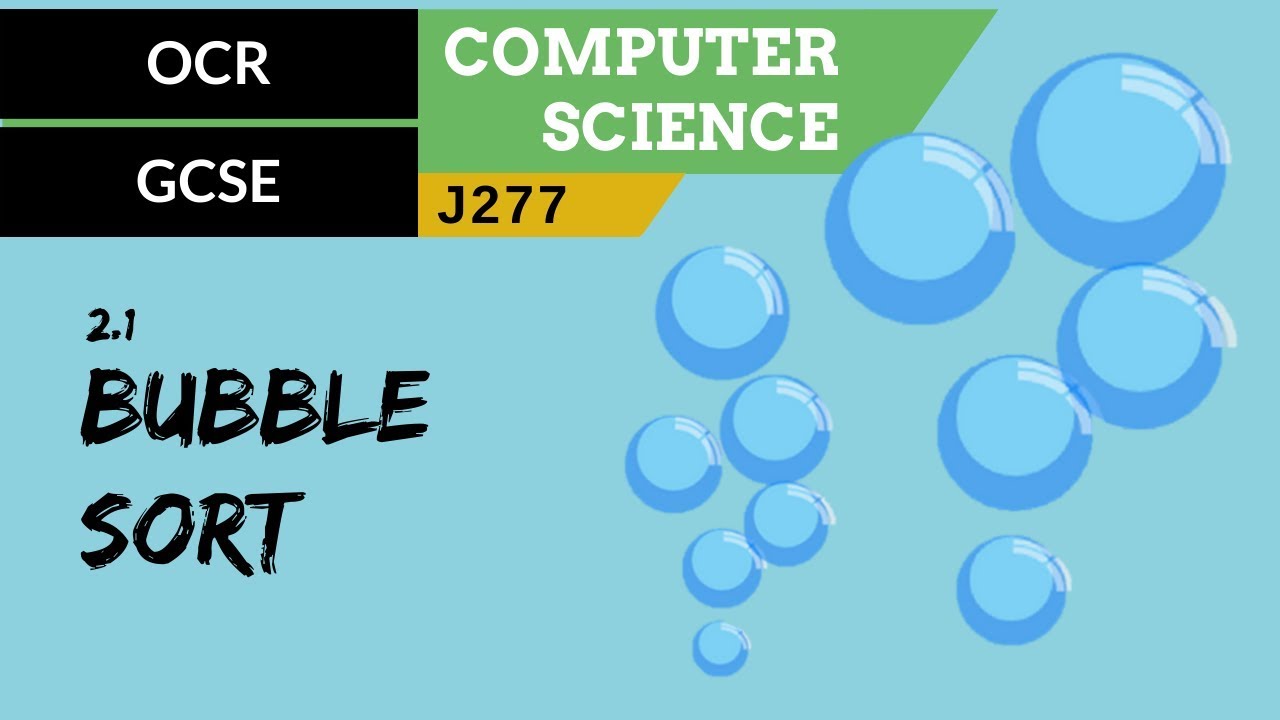
59. OCR GCSE (J277) 2.1 Bubble sort

58. OCR GCSE (J277) 2.1 Linear search
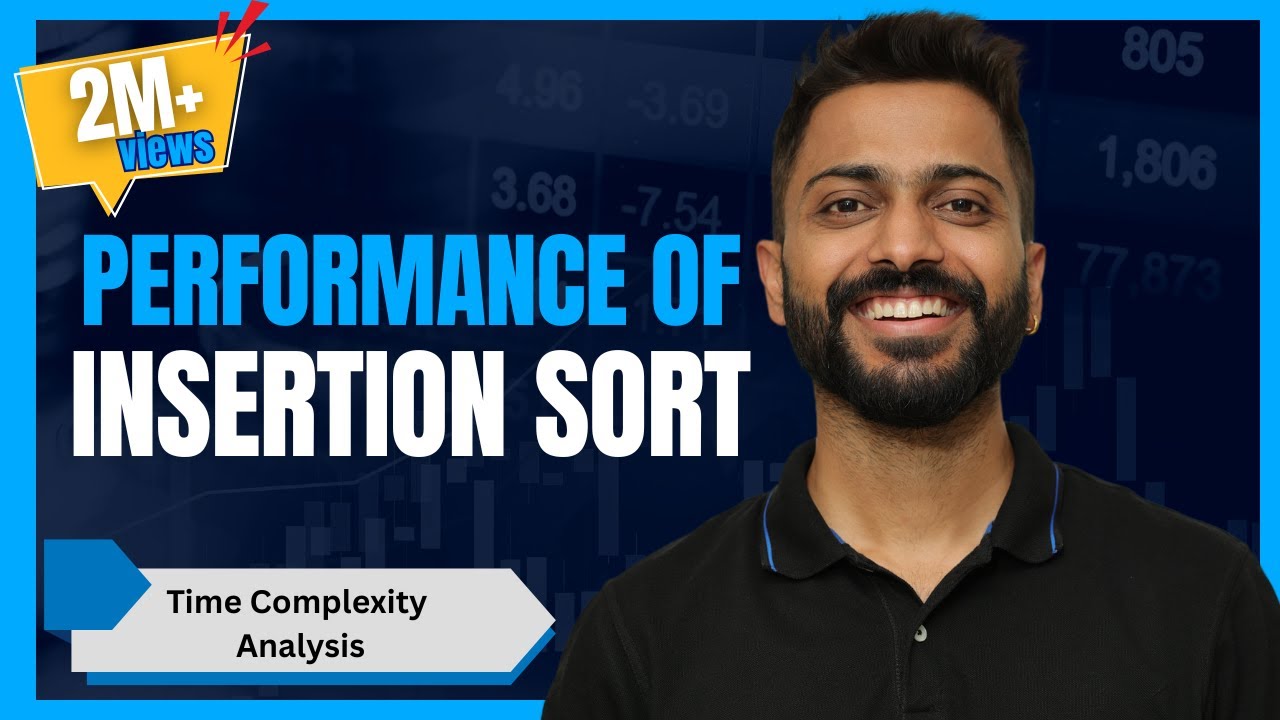
L-3.5: Insertion Sort | Time Complexity Analysis | Stable Sort | Inplace Sorting
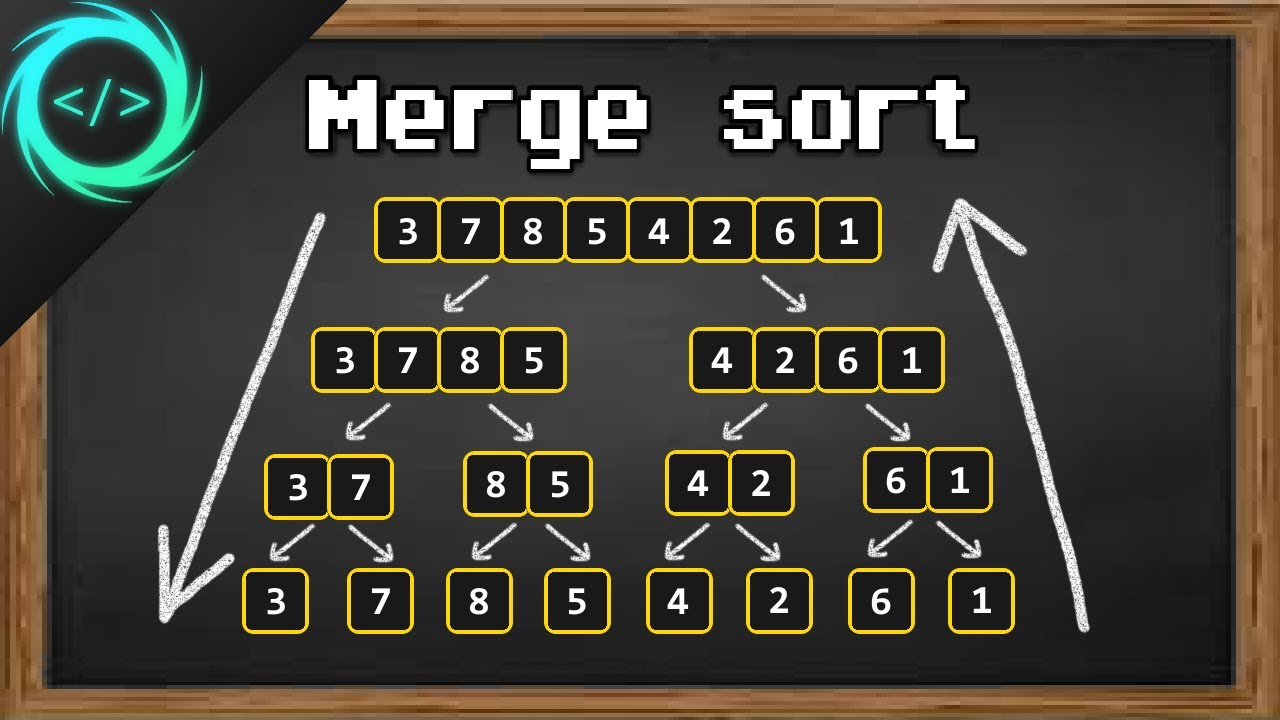
Learn Merge Sort in 13 minutes 🔪

Sorting - Part 1 | Selection Sort, Bubble Sort, Insertion Sort | Strivers A2Z DSA Course
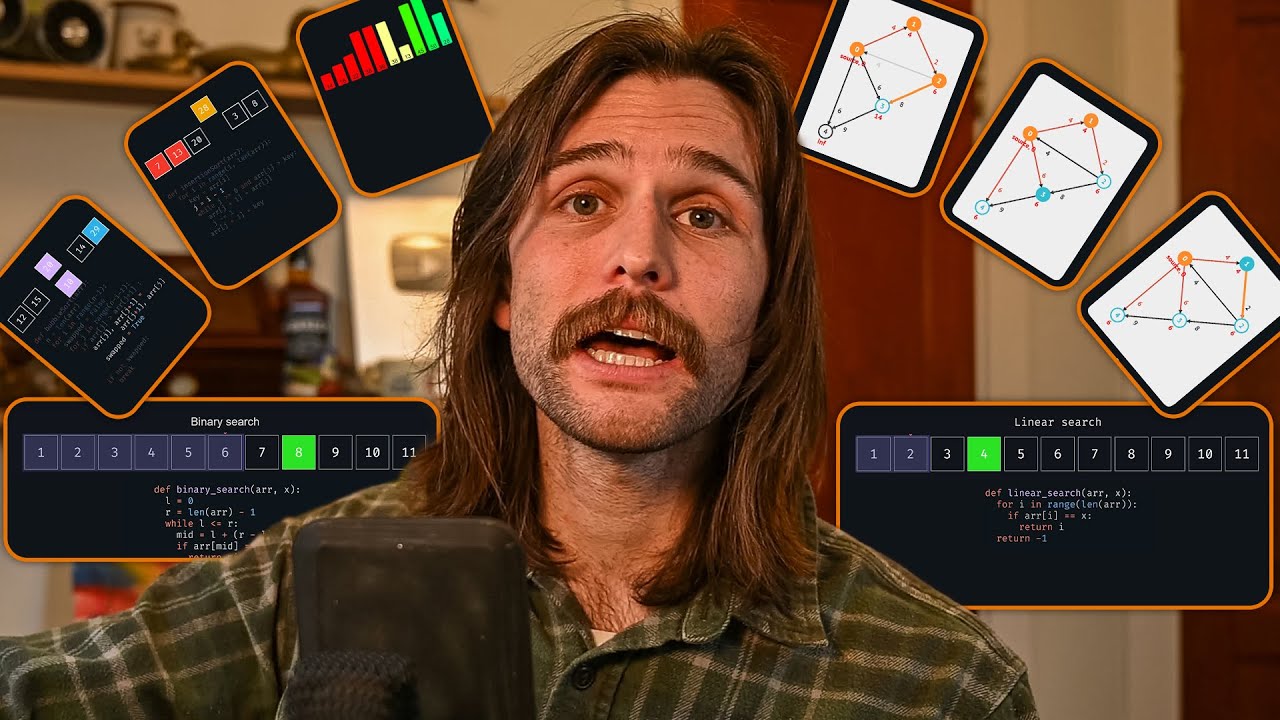
3 Types of Algorithms Every Programmer Needs to Know
5.0 / 5 (0 votes)