Dereferencing in C
Summary
TLDRThis video script delves into the concepts of referencing and dereferencing operators, as well as the array subscript operator in C programming. It explains how to create a pointer to a variable, use the dereference operator to access and modify the value at a memory address, and clarifies the distinction between the dereference operator and the type definition. The script also explores the array subscript operator, demonstrating its equivalence to pointer arithmetic and the peculiarities of its commutative property. The tutorial aims to demystify pointers and their operations for viewers, encouraging questions and further discussion.
Takeaways
- 📌 The dereferencing operator is used to access the value at the memory address a pointer is referencing, as opposed to the address itself.
- 🔍 To dereference a pointer in C, you use the * operator, which tells the compiler to get the value stored at the location the pointer is pointing to.
- 💡 The address-of operator (&) is used to obtain the memory address of a variable, which can then be assigned to a pointer.
- 👉 When defining a pointer, the * symbol is part of the type declaration, indicating that the variable is a pointer to the specified type.
- 🛠 The dereference operator can also be used to modify the value at the memory address a pointer is pointing to, by assigning a new value to it.
- 🚫 Attempting to dereference a pointer that points to an invalid or uninitialized address can lead to a runtime error, such as a read access violation.
- 🔄 The array subscript operator [] is essentially a shorthand for pointer arithmetic and dereferencing, allowing for easy access to array elements.
- 🔢 In C, arrays decay into pointers to their first element, which means you can use pointer arithmetic to access other elements in the array.
- 🤔 The commutative property of addition can lead to some unusual expressions, like 1 + array, which may seem strange but is valid in C due to how the array subscript operator works.
- 📚 Understanding the difference between l-values and r-values in C is important when working with pointers and dereferencing, as it affects what operations can be performed.
- 🗣️ The video encourages viewers to ask questions about pointers or any related topics on the comments section or the discord server for further clarification.
Q & A
What is the purpose of the dereference operator in C?
-The dereference operator in C is used to access the value stored at a memory address pointed to by a pointer. It allows you to get the value at that address rather than the address itself.
How do you create a pointer to a variable in C?
-You create a pointer to a variable in C by declaring a pointer of the same type as the variable and then assigning it the address of the variable using the address-of operator (&).
What is the difference between the dereference operator (*) and the type specifier (*) when defining a pointer?
-The dereference operator (*) is used to access the value at the memory address a pointer is pointing to. The type specifier (*) when defining a pointer indicates the type of data the pointer points to, such as an int pointer.
How can you print the value of a variable using its pointer in C?
-You can print the value of a variable using its pointer by using the dereference operator (*) with the printf function and the appropriate format specifier, such as %d for integers.
What happens if you try to dereference a pointer without first assigning it to a valid address?
-Attempting to dereference a pointer without first assigning it to a valid address can result in undefined behavior, which often leads to a runtime error such as a segmentation fault or access violation.
How can you change the value at the memory address pointed to by a pointer?
-You can change the value at the memory address pointed to by a pointer by using the dereference operator (*) and assigning a new value to it, like so: *pointer_name = new_value;
What is the relationship between the array subscript operator and pointers in C?
-The array subscript operator in C is essentially a shorthand for adding an offset to a pointer and then dereferencing it. It decays the array into a pointer to its first element and allows access to elements at specific offsets.
Why does the expression '1 + pointer' sometimes yield the same result as 'pointer + 1' in C?
-The expression '1 + pointer' and 'pointer + 1' can yield the same result in C because addition is commutative. The array subscript operator adds the index to the pointer and then dereferences it, which is the same as what happens when you add 1 to the pointer first and then dereference.
What is the significance of the term 'L-values' in the context of pointers and dereferencing in C?
-In C, 'L-values' refer to expressions that can appear on the left side of an assignment. When discussing pointers, an L-value is necessary to use the dereference operator to modify the value at the memory address pointed to by the pointer.
Can you use the dereference operator to access the first element of an array without using the array subscript operator?
-Yes, you can use the dereference operator to access the first element of an array by dereferencing the pointer to the array, which is equivalent to using the array subscript operator with index 0.
What is the potential issue with using '1 + pointer' instead of 'pointer + 1' in code?
-While '1 + pointer' and 'pointer + 1' can yield the same result due to the commutative property of addition, using '1 + pointer' can be less clear and may lead to confusion or errors, especially when the context is not immediately obvious.
Outlines
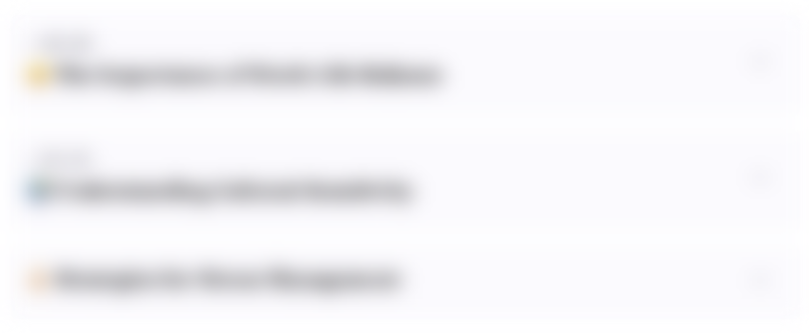
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
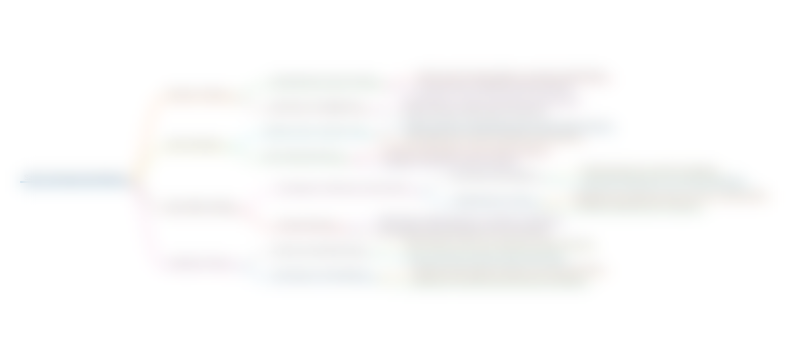
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
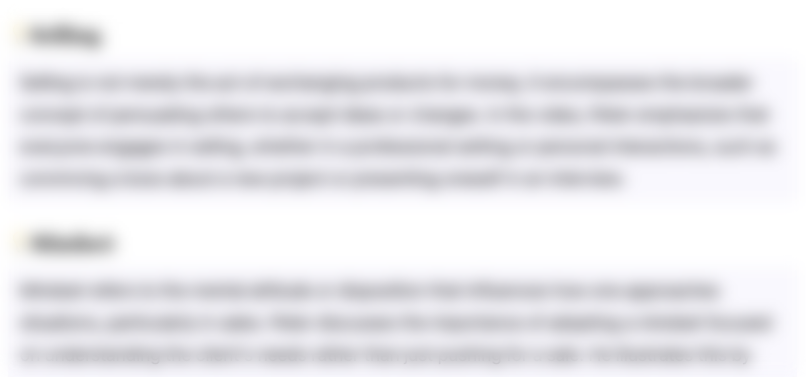
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
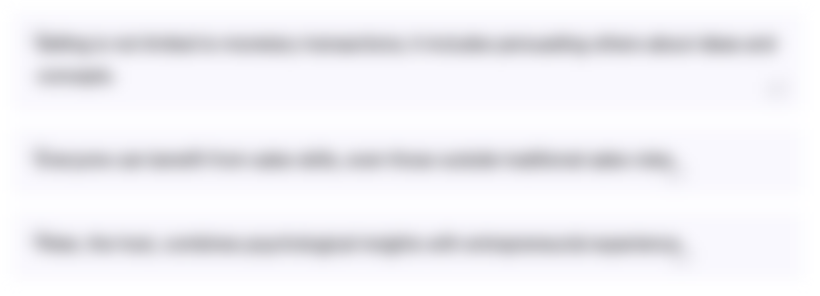
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
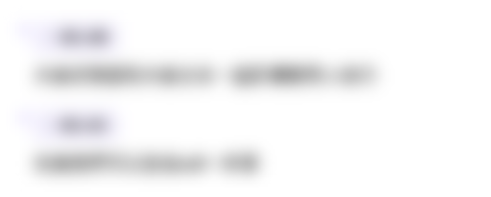
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen
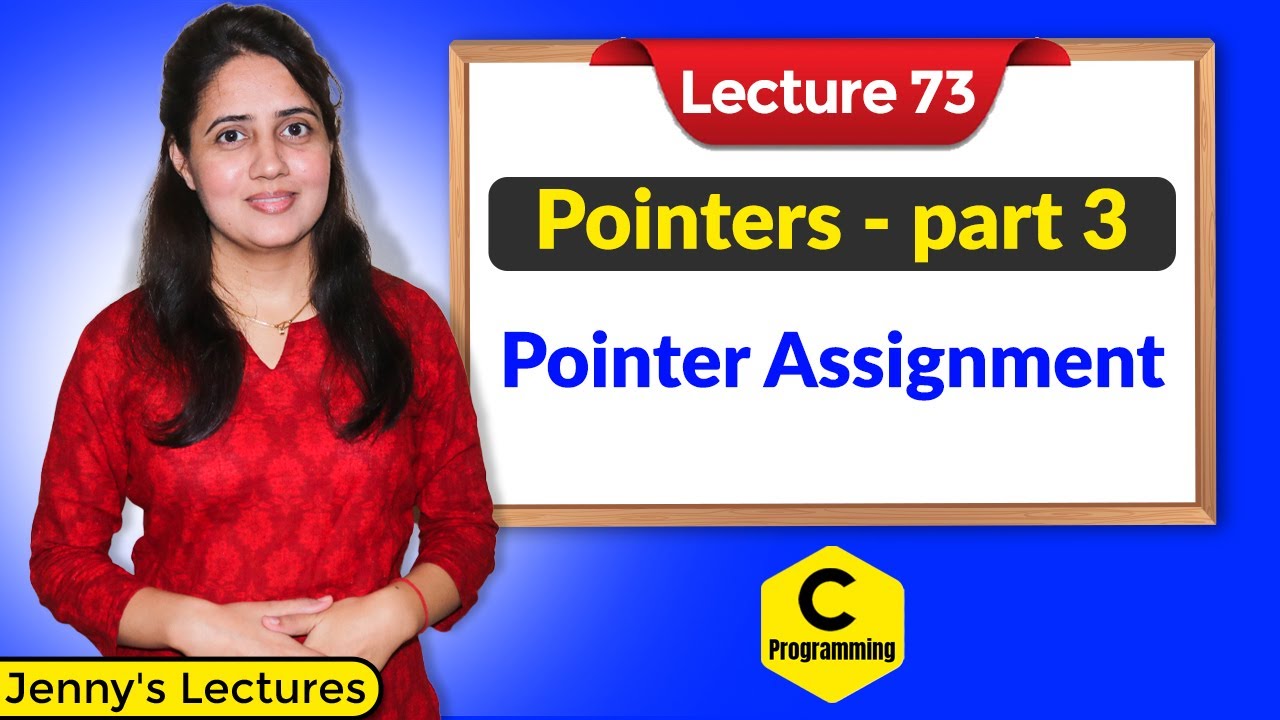
C_73 Pointers in C- part 3 | Pointer Assignment
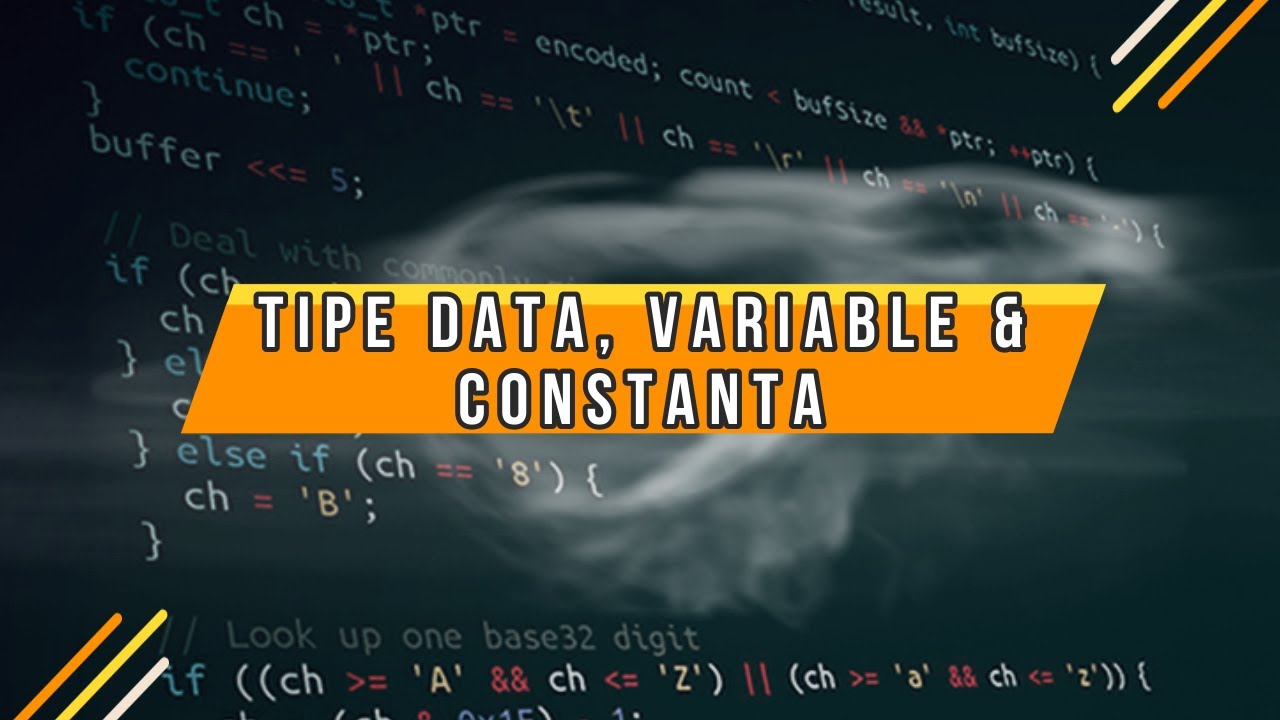
Pemrograman Dasar - Tipe Data, Variable & Constanta

C_13 Operators in C - Part 1 | Unary , Binary and Ternary Operators in C | C programming Tutorials
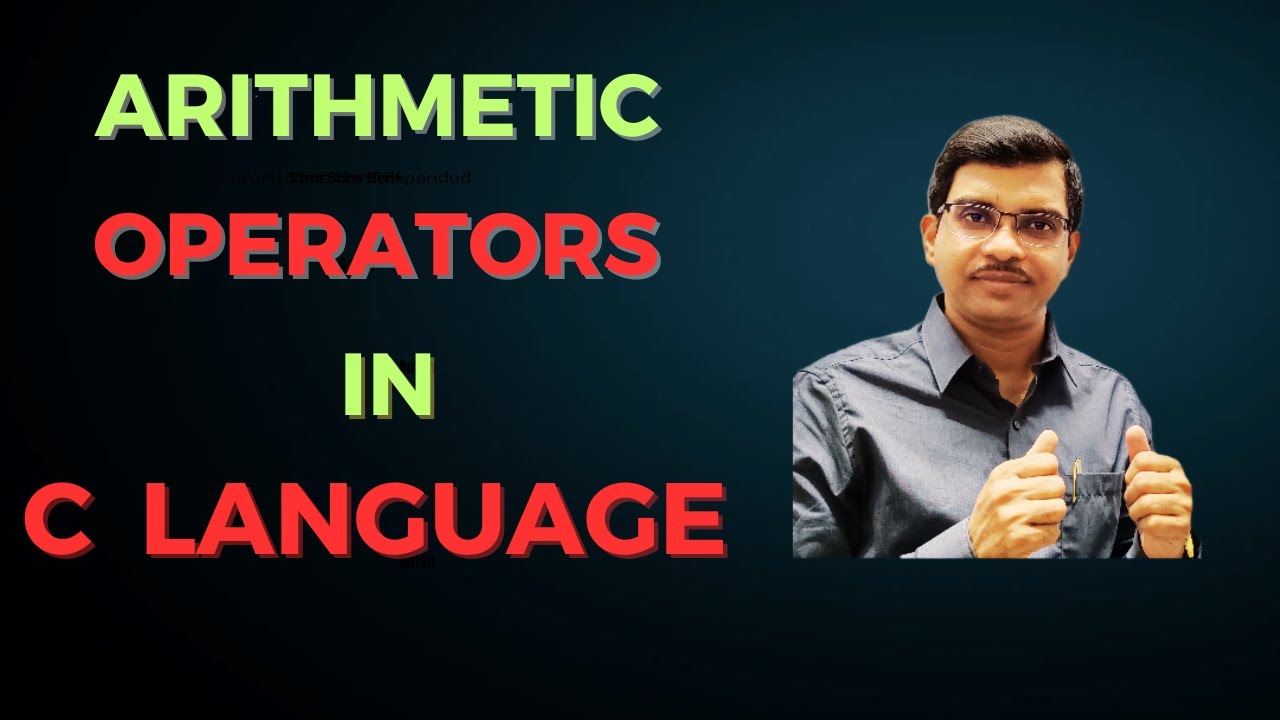
C_12 Arithmetic Operators in C Language | C Programming Tutorials

std::move and the Move Assignment Operator in C++

C_15 Operators in C - Part 3 | C Programming Tutorials
5.0 / 5 (0 votes)