Programming BASIC and Sorting - Computerphile
Summary
TLDRThis script explores the auditory and visual representation of sorting algorithms, focusing on the BBC Micro's A5000 computer. It discusses the use of BBC Basic to create a program that visually and audibly sorts elements, playing sounds to represent the values during the sorting process. The script compares various sorting algorithms like Bubble Sort, Quick Sort, and Heap Sort, demonstrating their efficiency and differences. It also touches on the use of libraries and the challenges of implementing certain algorithms due to outdated dependencies.
Takeaways
- 🔊 The script describes a sorting algorithm visualization where elements are swapped with accompanying sounds to represent their values.
- 📈 It was suggested to sort 100 elements side by side to visually compare different sorting algorithms.
- 💻 The BBC Micro and its descendant, the A5000, are discussed as early computers that introduced people to programming with BASIC.
- 🎵 The Acorn A5000 computer is highlighted for its built-in BBC BASIC interpreter and its ability to quickly boot into a GUI with an icon bar and hard drive.
- 👀 A program written in C++ for visualizing sorting algorithms was mentioned, which inspired the speaker to create their own implementation.
- 🛠️ The speaker discusses the use of libraries in programming, which are collections of code that perform specific tasks, often used for reliability.
- 📚 Reference is made to a book titled 'Games for your BBC Micro' which provided insights on how to use sound in programming.
- 🔢 A demonstration of the 'Doctor Audio' game from the book is given, explaining how sound is used to provide feedback based on the proximity of a guess to a number.
- 📉 The script covers various sorting algorithms, including Bubble Sort, Quick Sort, Selection Sort, and Heapsort, noting their complexities and performance.
- ⏱️ Performance metrics such as time taken, number of comparisons, and swaps are highlighted as important factors in evaluating sorting algorithms.
- 📊 A visual comparison of sorting algorithms is conducted with 100 elements to demonstrate the differences in speed and efficiency.
Q & A
What is the purpose of the sound played when swapping elements in a sorting algorithm?
-The sound is played to provide an auditory cue that a swap has occurred, with the pitch of the sound indicating the values of the elements being swapped.
Why was the BBC Micro commissioned and by whom?
-The BBC Micro was commissioned by the BBC to introduce people to computers and programming as part of their educational project.
What is the significance of the ARM chip in the Acorn A5000?
-The ARM chip is significant as it was a feature not present in the original BBC Micro, indicating an advancement in technology and performance.
What is the difference between the BBC Micro and the Acorn A5000 in terms of boot-up time?
-The Acorn A5000 boots up more quickly than the BBC Micro because its operating system is stored in ROM, allowing for a faster start-up process.
What is the role of the 'Icon Bar' in the Acorn A5000's GUI?
-The Icon Bar in the Acorn A5000's GUI provides quick access to various applications and system functions, similar to taskbars in modern operating systems.
How does the 'Doctor Audio' game provide feedback on the player's guess?
-In 'Doctor Audio', feedback is given as a tone, with the pitch of the note varying according to how close the player's guess is to the computer's number.
What is the role of the 'rem' command in BBC Basic?
-The 'rem' command in BBC Basic is used to insert comments or reminders in the code, which are ignored during execution.
What is the time complexity of Bubble Sort and why is it considered inefficient for large datasets?
-Bubble Sort has a time complexity of O(n^2), making it inefficient for large datasets because the number of comparisons and swaps grows quadratically with the size of the input.
How does Quick Sort differ from Bubble Sort in terms of efficiency?
-Quick Sort has a time complexity of O(n log n), making it significantly faster than Bubble Sort for larger datasets due to its divide-and-conquer approach.
What is the concept of a 'heap' in the context of Heap Sort?
-A 'heap' in Heap Sort is a specialized tree-based data structure where every parent node is larger than its children, allowing for efficient extraction of the largest element.
How does the Selection Sort algorithm find the smallest element in an unsorted list?
-Selection Sort works by repeatedly scanning the unsorted section of the list to find the smallest element and then swapping it with the first unsorted element, moving the boundary of the sorted and unsorted sections each time.
Outlines
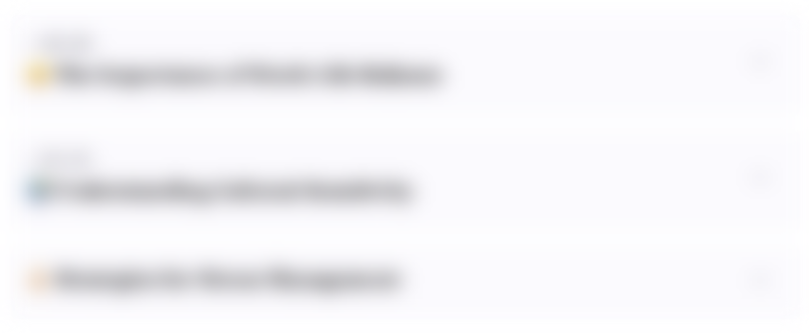
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
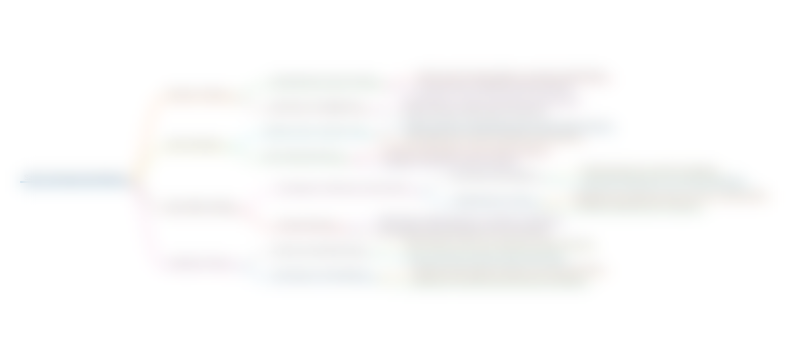
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
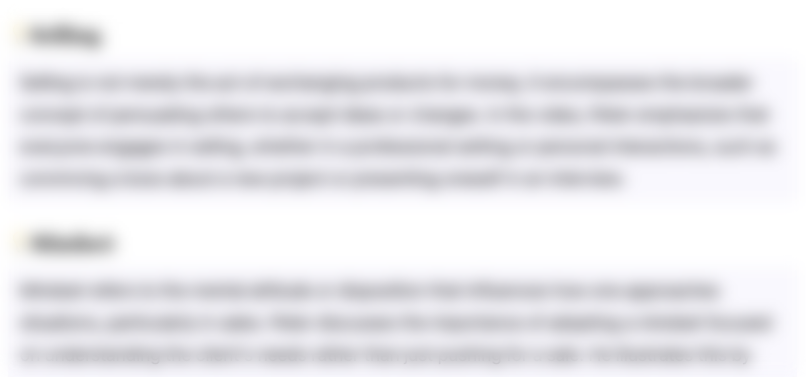
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
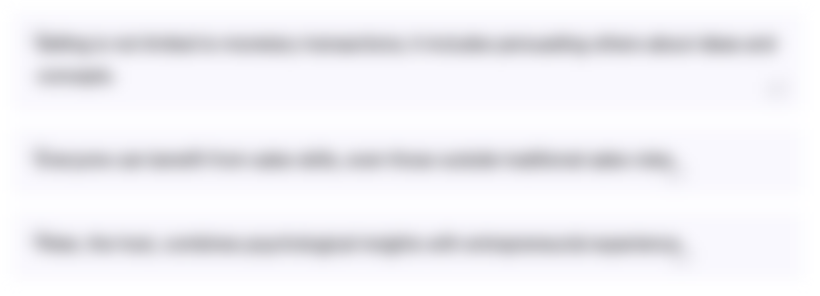
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
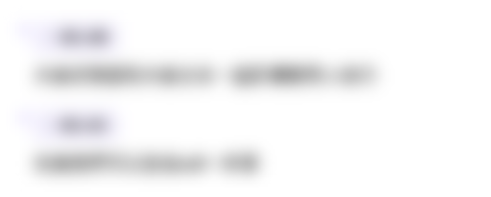
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen
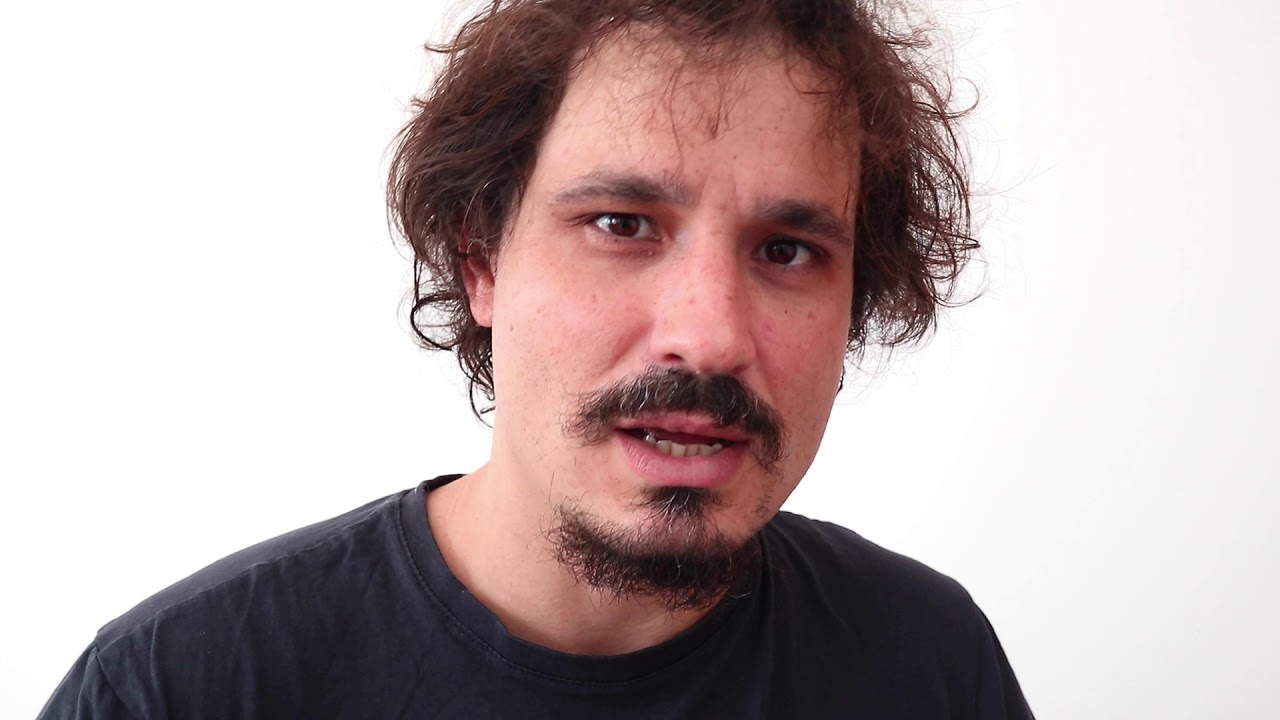
EDB1 IMD UFRN : Introdução a TAD Sequência ED Vetor
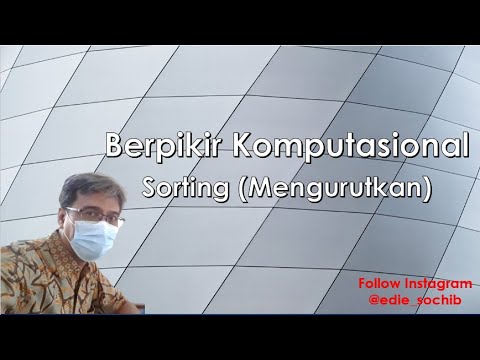
Berpikir Komputasional - Mengurutkan (Sorting), Tumpukan (Stack), dan Antrian (Queue)
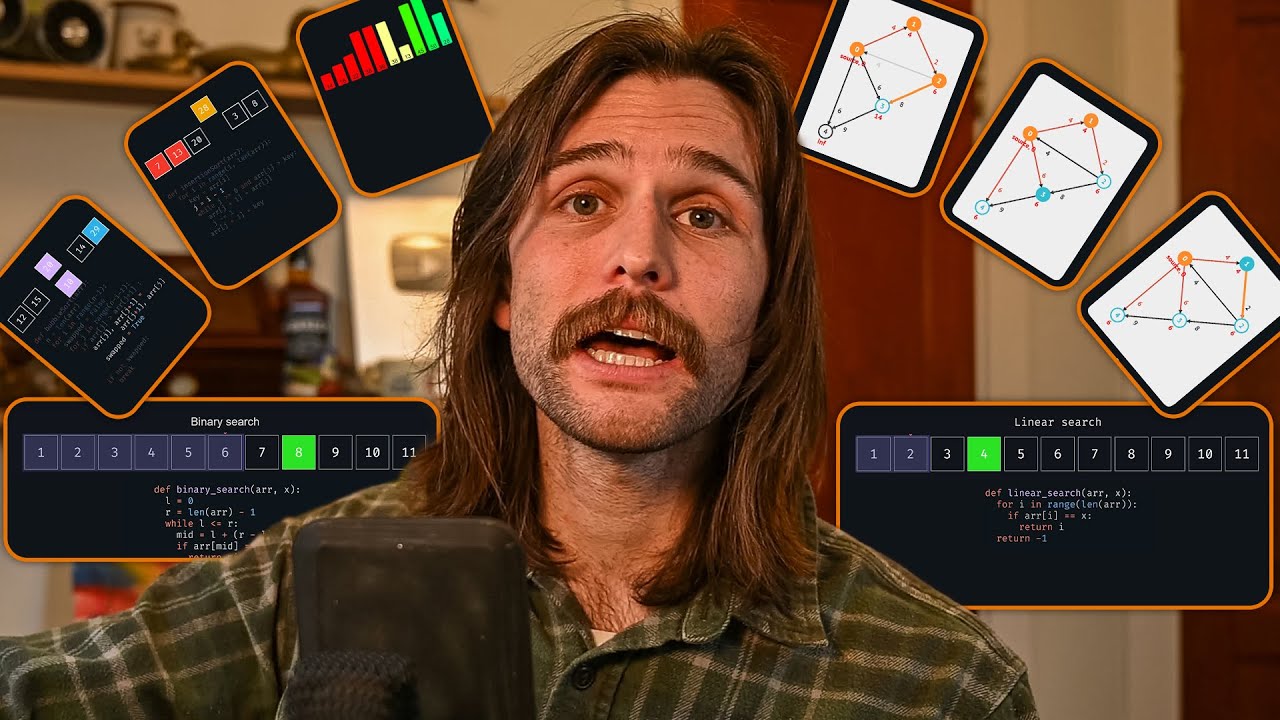
3 Types of Algorithms Every Programmer Needs to Know

EDB1 IMD UFRN (2020.6): Problema de Ordenação

Sorting Secret - Computerphile
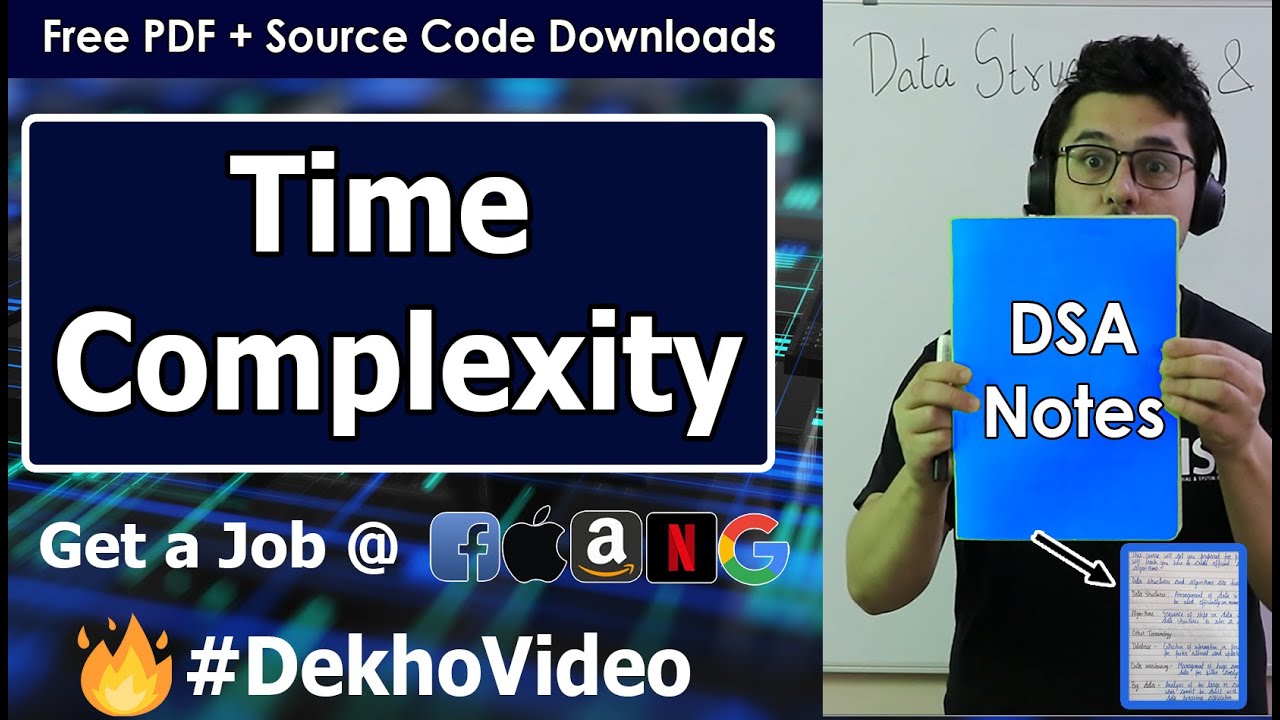
Time Complexity and Big O Notation (with notes)
5.0 / 5 (0 votes)